diff --git a/.eslintignore b/.eslintignore
index a3137ad2..fa5eef33 100644
--- a/.eslintignore
+++ b/.eslintignore
@@ -1,4 +1,3 @@
**/node_modules/
node_modules/
-
**/builds/
diff --git a/.eslintrc b/.eslintrc
index 7ed17151..413dd97a 100644
--- a/.eslintrc
+++ b/.eslintrc
@@ -1,23 +1,20 @@
{
+ "root": true,
"env": {
"es6": true,
"browser": true,
"node": true
},
"parserOptions": {
- "ecmaVersion": 2017,
+ "ecmaVersion": 2018,
"sourceType": "module",
- "ecmaFeatures": { }
+ "ecmaFeatures": {}
},
- "plugins": [ "jsdoc" ],
- "extends": [
- "plugin:compat/recommended",
- "plugin:jsdoc/recommended"
- ],
+ "extends": ["plugin:compat/recommended", "plugin:regexp/recommended"],
"rules": {
- "semi": [ "warn", "never" ],
- "indent": [ "error", 2 ],
- "spaced-comment": [ "error", "never" ],
+ "semi": ["warn", "never"],
+ "indent": ["error", 2],
+ "spaced-comment": [0, "never"],
"no-cond-assign": 2,
"no-var": 1,
"prefer-const": 0,
@@ -30,12 +27,7 @@
"no-native-reassign": 2,
"no-redeclare": 2,
"radix": 1,
- "quotes": [
-
- 0,
- "single",
- "avoid-escape"
- ],
+ "quotes": [0, "single", "avoid-escape"],
"no-shadow": 2,
"no-unused-vars": 1,
"no-lonely-if": 1,
@@ -47,19 +39,18 @@
"no-octal-escape": 2,
"no-constant-condition": 1,
"no-unused-expressions": 2,
- "comma-dangle": [
- 1,
- "only-multiline"
- ],
+ "comma-dangle": [1, "only-multiline"],
"space-before-function-paren": [
- "error", {
+ "error",
+ {
"anonymous": "always",
"named": "never",
"asyncArrow": "always"
}
],
"max-len": [
- "error", {
+ "error",
+ {
"code": 120,
"ignoreComments": true,
"ignoreStrings": true,
@@ -67,17 +58,7 @@
"ignoreRegExpLiterals": true
}
],
- "require-jsdoc": [
- 1,
- {
- "require": {
- "ArrowFunctionExpression": false,
- "ClassDeclaration": true,
- "FunctionDeclaration": true,
- "FunctionExpression": true,
- "MethodDefinition": true
- }
- }
- ]
+ "regexp/prefer-d": 0,
+ "regexp/prefer-w": 0
}
}
diff --git a/.github/workflows/build-and-test.yml b/.github/workflows/build-and-test.yml
index 1b08832a..3da03794 100644
--- a/.github/workflows/build-and-test.yml
+++ b/.github/workflows/build-and-test.yml
@@ -8,7 +8,7 @@ jobs:
strategy:
matrix:
- node-version: [10.x, 13.x]
+ node-version: [10.x, 15.x]
steps:
- uses: actions/checkout@v2
@@ -27,11 +27,12 @@ jobs:
${{ runner.os }}-npm-${{ matrix.node-version }}-
${{ runner.os }}-npm-
- - name: npm install, build, and test
+ - name: npm install, build, lint, and test
run: |
npm ci
npm run build
- npm run testb
+ npm run lint
+ npm run test:build
npm run test:fetch
env:
CI: true
diff --git a/.github/workflows/lint.yml b/.github/workflows/lint.yml
deleted file mode 100644
index 1b3b603e..00000000
--- a/.github/workflows/lint.yml
+++ /dev/null
@@ -1,14 +0,0 @@
-name: Lint
-
-on:
- push:
- branches: [ master ]
- pull_request:
- branches: [ master ]
-
-jobs:
- eslint:
- runs-on: ubuntu-latest
- steps:
- - uses: actions/checkout@v2
- - uses: bradennapier/eslint-plus-action@v3.4.2
diff --git a/.gitignore b/.gitignore
index f31400ec..270673c7 100644
--- a/.gitignore
+++ b/.gitignore
@@ -1,6 +1,5 @@
node_modules
.git
-viz
coverage
coverage.lcov
codecov.yml
@@ -8,3 +7,4 @@ npm-debug.log
.nyc_output
*.DS_Store
*.tsv
+_missing_templates.json
\ No newline at end of file
diff --git a/README.md b/README.md
index 54ebc378..41d4c94c 100644
--- a/README.md
+++ b/README.md
@@ -50,26 +50,26 @@
-
-
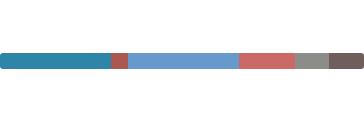
-
```js
const wtf = require('wtf_wikipedia')
wtf.fetch('Toronto Raptors').then((doc) => {
- doc.sentences(0).text()
- //'The Toronto Raptors are a Canadian professional basketball team ...'
-
let coach = doc.infobox().get('coach')
coach.text() //'Nick Nurse'
+
+ doc.sentences()[0].text()
+ //'The Toronto Raptors are a Canadian professional basketball team ...'
})
```
+
+
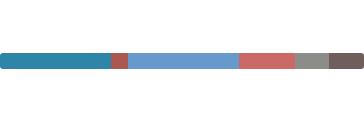
+
-## .text
+## .text()
get clean plaintext:
@@ -92,7 +92,7 @@ doc.text()
-## .json
+## .json()
get all the data from a page:
@@ -103,7 +103,7 @@ doc.json()
// { categories: ['Oral communication', 'Vocal skills'], sections: [{ title: 'Techniques' }], ...}
```
-the default json output is [really verbose](https://observablehq.com/@spencermountain/wtf-wikipedia-json), but you can cherry-pick things like this:
+the default .json() output is _[really verbose](https://observablehq.com/@spencermountain/wtf-wikipedia-json)_, but you can cherry-pick data by poking-around like this:
```js
// get just the links:
@@ -111,11 +111,11 @@ doc.links().map((link) => link.json())
//[{ page: 'Theatrical superstitions', text: 'supersitions' }]
// just the images:
-doc.images(0).json()
+doc.images()[0].json()
// { file: 'Image:Duveneck Whistling Boy.jpg', url: 'https://commons.wiki...' }
// json for a particular section:
-doc.sections('see also').links(0).json()
+doc.section('see also').link().json()
// { page: 'Slide Whistle' }
```
@@ -134,8 +134,7 @@ run it on the client-side:
```html
```
-or from Deno/typescript/webpack:
+or in Deno/typescript/webpack:
```js
import spacetime from 'https://unpkg.com/spacetime/builds/spacetime.mjs'
@@ -197,22 +196,20 @@ these add all sorts of new functionality:
```js
wtf.extend(require('wtf-plugin-classify'))
-wtf.fetch('Toronto Raptors').then((doc) => doc.classify())
+await wtf.fetch('Toronto Raptors').classify()
// 'Organization/SportsTeam'
wtf.extend(require('wtf-plugin-summary'))
-wtf.fetch('Pulp Fiction').then((doc) => doc.summary())
+await wtf.fetch('Pulp Fiction').summary()
// 'a 1994 American crime film'
wtf.extend(require('wtf-plugin-person'))
-wtf.fetch('David Bowie').then((doc) => doc.birthDate())
+await wtf.fetch('David Bowie').birthDate()
// {year:1947, date:8, month:1}
wtf.extend(require('wtf-plugin-i18n'))
-wtf.fetch('Ziggy Stardust', 'fr').then((doc) => {
- doc.infobox().json()
- //{ nom:{text:"Ziggy Stardust"}, oeuvre:{text:"The Rise and Fall of Ziggy Stardust"} }
-})
+await wtf.fetch('Ziggy Stardust', 'fr').infobox().json()
+// {nom:{text:"Ziggy Stardust"}, oeuvre:{text:"The Rise and Fall of Ziggy Stardust"}}
```
| **Plugin** | |
@@ -220,7 +217,7 @@ wtf.fetch('Ziggy Stardust', 'fr').then((doc) => {
| [classify](./plugins/classify) | person/place/thing |
| [summary](./plugins/summary) | short description text |
| [person](./plugins/person) | birth/death information |
-| [category](./plugins/category) | parse all articles in a category |
+| [api](./plugins/api) | fetch more data from the API |
| [i18n](./plugins/i18n) | improves multilingual template coverage |
| [wtf-mlb](https://github.com/spencermountain/wtf-mlb) | fetch baseball data |
| [wtf-nhl](https://github.com/spencermountain/wtf-nhl) | fetch hockey data |
@@ -323,7 +320,7 @@ import wtf from 'wtf_wikipedia'
let txt = `
==Wood in Popular Culture==
* harry potter's wand
-* the simpsons fence
+* the simpson's fence
`
wtf(txt)
// Document {text(), json(), lists()...}
@@ -332,9 +329,10 @@ wtf(txt)
#### **doc.links()**
```javascript
-let str = `Whistling is featured in a number of television shows, such as [[Lassie (1954 TV series)|''Lassie'']], and the title theme for ''[[The X-Files]]''.`
-let doc = wtf(str)
-doc.links().map((l) => l.page())
+let txt = `Whistling is featured in a number of television shows, such as [[Lassie (1954 TV series)|''Lassie'']], and the title theme for ''[[The X-Files]]''.`
+wtf(txt)
+ .links()
+ .map((l) => l.page())
// [ 'Lassie (1954 TV series)', 'The X-Files' ]
```
@@ -343,9 +341,9 @@ doc.links().map((l) => l.page())
returns nice plain-text of the article
```js
-var wiki =
+var txt =
"[[Greater_Boston|Boston]]'s [[Fenway_Park|baseball field]] has a {{convert|37|ft}} wall.[{{cite web|blah}}]"
-var text = wtf(wiki).text()
+wtf(txt).text()
//"Boston's baseball field has a 37ft wall."
```
@@ -354,14 +352,14 @@ var text = wtf(wiki).text()
a section is a heading _'==Like This=='_
```js
-wtf(page).sections(1).children() //traverse nested sections
-wtf(page).sections('see also').remove() //delete one
+wtf(page).sections()[1].children() //traverse nested sections
+wtf(page).section('see also').remove() //delete one
```
#### **doc.sentences()**
```js
-s = wtf(page).sentences(4)
+let s = wtf(page).sentences()[4]
s.links()
s.bolds()
s.italics()
@@ -370,15 +368,14 @@ s.italics()
#### **doc.categories()**
```js
-let doc = await wtf.fetch('Whistling')
-doc.categories()
+await wtf.fetch('Whistling').categories()
//['Oral communication', 'Vocal music', 'Vocal skills']
```
#### **doc.images()**
```js
-img = wtf(page).images(0)
+let img = wtf(page).images()[0]
img.url() // the full-size wikimedia-hosted url
img.thumbnail() // 300px, by default
img.format() // jpg, png, ..
@@ -389,7 +386,7 @@ img.format() // jpg, png, ..
## Fetch
-This library can grab, and automatically-parse articles from [any wikimedia api](https://www.mediawiki.org/wiki/API:Main_page).
+You can grab and parse articles from _[any wiki api](https://www.mediawiki.org/wiki/API:Main_page)_.
This includes any language, any wiki-project, and most **3rd-party wikis**.
```js
@@ -398,14 +395,14 @@ let doc = await wtf.fetch('https://muppet.fandom.com/wiki/Miss_Piggy')
// wikipedia français
doc = await wtf.fetch('Tony Hawk', 'fr')
-doc.sentences(0).text() // 'Tony Hawk est un skateboarder professionnel et un acteur ...'
+doc.sentence().text() // 'Tony Hplawk est un skateboarder professionnel et un acteur ...'
// accept an array, or wikimedia pageIDs
let docs = wtf.fetch(['Whistling', 2983], { follow_redirects: false })
// article from german wikivoyage
wtf.fetch('Toronto', { lang: 'de', wiki: 'wikivoyage' }).then((doc) => {
- console.log(doc.sentences(0).text()) // 'Toronto ist die Hauptstadt der Provinz Ontario'
+ console.log(doc.sentences()[0].text()) // 'Toronto ist die Hauptstadt der Provinz Ontario'
})
```
@@ -417,35 +414,37 @@ let doc = await wtf.fetch(64646, 'de')
the fetch method follows redirects.
-### fetch categories:
+### API plugin
**wtf.category(title, [lang], [options | callback])**
retrieves all pages and sub-categories belonging to a given category:
```js
+wtf.extend(require('wtf-plugin-api'))
let result = await wtf.category('Category:Politicians_from_Paris')
-//{
-// pages: [{title: 'Paul Bacon', pageid: 1266127 }, ...],
-// categories: [ {title: 'Category:Mayors of Paris' } ]
-//}
+/*
+{
+ pages: [{title: 'Paul Bacon', pageid: 1266127 }, ...],
+ categories: [ {title: 'Category:Mayors of Paris' } ]
+}
+*/
```
-to fetch and parse all pages in a category, in an optimized way, see [wtf-plugin-category](./plugins/category)
-
-### fetch random article:
-
**wtf.random([lang], [options], [callback])**
fetches a random wikipedia article, from a given language or domain
```js
+wtf.extend(require('wtf-plugin-api'))
wtf.random().then((doc) => {
console.log(doc.title(), doc.categories())
//'Whistling' ['Oral communication', 'Vocal skills']
})
```
+see [wtf-plugin-api](./plugins/api)
+
### Good practice:
The wikipedia api is [pretty welcoming](https://www.mediawiki.org/wiki/API:Etiquette#Request_limit) though recommends three things, if you're going to hit it heavily -
@@ -482,62 +481,66 @@ wtf
- **.isRedirect()** - if the page is just a redirect to another page
- **.redirectTo()** - the page this redirects to
- **.isDisambiguation()** - is this a placeholder page to direct you to one-of-many possible pages
-- **.categories()** -
-- **.sections()** - return a list, or given-index of the Document's sections
-- **.paragraphs()** - return a list, or given-index of Paragraphs, in all sections
-- **.sentences()** - return a list, or given-index of all sentences in the document
-- **.images()** -
-- **.links()** - return a list, or given-index of all links, in all parts of the document
+- **.categories()** - return all categories of the document
+- **.sections()** - return a list of the Document's sections
+- **.paragraphs()** - return a list of Paragraphs, in all sections
+- **.sentences()** - return a list of all sentences in the document
+- **.images()** - return all images found in the document
+- **.links()** - return a list of all links, in all parts of the document
- **.lists()** - sections in a page where each line begins with a bullet point
-- **.tables()** - return a list, or given-index of all structured tables in the document
+- **.tables()** - return a list of all structured tables in the document
- **.templates()** - any type of structured-data elements, typically wrapped in like {{this}}
- **.infoboxes()** - specific type of template, that appear on the top-right of the page
-- **.references()** - return a list, or given-index of 'citations' in the document
+- **.references()** - return a list of 'citations' in the document
- **.coordinates()** - geo-locations that appear on the page
- **.text()** - plaintext, human-readable output for the page
- **.json()** - a 'stringifyable' output of the page's main data
+- **.wikitext()** - original wiki markup
### Section
- **.title()** - the name of the section, between ==these tags==
- **.index()** - which number section is this, in the whole document.
- **.indentation()** - how many steps deep into the table of contents it is
-- **.sentences()** - return a list, or given-index, of sentences in this section
-- **.paragraphs()** - return a list, or given-index, of paragraphs in this section
-- **.links()** -
-- **.tables()** -
-- **.templates()** -
-- **.infoboxes()** -
-- **.coordinates()** -
-- **.lists()** -
+- **.sentences()** - return a list of sentences in this section
+- **.paragraphs()** - return a list of paragraphs in this section
+- **.links()** - list of all links, in all paragraphs and templates
+- **.tables()** - list of all html tables
+- **.templates()** - list of all templates in this section
+- **.infoboxes()** - list of all infoboxes found in this section
+- **.coordinates()** - list of all coordinate templates found in this section
+- **.lists()** - list of all lists in this section
- **.interwiki()** - any links to other language wikis
-- **.images()** - return a list, or given index, of any images in this section
-- **.references()** - return a list, or given index, of 'citations' in this section
+- **.images()** - return a list of any images in this section
+- **.references()** - return a list of 'citations' in this section
- **.remove()** - remove the current section from the document
- **.nextSibling()** - a section following this one, under the current parent: eg. 1920s → 1930s
- **.lastSibling()** - a section before this one, under the current parent: eg. 1930s → 1920s
- **.children()** - any sections more specific than this one: eg. History → [PreHistory, 1920s, 1930s]
- **.parent()** - the section, broader than this one: eg. 1920s → History
-- **.text()** -
-- **.json()** -
+- **.text()** - readable plaintext for this section
+- **.json()** - return all section data
+- **.wikitext()** - original wiki markup
### Paragraph
-- **.sentences()** -
-- **.references()** -
-- **.lists()** -
-- **.images()** -
-- **.links()** -
-- **.interwiki()** -
+- **.sentences()** - return a list of sentence objects in this paragraph
+- **.references()** - any citations, or references in all sentences
+- **.lists()** - any lists found in this paragraph
+- **.images()** - any images found in this paragraph
+- **.links()** - list of all links in all sentences
+- **.interwiki()** - any links to other language wikis
- **.text()** - generate readable plaintext for this paragraph
- **.json()** - generate some generic data for this paragraph in JSON format
+- **.wikitext()** - original wiki markup
### Sentence
-- **.links()** -
-- **.bolds()** -
-- **.italics()** -
-- **.json()** -
+- **.links()** - list of all links
+- **.bolds()** - list of all bold texts
+- **.italics()** - list of all italic formatted text
+- **.json()** - return all sentence data
+- **.wikitext()** - original wiki markup
### Image
@@ -547,16 +550,24 @@ wtf
- **.format()** - get file format (e.g. `jpg`)
- **.json()** - return some generic metadata for this image
- **.text()** - does nothing
+- **.wikitext()** - original wiki markup
+
+### Template
+
+- **.text()** - does this template generate any readable plaintext?
+- **.json()** - get all the data for this template
+- **.wikitext()** - original wiki markup
### Infobox
-- **.links()** -
+- **.links()** - any internal or external links in this infobox
- **.keyValue()** - generate simple key:value strings from this infobox
- **.image()** - grab the main image from this infobox
- **.get()** - lookup properties from their key
- **.template()** - which infobox, eg 'Infobox Person'
- **.text()** - generate readable plaintext for this infobox
- **.json()** - generate some generic 'stringifyable' data for this infobox
+- **.wikitext()** - original wiki markup
### List
@@ -564,6 +575,7 @@ wtf
- **.links()** - get all links mentioned in this list
- **.text()** - generate readable plaintext for this list
- **.json()** - generate some generic easily-parsable data for this list
+- **.wikitext()** - original wiki markup
### Reference
@@ -571,6 +583,7 @@ wtf
- **.links()** - get any links mentioned in this reference
- **.text()** - returns nothing
- **.json()** - generate some generic metadata data for this reference
+- **.wikitext()** - original wiki markup
### Table
@@ -578,6 +591,7 @@ wtf
- **.keyValue()** - generate a simple list of key:value objects for this table
- **.text()** - returns nothing
- **.json()** - generate some useful metadata data for this table
+- **.wikitext()** - original wiki markup
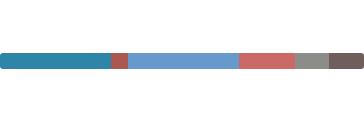
@@ -597,7 +611,7 @@ wtf.extend((models) => {
}
})
-await wtf.fetch('Stephen Harper').isPerson() //hmm?
+await wtf.fetch('Stephen Harper').isPerson()
```
### Adding new templates:
@@ -607,8 +621,9 @@ does your wiki use a `{{foo}}` template? Add a custom parser for it:
```js
wtf.extend((models, templates) => {
// create a custom parser function
- templates.foo = (text, data) => {
- data.templates.push({ name: 'foo', cool: true })
+ templates.foo = (tmpl, list, parse) => {
+ let obj = parse(tmpl) //or do a custom regex
+ list.push(obj)
return 'new-text'
}
@@ -623,6 +638,14 @@ wtf.extend((models, templates) => {
})
```
+you can determine which templates are understood to be 'infoboxes' with the 3rd parameter:
+
+```js
+wtf.extend((models, templates, infoboxes) => {
+ Object.assign(infoboxes, { person: true, place: true, thing: true })
+})
+```
+
@@ -651,7 +674,7 @@ some wikis will change the path of their API, from `./api.php` to elsewhere. If
```js
wtf.fetch('2016-06-04_-_J.Fernandes_@_FIL,_Lisbon', { domain: 'www.mixesdb.com', path: 'db/api.php' }).then((doc) => {
- console.log(doc.templates('player'))
+ console.log(doc.template('player').json())
})
```
diff --git a/builds/wtf_wikipedia-client.js b/builds/wtf_wikipedia-client.js
index 1ce6deb2..e17a0113 100644
--- a/builds/wtf_wikipedia-client.js
+++ b/builds/wtf_wikipedia-client.js
@@ -1,14 +1,71 @@
-/* wtf_wikipedia 8.5.1 MIT */
+/* wtf_wikipedia 9.0.0 MIT */
(function (global, factory) {
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory() :
typeof define === 'function' && define.amd ? define(factory) :
(global = typeof globalThis !== 'undefined' ? globalThis : global || self, global.wtf = factory());
}(this, (function () { 'use strict';
- var parseUrl = function parseUrl(url) {
- var parsed = new URL(url); //eslint-disable-line
+ function require$$0 (e, n) {
+ return n = n || {}, new Promise(function (t, r) {
+ var s = new XMLHttpRequest(),
+ o = [],
+ u = [],
+ i = {},
+ a = function () {
+ return {
+ ok: 2 == (s.status / 100 | 0),
+ statusText: s.statusText,
+ status: s.status,
+ url: s.responseURL,
+ text: function () {
+ return Promise.resolve(s.responseText);
+ },
+ json: function () {
+ return Promise.resolve(s.responseText).then(JSON.parse);
+ },
+ blob: function () {
+ return Promise.resolve(new Blob([s.response]));
+ },
+ clone: a,
+ headers: {
+ keys: function () {
+ return o;
+ },
+ entries: function () {
+ return u;
+ },
+ get: function (e) {
+ return i[e.toLowerCase()];
+ },
+ has: function (e) {
+ return e.toLowerCase() in i;
+ }
+ }
+ };
+ };
+
+ for (var l in s.open(n.method || "get", e, !0), s.onload = function () {
+ s.getAllResponseHeaders().replace(/^(.*?):[^\S\n]*([\s\S]*?)$/gm, function (e, n, t) {
+ o.push(n = n.toLowerCase()), u.push([n, t]), i[n] = i[n] ? i[n] + "," + t : t;
+ }), t(a());
+ }, s.onerror = r, s.withCredentials = "include" == n.credentials, n.headers) s.setRequestHeader(l, n.headers[l]);
+
+ s.send(n.body || null);
+ });
+ }
- var title = parsed.pathname.replace(/^\/(wiki\/)?/, '');
+ var browser = self.fetch || (self.fetch = require$$0.default || require$$0);
+
+ /**
+ * Parses out the domain and title from a url
+ *
+ * @private
+ * @param {string} url The url that will be parsed
+ * @returns {{domain: string, title: string}} The domain and title of a url
+ */
+ const parseUrl = function (url) {
+ let parsed = new URL(url);
+ let title = parsed.pathname.replace(/^\/(wiki\/)?/, '');
title = decodeURIComponent(title);
return {
domain: parsed.host,
@@ -16,85 +73,84 @@
};
};
- var _00ParseUrl = parseUrl;
-
- function _typeof(obj) {
- "@babel/helpers - typeof";
+ var parseUrl_1 = parseUrl;
- if (typeof Symbol === "function" && typeof Symbol.iterator === "symbol") {
- _typeof = function (obj) {
- return typeof obj;
- };
- } else {
- _typeof = function (obj) {
- return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj;
- };
+ /**
+ * capitalizes the input
+ * hello -> Hello
+ * hello there -> Hello there
+ *
+ * @private
+ * @param {string} [str] the string that will be capitalized
+ * @returns {string} the capitalized string
+ */
+ function capitalise(str) {
+ if (str && typeof str === 'string') {
+ return str.charAt(0).toUpperCase() + str.slice(1);
}
- return _typeof(obj);
- }
-
- function _slicedToArray(arr, i) {
- return _arrayWithHoles(arr) || _iterableToArrayLimit(arr, i) || _unsupportedIterableToArray(arr, i) || _nonIterableRest();
- }
-
- function _arrayWithHoles(arr) {
- if (Array.isArray(arr)) return arr;
+ return '';
}
-
- function _iterableToArrayLimit(arr, i) {
- if (typeof Symbol === "undefined" || !(Symbol.iterator in Object(arr))) return;
- var _arr = [];
- var _n = true;
- var _d = false;
- var _e = undefined;
-
- try {
- for (var _i = arr[Symbol.iterator](), _s; !(_n = (_s = _i.next()).done); _n = true) {
- _arr.push(_s.value);
-
- if (i && _arr.length === i) break;
- }
- } catch (err) {
- _d = true;
- _e = err;
- } finally {
- try {
- if (!_n && _i["return"] != null) _i["return"]();
- } finally {
- if (_d) throw _e;
- }
+ /**
+ * trim whitespaces of the ends normalize 2 spaces into one and removes whitespaces before commas
+ *
+ * @private
+ * @param {string} [str] the string that will be processed
+ * @returns {string} the processed string
+ */
+
+
+ function trim_whitespace$1(str) {
+ if (str && typeof str === 'string') {
+ str = str.replace(/^\s\s*/, '');
+ str = str.replace(/\s\s*$/, '');
+ str = str.replace(/ {2}/, ' ');
+ str = str.replace(/\s, /, ', ');
+ return str;
}
- return _arr;
+ return '';
}
+ /**
+ * determines if an variable is an array or not
+ *
+ * @private
+ * @param {*} x the variable that needs to be checked
+ * @returns {boolean} whether the variable is an array
+ */
- function _unsupportedIterableToArray(o, minLen) {
- if (!o) return;
- if (typeof o === "string") return _arrayLikeToArray(o, minLen);
- var n = Object.prototype.toString.call(o).slice(8, -1);
- if (n === "Object" && o.constructor) n = o.constructor.name;
- if (n === "Map" || n === "Set") return Array.from(o);
- if (n === "Arguments" || /^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(n)) return _arrayLikeToArray(o, minLen);
- }
- function _arrayLikeToArray(arr, len) {
- if (len == null || len > arr.length) len = arr.length;
+ function isArray$3(x) {
+ return Object.prototype.toString.call(x) === '[object Array]';
+ }
+ /**
+ * determines if an variable is an object or not
+ *
+ * @private
+ * @param {*} x the variable that needs to be checked
+ * @returns {boolean} whether the variable is an object
+ */
- for (var i = 0, arr2 = new Array(len); i < len; i++) arr2[i] = arr[i];
- return arr2;
+ function isObject$1(x) {
+ return x && Object.prototype.toString.call(x) === '[object Object]';
}
- function _nonIterableRest() {
- throw new TypeError("Invalid attempt to destructure non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.");
- }
+ var helpers = {
+ capitalise,
+ trim_whitespace: trim_whitespace$1,
+ isArray: isArray$3,
+ isObject: isObject$1
+ };
- var isInterWiki = /(wikibooks|wikidata|wikimedia|wikinews|wikipedia|wikiquote|wikisource|wikispecies|wikiversity|wikivoyage|wiktionary|foundation|meta)\.org/;
- var defaults = {
+ const {
+ isArray: isArray$2
+ } = helpers;
+ const isInterWiki = /(wikibooks|wikidata|wikimedia|wikinews|wikipedia|wikiquote|wikisource|wikispecies|wikiversity|wikivoyage|wiktionary|foundation|meta)\.org/;
+ const defaults$c = {
action: 'query',
prop: 'revisions|pageprops',
- //we use the 'revisions' api here, instead of the Raw api, for its CORS-rules..
+ // we use the 'revisions' api here, instead of the Raw api, for its CORS-rules..
rvprop: 'content',
maxlag: 5,
rvslots: 'main',
@@ -102,97 +158,119 @@
format: 'json',
redirects: 'true'
};
+ /**
+ * turns a object into a query string
+ *
+ * @private
+ * @param {Object
} obj
+ * @returns {string} QueryString
+ */
- var toQueryString = function toQueryString(obj) {
- return Object.entries(obj).map(function (_ref) {
- var _ref2 = _slicedToArray(_ref, 2),
- key = _ref2[0],
- value = _ref2[1];
-
- return "".concat(encodeURIComponent(key), "=").concat(encodeURIComponent(value));
- }).join('&');
- };
-
- var isArray = function isArray(arr) {
- return Object.prototype.toString.call(arr) === '[object Array]';
+ const toQueryString = function (obj) {
+ return Object.entries(obj).map(([key, value]) => `${encodeURIComponent(key)}=${encodeURIComponent(value)}`).join('&');
};
+ /**
+ * cleans and prepares the tile by replacing the spaces with underscores (_) and trimming the white spaces of the ends
+ *
+ * @private
+ * @param {string} page the title that needs cleaning
+ * @returns {string} the cleaned title
+ */
- var cleanTitle = function cleanTitle(page) {
- page = page.replace(/ /g, '_');
- page = page.trim(); // page = encodeURIComponent(page)
- return page;
+ const cleanTitle = page => {
+ return page.replace(/ /g, '_').trim();
};
+ /**
+ * generates the url for fetching the pages
+ *
+ * @private
+ * @param {import('.').fetchDefaults} options
+ * @returns {string} the url that can be used to make the fetch
+ */
- var makeUrl = function makeUrl(options) {
- var params = Object.assign({}, defaults); // default url
- var url = "https://".concat(options.lang, ".").concat(options.wiki, ".org/w/api.php?"); // from a 3rd party wiki
+ const makeUrl = function (options) {
+ let params = Object.assign({}, defaults$c); //default url
- options.domain = options.domain || options.wikiUrl; //support old syntax
+ let apiPath = ''; //add support for third party apis
if (options.domain) {
- var path = options.path; //wikimedia api uses ./w/api path. no others do
-
- if (isInterWiki.test(options.domain)) {
- path = 'w/api.php';
- }
-
- url = "https://".concat(options.domain, "/").concat(path, "?");
+ //wikimedia is the only api that uses `/w/api` as its path. other wikis use other paths
+ let path = isInterWiki.test(options.domain) ? 'w/api.php' : options.path;
+ apiPath = `https://${options.domain}/${path}?`;
+ } else if (options.lang && options.wiki) {
+ apiPath = `https://${options.lang}.${options.wiki}.org/w/api.php?`;
+ } else {
+ return '';
}
if (!options.follow_redirects) {
delete params.redirects;
- } // support numerical ids
-
-
- var page = options.title;
-
- if (typeof page === 'number') {
- params.pageids = page; //single pageId
- } else if (isArray(page) && typeof page[0] === 'number') {
- params.pageids = page.join('|'); //pageid array
- } else if (isArray(page) === true) {
- //support array
- params.titles = page.map(cleanTitle).join('|');
+ } //support numerical ids
+
+
+ let title = options.title;
+
+ if (typeof title === 'number') {
+ //single pageId
+ params.pageids = title;
+ } else if (typeof title === 'string') {
+ //single page title
+ params.titles = cleanTitle(title);
+ } else if (title !== undefined && isArray$2(title) && typeof title[0] === 'number') {
+ //pageid array
+ params.pageids = title.join('|');
+ } else if (title !== undefined && isArray$2(title) === true && typeof title[0] === 'string') {
+ //title array
+ params.titles = title.map(cleanTitle).join('|');
} else {
- // single page
- params.titles = cleanTitle(page);
- } // make it!
+ return '';
+ } //make it!
- url += toQueryString(params);
- return url;
+ return `${apiPath}${toQueryString(params)}`;
};
- var _01MakeUrl = makeUrl;
+ var makeUrl_1 = makeUrl;
- //this data-format from mediawiki api is nutso
- var getResult = function getResult(data, options) {
- options = options || {};
- var pages = Object.keys(data.query.pages);
- var docs = pages.map(function (id) {
- var page = data.query.pages[id] || {};
+ /**
+ * parses the media wiki api response to something we can use
+ *
+ * the data-format from mediawiki api is nutso
+ *
+ * @private
+ * @param {object} data
+ * @param {object} [options]
+ * @returns {*} result
+ */
+ const getResult = function (data, options = {}) {
+ //get all the pagesIds from the result
+ let pages = Object.keys(data.query.pages); // map over the pageIds to parse out all the information
+
+ return pages.map(id => {
+ // get the page by pageID
+ let page = data.query.pages[id] || {}; // if the page is missing or not found than return null
if (page.hasOwnProperty('missing') || page.hasOwnProperty('invalid')) {
return null;
- }
+ } // get the text from the object
- var text = page.revisions[0]['*']; // console.log(page.revisions[0])
- //us the 'generator' result format, for the random() method
+
+ let text = page.revisions[0]['*']; // if the text is not found in the regular place than it is at the other place
if (!text && page.revisions[0].slots) {
text = page.revisions[0].slots.main['*'];
}
page.pageprops = page.pageprops || {};
- var domain = options.domain;
+ let domain = options.domain;
if (!domain && options.wiki) {
- domain = "".concat(options.wiki, ".org");
+ domain = `${options.wiki}.org`;
}
- var meta = Object.assign({}, options, {
+ let meta = Object.assign({}, options, {
title: page.title,
pageID: page.pageid,
namespace: page.ns,
@@ -200,27 +278,28 @@
wikidata: page.pageprops.wikibase_item,
description: page.pageprops['wikibase-shortdesc']
});
-
- try {
- return {
- wiki: text,
- meta: meta
- };
- } catch (e) {
- console.error(e);
- throw e;
- }
+ return {
+ wiki: text,
+ meta: meta
+ };
});
- return docs;
};
- var _02GetResult = getResult;
+ var getResult_1 = getResult;
- //helper for looping around all sections of a document
- var sectionMap = function sectionMap(doc, fn, clue) {
- var arr = [];
- doc.sections().forEach(function (sec) {
- var list = [];
+ /**
+ * helper for looping around all sections of a document
+ *
+ * @private
+ * @param {Document} doc the document with the sections
+ * @param {string} fn the function name of the function that will be called
+ * @param {string | number} [clue] the clue that will be used with the function
+ * @returns {Array|*} the array of item at the index of the clue
+ */
+ const sectionMap = function (doc, fn, clue) {
+ let arr = [];
+ doc.sections().forEach(sec => {
+ let list = [];
if (typeof clue === 'string') {
list = sec[fn](clue);
@@ -228,13 +307,17 @@
list = sec[fn]();
}
- list.forEach(function (t) {
+ list.forEach(t => {
arr.push(t);
});
});
if (typeof clue === 'number') {
- return arr[clue];
+ if (arr[clue] === undefined) {
+ return [];
+ }
+
+ return [arr[clue]];
}
return arr;
@@ -242,23 +325,71 @@
var _sectionMap = sectionMap;
- //
- var setDefaults = function setDefaults(options, defaults) {
+ /**
+ * applies the the key values of defaults to options
+ *
+ * @private
+ * @param {object} options the user options
+ * @param {object} defaults the defaults
+ * @returns {object} the user options with the defaults applied
+ */
+ const setDefaults = function (options, defaults) {
return Object.assign({}, defaults, options);
};
var setDefaults_1 = setDefaults;
- var defaults$1 = {
+ /**
+ * @typedef DocumentToJsonOptions
+ * @property {boolean | undefined} title
+ * @property {boolean | undefined} pageID
+ * @property {boolean | undefined} categories
+ * @property {boolean | undefined} sections
+ * @property {boolean | undefined} coordinates
+ * @property {boolean | undefined} infoboxes
+ * @property {boolean | undefined} images
+ * @property {boolean | undefined} plaintext
+ * @property {boolean | undefined} citations
+ * @property {boolean | undefined} references
+ */
+
+ const defaults$b = {
title: true,
sections: true,
pageID: true,
categories: true
- }; //an opinionated output of the most-wanted data
-
- var toJSON = function toJSON(doc, options) {
- options = setDefaults_1(options, defaults$1);
- var data = {};
+ };
+ /**
+ * @typedef documentToJsonReturn
+ * @property {string | undefined} title
+ * @property {number | null | undefined} pageID
+ * @property {string[] | undefined} categories
+ * @property {object[] | undefined} sections
+ * @property {boolean | undefined} isRedirect
+ * @property {object | undefined} redirectTo
+ * @property {object[] | undefined} coordinates
+ * @property {object[] | undefined} infoboxes
+ * @property {Image[] | undefined} images
+ * @property {string | undefined} plaintext
+ * @property {object[] | undefined} references
+ */
+
+ /**
+ * an opinionated output of the most-wanted data
+ *
+ * @private
+ * @param {Document} doc
+ * @param {DocumentToJsonOptions} options
+ * @returns {documentToJsonReturn}
+ */
+
+ const toJSON$3 = function (doc, options) {
+ options = setDefaults_1(options, defaults$b);
+ /**
+ * @type {documentToJsonReturn}
+ */
+
+ let data = {};
if (options.title) {
data.title = doc.title();
@@ -273,14 +404,12 @@
}
if (options.sections) {
- data.sections = doc.sections().map(function (i) {
- return i.json(options);
- });
+ data.sections = doc.sections().map(i => i.json(options));
}
if (doc.isRedirect() === true) {
data.isRedirect = true;
- data.redirectTo = doc.data.redirectTo;
+ data.redirectTo = doc.redirectTo();
data.sections = [];
} //these are default-off
@@ -290,15 +419,11 @@
}
if (options.infoboxes) {
- data.infoboxes = doc.infoboxes().map(function (i) {
- return i.json(options);
- });
+ data.infoboxes = doc.infoboxes().map(i => i.json(options));
}
if (options.images) {
- data.images = doc.images().map(function (i) {
- return i.json(options);
- });
+ data.images = doc.images().map(i => i.json(options));
}
if (options.plaintext) {
@@ -312,9 +437,67 @@
return data;
};
- var toJson = toJSON;
+ var toJson$6 = toJSON$3;
+
+ var categories$1 = ['category', //en
+ 'abdeeling', // pdc
+ 'bólkur', // fo
+ 'catagóir', // ga
+ 'categori', // cy
+ 'categoria', 'categoria', // co
+ 'categoría', // es
+ 'categorîa', // lij
+ 'categorìa', // pms
+ 'catégorie', 'categorie', 'catègorie', // frp
+ 'category', 'categuria', // lmo
+ 'catigurìa', // scn
+ 'class', // kw
+ 'ẹ̀ka', // yo
+ 'flocc', 'flocc', // ang
+ 'flokkur', 'grup', // tpi
+ 'jamii', // sw
+ 'kaarangay', // war
+ 'kateggoría', // lad
+ 'kategooria', // et
+ 'kategori', // da
+ 'kategorî', // ku
+ 'kategoria', // eu
+ 'kategória', // hu
+ 'kategorie', //de
+ 'kategoriija', // se
+ 'kategorija', // sl
+ 'kategorio', // eo
+ 'kategoriya', 'kategoriýa', // tk
+ 'kategoriye', // diq
+ 'kategory', // fy
+ 'kategorya', // tl
+ 'kateqoriya', // az
+ 'katiguriya', // qu
+ 'klad', // vo
+ 'luokka', 'ñemohenda', // gn
+ 'roinn', //-seòrsa gd
+ 'ronney', // gv
+ 'rummad', // br
+ 'setensele', // nso
+ 'sokajy', // mg
+ 'sumut', // atassuseq kl
+ 'thể', // loại vi
+ 'turkum', // uz
+ 'категорија', 'категория', // ru
+ 'категорія', // uk
+ 'катэгорыя', 'төркем', // tt
+ 'קטגוריה', // he
+ 'تصنيف', 'تۈر', // ug
+ 'رده', 'श्रेणी', 'श्रेणी', // hi
+ 'বিষয়শ্রেণী', // bn
+ 'หมวดหมู่', // th
+ '분류', // ko
+ '분류', //ko
+ '分类' // za
+ //--
+ ];
- var disambig = ['dab', //en
+ var disambig_templates = ['dab', //en
'disamb', //en
'disambig', //en
'disambiguation', //en
@@ -486,44 +669,295 @@
// 'သဵင်မိူၼ် တူၼ်ႈထႅဝ်ပႅၵ်ႇ', //shn
];
- var i18n = disambig.reduce(function (h, str) {
- h[str] = true;
- return h;
- }, {}); //special disambig-templates en-wikipedia uses
+ // used in titles to denote disambiguation pages
+ // see 'Football_(disambiguation)'
+ var disambig_titles = ['disambiguation', //en
+ 'homonymie', //fr
+ 'توضيح', //ar
+ 'desambiguação', //pt
+ 'Begriffsklärung', //de
+ 'disambigua', //it
+ '曖昧さ回避', //ja
+ '消歧義', //zh
+ '搞清楚', //zh-yue
+ 'значения', //ru
+ 'ابهامزدایی', //fa
+ 'د ابہام', //ur
+ '동음이의', //ko
+ 'dubbelsinnig', //af
+ 'այլ կիրառումներ', //hy
+ 'ujednoznacznienie' //pl
+ ];
- var d = ' disambiguation';
- var english = ['dab', 'dab', 'disamb', 'disambig', 'geodis', 'hndis', 'setindex', 'ship index', 'split dab', 'sport index', 'wp disambig', 'disambiguation cleanup', 'airport' + d, 'biology' + d, 'call sign' + d, 'caselaw' + d, 'chinese title' + d, 'genus' + d, 'hospital' + d, 'lake index', 'letter' + d, 'letter-number combination' + d, 'mathematical' + d, 'military unit' + d, 'mountainindex', 'number' + d, 'phonetics' + d, 'place name' + d, 'portal' + d, 'road' + d, 'school' + d, 'species latin name abbreviation' + d, 'species latin name' + d, 'station' + d, 'synagogue' + d, 'taxonomic authority' + d, 'taxonomy' + d].reduce(function (h, str) {
- h[str] = true;
- return h;
- }, {});
+ var images = ['file', //en
+ 'image', //en
+ 'चित्र', //img
+ 'archivo', //es
+ 'attēls', //lv
+ 'berkas', //id
+ 'bestand', //nl
+ 'datei', //de
+ 'dosiero', //eo
+ 'dosya', //lad
+ 'fájl', //hu
+ 'fasciculus', //la
+ 'fichier', //fr
+ 'fil', //da
+ 'fitxategi', //eu
+ 'fitxer', //ca
+ 'gambar', //su
+ 'imagem', //pt
+ 'imej', //ms
+ 'immagine', //it
+ 'larawan', //tl
+ 'lêer', //af
+ 'plik', //pl
+ 'restr', //br
+ 'slika', //bs
+ 'wêne', //ku
+ 'wobraz', //dsb
+ 'выява', //be
+ 'податотека', //mk
+ 'слика', //sr
+ 'файл', //ru
+ 'სურათი', //ka
+ 'պատկեր', //hy
+ 'קובץ', //he
+ 'پرونده', //fa
+ 'دوتنه', //ps
+ 'ملف', //ar
+ 'وێنە', //ckb
+ 'चित्र', //hi
+ 'ไฟล์', //th
+ '파일', //ko
+ 'ファイル' //ja
+ ];
- var isDisambig = function isDisambig(doc) {
- var templates = doc.templates();
- var found = templates.find(function (obj) {
- return english.hasOwnProperty(obj.template) || i18n.hasOwnProperty(obj.template);
- });
+ var infoboxes = ['infobox', //en
+ 'anfo', //mwl
+ 'anuāmapa', //haw
+ 'bilgi kutusu', //tr
+ 'bilgi', //tr
+ 'bilgiquti', //uz
+ 'boaty', //mg
+ 'boestkelaouiñ', //br
+ 'bosca', //ga
+ 'capsa', //la
+ 'diehtokássa', //se
+ 'faktamall', //sv
+ 'ficha', //es
+ 'generalni', //hr
+ 'gwybodlen3', //cy
+ 'info', //pt
+ 'infobokis', //tpi
+ 'infoboks', //da
+ 'infochascha', //rm
+ 'infokašćik', //dsb
+ 'infokast', //et
+ 'infokutija', //bs
+ 'infolentelė', //lt
+ 'infopolje', //sl
+ 'informkesto', //eo
+ 'infoskreine', //ltg
+ 'infotaula', //eu
+ 'inligtingskas', 'inligtingskas3', //af
+ 'inligtingskas4', //af
+ 'kishtey', //gv
+ 'kotak', //su
+ 'tertcita', //jbo
+ 'tietolaatikko', //fi
+ 'yerleşim bilgi kutusu', 'ynfoboks', //fy
+ 'πλαίσιο', //el
+ 'акарточка', //ab
+ 'аҥа', //mhr
+ 'инфобокс', //kk
+ 'инфокутија', //sr
+ 'инфокутия', //bg
+ 'інфобокс', //rue
+ 'канадский', 'картка', //be
+ 'карточка', //ru
+ 'карточка2', //mdf
+ 'карточкарус', //ba
+ 'картуш', //koi
+ 'қуттӣ', //tg
+ 'ინფოდაფა', //ka
+ 'տեղեկաքարտ', //hy
+ 'אינפאקעסטל', //yi
+ 'תבנית', //he
+ 'بطاقة', //ar
+ 'ڄاڻخانو', //sd
+ 'خانہ', //ur
+ 'لغة', 'ज्ञानसन्दूक', //hi
+ 'তথ্যছক', //bn
+ 'ਜਾਣਕਾਰੀਡੱਬਾ', //pa
+ 'సమాచారపెట్టె', //te
+ 'තොරතුරුකොටුව', //si
+ 'กล่องข้อมูล', //th
+ 'ប្រអប់ព័ត៌មាន', //km
+ '정보상자', //ko
+ '明細' //zh_yue
+ ];
- if (found) {
- return true;
- } // try 'may refer to' on first line for en-wiki?
+ var redirects$1 = ['adkas', //br
+ 'aýdaw', 'doorverwijzing', //nl
+ 'ohjaus', 'patrz', //pl
+ 'přesměruj', 'redirección', 'redireccion', 'redirección', //es
+ 'redirecionamento', //pt
+ 'redirect', //en
+ 'redirection', 'redirection', //fr
+ 'rinvia', //it
+ 'tilvísun', 'uudelleenohjaus', 'weiterleitung', 'weiterleitung', //de
+ 'yönlendi̇r', 'yönlendirme', 'yönlendi̇rme', //tr
+ 'ανακατευθυνση', //el
+ 'айдау', 'перанакіраваньне', 'перенаправлення', //uk
+ 'пренасочување', //mk
+ 'преусмери', 'преусмјери', 'تغییر_مسیر', 'تغییرمسیر', 'تغییرمسیر', //fa
+ 'เปลี่ยนทาง', //th
+ 'ប្តូរទីតាំងទៅ', //km
+ '転送', //ja
+ '重定向'];
+ var references = ['references', 'reference', 'einzelnachweise', 'referencias', 'références', 'notes et références', '脚注', 'referenser', 'bronnen', 'примечания'];
- if (doc.sentences(0)) {
- var firstLine = doc.sentences(0).text();
+ /**
+ * wikipedia special terms lifted and augmented from parsoid parser april 2015
+ * and then manually on March 2020
+ *
+ * @private
+ * @type {{
+ * images: string[],
+ * references: string[],
+ * redirects: string[],
+ * infoboxes: string[],
+ * categories: string[],
+ * disambig: string[]
+ * }}
+ */
+
+ var i18n = {
+ categories: categories$1,
+ disambig_templates: disambig_templates,
+ disambig_titles: disambig_titles,
+ images: images,
+ infoboxes: infoboxes,
+ redirects: redirects$1,
+ references: references //specials: [
+ //'спэцыяльныя',
+ //'especial',
+ //'speciální',
+ //'spezial',
+ //'special',
+ //'ویژه',
+ //'toiminnot',
+ //'kerfissíða',
+ //'arnawlı',
+ //'spécial',
+ //'speciaal',
+ //'посебно',
+ //'özel',
+ //'特別'
+ //],
+ //users: [
+ //'удзельнік',
+ //'usuari',
+ //'uživatel',
+ //'benutzer',
+ //'user',
+ //'usuario',
+ //'کاربر',
+ //'käyttäjä',
+ //'notandi',
+ //'paydalanıwshı',
+ //'utilisateur',
+ //'gebruiker',
+ //'корисник',
+ //'kullanıcı',
+ //'利用者'
+ //],
+ //sources: [
+ ////blacklist these headings, as they're not plain-text
+ //'references',
+ //'see also',
+ //'external links',
+ //'further reading',
+ //'notes et références',
+ //'voir aussi',
+ //'liens externes',
+ //'参考文献', //references (ja)
+ //'脚注', //citations (ja)
+ //'関連項目', //see also (ja)
+ //'外部リンク' //external links (ja)
+ //]
+
+ };
+
+ //alt disambig-templates en-wikipedia uses
+ let d = ' disambiguation';
+ const templates$a = ['dab', 'dab', 'disamb', 'disambig', 'geodis', 'hndis', 'setindex', 'ship index', 'split dab', 'sport index', 'wp disambig', 'disambiguation cleanup', 'airport' + d, 'biology' + d, 'call sign' + d, 'caselaw' + d, 'chinese title' + d, 'genus' + d, 'hospital' + d, 'lake index', 'letter' + d, 'letter-number combination' + d, 'mathematical' + d, 'military unit' + d, 'mountainindex', 'number' + d, 'phonetics' + d, 'place name' + d, 'portal' + d, 'road' + d, 'school' + d, 'species latin name abbreviation' + d, 'species latin name' + d, 'station' + d, 'synagogue' + d, 'taxonomic authority' + d, 'taxonomy' + d].reduce((h, str) => {
+ h[str] = true;
+ return h;
+ }, {});
+ var _disambig = templates$a;
- if (firstLine !== null && firstLine[0]) {
- if (/. may refer to ./i.test(firstLine) === true) {
- return true;
- }
+ const inTitle = new RegExp('. \\((' + i18n.disambig_titles.join('|') + ')\\)$', 'i');
+ const i18n_templates = i18n.disambig_templates.reduce((h, str) => {
+ h[str] = true;
+ return h;
+ }, {}); // look for '... may refer to'
+
+ const byText = function (s) {
+ if (!s) {
+ return false;
+ }
+
+ let txt = s.text();
+
+ if (txt !== null && txt[0]) {
+ if (/. may (also)? refer to\b/i.test(txt) === true) {
+ return true;
}
}
return false;
};
+ /**
+ * Parses the wikitext to find out if this page is a disambiguation
+ *
+ * @private
+ * @param {Document} doc the document that is examined
+ * @returns {boolean} an indication if the document is a disambiguation page
+ */
+
+
+ const isDisambig = function (doc) {
+ // check for a {{disambig}} template
+ let templates = doc.templates().map(tmpl => tmpl.json());
+ let found = templates.find(obj => {
+ return _disambig.hasOwnProperty(obj.template) || i18n_templates.hasOwnProperty(obj.template);
+ });
+
+ if (found) {
+ return true;
+ } // check for (disambiguation) in title
+
+
+ let title = doc.title();
+
+ if (title && inTitle.test(title) === true) {
+ return true;
+ } //try 'may refer to' on first line for en-wiki?
+
+
+ if (byText(doc.sentence(0)) === true || byText(doc.sentence(1)) === true) {
+ return true;
+ }
+
+ return false;
+ };
- var disambig$1 = isDisambig;
+ var isDisambig_1 = isDisambig;
- var defaults$2 = {
+ const defaults$a = {
caption: true,
alt: true,
links: true,
@@ -531,9 +965,9 @@
url: true
}; //
- var toJson$1 = function toJson(img, options) {
- options = setDefaults_1(options, defaults$2);
- var json = {
+ const toJson$5 = function (img, options) {
+ options = setDefaults_1(options, defaults$a);
+ let json = {
file: img.file()
};
@@ -561,12 +995,12 @@
return json;
};
- var toJson_1 = toJson$1;
+ var toJson_1$3 = toJson$5;
- var server = 'wikipedia.org';
+ const server = 'wikipedia.org';
- var encodeTitle = function encodeTitle(file) {
- var title = file.replace(/^(image|file?)\:/i, ''); //titlecase it
+ const encodeTitle = function (file) {
+ let title = file.replace(/^(image|file?)\:/i, ''); //titlecase it
title = title.charAt(0).toUpperCase() + title.substring(1); //spaces to underscores
@@ -575,57 +1009,63 @@
}; //the wikimedia image url is a little silly:
- var makeSrc = function makeSrc(file) {
- var title = encodeTitle(file);
+ const makeSrc = function (file) {
+ let title = encodeTitle(file);
title = encodeURIComponent(title);
return title;
}; //the class for our image generation functions
- var Image = function Image(data) {
+ const Image = function (data) {
Object.defineProperty(this, 'data', {
enumerable: false,
value: data
});
};
- var methods = {
- file: function file() {
+ const methods$8 = {
+ file() {
return this.data.file || '';
},
- alt: function alt() {
- var str = this.data.alt || this.data.file || '';
+
+ alt() {
+ let str = this.data.alt || this.data.file || '';
str = str.replace(/^(file|image):/i, '');
str = str.replace(/\.(jpg|jpeg|png|gif|svg)/i, '');
return str.replace(/_/g, ' ');
},
- caption: function caption() {
+
+ caption() {
if (this.data.caption) {
return this.data.caption.text();
}
return '';
},
- links: function links() {
+
+ links() {
if (this.data.caption) {
return this.data.caption.links();
}
return [];
},
- url: function url() {
+
+ url() {
// let lang = 'en' //this.language() || 'en' //hmm: get actual language?
- var fileName = makeSrc(this.file());
- var domain = this.data.domain || server;
- var path = "wiki/Special:Redirect/file";
- return "https://".concat(domain, "/").concat(path, "/").concat(fileName);
+ let fileName = makeSrc(this.file());
+ let domain = this.data.domain || server;
+ let path = `wiki/Special:Redirect/file`;
+ return `https://${domain}/${path}/${fileName}`;
},
- thumbnail: function thumbnail(size) {
+
+ thumbnail(size) {
size = size || 300;
return this.url() + '?width=' + size;
},
- format: function format() {
- var arr = this.file().split('.');
+
+ format() {
+ let arr = this.file().split('.');
if (arr[arr.length - 1]) {
return arr[arr.length - 1].toLowerCase();
@@ -633,566 +1073,25 @@
return null;
},
- json: function json(options) {
+
+ json: function (options) {
options = options || {};
- return toJson_1(this, options);
+ return toJson_1$3(this, options);
},
- text: function text() {
+ text: function () {
return '';
+ },
+ wikitext: function () {
+ return this.data.wiki || '';
}
};
- Object.keys(methods).forEach(function (k) {
- Image.prototype[k] = methods[k];
+ Object.keys(methods$8).forEach(k => {
+ Image.prototype[k] = methods$8[k];
});
Image.prototype.src = Image.prototype.url;
Image.prototype.thumb = Image.prototype.thumbnail;
var Image_1 = Image;
- var defaults$3 = {
- tables: true,
- lists: true,
- paragraphs: true
- }; //
-
- var Document = function Document(data) {
- Object.defineProperty(this, 'data', {
- enumerable: false,
- value: data
- });
- };
-
- var methods$1 = {
- title: function title(str) {
- //use like a setter
- if (str !== undefined) {
- this.data.title = str;
- return str;
- } //if we have it already
-
-
- if (this.data.title) {
- return this.data.title;
- } //guess the title of this page from first sentence bolding
-
-
- var guess = null;
- var sen = this.sentences(0);
-
- if (sen) {
- guess = sen.bolds(0);
- }
-
- return guess;
- },
- pageID: function pageID(id) {
- if (id !== undefined) {
- this.data.pageID = id;
- }
-
- return this.data.pageID;
- },
- wikidata: function wikidata(id) {
- if (id !== undefined) {
- this.data.wikidata = id;
- }
-
- return this.data.wikidata;
- },
- domain: function domain(str) {
- if (str !== undefined) {
- this.data.domain = str;
- }
-
- return this.data.domain;
- },
- language: function language(lang) {
- if (lang !== undefined) {
- this.data.lang = lang;
- }
-
- return this.data.lang;
- },
- url: function url() {
- var title = this.title();
-
- if (!title) {
- return null;
- }
-
- var lang = this.language() || 'en';
- var domain = this.domain() || 'wikipedia.org'; // replace blank to underscore
-
- title = title.replace(/ /g, '_');
- title = encodeURIComponent(title);
- return "https://".concat(lang, ".").concat(domain, "/wiki/").concat(title);
- },
- namespace: function namespace(ns) {
- if (ns !== undefined) {
- this.data.namespace = ns;
- }
-
- return this.data.namespace;
- },
- isRedirect: function isRedirect() {
- return this.data.type === 'redirect';
- },
- redirectTo: function redirectTo() {
- return this.data.redirectTo;
- },
- isDisambiguation: function isDisambiguation() {
- return disambig$1(this);
- },
- categories: function categories(clue) {
- if (typeof clue === 'number') {
- return this.data.categories[clue];
- }
-
- return this.data.categories || [];
- },
- sections: function sections(clue) {
- var _this = this;
-
- var arr = this.data.sections || [];
- arr.forEach(function (sec) {
- return sec.doc = _this;
- }); //grab a specific section, by its title
-
- if (typeof clue === 'string') {
- var str = clue.toLowerCase().trim();
- return arr.find(function (s) {
- return s.title().toLowerCase() === str;
- });
- }
-
- if (typeof clue === 'number') {
- return arr[clue];
- }
-
- return arr;
- },
- paragraphs: function paragraphs(n) {
- var arr = [];
- this.data.sections.forEach(function (s) {
- arr = arr.concat(s.paragraphs());
- });
-
- if (typeof n === 'number') {
- return arr[n];
- }
-
- return arr;
- },
- paragraph: function paragraph(n) {
- var arr = this.paragraphs() || [];
-
- if (typeof n === 'number') {
- return arr[n];
- }
-
- return arr[0];
- },
- sentences: function sentences(n) {
- var arr = [];
- this.sections().forEach(function (sec) {
- arr = arr.concat(sec.sentences());
- });
-
- if (typeof n === 'number') {
- return arr[n];
- }
-
- return arr;
- },
- sentence: function sentence() {
- return this.sentences(0);
- },
- images: function images(clue) {
- var _this2 = this;
-
- var arr = _sectionMap(this, 'images', null); //grab image from infobox, first
-
- this.infoboxes().forEach(function (info) {
- var img = info.image();
-
- if (img) {
- arr.unshift(img); //put it at the top
- }
- }); //look for 'gallery' templates, too
-
- this.templates().forEach(function (obj) {
- if (obj.template === 'gallery') {
- obj.images = obj.images || [];
- obj.images.forEach(function (img) {
- if (img instanceof Image_1 === false) {
- img.language = _this2.language();
- img.domain = _this2.domain();
- img = new Image_1(img);
- }
-
- arr.push(img);
- });
- }
- });
-
- if (typeof clue === 'number') {
- return arr[clue];
- }
-
- return arr;
- },
- image: function image() {
- return this.images(0);
- },
- links: function links(clue) {
- return _sectionMap(this, 'links', clue);
- },
- interwiki: function interwiki(clue) {
- return _sectionMap(this, 'interwiki', clue);
- },
- lists: function lists(clue) {
- return _sectionMap(this, 'lists', clue);
- },
- tables: function tables(clue) {
- return _sectionMap(this, 'tables', clue);
- },
- templates: function templates(clue) {
- return _sectionMap(this, 'templates', clue);
- },
- references: function references(clue) {
- return _sectionMap(this, 'references', clue);
- },
- coordinates: function coordinates(clue) {
- return _sectionMap(this, 'coordinates', clue);
- },
- infoboxes: function infoboxes(clue) {
- var arr = _sectionMap(this, 'infoboxes'); //sort them by biggest-first
-
- arr = arr.sort(function (a, b) {
- if (Object.keys(a.data).length > Object.keys(b.data).length) {
- return -1;
- }
-
- return 1;
- });
-
- if (typeof clue === 'number') {
- return arr[clue];
- }
-
- return arr;
- },
- text: function text(options) {
- options = setDefaults_1(options, defaults$3); //nah, skip these.
-
- if (this.isRedirect() === true) {
- return '';
- }
-
- var arr = this.sections().map(function (sec) {
- return sec.text(options);
- });
- return arr.join('\n\n');
- },
- json: function json(options) {
- options = setDefaults_1(options, defaults$3);
- return toJson(this, options);
- },
- debug: function debug() {
- console.log('\n');
- this.sections().forEach(function (sec) {
- var indent = ' - ';
-
- for (var i = 0; i < sec.depth; i += 1) {
- indent = ' -' + indent;
- }
-
- console.log(indent + (sec.title() || '(Intro)'));
- });
- return this;
- }
- };
-
- var isArray$1 = function isArray(arr) {
- return Object.prototype.toString.call(arr) === '[object Array]';
- }; //add singular-methods, too
-
-
- var plurals = ['sections', 'infoboxes', 'sentences', 'citations', 'references', 'coordinates', 'tables', 'lists', 'links', 'images', 'templates', 'categories'];
- plurals.forEach(function (fn) {
- var sing = fn.replace(/ies$/, 'y');
- sing = sing.replace(/oxes$/, 'ox');
- sing = sing.replace(/s$/, '');
-
- methods$1[sing] = function (n) {
- n = n || 0;
- var res = this[fn](n);
-
- if (isArray$1(res)) {
- return res[0];
- }
-
- return res;
- };
- });
- Object.keys(methods$1).forEach(function (k) {
- Document.prototype[k] = methods$1[k];
- }); //alias these ones
-
- Document.prototype.lang = Document.prototype.language;
- Document.prototype.ns = Document.prototype.namespace;
- Document.prototype.plaintext = Document.prototype.text;
- Document.prototype.isDisambig = Document.prototype.isDisambiguation;
- Document.prototype.citations = Document.prototype.references;
- Document.prototype.redirectsTo = Document.prototype.redirectTo;
- Document.prototype.redirect = Document.prototype.redirectTo;
- Document.prototype.redirects = Document.prototype.redirectTo;
- var Document_1 = Document;
-
- var categories = ['category', //en
- 'abdeeling', // pdc
- 'bólkur', // fo
- 'catagóir', // ga
- 'categori', // cy
- 'categoria', 'categoria', // co
- 'categoría', // es
- 'categorîa', // lij
- 'categorìa', // pms
- 'catégorie', 'categorie', 'catègorie', // frp
- 'category', 'categuria', // lmo
- 'catigurìa', // scn
- 'class', // kw
- 'ẹ̀ka', // yo
- 'flocc', 'flocc', // ang
- 'flokkur', 'grup', // tpi
- 'jamii', // sw
- 'kaarangay', // war
- 'kateggoría', // lad
- 'kategooria', // et
- 'kategori', // da
- 'kategorî', // ku
- 'kategoria', // eu
- 'kategória', // hu
- 'kategorie', //de
- 'kategoriija', // se
- 'kategorija', // sl
- 'kategorio', // eo
- 'kategoriya', 'kategoriýa', // tk
- 'kategoriye', // diq
- 'kategory', // fy
- 'kategorya', // tl
- 'kateqoriya', // az
- 'katiguriya', // qu
- 'klad', // vo
- 'luokka', 'ñemohenda', // gn
- 'roinn', //-seòrsa gd
- 'ronney', // gv
- 'rummad', // br
- 'setensele', // nso
- 'sokajy', // mg
- 'sumut', // atassuseq kl
- 'thể', // loại vi
- 'turkum', // uz
- 'категорија', 'категория', // ru
- 'категорія', // uk
- 'катэгорыя', 'төркем', // tt
- 'קטגוריה', // he
- 'تصنيف', 'تۈر', // ug
- 'رده', 'श्रेणी', 'श्रेणी', // hi
- 'বিষয়শ্রেণী', // bn
- 'หมวดหมู่', // th
- '분류', // ko
- '분류', //ko
- '分类' // za
- //--
- ];
-
- var images = ['file', //en
- 'image', //en
- 'चित्र', //img
- 'archivo', //es
- 'attēls', //lv
- 'berkas', //id
- 'bestand', //nl
- 'datei', //de
- 'dosiero', //eo
- 'dosya', //lad
- 'fájl', //hu
- 'fasciculus', //la
- 'fichier', //fr
- 'fil', //da
- 'fitxategi', //eu
- 'fitxer', //ca
- 'gambar', //su
- 'imagem', //pt
- 'imej', //ms
- 'immagine', //it
- 'larawan', //tl
- 'lêer', //af
- 'plik', //pl
- 'restr', //br
- 'slika', //bs
- 'wêne', //ku
- 'wobraz', //dsb
- 'выява', //be
- 'податотека', //mk
- 'слика', //sr
- 'файл', //ru
- 'სურათი', //ka
- 'պատկեր', //hy
- 'קובץ', //he
- 'پرونده', //fa
- 'دوتنه', //ps
- 'ملف', //ar
- 'وێنە', //ckb
- 'चित्र', //hi
- 'ไฟล์', //th
- '파일', //ko
- 'ファイル' //ja
- ];
-
- var infoboxes = ['infobox', //en
- 'anfo', //mwl
- 'anuāmapa', //haw
- 'bilgi kutusu', //tr
- 'bilgi', //tr
- 'bilgiquti', //uz
- 'boaty', //mg
- 'boestkelaouiñ', //br
- 'bosca', //ga
- 'capsa', //la
- 'diehtokássa', //se
- 'faktamall', //sv
- 'ficha', //es
- 'generalni', //hr
- 'gwybodlen3', //cy
- 'info', //pt
- 'infobokis', //tpi
- 'infoboks', //da
- 'infochascha', //rm
- 'infokašćik', //dsb
- 'infokast', //et
- 'infokutija', //bs
- 'infolentelė', //lt
- 'infopolje', //sl
- 'informkesto', //eo
- 'infoskreine', //ltg
- 'infotaula', //eu
- 'inligtingskas', 'inligtingskas3', //af
- 'inligtingskas4', //af
- 'kishtey', //gv
- 'kotak', //su
- 'tertcita', //jbo
- 'tietolaatikko', //fi
- 'yerleşim bilgi kutusu', 'ynfoboks', //fy
- 'πλαίσιο', //el
- 'акарточка', //ab
- 'аҥа', //mhr
- 'инфобокс', //kk
- 'инфокутија', //sr
- 'инфокутия', //bg
- 'інфобокс', //rue
- 'канадский', 'картка', //be
- 'карточка', //ru
- 'карточка2', //mdf
- 'карточкарус', //ba
- 'картуш', //koi
- 'қуттӣ', //tg
- 'ინფოდაფა', //ka
- 'տեղեկաքարտ', //hy
- 'אינפאקעסטל', //yi
- 'תבנית', //he
- 'بطاقة', //ar
- 'ڄاڻخانو', //sd
- 'خانہ', //ur
- 'لغة', 'ज्ञानसन्दूक', //hi
- 'তথ্যছক', //bn
- 'ਜਾਣਕਾਰੀਡੱਬਾ', //pa
- 'సమాచారపెట్టె', //te
- 'තොරතුරුකොටුව', //si
- 'กล่องข้อมูล', //th
- 'ប្រអប់ព័ត៌មាន', //km
- '정보상자', //ko
- '明細' //zh_yue
- ];
-
- var redirects = ['adkas', //br
- 'aýdaw', 'doorverwijzing', 'ohjaus', 'patrz', //pl
- 'přesměruj', 'redirección', 'redireccion', 'redirección', //es
- 'redirecionamento', //pt
- 'redirect', //en
- 'redirection', 'redirection', //fr
- 'rinvia', //it
- 'tilvísun', 'uudelleenohjaus', 'weiterleitung', 'weiterleitung', //de
- 'yönlendi̇r', 'yönlendirme', 'yönlendi̇rme', //tr
- 'ανακατευθυνση', //el
- 'айдау', 'перанакіраваньне', 'перенаправлення', //uk
- 'пренасочување', //mk
- 'преусмери', 'преусмјери', 'تغییر_مسیر', 'تغییرمسیر', 'تغییرمسیر', //fa
- 'เปลี่ยนทาง', //th
- 'ប្តូរទីតាំងទៅ', //km
- '転送', //ja
- '重定向'];
-
- var references = ['references', 'reference', 'einzelnachweise', 'referencias', 'références', 'notes et références', '脚注', 'referenser', 'bronnen', 'примечания'];
-
- // and then manually on March 2020
-
- var i18n$1 = {
- categories: categories,
- disambig: disambig,
- images: images,
- infoboxes: infoboxes,
- redirects: redirects,
- references: references // specials: [
- // 'спэцыяльныя',
- // 'especial',
- // 'speciální',
- // 'spezial',
- // 'special',
- // 'ویژه',
- // 'toiminnot',
- // 'kerfissíða',
- // 'arnawlı',
- // 'spécial',
- // 'speciaal',
- // 'посебно',
- // 'özel',
- // '特別'
- // ],
- // users: [
- // 'удзельнік',
- // 'usuari',
- // 'uživatel',
- // 'benutzer',
- // 'user',
- // 'usuario',
- // 'کاربر',
- // 'käyttäjä',
- // 'notandi',
- // 'paydalanıwshı',
- // 'utilisateur',
- // 'gebruiker',
- // 'корисник',
- // 'kullanıcı',
- // '利用者'
- // ],
- // sources: [
- // //blacklist these headings, as they're not plain-text
- // 'references',
- // 'see also',
- // 'external links',
- // 'further reading',
- // 'notes et références',
- // 'voir aussi',
- // 'liens externes',
- // '参考文献', //references (ja)
- // '脚注', //citations (ja)
- // '関連項目', //see also (ja)
- // '外部リンク' //external links (ja)
- // ]
-
- };
-
var languages = {
aa: 'Afar',
//Afar
@@ -1506,7 +1405,7 @@
//Dutch
ne: 'नेपाली',
//Nepali
- "new": 'नेपालभाषा',
+ new: 'नेपालभाषा',
//Newar
ng: 'Oshiwambo',
//Ndonga
@@ -1702,10 +1601,10 @@
};
- var wp = '.wikipedia.org/wiki/$1';
- var wm = '.wikimedia.org/wiki/$1';
- var w = 'www.';
- var interwiki = {
+ const wp = '.wikipedia.org/wiki/$1';
+ const wm = '.wikimedia.org/wiki/$1';
+ const w = 'www.';
+ var interwiki$1 = {
acronym: w + 'acronymfinder.com/$1.html',
advisory: 'advisory' + wm,
advogato: w + 'advogato.org/$1',
@@ -2169,32 +2068,28 @@
//so we have to whitelist allowable interwiki links
//add language prefixes too..
- Object.keys(languages).forEach(function (k) {
- interwiki[k] = k + '.wikipedia.org/wiki/$1';
+ Object.keys(languages).forEach(k => {
+ interwiki$1[k] = k + '.wikipedia.org/wiki/$1';
}); //this is predictably very complicated.
// https://meta.wikimedia.org/wiki/Help:Interwiki_linking
- var parseInterwiki = function parseInterwiki(obj) {
- var str = obj.page || '';
+ const parseInterwiki = function (obj) {
+ let str = obj.page || '';
if (str.indexOf(':') !== -1) {
- var m = str.match(/^(.*):(.*)/);
+ let m = str.match(/^(.*):(.*)/);
if (m === null) {
return obj;
}
- var site = m[1] || '';
+ let site = m[1] || '';
site = site.toLowerCase();
if (site.indexOf(':') !== -1) {
- var _site$match = site.match(/^:?(.*):(.*)/),
- _site$match2 = _slicedToArray(_site$match, 3),
- wiki = _site$match2[1],
- lang = _site$match2[2]; //only allow interwikis to these specific places
+ let [, wiki, lang] = site.match(/^:?(.*):(.*)/); //only allow interwikis to these specific places
-
- if (interwiki.hasOwnProperty(wiki) && languages.hasOwnProperty(lang) === false) {
+ if (interwiki$1.hasOwnProperty(wiki) && languages.hasOwnProperty(lang) === false) {
return obj;
}
@@ -2203,7 +2098,7 @@
lang: lang
};
} else {
- if (interwiki.hasOwnProperty(site) === false) {
+ if (interwiki$1.hasOwnProperty(site) === false) {
return obj;
}
@@ -2216,13 +2111,13 @@
return obj;
};
- var interwiki$1 = parseInterwiki;
+ var interwiki = parseInterwiki;
- var ignore_links = /^:?(category|catégorie|Kategorie|Categoría|Categoria|Categorie|Kategoria|تصنيف|image|file|image|fichier|datei|media):/i;
- var external_link = /\[(https?|news|ftp|mailto|gopher|irc)(:\/\/[^\]\| ]{4,1500})([\| ].*?)?\]/g;
- var link_reg = /\[\[(.{0,160}?)\]\]([a-z]+)?/gi; //allow dangling suffixes - "[[flanders]]s"
+ const ignore_links = /^:?(category|catégorie|Kategorie|Categoría|Categoria|Categorie|Kategoria|تصنيف|image|file|image|fichier|datei|media):/i;
+ const external_link = /\[(https?|news|ftp|mailto|gopher|irc)(:\/\/[^\]\| ]{4,1500})([\| ].*?)?\]/g;
+ const link_reg = /\[\[(.{0,160}?)\]\]([a-z]+)?/gi; //allow dangling suffixes - "[[flanders]]s"
- var external_links = function external_links(links, str) {
+ const external_links = function (links, str) {
str.replace(external_link, function (raw, protocol, link, text) {
text = text || '';
links.push({
@@ -2236,12 +2131,12 @@
return links;
};
- var internal_links = function internal_links(links, str) {
+ const internal_links = function (links, str) {
//regular links
str.replace(link_reg, function (raw, s, suffix) {
- var txt = null; //make a copy of original
+ let txt = null; //make a copy of original
- var link = s;
+ let link = s;
if (s.match(/\|/)) {
//replacement link [[link|text]]
@@ -2268,16 +2163,16 @@
//remove anchors from end [[toronto#history]]
- var obj = {
+ let obj = {
page: link,
raw: raw
};
- obj.page = obj.page.replace(/#(.*)/, function (a, b) {
+ obj.page = obj.page.replace(/#(.*)/, (a, b) => {
obj.anchor = b;
return '';
}); //grab any fr:Paris parts
- obj = interwiki$1(obj);
+ obj = interwiki(obj);
if (obj.wiki) {
obj.type = 'interwiki';
@@ -2309,8 +2204,8 @@
}; //grab an array of internal links in the text
- var parse_links = function parse_links(str) {
- var links = []; //first, parse external links
+ const parse_links = function (str) {
+ let links = []; //first, parse external links
links = external_links(links, str); //internal links
@@ -2323,11 +2218,11 @@
return links;
};
- var parse = parse_links;
+ var parse$8 = parse_links;
- var REDIRECT_REGEX = new RegExp('^[ \n\t]*?#(' + i18n$1.redirects.join('|') + ') *?(\\[\\[.{2,180}?\\]\\])', 'i');
+ const REDIRECT_REGEX = new RegExp('^[ \n\t]*?#(' + i18n.redirects.join('|') + ') *?(\\[\\[.{2,180}?\\]\\])', 'i');
- var isRedirect = function isRedirect(wiki) {
+ const isRedirect = function (wiki) {
//too long to be a redirect?
if (!wiki || wiki.length > 500) {
return false;
@@ -2336,49 +2231,49 @@
return REDIRECT_REGEX.test(wiki);
};
- var parse$1 = function parse$1(wiki) {
- var m = wiki.match(REDIRECT_REGEX);
+ const parse$7 = function (wiki) {
+ let m = wiki.match(REDIRECT_REGEX);
if (m && m[2]) {
- var links = parse(m[2]) || [];
+ let links = parse$8(m[2]) || [];
return links[0];
}
return {};
};
- var redirects$1 = {
+ var redirects = {
isRedirect: isRedirect,
- parse: parse$1
+ parse: parse$7
};
//okay, i know you're not supposed to regex html, but...
//https://en.wikipedia.org/wiki/Help:HTML_in_wikitext
//these are things we throw-away
//these will mess-up if they're nested, but they're not usually.
- var ignore = ['table', 'code', 'score', 'data', 'categorytree', 'charinsert', 'hiero', 'imagemap', 'inputbox', 'nowiki', 'poem', 'references', 'source', 'syntaxhighlight', 'timeline'];
- var openTag = "< ?(".concat(ignore.join('|'), ") ?[^>]{0,200}?>");
- var closeTag = "< ?/ ?(".concat(ignore.join('|'), ") ?>");
- var anyChar = '\\s\\S'; //including newline
+ const ignore$1 = ['table', 'code', 'score', 'data', 'categorytree', 'charinsert', 'hiero', 'imagemap', 'inputbox', 'nowiki', 'poem', 'references', 'source', 'syntaxhighlight', 'timeline'];
+ const openTag = `< ?(${ignore$1.join('|')}) ?[^>]{0,200}?>`;
+ const closeTag = `< ?/ ?(${ignore$1.join('|')}) ?>`;
+ const anyChar = '\\s\\S'; //including newline
- var noThanks = new RegExp("".concat(openTag, "[").concat(anyChar, "]+?").concat(closeTag), 'ig');
+ const noThanks = new RegExp(`${openTag}[${anyChar}]+?${closeTag}`, 'ig');
- var kill_xml = function kill_xml(wiki) {
+ const kill_xml = function (wiki) {
//([ tags are parsed in Section class) - luckily, refs can't be recursive.
//types of html/xml that we want to trash completely.
wiki = wiki.replace(noThanks, ' '); //some xml-like fragments we can also kill
- wiki = wiki.replace(/ ?< ?(span|div|table|data) [a-zA-Z0-9=%\.#:;'" ]{2,100}\/? ?> ?/g, ' '); //][
+ wiki = wiki.replace(/ ?< ?(span|div|table|data) [a-zA-Z0-9=%\.\-#:;'" ]{2,100}\/? ?> ?/g, ' '); //][
//only kill ref tags if they are selfclosing
wiki = wiki.replace(/ ?< ?(ref) [a-zA-Z0-9=" ]{2,100}\/ ?> ?/g, ' '); //]
// convert these html tags to known formatting
- wiki = wiki.replace(/(.*?)<\/i>/g, "''$1''");
- wiki = wiki.replace(/(.*?)<\/b>/g, "'''$1'''"); // these are better-handled with templates
+ wiki = wiki.replace(/(.*?)<\/i>/g, `''$1''`);
+ wiki = wiki.replace(/(.*?)<\/b>/g, `'''$1'''`); // these are better-handled with templates
- wiki = wiki.replace(/(.*?)<\/sub>/g, "{{sub|$1}}");
- wiki = wiki.replace(/(.*?)<\/sup>/g, "{{sup|$1}}"); //some formatting xml, we'll keep their insides though
+ wiki = wiki.replace(/(.*?)<\/sub>/g, `{{sub|$1}}`);
+ wiki = wiki.replace(/(.*?)<\/sup>/g, `{{sup|$1}}`); //some formatting xml, we'll keep their insides though
wiki = wiki.replace(/ ?<[ \/]?(p|sub|sup|span|nowiki|div|table|br|tr|td|th|pre|pre2|hr)[ \/]?> ?/g, ' '); //,
@@ -2393,9 +2288,17 @@
var kill_xml_1 = kill_xml;
- function preProcess(doc) {
- var wiki = doc.wiki; //remove comments
-
+ /**
+ * removes unnecessary strings from the wikitext
+ * it is mostly-formatting stuff can be cleaned-up first, to make life easier
+ *
+ * @private
+ * @param {string} wiki the wikitext that needs processing
+ * @returns {string} the processed text
+ */
+
+ function preProcess(wiki) {
+ //remove comments
wiki = wiki.replace(//g, '');
wiki = wiki.replace(/__(NOTOC|NOEDITSECTION|FORCETOC|TOC)__/gi, ''); //signitures
@@ -2417,34 +2320,40 @@
wiki = wiki.replace(/\([,;: ]+?\)/g, ''); //these templates just screw things up, too
wiki = wiki.replace(/{{(baseball|basketball) (primary|secondary) (style|color).*?\}\}/i, '');
- doc.wiki = wiki;
+ return wiki;
}
var preProcess_1 = preProcess;
- // dumpster-dive throws everything into mongodb - github.com/spencermountain/dumpster-dive
- // mongo has some opinions about what characters are allowed as keys and ids.
+ //dumpster-dive throws everything into mongodb - github.com/spencermountain/dumpster-dive
+ //mongo has some opinions about what characters are allowed as keys and ids.
//https://stackoverflow.com/questions/12397118/mongodb-dot-in-key-name/30254815#30254815
- var specialChar = /[\\\.$]/;
-
- var encodeStr = function encodeStr(str) {
+ const specialChar = /[\\\.$]/;
+ /**
+ * this function encodes a string to make it mongodb compatible.
+ * https://stackoverflow.com/questions/12397118/mongodb-dot-in-key-name/30254815#30254815
+ *
+ * @param {string} str
+ * @returns {string} the encoded string
+ */
+
+ const encodeStr = function (str) {
if (typeof str !== 'string') {
str = '';
}
str = str.replace(/\\/g, '\\\\');
- str = str.replace(/^\$/, "\\u0024");
- str = str.replace(/\./g, "\\u002e");
+ str = str.replace(/^\$/, '\\u0024');
+ str = str.replace(/\./g, '\\u002e');
return str;
};
- var encodeObj = function encodeObj() {
- var obj = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {};
- var keys = Object.keys(obj);
+ const encodeObj = function (obj = {}) {
+ let keys = Object.keys(obj);
- for (var i = 0; i < keys.length; i += 1) {
+ for (let i = 0; i < keys.length; i += 1) {
if (specialChar.test(keys[i]) === true) {
- var str = encodeStr(keys[i]);
+ let str = encodeStr(keys[i]);
if (str !== keys[i]) {
obj[str] = obj[keys[i]];
@@ -2460,7 +2369,7 @@
encodeObj: encodeObj
};
- var defaults$4 = {
+ const defaults$9 = {
headers: true,
depth: true,
paragraphs: true,
@@ -2470,25 +2379,33 @@
infoboxes: true,
lists: true,
references: true
- }; //
+ };
+ /**
+ *
+ * @param {Section} section
+ * @param {sectionToJsonOptions} options
+ * @returns {object}
+ */
+
+ const toJSON$2 = function (section, options) {
+ options = setDefaults_1(options, defaults$9);
+ /**
+ * @type {object}
+ */
- var toJSON$1 = function toJSON(section, options) {
- options = setDefaults_1(options, defaults$4);
- var data = {};
+ let data = {};
if (options.headers === true) {
data.title = section.title();
}
if (options.depth === true) {
- data.depth = section.depth;
+ data.depth = section.depth();
} //these return objects
if (options.paragraphs === true) {
- var paragraphs = section.paragraphs().map(function (p) {
- return p.json(options);
- });
+ let paragraphs = section.paragraphs().map(p => p.json(options));
if (paragraphs.length > 0) {
data.paragraphs = paragraphs;
@@ -2497,9 +2414,7 @@
if (options.images === true) {
- var images = section.images().map(function (img) {
- return img.json(options);
- });
+ let images = section.images().map(img => img.json(options));
if (images.length > 0) {
data.images = images;
@@ -2508,9 +2423,7 @@
if (options.tables === true) {
- var tables = section.tables().map(function (t) {
- return t.json(options);
- });
+ let tables = section.tables().map(t => t.json(options));
if (tables.length > 0) {
data.tables = tables;
@@ -2519,24 +2432,20 @@
if (options.templates === true) {
- var templates = section.templates();
+ let templates = section.templates().map(tmpl => tmpl.json());
if (templates.length > 0) {
data.templates = templates; //encode them, for mongodb
if (options.encode === true) {
- data.templates.forEach(function (t) {
- return encode.encodeObj(t);
- });
+ data.templates.forEach(t => encode.encodeObj(t));
}
}
} //infobox json data
if (options.infoboxes === true) {
- var infoboxes = section.infoboxes().map(function (i) {
- return i.json(options);
- });
+ let infoboxes = section.infoboxes().map(i => i.json(options));
if (infoboxes.length > 0) {
data.infoboxes = infoboxes;
@@ -2545,9 +2454,7 @@
if (options.lists === true) {
- var lists = section.lists().map(function (list) {
- return list.json(options);
- });
+ let lists = section.lists().map(list => list.json(options));
if (lists.length > 0) {
data.lists = lists;
@@ -2556,9 +2463,7 @@
if (options.references === true || options.citations === true) {
- var references = section.references().map(function (ref) {
- return ref.json(options);
- });
+ let references = section.references().map(ref => ref.json(options));
if (references.length > 0) {
data.references = references;
@@ -2567,468 +2472,125 @@
if (options.sentences === true) {
- data.sentences = section.sentences().map(function (s) {
- return s.json(options);
- });
+ data.sentences = section.sentences().map(s => s.json(options));
}
return data;
};
- var toJson$2 = toJSON$1;
+ var toJson$4 = toJSON$2;
- var defaults$5 = {
- tables: true,
- references: true,
- paragraphs: true,
- templates: true,
- infoboxes: true
- }; //the stuff between headings - 'History' section for example
+ const defaults$8 = {
+ type: 'internal'
+ };
- var Section = function Section(data) {
- this.depth = data.depth;
- this.doc = null;
- this._title = data.title || '';
- Object.defineProperty(this, 'doc', {
- enumerable: false,
- value: null
- });
- data.templates = data.templates || [];
+ const Link = function (data) {
+ data = data || {};
+ data = Object.assign({}, defaults$8, data);
Object.defineProperty(this, 'data', {
enumerable: false,
value: data
});
};
- var methods$2 = {
- title: function title() {
- return this._title || '';
+ const methods$7 = {
+ text: function (str) {
+ if (str !== undefined) {
+ this.data.text = str;
+ }
+
+ let txt = this.data.text || this.data.page || ''; // remove bold/italics
+
+ txt = txt.replace(/''+/g, '');
+ return txt;
},
- index: function index() {
- if (!this.doc) {
- return null;
+ json: function () {
+ let obj = {
+ text: this.data.text,
+ type: this.type()
+ };
+
+ if (obj.type === 'internal') {
+ obj.page = this.page();
+ } else if (obj.type === 'interwiki') {
+ obj.wiki = this.wiki();
+ } else {
+ obj.site = this.site();
}
- var index = this.doc.sections().indexOf(this);
+ let anchor = this.anchor();
- if (index === -1) {
- return null;
+ if (anchor) {
+ obj.anchor = anchor;
}
- return index;
+ return obj;
},
- indentation: function indentation() {
- return this.depth;
+ wikitext: function () {
+ let txt = this.data.raw || '';
+ return txt;
},
- sentences: function sentences(n) {
- var arr = this.paragraphs().reduce(function (list, p) {
- return list.concat(p.sentences());
- }, []);
-
- if (typeof n === 'number') {
- return arr[n];
+ page: function (str) {
+ if (str !== undefined) {
+ this.data.page = str;
}
- return arr || [];
+ return this.data.page;
},
- paragraphs: function paragraphs(n) {
- var arr = this.data.paragraphs || [];
+ anchor: function (str) {
+ if (str !== undefined) {
+ this.data.anchor = str;
+ }
- if (typeof n === 'number') {
- return arr[n];
+ return this.data.anchor || '';
+ },
+ wiki: function (str) {
+ if (str !== undefined) {
+ this.data.wiki = str;
}
- return arr || [];
+ return this.data.wiki;
},
- paragraph: function paragraph(n) {
- var arr = this.data.paragraphs || [];
+ type: function (str) {
+ if (str !== undefined) {
+ this.data.type = str;
+ }
- if (typeof n === 'number') {
- return arr[n];
+ return this.data.type;
+ },
+ site: function (str) {
+ if (str !== undefined) {
+ this.data.site = str;
}
- return arr[0];
+ return this.data.site;
},
- links: function links(n) {
- var arr = [];
- this.infoboxes().forEach(function (templ) {
- templ.links(n).forEach(function (link) {
- return arr.push(link);
- });
- });
- this.sentences().forEach(function (s) {
- s.links(n).forEach(function (link) {
- return arr.push(link);
- });
- });
- this.tables().forEach(function (t) {
- t.links(n).forEach(function (link) {
- return arr.push(link);
- });
- });
- this.lists().forEach(function (list) {
- list.links(n).forEach(function (link) {
- return arr.push(link);
- });
- });
-
- if (typeof n === 'number') {
- return arr[n];
- } else if (typeof n === 'string') {
- //grab a link like .links('Fortnight')
- n = n.charAt(0).toUpperCase() + n.substring(1); //titlecase it
-
- var link = arr.find(function (o) {
- return o.page() === n;
- });
- return link === undefined ? [] : [link];
- }
-
- return arr;
- },
- tables: function tables(clue) {
- var arr = this.data.tables || [];
-
- if (typeof clue === 'number') {
- return arr[clue];
- }
-
- return arr;
- },
- templates: function templates(clue) {
- var arr = this.data.templates || [];
- arr = arr.map(function (t) {
- return t.json();
- });
-
- if (typeof clue === 'number') {
- return arr[clue];
- }
-
- if (typeof clue === 'string') {
- clue = clue.toLowerCase();
- return arr.filter(function (o) {
- return o.template === clue || o.name === clue;
- });
- }
-
- return arr;
- },
- infoboxes: function infoboxes(clue) {
- var arr = this.data.infoboxes || [];
-
- if (typeof clue === 'number') {
- return arr[clue];
- }
-
- return arr;
- },
- coordinates: function coordinates(clue) {
- var arr = [].concat(this.templates('coord'), this.templates('coor'));
-
- if (typeof clue === 'number') {
- if (!arr[clue]) {
- return [];
- }
-
- return arr[clue];
- }
-
- return arr;
- },
- lists: function lists(clue) {
- var arr = [];
- this.paragraphs().forEach(function (p) {
- arr = arr.concat(p.lists());
- });
-
- if (typeof clue === 'number') {
- return arr[clue];
- }
-
- return arr;
- },
- interwiki: function interwiki(num) {
- var arr = [];
- this.paragraphs().forEach(function (p) {
- arr = arr.concat(p.interwiki());
- });
-
- if (typeof num === 'number') {
- return arr[num];
- }
-
- return arr || [];
- },
- images: function images(clue) {
- var arr = [];
- this.paragraphs().forEach(function (p) {
- arr = arr.concat(p.images());
- });
-
- if (typeof clue === 'number') {
- return arr[clue];
- }
-
- return arr || [];
- },
- references: function references(clue) {
- var arr = this.data.references || [];
-
- if (typeof clue === 'number') {
- return arr[clue];
- }
-
- return arr;
- },
- //transformations
- remove: function remove() {
- if (!this.doc) {
- return null;
- }
-
- var bads = {};
- bads[this.title()] = true; //remove children too
-
- this.children().forEach(function (sec) {
- return bads[sec.title()] = true;
- });
- var arr = this.doc.data.sections;
- arr = arr.filter(function (sec) {
- return bads.hasOwnProperty(sec.title()) !== true;
- });
- this.doc.data.sections = arr;
- return this.doc;
- },
- //move-around sections like in jquery
- nextSibling: function nextSibling() {
- if (!this.doc) {
- return null;
- }
-
- var sections = this.doc.sections();
- var index = this.index();
-
- for (var i = index + 1; i < sections.length; i += 1) {
- if (sections[i].depth < this.depth) {
- return null;
- }
-
- if (sections[i].depth === this.depth) {
- return sections[i];
- }
- }
-
- return null;
- },
- lastSibling: function lastSibling() {
- if (!this.doc) {
- return null;
- }
-
- var sections = this.doc.sections();
- var index = this.index();
- return sections[index - 1] || null;
- },
- children: function children(n) {
- if (!this.doc) {
- return null;
- }
-
- var sections = this.doc.sections();
- var index = this.index();
- var children = []; //(immediately preceding sections with higher depth)
-
- if (sections[index + 1] && sections[index + 1].depth > this.depth) {
- for (var i = index + 1; i < sections.length; i += 1) {
- if (sections[i].depth > this.depth) {
- children.push(sections[i]);
- } else {
- break;
- }
- }
- }
-
- if (typeof n === 'string') {
- n = n.toLowerCase();
- return children.find(function (s) {
- return s.title().toLowerCase() === n;
- });
- }
-
- if (typeof n === 'number') {
- return children[n];
- }
-
- return children;
- },
- parent: function parent() {
- if (!this.doc) {
- return null;
- }
-
- var sections = this.doc.sections();
- var index = this.index();
-
- for (var i = index; i >= 0; i -= 1) {
- if (sections[i] && sections[i].depth < this.depth) {
- return sections[i];
- }
- }
-
- return null;
- },
- text: function text(options) {
- options = setDefaults_1(options, defaults$5);
- var pList = this.paragraphs();
- pList = pList.map(function (p) {
- return p.text(options);
- });
- return pList.join('\n\n');
- },
- json: function json(options) {
- options = setDefaults_1(options, defaults$5);
- return toJson$2(this, options);
- }
- }; //aliases
-
- methods$2.next = methods$2.nextSibling;
- methods$2.last = methods$2.lastSibling;
- methods$2.previousSibling = methods$2.lastSibling;
- methods$2.previous = methods$2.lastSibling;
- methods$2.citations = methods$2.references;
- methods$2.sections = methods$2.children;
- Object.keys(methods$2).forEach(function (k) {
- Section.prototype[k] = methods$2[k];
- });
- var Section_1 = Section;
-
- var helpers = {
- capitalise: function capitalise(str) {
- if (str && typeof str === 'string') {
- return str.charAt(0).toUpperCase() + str.slice(1);
- }
-
- return '';
- },
- trim_whitespace: function trim_whitespace(str) {
- if (str && typeof str === 'string') {
- str = str.replace(/^\s\s*/, '');
- str = str.replace(/\s\s*$/, '');
- str = str.replace(/ {2}/, ' ');
- str = str.replace(/\s, /, ', ');
- return str;
- }
-
- return '';
- }
- };
-
- var defaults$6 = {
- type: 'internal'
- };
-
- var Link = function Link(data) {
- data = data || {};
- data = Object.assign({}, defaults$6, data); // console.log(data)
-
- Object.defineProperty(this, 'data', {
- enumerable: false,
- value: data
- });
- };
-
- var methods$3 = {
- text: function text(str) {
- if (str !== undefined) {
- this.data.text = str;
- }
-
- return this.data.text;
- },
- json: function json() {
- var obj = {
- text: this.text(),
- type: this.type()
- };
-
- if (obj.type === 'internal') {
- obj.page = this.page();
- } else if (obj.type === 'interwiki') {
- obj.wiki = this.wiki();
- } else {
- obj.site = this.site();
- }
-
- var anchor = this.anchor();
-
- if (anchor) {
- obj.anchor = anchor;
- }
-
- return obj;
- },
- page: function page(str) {
- if (str !== undefined) {
- this.data.page = str;
- }
-
- return this.data.page;
- },
- anchor: function anchor(str) {
- if (str !== undefined) {
- this.data.anchor = str;
- }
-
- return this.data.anchor || '';
- },
- wiki: function wiki(str) {
- if (str !== undefined) {
- this.data.wiki = str;
- }
-
- return this.data.wiki;
- },
- type: function type(str) {
- if (str !== undefined) {
- this.data.type = str;
- }
-
- return this.data.type;
- },
- site: function site(str) {
- if (str !== undefined) {
- this.data.site = str;
- }
-
- return this.data.site;
- },
- // create a url for any type of link
- href: function href() {
- var type = this.type();
+ //create a url for any type of link
+ href: function () {
+ let type = this.type();
if (type === 'external') {
return this.site();
}
- var page = this.page();
+ let page = this.page();
page = page.replace(/ /g, '_');
page = encodeURIComponent(page);
- var url = '';
+ let url = '';
if (type === 'interwiki') {
- var wiki = this.wiki();
+ let wiki = this.wiki();
url = 'https://en.wikipedia.org/wiki/$1';
- if (interwiki.hasOwnProperty(wiki)) {
- url = 'http://' + interwiki[this.wiki()];
+ if (interwiki$1.hasOwnProperty(wiki)) {
+ url = 'http://' + interwiki$1[this.wiki()];
}
url = url.replace(/\$1/g, page);
} else {
//internal link
- url = "./".concat(this.page());
- } // add anchor on the end
+ url = `./${this.page()}`;
+ } //add anchor on the end
if (this.anchor()) {
@@ -3038,26 +2600,26 @@
return url;
}
};
- Object.keys(methods$3).forEach(function (k) {
- Link.prototype[k] = methods$3[k];
+ Object.keys(methods$7).forEach(k => {
+ Link.prototype[k] = methods$7[k];
});
var Link_1 = Link;
// const cat_reg = new RegExp('\\[\\[:?(' + i18n.categories.join('|') + '):[^\\]\\]]{2,80}\\]\\]', 'gi')
//return only rendered text of wiki links
- var removeLinks = function removeLinks(line) {
+ const removeLinks = function (line) {
// [[File:with|Size]]
line = line.replace(/\[\[File:(.{2,80}?)\|([^\]]+?)\]\](\w{0,5})/g, '$1');
return line;
};
- var getLinks = function getLinks(data) {
- var wiki = data.text;
- var links = parse(wiki) || [];
- data.links = links.map(function (link) {
- wiki = wiki.replace(link.raw, link.text || link.page || '');
- delete link.raw;
+ const getLinks = function (data) {
+ let wiki = data.text;
+ let links = parse$8(wiki) || [];
+ data.links = links.map(link => {
+ wiki = wiki.replace(link.raw, link.text || link.page || ''); // delete link.raw
+
return new Link_1(link);
});
wiki = removeLinks(wiki);
@@ -3067,28 +2629,28 @@
var link = getLinks;
//handle the bold/italics
- var formatting = function formatting(obj) {
- var bolds = [];
- var italics = [];
- var wiki = obj.text || ''; //bold and italics combined 5 's
+ const formatting = function (obj) {
+ let bolds = [];
+ let italics = [];
+ let wiki = obj.text || ''; //bold and italics combined 5 's
- wiki = wiki.replace(/'''''(.{0,200}?)'''''/g, function (a, b) {
+ wiki = wiki.replace(/'''''(.{0,200}?)'''''/g, (a, b) => {
bolds.push(b);
italics.push(b);
return b;
}); //''''four'''' → bold with quotes
- wiki = wiki.replace(/''''(.{0,200}?)''''/g, function (a, b) {
- bolds.push("'".concat(b, "'"));
- return "'".concat(b, "'");
+ wiki = wiki.replace(/''''(.{0,200}?)''''/g, (a, b) => {
+ bolds.push(`'${b}'`);
+ return `'${b}'`;
}); //'''bold'''
- wiki = wiki.replace(/'''(.{0,200}?)'''/g, function (a, b) {
+ wiki = wiki.replace(/'''(.{0,200}?)'''/g, (a, b) => {
bolds.push(b);
return b;
}); //''italic''
- wiki = wiki.replace(/''(.{0,200}?)''/g, function (a, b) {
+ wiki = wiki.replace(/''(.{0,200}?)''/g, (a, b) => {
italics.push(b);
return b;
}); //pack it all up..
@@ -3110,18 +2672,18 @@
var formatting_1 = formatting;
- var isNumber = /^[0-9,.]+$/;
- var defaults$7 = {
+ const isNumber = /^[0-9,.]+$/;
+ const defaults$7 = {
text: true,
links: true,
formatting: true,
numbers: true
};
- var toJSON$2 = function toJSON(s, options) {
+ const toJSON$1 = function (s, options) {
options = setDefaults_1(options, defaults$7);
- var data = {};
- var text = s.text();
+ let data = {};
+ let text = s.text();
if (options.text === true) {
data.text = text;
@@ -3129,7 +2691,7 @@
if (options.numbers === true && isNumber.test(text)) {
- var num = Number(text.replace(/,/g, ''));
+ let num = Number(text.replace(/,/g, ''));
if (isNaN(num) === false) {
data.number = num;
@@ -3137,9 +2699,7 @@
}
if (options.links && s.links().length > 0) {
- data.links = s.links().map(function (l) {
- return l.json();
- });
+ data.links = s.links().map(l => l.json());
}
if (options.formatting && s.data.fmt) {
@@ -3149,80 +2709,47 @@
return data;
};
- var toJson$3 = toJSON$2;
+ var toJson$3 = toJSON$1;
- var Sentence = function Sentence(data) {
+ const Sentence = function (data = {}) {
Object.defineProperty(this, 'data', {
enumerable: false,
value: data
});
};
- var methods$4 = {
- links: function links(n) {
- var arr = this.data.links || [];
+ const methods$6 = {
+ links: function (n) {
+ let arr = this.data.links || [];
- if (typeof n === 'number') {
- return arr[n];
- } else if (typeof n === 'string') {
+ if (typeof n === 'string') {
//grab a link like .links('Fortnight')
n = n.charAt(0).toUpperCase() + n.substring(1); //titlecase it
- var link = arr.find(function (o) {
- return o.page === n;
- });
+ let link = arr.find(o => o.page === n);
return link === undefined ? [] : [link];
}
return arr;
},
- interwiki: function interwiki(n) {
- var arr = this.links().filter(function (l) {
- return l.wiki !== undefined;
- });
-
- if (typeof n === 'number') {
- return arr[n];
- }
-
- return arr;
+ interwiki: function () {
+ return this.links().filter(l => l.wiki !== undefined);
},
- bolds: function bolds(n) {
- var arr = [];
-
+ bolds: function () {
if (this.data && this.data.fmt && this.data.fmt.bold) {
- arr = this.data.fmt.bold || [];
- }
-
- if (typeof n === 'number') {
- return arr[n];
+ return this.data.fmt.bold || [];
}
- return arr;
+ return [];
},
- italics: function italics(n) {
- var arr = [];
-
+ italics: function () {
if (this.data && this.data.fmt && this.data.fmt.italic) {
- arr = this.data.fmt.italic || [];
- }
-
- if (typeof n === 'number') {
- return arr[n];
- }
-
- return arr;
- },
- dates: function dates(n) {
- var arr = [];
-
- if (typeof n === 'number') {
- return arr[n];
+ return this.data.fmt.italic || [];
}
- return arr;
+ return [];
},
- text: function text(str) {
+ text: function (str) {
if (str !== undefined && typeof str === 'string') {
//set the text?
this.data.text = str;
@@ -3230,15 +2757,38 @@
return this.data.text || '';
},
- json: function json(options) {
+ json: function (options) {
return toJson$3(this, options);
+ },
+ wikitext: function () {
+ return this.data.wiki || '';
+ },
+ isEmpty: function () {
+ return this.data.text === '';
}
};
- Object.keys(methods$4).forEach(function (k) {
- Sentence.prototype[k] = methods$4[k];
+ Object.keys(methods$6).forEach(k => {
+ Sentence.prototype[k] = methods$6[k];
+ }); // aliases
+
+ const singular$3 = {
+ links: 'link',
+ bolds: 'bold',
+ italics: 'italic'
+ };
+ Object.keys(singular$3).forEach(k => {
+ let sing = singular$3[k];
+
+ Sentence.prototype[sing] = function (clue) {
+ let arr = this[k](clue);
+
+ if (typeof clue === 'number') {
+ return arr[clue];
+ }
+
+ return arr[0];
+ };
});
- Sentence.prototype.italic = Sentence.prototype.italics;
- Sentence.prototype.bold = Sentence.prototype.bolds;
Sentence.prototype.plaintext = Sentence.prototype.text;
var Sentence_1 = Sentence;
@@ -3250,27 +2800,25 @@
// Ignore periods/questions/exclamations used in acronyms/abbreviations/numbers, etc.
// @spencermountain 2015 MIT
- var abbreviations = _abbreviations.concat('[^]][^]]');
- var abbrev_reg = new RegExp("(^| |')(" + abbreviations.join('|') + ")[.!?] ?$", 'i');
- var acronym_reg = new RegExp("[ |.|'|[][A-Z].? *?$", 'i');
- var elipses_reg = new RegExp('\\.\\.\\.* +?$');
- var circa_reg = / c\. $/;
- var hasWord = new RegExp('[a-zа-яぁ-ゟ][a-zа-яぁ-ゟ゠-ヿ]', 'iu'); //turn a nested array into one array
+ const abbreviations = _abbreviations.concat('[^]][^]]');
+ const abbrev_reg = new RegExp("(^| |')(" + abbreviations.join('|') + `)[.!?] ?$`, 'i');
+ const acronym_reg = /[ .'][A-Z].? *?$/i;
+ const elipses_reg = /\.\.\.* +?$/;
+ const circa_reg = / c\.\s$/;
+ const hasWord = /\p{Letter}/iu; //turn a nested array into one array
- var flatten = function flatten(arr) {
- var all = [];
+ const flatten = function (arr) {
+ let all = [];
arr.forEach(function (a) {
all = all.concat(a);
});
return all;
};
- var naiive_split = function naiive_split(text) {
+ const naiive_split = function (text) {
//first, split by newline
- var splits = text.split(/(\n+)/);
- splits = splits.filter(function (s) {
- return s.match(/\S/);
- }); //split by period, question-mark, and exclamation-mark
+ let splits = text.split(/(\n+)/);
+ splits = splits.filter(s => s.match(/\S/)); //split by period, question-mark, and exclamation-mark
splits = splits.map(function (str) {
return str.split(/(\S.+?[.!?]"?)(?=\s+|$)/g); //\u3002
@@ -3279,17 +2827,17 @@
}; // if this looks like a period within a wikipedia link, return false
- var isBalanced = function isBalanced(str) {
+ const isBalanced = function (str) {
str = str || '';
- var open = str.split(/\[\[/) || [];
- var closed = str.split(/\]\]/) || [];
+ const open = str.split(/\[\[/) || [];
+ const closed = str.split(/\]\]/) || [];
if (open.length > closed.length) {
return false;
} //make sure quotes are closed too
- var quotes = str.match(/"/g);
+ const quotes = str.match(/"/g);
if (quotes && quotes.length % 2 !== 0 && str.length < 900) {
return false;
@@ -3298,10 +2846,10 @@
return true;
};
- var sentence_parser = function sentence_parser(text) {
- var sentences = []; //first do a greedy-split..
+ const sentence_parser = function (text) {
+ let sentences = []; //first do a greedy-split..
- var chunks = []; //ensure it 'smells like' a sentence
+ let chunks = []; //ensure it 'smells like' a sentence
if (!text || typeof text !== 'string' || text.trim().length === 0) {
return sentences;
@@ -3310,10 +2858,10 @@
// todo: look for side effects in this regex replacement:
- var splits = naiive_split(text); //filter-out the grap ones
+ let splits = naiive_split(text); //filter-out the grap ones
- for (var i = 0; i < splits.length; i++) {
- var s = splits[i];
+ for (let i = 0; i < splits.length; i++) {
+ let s = splits[i];
if (!s || s === '') {
continue;
@@ -3336,7 +2884,7 @@
} //detection of non-sentence chunks
- var isSentence = function isSentence(hmm) {
+ const isSentence = function (hmm) {
if (hmm.match(abbrev_reg) || hmm.match(acronym_reg) || hmm.match(elipses_reg) || hmm.match(circa_reg)) {
return false;
} //too short? - no consecutive letters
@@ -3354,14 +2902,14 @@
}; //loop through these chunks, and join the non-sentence chunks back together..
- for (var _i = 0; _i < chunks.length; _i++) {
+ for (let i = 0; i < chunks.length; i++) {
//should this chunk be combined with the next one?
- if (chunks[_i + 1] && !isSentence(chunks[_i])) {
- chunks[_i + 1] = chunks[_i] + (chunks[_i + 1] || ''); //.replace(/ +/g, ' ');
- } else if (chunks[_i] && chunks[_i].length > 0) {
+ if (chunks[i + 1] && !isSentence(chunks[i])) {
+ chunks[i + 1] = chunks[i] + (chunks[i + 1] || ''); //.replace(/ +/g, ' ');
+ } else if (chunks[i] && chunks[i].length > 0) {
//this chunk is a proper sentence..
- sentences.push(chunks[_i]);
- chunks[_i] = '';
+ sentences.push(chunks[i]);
+ chunks[i] = '';
}
} //if we never got a sentence, return the given text
@@ -3373,7 +2921,15 @@
return sentences;
};
- var parse$2 = sentence_parser;
+ var parse$6 = sentence_parser;
+
+ /**
+ * This function removes some final characters from the sentence
+ *
+ * @private
+ * @param {string} line the wiki text for processing
+ * @returns {string} the processed string
+ */
function postprocess(line) {
//remove empty parentheses (sometimes caused by removing templates)
@@ -3384,11 +2940,18 @@
line = helpers.trim_whitespace(line);
line = line.replace(/ +\.$/, '.');
return line;
- } // returns one sentence object
+ }
+ /**
+ * returns one sentence object
+ *
+ * @param {string} str create a object from a sentence
+ * @returns {Sentence} the Sentence created from the text
+ */
function fromText(str) {
- var obj = {
+ let obj = {
+ wiki: str,
text: str
}; //pull-out the [[links]]
@@ -3401,9 +2964,9 @@
} //used for consistency with other class-definitions
- var byParagraph = function byParagraph(paragraph) {
- // array of texts
- var sentences = parse$2(paragraph.wiki); // sentence objects
+ const byParagraph = function (paragraph) {
+ //array of texts
+ let sentences = parse$6(paragraph.wiki); //sentence objects
sentences = sentences.map(fromText); //remove :indented first line, as it is often a disambiguation
@@ -3419,17 +2982,24 @@
byParagraph: byParagraph
};
- //remove the top/bottom off the template
- var strip = function strip(tmpl) {
- tmpl = tmpl.replace(/^\{\{/, '');
- tmpl = tmpl.replace(/\}\}$/, '');
+ /**
+ * removes the top and bottom off the template
+ * so it removes tje '{{' and '}}'
+ *
+ * @private
+ * @param {string} tmpl the string to be striped
+ * @returns {string} the striped string
+ */
+ const strip = function (tmpl) {
+ tmpl = tmpl.replace(/^{{/, '');
+ tmpl = tmpl.replace(/}}$/, '');
return tmpl;
};
var _strip = strip;
//normalize template names
- var fmtName = function fmtName(name) {
+ const fmtName = function (name) {
name = (name || '').trim();
name = name.toLowerCase();
name = name.replace(/_/g, ' ');
@@ -3438,33 +3008,36 @@
var _fmtName = fmtName;
- //turn {{name|one|two|three}} into [name, one, two, three]
- var pipeSplitter = function pipeSplitter(tmpl) {
- //start with a naiive '|' split
- var arr = tmpl.split(/\n?\|/); //we've split by '|', which is pretty lame
+ /**
+ * turn {{name|one|two|three}} into [name, one, two, three]
+ *
+ * @private
+ * @param {string} tmpl the template text
+ * @returns {string[]} a array containing all the split parameters
+ */
+ const pipeSplitter = function (tmpl) {
+ //start with a naive '|' split
+ let arr = tmpl.split(/\n?\|/); //we've split by '|', which is pretty lame
//look for broken-up links and fix them :/
- arr.forEach(function (a, i) {
+ arr.forEach((a, i) => {
if (a === null) {
return;
} //has '[[' but no ']]'
- //has equal number of openning and closing tags. handle nested case '[[[[' ']]'
+ //has equal number of opening and closing tags. handle nested case '[[[[' ']]'
- if (/\[\[[^\]]+$/.test(a) || /\{\{[^\}]+$/.test(a) || a.split('{{').length !== a.split('}}').length || a.split('[[').length !== a.split(']]').length) {
- arr[i + 1] = arr[i] + '|' + arr[i + 1];
+ if (/\[\[[^\]]+$/.test(a) || /{{[^}]+$/.test(a) || a.split('{{').length !== a.split('}}').length || a.split('[[').length !== a.split(']]').length) {
+ arr[i + 1] = arr[i] + '|' + arr[i + 1]; //@ts-expect-error we can ignore this error because we filter out all nulls later in
+
arr[i] = null;
}
}); //cleanup any mistakes we've made
- arr = arr.filter(function (a) {
- return a !== null;
- });
- arr = arr.map(function (a) {
- return (a || '').trim();
- }); //remove empty fields, only at the end:
+ arr = arr.filter(a => a !== null);
+ arr = arr.map(a => (a || '').trim()); //remove empty fields, only at the end:
- for (var i = arr.length - 1; i >= 0; i -= 1) {
+ for (let i = arr.length - 1; i >= 0; i -= 1) {
if (arr[i] === '') {
arr.pop();
}
@@ -3477,21 +3050,33 @@
var _01PipeSplitter = pipeSplitter;
- // every value in {{tmpl|a|b|c}} needs a name
- // here we come up with names for them
- var hasKey = /^[ '-\)\x2D\.0-9_a-z\xC0-\xFF\u0153\u017F\u1E9E\u212A\u212B]+=/i; //templates with these properties are asking for trouble
+ //every value in {{tmpl|a|b|c}} needs a name
+ //here we come up with names for them
+ const hasKey = /^[\p{Letter}0-9\._\- '()œ]+=/iu; //templates with these properties are asking for trouble
- var reserved = {
+ const reserved = {
template: true,
list: true,
prototype: true
- }; //turn 'key=val' into {key:key, val:val}
-
- var parseKey = function parseKey(str) {
- var parts = str.split('=');
- var key = parts[0] || '';
+ };
+ /**
+ * @typedef parseKeyReturn
+ * @property {string} val
+ * @property {string} key
+ */
+
+ /**
+ * turn 'key=val' into {key:key, val:val}
+ *
+ * @param {string} str the string that will be parsed
+ * @returns {parseKeyReturn} the spit string
+ */
+
+ const parseKey = function (str) {
+ let parts = str.split('=');
+ let key = parts[0] || '';
key = key.toLowerCase().trim();
- var val = parts.slice(1).join('='); //don't let it be called 'template'..
+ let val = parts.slice(1).join('='); //don't let it be called 'template'..
if (reserved.hasOwnProperty(key)) {
key = '_' + key;
@@ -3501,47 +3086,54 @@
key: key,
val: val.trim()
};
- }; //turn [a, b=v, c] into {'1':a, b:v, '2':c}
+ };
+ /**
+ * turn [a, b=v, c] into {'1':a, b:v, '2':c}
+ *
+ * @private
+ * @param {string[]} arr the array of parameters
+ * @param {string[]} [order] the order in which the parameters are returned
+ * @returns {object} and object with the names as the keys and the values as the values
+ */
- var keyMaker = function keyMaker(arr, order) {
- var o = 0;
- return arr.reduce(function (h, str) {
- str = (str || '').trim(); //support named keys - 'foo=bar'
+ const keyMaker = function (arr, order) {
+ let keyIndex = 0;
+ return arr.reduce((h, str = '') => {
+ str = str.trim(); //support named keys - 'foo=bar'
if (hasKey.test(str) === true) {
- var res = parseKey(str);
+ let res = parseKey(str);
if (res.key) {
h[res.key] = res.val;
return h;
}
- } //try a key from given 'order' names
-
+ } //if the current index is present in the order array then we have a name for the key
- if (order && order[o]) {
- var key = order[o]; //here goes!
+ if (order && order[keyIndex]) {
+ let key = order[keyIndex];
h[key] = str;
} else {
h.list = h.list || [];
h.list.push(str);
}
- o += 1;
+ keyIndex += 1;
return h;
}, {});
};
var _02KeyMaker = keyMaker;
- var whoCares = {
+ const whoCares = {
classname: true,
style: true,
align: true,
margin: true,
left: true,
- "break": true,
+ break: true,
boxsize: true,
framestyle: true,
item_style: true,
@@ -3551,8 +3143,8 @@
colwidth: true
}; //remove wiki-cruft & some styling info from templates
- var cleanup = function cleanup(obj) {
- Object.keys(obj).forEach(function (k) {
+ const cleanup$1 = function (obj) {
+ Object.keys(obj).forEach(k => {
if (whoCares[k.toLowerCase()] === true) {
delete obj[k];
} //remove empty values, too
@@ -3565,12 +3157,20 @@
return obj;
};
- var _03Cleanup = cleanup;
+ var _03Cleanup = cleanup$1;
- var parseSentence = _04Sentence.fromText; // most templates just want plaintext...
+ const parseSentence$7 = _04Sentence.fromText;
+ /**
+ * most templates just want plaintext...
+ *
+ * @private
+ * @param {str} str
+ * @param {'json' | 'raw'} [fmt]
+ * @returns {string} text
+ */
- var makeFormat = function makeFormat(str, fmt) {
- var s = parseSentence(str); //support various output formats
+ const makeFormat = function (str, fmt) {
+ let s = parseSentence$7(str); //support various output formats
if (fmt === 'json') {
return s.json();
@@ -3580,21 +3180,29 @@
return s.text();
- }; //
-
+ };
+ /**
+ * parses the parameters of a template to a usable format
+ *
+ * @private
+ * @param {string} tmpl the template text
+ * @param {string[]} [order] the order in which the parameters are returned
+ * @param {'json' | 'raw'} [fmt] whether you wan to parse the text of the template the raw object or just the text
+ * @returns {object} the parameters of the template in a usable format
+ */
- var parser = function parser(tmpl, order, fmt) {
- order = order || []; //renomove {{}}'s
+ const parser = function (tmpl, order = [], fmt) {
+ //remove {{}}'s and split based on pipes
tmpl = _strip(tmpl || '');
- var arr = _01PipeSplitter(tmpl); //get template name
+ let arr = _01PipeSplitter(tmpl); //get template name
- var name = arr.shift(); //name each value
+ let name = arr.shift(); //name each value
- var obj = _02KeyMaker(arr, order); //remove wiki-junk
+ let obj = _02KeyMaker(arr, order); //remove wiki-junk
obj = _03Cleanup(obj); //is this a infobox/reference?
- // let known = isKnown(obj);
+ //let known = isKnown(obj);
//using '|1=content' is an escaping-thing..
if (obj['1'] && order[0] && obj.hasOwnProperty(order[0]) === false) {
@@ -3603,11 +3211,9 @@
delete obj['1'];
}
- Object.keys(obj).forEach(function (k) {
+ Object.keys(obj).forEach(k => {
if (k === 'list') {
- obj[k] = obj[k].map(function (v) {
- return makeFormat(v, fmt);
- });
+ obj[k] = obj[k].map(v => makeFormat(v, fmt));
return;
}
@@ -3621,22 +3227,26 @@
return obj;
};
- var parse$3 = parser;
+ var toJSON = parser;
- var Reference = function Reference(data) {
+ const Reference = function (data, wiki) {
Object.defineProperty(this, 'data', {
enumerable: false,
value: data
});
+ Object.defineProperty(this, 'wiki', {
+ enumerable: false,
+ value: wiki
+ });
};
- var methods$5 = {
- title: function title() {
- var data = this.data;
+ const methods$5 = {
+ title: function () {
+ let data = this.data;
return data.title || data.encyclopedia || data.author || '';
},
- links: function links(n) {
- var arr = [];
+ links: function (n) {
+ let arr = [];
if (typeof n === 'number') {
return arr[n];
@@ -3649,20 +3259,20 @@
//grab a link like .links('Fortnight')
n = n.charAt(0).toUpperCase() + n.substring(1); //titlecase it
- var link = arr.find(function (o) {
- return o.page() === n;
- });
+ let link = arr.find(o => o.page() === n);
return link === undefined ? [] : [link];
}
return arr || [];
},
- text: function text() {
+ text: function () {
return ''; //nah, skip these.
},
- json: function json() {
- var options = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {};
- var json = this.data || {}; //encode them, for mongodb
+ wikitext: function () {
+ return this.wiki || '';
+ },
+ json: function (options = {}) {
+ let json = this.data || {}; //encode them, for mongodb
if (options.encode === true) {
json = Object.assign({}, json);
@@ -3672,68 +3282,80 @@
return json;
}
};
- Object.keys(methods$5).forEach(function (k) {
+ Object.keys(methods$5).forEach(k => {
Reference.prototype[k] = methods$5[k];
});
var Reference_1 = Reference;
- var parseSentence$1 = _04Sentence.fromText; //structured Cite templates - [{{Cite..]
+ const parseSentence$6 = _04Sentence.fromText; //structured Cite templates - [{{Cite..]
- var hasCitation = function hasCitation(str) {
+ const hasCitation = function (str) {
return /^ *?\{\{ *?(cite|citation)/i.test(str) && /\}\} *?$/.test(str) && /citation needed/i.test(str) === false;
};
- var parseCitation = function parseCitation(tmpl) {
- var obj = parse$3(tmpl);
+ const parseCitation = function (tmpl) {
+ let obj = toJSON(tmpl);
obj.type = obj.template.replace(/cite /, '');
obj.template = 'citation';
return obj;
}; //handle unstructured ones - [some text]
- var parseInline = function parseInline(str) {
- var obj = parseSentence$1(str) || {};
+ const parseInline = function (str) {
+ let obj = parseSentence$6(str) || {};
return {
template: 'citation',
type: 'inline',
data: {},
inline: obj
};
- }; // parse xml tags
+ }; //parse xml tags
- var parseRefs = function parseRefs(section) {
- var references = [];
- var wiki = section.wiki;
- wiki = wiki.replace(/ ?[([\s\S]{0,1800}?)<\/ref> ?/gi, function (a, tmpl) {
+ const parseRefs = function (section) {
+ let references = [];
+ let wiki = section._wiki;
+ wiki = wiki.replace(/ ?][([\s\S]{0,1800}?)<\/ref> ?/gi, function (all, tmpl) {
if (hasCitation(tmpl)) {
- var obj = parseCitation(tmpl);
+ let obj = parseCitation(tmpl);
if (obj) {
- references.push(obj);
+ references.push({
+ json: obj,
+ wiki: all
+ });
}
wiki = wiki.replace(tmpl, '');
} else {
- references.push(parseInline(tmpl));
+ references.push({
+ json: parseInline(tmpl),
+ wiki: all
+ });
}
return ' ';
- }); // ]
+ }); //
- wiki = wiki.replace(/ ?[]{0,200}?\/> ?/gi, ' '); // ]
+ wiki = wiki.replace(/ ?[]{0,200}?\/> ?/gi, ' '); //]
- wiki = wiki.replace(/ ?[]{0,200}?>([\s\S]{0,1800}?)<\/ref> ?/gi, function (a, tmpl) {
+ wiki = wiki.replace(/ ?][]{0,200}?>([\s\S]{0,1800}?)<\/ref> ?/gi, function (all, tmpl) {
if (hasCitation(tmpl)) {
- var obj = parseCitation(tmpl);
+ let obj = parseCitation(tmpl);
if (obj) {
- references.push(obj);
+ references.push({
+ json: obj,
+ wiki: tmpl
+ });
}
wiki = wiki.replace(tmpl, '');
} else {
- references.push(parseInline(tmpl));
+ references.push({
+ json: parseInline(tmpl),
+ wiki: all
+ });
}
return ' ';
@@ -3741,19 +3363,35 @@
wiki = wiki.replace(/ ?<[ \/]?[a-z0-9]{1,8}[a-z0-9=" ]{2,20}[ \/]?> ?/g, ' '); //
- section.references = references.map(function (r) {
- return new Reference_1(r);
- });
- section.wiki = wiki;
+ section._references = references.map(obj => new Reference_1(obj.json, obj.wiki));
+ section._wiki = wiki;
};
var reference = parseRefs;
- var parseSentence$2 = _04Sentence.fromText;
- var heading_reg = /^(={1,5})(.{1,200}?)={1,5}$/; //interpret depth, title of headings like '==See also=='
-
- var parseHeading = function parseHeading(section, str) {
- var m = str.match(heading_reg);
+ const {
+ trim_whitespace
+ } = helpers;
+ const parseSentence$5 = _04Sentence.fromText;
+ const heading_reg = /^(={1,5})(.{1,200}?)={1,5}$/;
+ /**
+ * @typedef fakeSection
+ * @property {string} title
+ * @property {null | number} depth
+ * @property {string} wiki
+ */
+
+ /**
+ * estimates the depth of a section and parses the title to a normal format
+ *
+ * @private
+ * @param {fakeSection} section
+ * @param {string} str
+ * @returns {fakeSection} section the depth in a object
+ */
+
+ const parseHeading = function (section, str) {
+ let m = str.match(heading_reg);
if (!m) {
section.title = '';
@@ -3761,20 +3399,20 @@
return section;
}
- var title = m[2] || '';
- title = parseSentence$2(title).text(); //amazingly, you can see inline {{templates}} in this text, too
+ let title = m[2] || '';
+ title = parseSentence$5(title).text(); //amazingly, you can see inline {{templates}} in this text, too
//... let's not think about that now.
title = title.replace(/\{\{.+?\}\}/, ''); //same for references (i know..)
- var obj = {
- wiki: title
+ let obj = {
+ _wiki: title
};
reference(obj);
- title = obj.wiki; //trim leading/trailing whitespace
+ title = obj._wiki; //trim leading/trailing whitespace
- title = helpers.trim_whitespace(title);
- var depth = 0;
+ title = trim_whitespace(title);
+ let depth = 0;
if (m[1]) {
depth = m[1].length - 2;
@@ -3788,8 +3426,8 @@
var heading = parseHeading;
//remove top-bottoms
- var cleanup$1 = function cleanup(lines) {
- lines = lines.filter(function (line) {
+ const cleanup = function (lines) {
+ lines = lines.filter(line => {
//a '|+' row is a 'table caption', remove it.
return line && /^\|\+/.test(line) !== true;
});
@@ -3810,13 +3448,13 @@
}; //turn newline seperated into '|-' seperated
- var findRows = function findRows(lines) {
- var rows = [];
- var row = [];
- lines = cleanup$1(lines);
+ const findRows = function (lines) {
+ let rows = [];
+ let row = [];
+ lines = cleanup(lines);
- for (var i = 0; i < lines.length; i += 1) {
- var line = lines[i]; //'|-' is a row-seperator
+ for (let i = 0; i < lines.length; i += 1) {
+ let line = lines[i]; //'|-' is a row-seperator
if (/^\|-/.test(line) === true) {
//okay, we're done the row
@@ -3832,7 +3470,7 @@
line.shift();
}
- line.forEach(function (l) {
+ line.forEach(l => {
l = l.replace(/^\| */, '');
l = l.trim();
row.push(l);
@@ -3850,16 +3488,16 @@
var _findRows = findRows;
- var getRowSpan = /.*rowspan *?= *?["']?([0-9]+)["']?[ \|]*/;
- var getColSpan = /.*colspan *?= *?["']?([0-9]+)["']?[ \|]*/; //colspans stretch ←left/right→
+ const getRowSpan = /.*rowspan *?= *?["']?([0-9]+)["']?[ \|]*/;
+ const getColSpan = /.*colspan *?= *?["']?([0-9]+)["']?[ \|]*/; //colspans stretch ←left/right→
- var doColSpan = function doColSpan(rows) {
- rows.forEach(function (row) {
- row.forEach(function (str, c) {
- var m = str.match(getColSpan);
+ const doColSpan = function (rows) {
+ rows.forEach(row => {
+ row.forEach((str, c) => {
+ let m = str.match(getColSpan);
if (m !== null) {
- var num = parseInt(m[1], 10); //...maybe if num is so big, and centered, remove it?
+ let num = parseInt(m[1], 10); //...maybe if num is so big, and centered, remove it?
// if (num > 3) {
// rows[r] = []
// return
@@ -3868,31 +3506,29 @@
row[c] = str.replace(getColSpan, '');
- for (var i = 1; i < num; i += 1) {
+ for (let i = 1; i < num; i += 1) {
row.splice(c + 1, 0, '');
}
}
});
});
- rows = rows.filter(function (r) {
- return r.length > 0;
- });
+ rows = rows.filter(r => r.length > 0);
return rows;
}; //colspans stretch up/down
- var doRowSpan = function doRowSpan(rows) {
- rows.forEach(function (row, r) {
- row.forEach(function (str, c) {
- var m = str.match(getRowSpan);
+ const doRowSpan = function (rows) {
+ rows.forEach((row, r) => {
+ row.forEach((str, c) => {
+ let m = str.match(getRowSpan);
if (m !== null) {
- var num = parseInt(m[1], 10); //copy this cell down n rows
+ let num = parseInt(m[1], 10); //copy this cell down n rows
str = str.replace(getRowSpan, '');
row[c] = str;
- for (var i = r + 1; i < r + num; i += 1) {
+ for (let i = r + 1; i < r + num; i += 1) {
if (!rows[i]) {
break;
}
@@ -3906,7 +3542,7 @@
}; //
- var handleSpans = function handleSpans(rows) {
+ const handleSpans = function (rows) {
rows = doColSpan(rows);
rows = doRowSpan(rows);
return rows;
@@ -3914,10 +3550,10 @@
var _spans = handleSpans;
- var parseSentence$3 = _04Sentence.fromText;
- var isHeading = /^!/; //common ones
+ const parseSentence$4 = _04Sentence.fromText;
+ const isHeading = /^!/; //common ones
- var headings = {
+ const headings$1 = {
name: true,
age: true,
born: true,
@@ -3930,8 +3566,8 @@
number: true
}; //additional table-cruft to remove before parseLine method
- var cleanText = function cleanText(str) {
- str = parseSentence$3(str).text(); //anything before a single-pipe is styling, so remove it
+ const cleanText = function (str) {
+ str = parseSentence$4(str).text(); //anything before a single-pipe is styling, so remove it
if (str.match(/\|/)) {
str = str.replace(/.+\| ?/, ''); //class="unsortable"|title
@@ -3939,17 +3575,17 @@
str = str.replace(/style=['"].*?["']/, ''); //'!' is used as a highlighed-column
- str = str.replace(/^!/, '');
- str = str.trim();
+ str = str.replace(/^!/, ''); // str = str.replace(/\(.*?\)/, '')
+
+ str = str.trim(); // str = str.toLowerCase()
+
return str;
};
- var skipSpanRow = function skipSpanRow(row) {
+ const skipSpanRow = function (row) {
row = row || [];
- var len = row.length;
- var hasTxt = row.filter(function (str) {
- return str;
- }).length; //does it have 3 empty spaces?
+ let len = row.length;
+ let hasTxt = row.filter(str => str).length; //does it have 3 empty spaces?
if (len - hasTxt > 3) {
return true;
@@ -3959,8 +3595,8 @@
}; //remove non-header span rows
- var removeMidSpans = function removeMidSpans(rows) {
- rows = rows.filter(function (row) {
+ const removeMidSpans = function (rows) {
+ rows = rows.filter(row => {
if (row.length === 1 && row[0] && isHeading.test(row[0]) && /rowspan/i.test(row[0]) === false) {
return false;
}
@@ -3971,18 +3607,17 @@
}; //'!' starts a header-row
- var findHeaders = function findHeaders() {
- var rows = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : [];
- var headers = []; // is the first-row just a ton of colspan?
+ const findHeaders = function (rows = []) {
+ let headers = []; // is the first-row just a ton of colspan?
if (skipSpanRow(rows[0])) {
rows.shift();
}
- var first = rows[0];
+ let first = rows[0];
if (first && first[0] && first[1] && (/^!/.test(first[0]) || /^!/.test(first[1]))) {
- headers = first.map(function (h) {
+ headers = first.map(h => {
h = h.replace(/^\! */, '');
h = cleanText(h);
return h;
@@ -3994,7 +3629,7 @@
first = rows[0];
if (first && first[0] && first[1] && /^!/.test(first[0]) && /^!/.test(first[1])) {
- first.forEach(function (h, i) {
+ first.forEach((h, i) => {
h = h.replace(/^\! */, '');
h = cleanText(h);
@@ -4009,11 +3644,11 @@
}; //turn headers, array into an object
- var parseRow = function parseRow(arr, headers) {
- var row = {};
- arr.forEach(function (str, i) {
- var h = headers[i] || 'col' + (i + 1);
- var s = parseSentence$3(str);
+ const parseRow = function (arr, headers) {
+ let row = {};
+ arr.forEach((str, i) => {
+ let h = headers[i] || 'col' + (i + 1);
+ let s = parseSentence$4(str);
s.text(cleanText(s.text()));
row[h] = s;
});
@@ -4021,22 +3656,22 @@
}; //should we use the first row as a the headers?
- var firstRowHeader = function firstRowHeader(rows) {
+ const firstRowHeader = function (rows) {
if (rows.length <= 3) {
return [];
}
- var headers = rows[0].slice(0);
- headers = headers.map(function (h) {
+ let headers = rows[0].slice(0);
+ headers = headers.map(h => {
h = h.replace(/^\! */, '');
- h = parseSentence$3(h).text();
+ h = parseSentence$4(h).text();
h = cleanText(h);
h = h.toLowerCase();
return h;
});
- for (var i = 0; i < headers.length; i += 1) {
- if (headings.hasOwnProperty(headers[i])) {
+ for (let i = 0; i < headers.length; i += 1) {
+ if (headings$1.hasOwnProperty(headers[i])) {
rows.shift();
return headers;
}
@@ -4046,15 +3681,11 @@
}; //turn a {|...table string into an array of arrays
- var parseTable = function parseTable(wiki) {
- var lines = wiki.replace(/\r/g, '').replace(/\n(\s*[^|!{\s])/g, ' $1') //remove unecessary newlines
- .split(/\n/).map(function (l) {
- return l.trim();
- });
- var rows = _findRows(lines);
- rows = rows.filter(function (r) {
- return r;
- });
+ const parseTable = function (wiki) {
+ let lines = wiki.replace(/\r/g, '').replace(/\n(\s*[^|!{\s])/g, ' $1') //remove unecessary newlines
+ .split(/\n/).map(l => l.trim());
+ let rows = _findRows(lines);
+ rows = rows.filter(r => r);
if (rows.length === 0) {
return [];
@@ -4065,11 +3696,11 @@
rows = _spans(rows); //grab the header rows
- var headers = findHeaders(rows);
+ let headers = findHeaders(rows);
if (!headers || headers.length <= 1) {
headers = firstRowHeader(rows);
- var want = rows[rows.length - 1] || []; //try the second row
+ let want = rows[rows.length - 1] || []; //try the second row
if (headers.length <= 1 && want.length > 2) {
headers = firstRowHeader(rows.slice(1));
@@ -4081,18 +3712,18 @@
} //index each column by it's header
- var table = rows.map(function (arr) {
+ let table = rows.map(arr => {
return parseRow(arr, headers);
});
return table;
};
- var parse$4 = parseTable;
+ var parse$5 = parseTable;
- var toJson$4 = function toJson(tables, options) {
- return tables.map(function (table) {
- var row = {};
- Object.keys(table).forEach(function (k) {
+ const toJson$2 = function (tables, options) {
+ return tables.map(table => {
+ let row = {};
+ Object.keys(table).forEach(k => {
row[k] = table[k].json(); //(they're sentence objects)
}); //encode them, for mongodb
@@ -4104,74 +3735,121 @@
});
};
- var toJson_1$1 = toJson$4;
+ var toJson_1$2 = toJson$2;
- var defaults$8 = {};
+ const defaults$6 = {};
+
+ const normalize$1 = function (key = '') {
+ key = key.toLowerCase();
+ key = key.replace(/[_-]/g, ' ');
+ key = key.replace(/\(.*?\)/, '');
+ key = key.trim();
+ return key;
+ };
- var Table = function Table(data) {
+ const Table = function (data, wiki = '') {
Object.defineProperty(this, 'data', {
enumerable: false,
value: data
});
+ Object.defineProperty(this, '_wiki', {
+ enumerable: false,
+ value: wiki
+ });
};
- var methods$6 = {
- links: function links(n) {
- var links = [];
- this.data.forEach(function (r) {
- Object.keys(r).forEach(function (k) {
+ const methods$4 = {
+ links(n) {
+ let links = [];
+ this.data.forEach(r => {
+ Object.keys(r).forEach(k => {
links = links.concat(r[k].links());
});
- }); //grab a specific link..
+ });
- if (typeof n === 'number') {
- return links[n];
- } else if (typeof n === 'string') {
+ if (typeof n === 'string') {
//grab a link like .links('Fortnight')
n = n.charAt(0).toUpperCase() + n.substring(1); //titlecase it
- var link = links.find(function (o) {
- return o.page() === n;
- });
+ let link = links.find(o => o.page() === n);
return link === undefined ? [] : [link];
}
return links;
},
- keyValue: function keyValue(options) {
- var rows = this.json(options);
- rows.forEach(function (row) {
- Object.keys(row).forEach(function (k) {
+
+ get(keys) {
+ // normalize mappings
+ let have = this.data[0] || {};
+ let mapping = Object.keys(have).reduce((h, k) => {
+ h[normalize$1(k)] = k;
+ return h;
+ }, {}); // string gets a flat-list
+
+ if (typeof keys === 'string') {
+ let key = normalize$1(keys);
+ key = mapping[key] || key;
+ return this.data.map(row => {
+ return row[key] ? row[key].text() : null;
+ });
+ } // array gets obj-list
+
+
+ keys = keys.map(normalize$1).map(k => mapping[k] || k);
+ return this.data.map(row => {
+ return keys.reduce((h, k) => {
+ if (row[k]) {
+ h[k] = row[k].text();
+ } else {
+ h[k] = '';
+ }
+
+ return h;
+ }, {});
+ });
+ },
+
+ keyValue(options) {
+ let rows = this.json(options);
+ rows.forEach(row => {
+ Object.keys(row).forEach(k => {
row[k] = row[k].text;
});
});
return rows;
},
- json: function json(options) {
- options = setDefaults_1(options, defaults$8);
- return toJson_1$1(this.data, options);
+
+ json(options) {
+ options = setDefaults_1(options, defaults$6);
+ return toJson_1$2(this.data, options);
},
- text: function text() {
+
+ text() {
return '';
+ },
+
+ wikitext() {
+ return this._wiki || '';
}
+
};
- methods$6.keyvalue = methods$6.keyValue;
- methods$6.keyval = methods$6.keyValue;
- Object.keys(methods$6).forEach(function (k) {
- Table.prototype[k] = methods$6[k];
+ methods$4.keyvalue = methods$4.keyValue;
+ methods$4.keyval = methods$4.keyValue;
+ Object.keys(methods$4).forEach(k => {
+ Table.prototype[k] = methods$4[k];
});
var Table_1 = Table;
- var openReg = /^\s*{\|/;
- var closeReg = /^\s*\|}/; //tables can be recursive, so looky-here.
+ const openReg = /^\s*{\|/;
+ const closeReg = /^\s*\|}/; //tables can be recursive, so looky-here.
- var findTables = function findTables(section) {
- var list = [];
- var wiki = section.wiki;
- var lines = wiki.split('\n');
- var stack = [];
+ const findTables = function (section) {
+ let list = [];
+ let wiki = section._wiki;
+ let lines = wiki.split('\n');
+ let stack = [];
- for (var i = 0; i < lines.length; i += 1) {
+ for (let i = 0; i < lines.length; i += 1) {
//start a table
if (openReg.test(lines[i]) === true) {
stack.push(lines[i]);
@@ -4181,10 +3859,8 @@
if (closeReg.test(lines[i]) === true) {
stack[stack.length - 1] += '\n' + lines[i];
-
- var _table = stack.pop();
-
- list.push(_table);
+ let table = stack.pop();
+ list.push(table);
continue;
} //keep-going on one
@@ -4195,157 +3871,164 @@
} //work-em together for a Table class
- var tables = [];
- list.forEach(function (str) {
+ let tables = [];
+ list.forEach(str => {
if (str) {
- //also reremove a newline at the end of the table (awkward)
+ //also re-remove a newline at the end of the table (awkward)
wiki = wiki.replace(str + '\n', '');
wiki = wiki.replace(str, '');
- var data = parse$4(str);
+ let data = parse$5(str);
if (data && data.length > 0) {
- tables.push(new Table_1(data));
+ tables.push(new Table_1(data, str));
}
}
});
if (tables.length > 0) {
- section.tables = tables;
+ section._tables = tables;
}
- section.wiki = wiki;
+ section._wiki = wiki;
};
var table = findTables;
- var defaults$9 = {
+ const defaults$5 = {
sentences: true
};
- var toJson$5 = function toJson(p, options) {
- options = setDefaults_1(options, defaults$9);
- var data = {};
+ const toJson$1 = function (p, options) {
+ options = setDefaults_1(options, defaults$5);
+ let data = {};
if (options.sentences === true) {
- data.sentences = p.sentences().map(function (s) {
- return s.json(options);
- });
+ data.sentences = p.sentences().map(s => s.json(options));
}
return data;
};
- var toJson_1$2 = toJson$5;
+ var toJson_1$1 = toJson$1;
- var defaults$a = {
+ const defaults$4 = {
sentences: true,
lists: true,
images: true
};
- var Paragraph = function Paragraph(data) {
+ const Paragraph = function (data) {
Object.defineProperty(this, 'data', {
enumerable: false,
value: data
});
};
- var methods$7 = {
- sentences: function sentences(num) {
- if (typeof num === 'number') {
- return this.data.sentences[num];
- }
-
+ const methods$3 = {
+ sentences: function () {
return this.data.sentences || [];
},
- references: function references(num) {
- if (typeof num === 'number') {
- return this.data.references[num];
- }
-
+ references: function () {
return this.data.references;
},
- lists: function lists(num) {
- if (typeof num === 'number') {
- return this.data.lists[num];
- }
-
+ lists: function () {
return this.data.lists;
},
- images: function images(num) {
- if (typeof num === 'number') {
- return this.data.images[num];
- }
+ images() {
return this.data.images || [];
},
- links: function links(n) {
- var arr = [];
- this.sentences().forEach(function (s) {
- arr = arr.concat(s.links(n));
+
+ links: function (clue) {
+ let arr = [];
+ this.sentences().forEach(s => {
+ arr = arr.concat(s.links(clue));
});
- if (typeof n === 'number') {
- return arr[n];
- } else if (typeof n === 'string') {
+ if (typeof clue === 'string') {
//grab a specific link like .links('Fortnight')
- n = n.charAt(0).toUpperCase() + n.substring(1); //titlecase it
+ clue = clue.charAt(0).toUpperCase() + clue.substring(1); //titlecase it
- var link = arr.find(function (o) {
- return o.page() === n;
- });
+ let link = arr.find(o => o.page() === clue);
return link === undefined ? [] : [link];
}
return arr || [];
},
- interwiki: function interwiki(num) {
- var arr = [];
- this.sentences().forEach(function (s) {
+
+ interwiki() {
+ let arr = [];
+ this.sentences().forEach(s => {
arr = arr.concat(s.interwiki());
});
-
- if (typeof num === 'number') {
- return arr[num];
- }
-
return arr || [];
},
- text: function text(options) {
- options = setDefaults_1(options, defaults$a);
- var str = this.sentences().map(function (s) {
- return s.text(options);
- }).join(' ');
- this.lists().forEach(function (list) {
+
+ text: function (options) {
+ options = setDefaults_1(options, defaults$4);
+ let str = this.sentences().map(s => s.text(options)).join(' ');
+ this.lists().forEach(list => {
str += '\n' + list.text();
});
return str;
},
- json: function json(options) {
- options = setDefaults_1(options, defaults$a);
- return toJson_1$2(this, options);
+ json: function (options) {
+ options = setDefaults_1(options, defaults$4);
+ return toJson_1$1(this, options);
+ },
+ wikitext: function () {
+ return this.data.wiki;
}
};
- methods$7.citations = methods$7.references;
- Object.keys(methods$7).forEach(function (k) {
- Paragraph.prototype[k] = methods$7[k];
+ methods$3.citations = methods$3.references;
+ Object.keys(methods$3).forEach(k => {
+ Paragraph.prototype[k] = methods$3[k];
+ }); // aliases
+
+ const singular$2 = {
+ sentences: 'sentence',
+ references: 'reference',
+ citation: 'citations',
+ lists: 'list',
+ images: 'image',
+ links: 'link'
+ };
+ Object.keys(singular$2).forEach(k => {
+ let sing = singular$2[k];
+
+ Paragraph.prototype[sing] = function (clue) {
+ let arr = this[k](clue);
+
+ if (typeof clue === 'number') {
+ return arr[clue];
+ }
+
+ return arr[0];
+ };
});
var Paragraph_1 = Paragraph;
- //find all the pairs of '[[...[[..]]...]]' in the text
- //used to properly root out recursive template calls, [[.. [[...]] ]]
- //basically just adds open tags, and subtracts closing tags
- var opener = '[';
- var closer = ']';
+ const opener = '[';
+ const closer = ']';
+ /**
+ *
+ * find all the pairs of '[[...[[..]]...]]' in the text
+ * used to properly root out recursive template calls, [[.. [[...]] ]]
+ * basically just adds open tags, and subtracts closing tags
+ *
+ * @private
+ * @param {string} text the text in which is searched in
+ * @returns {string[]} all the links in the text
+ */
function nested_find(text) {
- var out = [];
- var last = [];
- var chars = text.split('');
- var open = 0;
+ let out = [];
+ let last = [];
+ const chars = text.split('');
+ let open = 0;
- for (var i = 0; i < chars.length; i++) {
- var c = text[i]; //increment open tag
+ for (let i = 0; i < chars.length; i++) {
+ const c = text[i]; //increment open tag
if (c === opener) {
open += 1;
@@ -4357,8 +4040,8 @@
open = 0;
}
} else if (last.length === 0) {
- // If we're not inside of a pair of delimiters, we can discard the current letter.
- // The return of this function is only used to extract images.
+ //If we're not inside of a pair of delimiters, we can discard the current letter.
+ //The return of this function is only used to extract images.
continue;
}
@@ -4366,10 +4049,10 @@
if (open === 0 && last.length > 0) {
//first, fix botched parse
- var open_count = 0;
- var close_count = 0;
+ let open_count = 0;
+ let close_count = 0;
- for (var j = 0; j < last.length; j++) {
+ for (let j = 0; j < last.length; j++) {
if (last[j] === opener) {
open_count++;
} else if (last[j] === closer) {
@@ -4393,13 +4076,13 @@
var nested_find_1 = nested_find;
- var parseSentence$4 = _04Sentence.fromText; //regexes:
+ const parseSentence$3 = _04Sentence.fromText; //regexes:
- var isFile = new RegExp('(' + i18n$1.images.join('|') + '):', 'i');
- var fileNames = "(".concat(i18n$1.images.join('|'), ")");
- var file_reg = new RegExp(fileNames + ':(.+?)[\\||\\]]', 'iu'); //style directives for Wikipedia:Extended_image_syntax
+ const isFile = new RegExp('(' + i18n.images.join('|') + '):', 'i');
+ let fileNames = `(${i18n.images.join('|')})`;
+ const file_reg = new RegExp(fileNames + ':(.+?)[\\||\\]]', 'iu'); //style directives for Wikipedia:Extended_image_syntax
- var imgLayouts = {
+ const imgLayouts = {
thumb: true,
thumbnail: true,
border: true,
@@ -4413,48 +4096,47 @@
baseline: true,
middle: true,
sub: true,
- "super": true
+ super: true
}; //images are usually [[image:my_pic.jpg]]
- var oneImage = function oneImage(img, doc) {
- var m = img.match(file_reg);
+ const oneImage = function (img, doc) {
+ let m = img.match(file_reg);
if (m === null || !m[2]) {
return null;
}
- var file = "".concat(m[1], ":").concat(m[2] || '');
+ let file = `${m[1]}:${m[2] || ''}`;
file = file.trim(); //titlecase it
- var title = file.charAt(0).toUpperCase() + file.substring(1); //spaces to underscores
+ let title = file.charAt(0).toUpperCase() + file.substring(1); //spaces to underscores
title = title.replace(/ /g, '_');
if (title) {
- var obj = {
+ let obj = {
file: file,
- lang: doc.lang,
- domain: doc.domain
+ lang: doc._lang,
+ domain: doc._domain,
+ wiki: img
}; //try to grab other metadata, too
img = img.replace(/^\[\[/, '');
img = img.replace(/\]\]$/, ''); //https://en.wikipedia.org/wiki/Wikipedia:Extended_image_syntax
- // - [[File:Name|Type|Border|Location|Alignment|Size|link=Link|alt=Alt|lang=Langtag|Caption]]
+ //- [[File:Name|Type|Border|Location|Alignment|Size|link=Link|alt=Alt|lang=Langtag|Caption]]
- var imgData = parse$3(img);
- var arr = imgData.list || []; //parse-out alt text, if explicitly given
+ let imgData = toJSON(img);
+ let arr = imgData.list || []; //parse-out alt text, if explicitly given
if (imgData.alt) {
obj.alt = imgData.alt;
} //remove 'thumb' and things
- arr = arr.filter(function (str) {
- return imgLayouts.hasOwnProperty(str) === false;
- });
+ arr = arr.filter(str => imgLayouts.hasOwnProperty(str) === false);
if (arr[arr.length - 1]) {
- obj.caption = parseSentence$4(arr[arr.length - 1]);
+ obj.caption = parseSentence$3(arr[arr.length - 1]);
}
return new Image_1(obj);
@@ -4463,14 +4145,14 @@
return null;
};
- var parseImages = function parseImages(paragraph, doc) {
- var wiki = paragraph.wiki; //parse+remove scary '[[ [[]] ]]' stuff
+ const parseImages = function (paragraph, doc) {
+ let wiki = paragraph.wiki; //parse+remove scary '[[ [[]] ]]' stuff
- var matches = nested_find_1(wiki);
+ let matches = nested_find_1(wiki);
matches.forEach(function (s) {
if (isFile.test(s) === true) {
paragraph.images = paragraph.images || [];
- var img = oneImage(s, doc);
+ let img = oneImage(s, doc);
if (img) {
paragraph.images.push(img);
@@ -4484,80 +4166,84 @@
var image = parseImages;
- var defaults$b = {};
+ const defaults$3 = {};
- var toText = function toText(list, options) {
- return list.map(function (s) {
- var str = s.text(options);
+ const toText$2 = (list, options) => {
+ return list.map(s => {
+ let str = s.text(options);
return ' * ' + str;
}).join('\n');
};
- var List = function List(data) {
+ const List = function (data, wiki = '') {
Object.defineProperty(this, 'data', {
enumerable: false,
value: data
});
+ Object.defineProperty(this, 'wiki', {
+ enumerable: false,
+ value: wiki
+ });
};
- var methods$8 = {
- lines: function lines() {
+ const methods$2 = {
+ lines() {
return this.data;
},
- links: function links(n) {
- var links = [];
- this.lines().forEach(function (s) {
+
+ links(clue) {
+ let links = [];
+ this.lines().forEach(s => {
links = links.concat(s.links());
});
- if (typeof n === 'number') {
- return links[n];
- } else if (typeof n === 'string') {
+ if (typeof clue === 'string') {
//grab a link like .links('Fortnight')
- n = n.charAt(0).toUpperCase() + n.substring(1); //titlecase it
+ clue = clue.charAt(0).toUpperCase() + clue.substring(1); //titlecase it
- var link = links.find(function (o) {
- return o.page() === n;
- });
+ let link = links.find(o => o.page() === clue);
return link === undefined ? [] : [link];
}
return links;
},
- json: function json(options) {
- options = setDefaults_1(options, defaults$b);
- return this.lines().map(function (s) {
- return s.json(options);
- });
+
+ json(options) {
+ options = setDefaults_1(options, defaults$3);
+ return this.lines().map(s => s.json(options));
},
- text: function text() {
- return toText(this.data);
+
+ text() {
+ return toText$2(this.data);
+ },
+
+ wikitext() {
+ return this.wiki || '';
}
+
};
- Object.keys(methods$8).forEach(function (k) {
- List.prototype[k] = methods$8[k];
+ Object.keys(methods$2).forEach(k => {
+ List.prototype[k] = methods$2[k];
});
var List_1 = List;
- var parseSentence$5 = _04Sentence.fromText;
- var list_reg = /^[#\*:;\|]+/;
- var bullet_reg = /^\*+[^:,\|]{4}/;
- var number_reg = /^ ?\#[^:,\|]{4}/;
- var has_word = /[a-z_0-9\]\}]/i; // does it start with a bullet point or something?
+ const parseSentence$2 = _04Sentence.fromText;
+ const list_reg = /^[#\*:;\|]+/;
+ const bullet_reg = /^\*+[^:,\|]{4}/;
+ const number_reg = /^ ?\#[^:,\|]{4}/;
+ const has_word = /[a-z_0-9\]\}]/i; // does it start with a bullet point or something?
- var isList = function isList(line) {
+ const isList = function (line) {
return list_reg.test(line) || bullet_reg.test(line) || number_reg.test(line);
}; //make bullets/numbers into human-readable *'s
- var cleanList = function cleanList(list) {
- var number = 1;
- list = list.filter(function (l) {
- return l;
- });
+ const cleanList = function (list) {
+ let number = 1;
+ list = list.filter(l => l);
- for (var i = 0; i < list.length; i++) {
- var line = list[i]; //add # numberings formatting
+ for (let i = 0; i < list.length; i++) {
+ let line = list[i]; //add # numberings formatting
if (line.match(number_reg)) {
line = line.replace(/^ ?#*/, number + ') ');
@@ -4568,16 +4254,16 @@
line = line.replace(list_reg, '');
}
- list[i] = parseSentence$5(line);
+ list[i] = parseSentence$2(line);
}
return list;
};
- var grabList = function grabList(lines, i) {
- var sub = [];
+ const grabList = function (lines, i) {
+ let sub = [];
- for (var o = i; o < lines.length; o++) {
+ for (let o = i; o < lines.length; o++) {
if (isList(lines[o])) {
sub.push(lines[o]);
} else {
@@ -4585,23 +4271,20 @@
}
}
- sub = sub.filter(function (a) {
- return a && has_word.test(a);
- });
+ sub = sub.filter(a => a && has_word.test(a));
sub = cleanList(sub);
return sub;
};
- var parseList = function parseList(paragraph) {
- var wiki = paragraph.wiki;
- var lines = wiki.split(/\n/g); // lines = lines.filter(l => has_word.test(l));
+ const parseList = function (paragraph) {
+ let wiki = paragraph.wiki;
+ let lines = wiki.split(/\n/g);
+ let lists = [];
+ let theRest = [];
- var lists = [];
- var theRest = [];
-
- for (var i = 0; i < lines.length; i++) {
+ for (let i = 0; i < lines.length; i++) {
if (isList(lines[i])) {
- var sub = grabList(lines, i);
+ let sub = grabList(lines, i);
if (sub.length > 0) {
lists.push(sub);
@@ -4612,178 +4295,55 @@
}
}
- paragraph.lists = lists.map(function (l) {
- return new List_1(l);
- });
+ paragraph.lists = lists.map(l => new List_1(l, wiki));
paragraph.wiki = theRest.join('\n');
};
- var list = parseList;
+ var list$1 = parseList;
- var parseSentences = _04Sentence.byParagraph;
- var twoNewLines = /\r?\n\r?\n/;
- var parse$5 = {
+ const parseSentences = _04Sentence.byParagraph;
+ const twoNewLines = /\r?\n\r?\n/;
+ const parse$4 = {
image: image,
- list: list
+ list: list$1
};
- var parseParagraphs = function parseParagraphs(section, doc) {
- var wiki = section.wiki;
- var paragraphs = wiki.split(twoNewLines); //don't create empty paragraphs
+ const parseParagraphs = function (section, doc) {
+ let wiki = section._wiki;
+ let paragraphs = wiki.split(twoNewLines); //don't create empty paragraphs
- paragraphs = paragraphs.filter(function (p) {
- return p && p.trim().length > 0;
- });
- paragraphs = paragraphs.map(function (str) {
- var paragraph = {
+ paragraphs = paragraphs.filter(p => p && p.trim().length > 0);
+ paragraphs = paragraphs.map(str => {
+ let paragraph = {
wiki: str,
lists: [],
sentences: [],
images: []
}; //parse the lists
- parse$5.list(paragraph); // parse images
+ parse$4.list(paragraph); //parse images
- parse$5.image(paragraph, doc); //parse the sentences
+ parse$4.image(paragraph, doc); //parse the sentences
parseSentences(paragraph);
return new Paragraph_1(paragraph);
});
- section.wiki = wiki;
- section.paragraphs = paragraphs;
+ section._wiki = wiki;
+ section._paragraphs = paragraphs;
};
var _03Paragraph = parseParagraphs;
- var toJson$6 = function toJson(infobox, options) {
- var json = Object.keys(infobox.data).reduce(function (h, k) {
- if (infobox.data[k]) {
- h[k] = infobox.data[k].json();
- }
-
- return h;
- }, {}); //support mongo-encoding keys
-
- if (options.encode === true) {
- json = encode.encodeObj(json);
- }
-
- return json;
- };
-
- var toJson_1$3 = toJson$6;
-
- var normalize = function normalize(str) {
- str = str.toLowerCase();
- str = str.replace(/[-_]/g, ' ');
- return str.trim();
- }; //a formal key-value data table about a topic
-
-
- var Infobox = function Infobox(obj) {
- this._type = obj.type;
- this.domain = obj.domain;
- Object.defineProperty(this, 'data', {
- enumerable: false,
- value: obj.data
- });
- };
-
- var methods$9 = {
- type: function type() {
- return this._type;
- },
- links: function links(n) {
- var _this = this;
-
- var arr = [];
- Object.keys(this.data).forEach(function (k) {
- _this.data[k].links().forEach(function (l) {
- return arr.push(l);
- });
- });
-
- if (typeof n === 'number') {
- return arr[n];
- } else if (typeof n === 'string') {
- //grab a link like .links('Fortnight')
- n = n.charAt(0).toUpperCase() + n.substring(1); //titlecase it
-
- var link = arr.find(function (o) {
- return o.page() === n;
- });
- return link === undefined ? [] : [link];
- }
-
- return arr;
- },
- image: function image() {
- var s = this.get('image') || this.get('image2') || this.get('logo');
-
- if (!s) {
- return null;
- }
-
- var obj = s.json();
- obj.file = obj.text;
- obj.text = '';
- obj.domain = this.domain; // add domain information for image
-
- return new Image_1(obj);
- },
- get: function get() {
- var key = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : '';
- key = normalize(key);
- var keys = Object.keys(this.data);
-
- for (var i = 0; i < keys.length; i += 1) {
- var tmp = normalize(keys[i]);
-
- if (key === tmp) {
- return this.data[keys[i]];
- }
- }
-
- return null;
- },
- text: function text() {
- return '';
- },
- json: function json(options) {
- options = options || {};
- return toJson_1$3(this, options);
- },
- keyValue: function keyValue() {
- var _this2 = this;
-
- return Object.keys(this.data).reduce(function (h, k) {
- if (_this2.data[k]) {
- h[k] = _this2.data[k].text();
- }
-
- return h;
- }, {});
- }
- }; //aliases
-
- Object.keys(methods$9).forEach(function (k) {
- Infobox.prototype[k] = methods$9[k];
- });
- Infobox.prototype.data = Infobox.prototype.keyValue;
- Infobox.prototype.template = Infobox.prototype.type;
- Infobox.prototype.images = Infobox.prototype.image;
- var Infobox_1 = Infobox;
+ const open = '{';
+ const close = '}'; //grab all first-level recursions of '{{...}}'
- var open = '{';
- var close = '}'; //grab all first-level recursions of '{{...}}'
+ const findFlat = function (wiki) {
+ let depth = 0;
+ let list = [];
+ let carry = [];
- var findFlat = function findFlat(wiki) {
- var depth = 0;
- var list = [];
- var carry = [];
-
- for (var i = wiki.indexOf(open); i !== -1 && i < wiki.length; depth > 0 ? i++ : i = wiki.indexOf(open, i + 1)) {
- var c = wiki[i]; //open it
+ for (let i = wiki.indexOf(open); i !== -1 && i < wiki.length; depth > 0 ? i++ : i = wiki.indexOf(open, i + 1)) {
+ let c = wiki[i]; //open it
if (c === open) {
depth += 1;
@@ -4796,7 +4356,7 @@
if (depth === 0) {
carry.push(c);
- var tmpl = carry.join('');
+ let tmpl = carry.join('');
carry = []; //last check
if (/\{\{/.test(tmpl) && /\}\}/.test(tmpl)) {
@@ -4821,12 +4381,12 @@
return list;
};
- var flat = findFlat;
+ var _02Flat = findFlat;
//templates are usually '{{name|stuff}}'
- var getName = function getName(tmpl) {
- var name = null; //{{name|foo}}
+ const getName = function (tmpl) {
+ let name = null; //{{name|foo}}
if (/^\{\{[^\n]+\|/.test(tmpl)) {
name = (tmpl.match(/^\{\{(.+?)\|/) || [])[1];
@@ -4848,9 +4408,9 @@
var _getName = getName;
- var hasTemplate = /\{\{/;
+ const hasTemplate = /\{\{/;
- var parseTemplate = function parseTemplate(tmpl) {
+ const parseTemplate$1 = function (tmpl) {
// this is some unexplained Lua thing
tmpl = tmpl.replace(/#invoke:/, '');
return {
@@ -4860,21 +4420,21 @@
};
};
- var doEach = function doEach(obj) {
+ const doEach = function (obj) {
// peel-off top-level
- var wiki = obj.body.substr(2);
+ let wiki = obj.body.substr(2);
wiki = wiki.replace(/\}\}$/, ''); // get our child templates
- obj.children = flat(wiki);
- obj.children = obj.children.map(parseTemplate);
+ obj.children = _02Flat(wiki);
+ obj.children = obj.children.map(parseTemplate$1);
if (obj.children.length === 0) {
return obj;
} // recurse through children
- obj.children.forEach(function (ch) {
- var inside = ch.body.substr(2);
+ obj.children.forEach(ch => {
+ let inside = ch.body.substr(2);
if (hasTemplate.test(inside)) {
return doEach(ch); //keep going
@@ -4886,17 +4446,17 @@
}; // return a nested structure of all templates
- var findTemplates = function findTemplates(wiki) {
- var list = flat(wiki);
- list = list.map(parseTemplate);
+ const findTemplates = function (wiki) {
+ let list = _02Flat(wiki);
+ list = list.map(parseTemplate$1);
list = list.map(doEach);
return list;
};
- var find = findTemplates;
+ var _01Nested = findTemplates;
//we explicitly ignore these, because they sometimes have resolve some data
- var list$1 = [//https://en.wikipedia.org/wiki/category:templates_with_no_visible_output
+ const list = [//https://en.wikipedia.org/wiki/category:templates_with_no_visible_output
'anchor', 'defaultsort', 'use list-defined references', 'void', //https://en.wikipedia.org/wiki/Category:Protection_templates
'pp', 'pp-move-indef', 'pp-semi-indef', 'pp-vandalism', //https://en.wikipedia.org/wiki/Template:R
'r', //out-of-scope still - https://en.wikipedia.org/wiki/Template:Tag
@@ -4920,21 +4480,13 @@
// 'weasel inline',
//https://en.wikipedia.org/wiki/Template:End
'pope list end', 'shipwreck list end', 'starbox end', 'end box', 'end', 's-end'];
- var ignore$1 = list$1.reduce(function (h, str) {
+ const ignore = list.reduce((h, str) => {
h[str] = true;
return h;
}, {});
- var _ignore = ignore$1;
-
- var i18nReg = new RegExp('^(subst.)?(' + i18n$1.infoboxes.join('|') + ')[: \n]', 'i'); //some looser ones
+ var _ignore = ignore;
- var startReg = /^infobox /i;
- var endReg = / infobox$/i;
- var yearIn = /$Year in [A-Z]/i; //some known ones from
- // https://en.wikipedia.org/wiki/Wikipedia:List_of_infoboxes
- // and https://en.wikipedia.org/wiki/Category:Infobox_templates
-
- var known = {
+ var _infoboxes = {
'gnf protein box': true,
'automatic taxobox': true,
'chembox ': true,
@@ -4970,11 +4522,19 @@
'hockey team player': true,
'hockey team start': true,
mlbbioret: true
- }; //
+ };
+
+ const i18nReg = new RegExp('^(subst.)?(' + i18n.infoboxes.join('|') + ')[: \n]', 'i'); //some looser ones
- var isInfobox = function isInfobox(name) {
- // known
- if (known.hasOwnProperty(name) === true) {
+ const startReg = /^infobox /i;
+ const endReg = / infobox$/i;
+ const yearIn = /$Year in [A-Z]/i; //some known ones from
+ //https://en.wikipedia.org/wiki/Wikipedia:List_of_infoboxes
+ //and https://en.wikipedia.org/wiki/Category:Infobox_templates
+
+ const isInfobox = function (name) {
+ //known
+ if (_infoboxes.hasOwnProperty(name) === true) {
return true;
}
@@ -4992,25 +4552,24 @@
}
return false;
- }; //turns template data into good inforbox data
+ }; //turns template data into good infobox data
- var fmtInfobox = function fmtInfobox() {
- var obj = arguments.length > 0 && arguments[0] !== undefined ? arguments[0] : {};
- var m = obj.template.match(i18nReg);
- var type = obj.template;
+ const fmtInfobox = function (obj = {}) {
+ let m = obj.template.match(i18nReg);
+ let type = obj.template;
if (m && m[0]) {
type = type.replace(m[0], '');
}
type = type.trim();
- var infobox = {
+ let infobox = {
template: 'infobox',
type: type,
data: obj
};
- delete infobox.data.template; // already have this.
+ delete infobox.data.template; //already have this.
delete infobox.data.list; //just in case!
@@ -5022,1050 +4581,1456 @@
format: fmtInfobox
};
- var _months = [undefined, //1-based months.. :/
- 'January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'];
+ let aliases = {
+ imdb: 'imdb name',
+ 'imdb episodess': 'imdb episode',
+ localday: 'currentday',
+ localdayname: 'currentdayname',
+ localyear: 'currentyear',
+ 'birth date based on age at death': 'birth based on age as of date',
+ 'bare anchored list': 'anchored list',
+ cvt: 'convert',
+ cricon: 'flagicon',
+ sfrac: 'frac',
+ sqrt: 'radic',
+ 'unreferenced section': 'unreferenced',
+ redir: 'redirect',
+ sisterlinks: 'sister project links',
+ 'main article': 'main'
+ }; //multiple aliases
+
+ let multi = {
+ date: ['byline', 'dateline'],
+ //wikinews
+ citation: ['cite', 'source', 'source-pr', 'source-science'],
+ flagcountry: ['cr', 'cr-rt'],
+ trunc: ['str left', 'str crop'],
+ percentage: ['pct', 'percentage'],
+ rnd: ['rndfrac', 'rndnear'],
+ abbr: ['tooltip', 'abbrv', 'define'],
+ sfn: ['sfnref', 'harvid', 'harvnb'],
+ 'birth date and age': ['death date and age', 'bda'],
+ currentmonth: ['localmonth', 'currentmonthname', 'currentmonthabbrev'],
+ currency: ['monnaie', 'unité', 'nombre', 'nb', 'iso4217'],
+ coord: ['coor', 'coor title dms', 'coor title dec', 'coor dms', 'coor dm', 'coor dec'],
+ 'columns-list': ['cmn', 'col-list', 'columnslist', 'collist'],
+ nihongo: ['nihongo2', 'nihongo3', 'nihongo-s', 'nihongo foot'],
+ plainlist: ['flatlist', 'ublist', 'plain list'],
+ 'winning percentage': ['winpct', 'winperc'],
+ 'collapsible list': ['unbulleted list', 'ubl'],
+ 'election box begin': ['election box begin no change', 'election box begin no party', 'election box begin no party no change', 'election box inline begin', 'election box inline begin no change'],
+ 'election box candidate': ['election box candidate for alliance', 'election box candidate minor party', 'election box candidate no party link no change', 'election box candidate with party link', 'election box candidate with party link coalition 1918', 'election box candidate with party link no change', 'election box inline candidate', 'election box inline candidate no change', 'election box inline candidate with party link', 'election box inline candidate with party link no change', 'election box inline incumbent'],
+ '4teambracket': ['2teambracket', '4team2elimbracket', '8teambracket', '16teambracket', '32teambracket', '4roundbracket-byes', 'cwsbracket', 'nhlbracket', 'nhlbracket-reseed', '4teambracket-nhl', '4teambracket-ncaa', '4teambracket-mma', '4teambracket-mlb', '16teambracket-two-reseeds', '8teambracket-nhl', '8teambracket-mlb', '8teambracket-ncaa', '8teambracket-afc', '8teambracket-afl', '8teambracket-tennis3', '8teambracket-tennis5', '16teambracket-nhl', '16teambracket-nhl divisional', '16teambracket-nhl-reseed', '16teambracket-nba', '16teambracket-swtc', '16teambracket-afc', '16teambracket-tennis3', '16teambracket-tennis5'],
+ start: ['end', 'birth', 'death', 'start date', 'end date', 'birth date', 'death date', 'start date and age', 'end date and age', 'dob'],
+ 'start-date': ['end-date', 'birth-date', 'death-date', 'birth-date and age', 'birth-date and given age', 'death-date and age', 'death-date and given age'],
+ tl: ['lts', 't', 'tfd links', 'tiw', 'tltt', 'tetl', 'tsetl', 'ti', 'tic', 'tiw', 'tlt', 'ttl', 'twlh', 'tl2', 'tlu', 'demo', 'hatnote', 'xpd', 'para', 'elc', 'xtag', 'mli', 'mlix', '#invoke', 'url' //https://en.wikipedia.org/wiki/Template:URL
+ ]
+ }; // - other languages -
+ // Polish, {{IPAc-pl}} {{IPAc-pl|'|sz|cz|e|ć|i|n}} → [ˈʂt͡ʂɛt͡ɕin]
+ // Portuguese, {{IPAc-pt}} {{IPAc-pt|p|o|<|r|t|u|'|g|a|l|lang=pt}} and {{IPAc-pt|b|r|a|'|s|i|l|lang=br}} → [puɾtuˈɣaɫ] and [bɾaˈsiw]
- var monthName = _months.reduce(function (h, str, i) {
- if (i === 0) {
- return h;
- }
+ Object.keys(languages).forEach(lang => {
+ aliases['ipa-' + lang] = 'ipa';
+ aliases['ipac-' + lang] = 'ipac';
+ }); // add each alias in
- h[str.toLowerCase()] = i;
- return h;
- }, {}); //parse year|month|date numbers
+ Object.keys(multi).forEach(k => {
+ multi[k].forEach(str => {
+ aliases[str] = k;
+ });
+ });
+ var aliases_1 = aliases;
+
+ var hardcoded = {
+ '·': '·',
+ dot: '·',
+ middot: '·',
+ '•': ' • ',
+ ',': ',',
+ '1/2': '1⁄2',
+ '1/3': '1⁄3',
+ '2/3': '2⁄3',
+ '1/4': '1⁄4',
+ '3/4': '3⁄4',
+ '–': '–',
+ ndash: '–',
+ 'en dash': '–',
+ 'spaced ndash': ' – ',
+ '—': '—',
+ mdash: '—',
+ 'em dash': '—',
+ 'number sign': '#',
+ ibeam: 'I',
+ '&': '&',
+ ';': ';',
+ ampersand: '&',
+ snds: ' – ',
+ snd: ' – ',
+ '^': ' ',
+ '!': '|',
+ '\\': ' /',
+ '`': '`',
+ '=': '=',
+ bracket: '[',
+ '[': '[',
+ '*': '*',
+ asterisk: '*',
+ 'long dash': '———',
+ clear: '\n\n',
+ 'h.': 'ḥ',
+ profit: '▲',
+ loss: '▼',
+ gain: '▲'
+ };
+
+ let templates$9 = {
+ p1: 0,
+ p2: 1,
+ p3: 2,
+ resize: 1,
+ //https://en.wikipedia.org/wiki/Template:Resize
+ lang: 1,
+ 'rtl-lang': 1,
+ l: 2,
+ h: 1,
+ //https://en.wikipedia.org/wiki/Template:Hover_title
+ sort: 1 //https://en.wikipedia.org/wiki/Template:Sort
- var ymd = function ymd(arr) {
- var obj = {};
- var units = ['year', 'month', 'date', 'hour', 'minute', 'second']; //parse each unit in sequence..
+ }; //templates that we simply grab their insides as plaintext
- for (var i = 0; i < units.length; i += 1) {
- //skip it
- if (!arr[i] && arr[1] !== 0) {
- continue;
- }
+ let zeros = ['defn', 'lino', //https://en.wikipedia.org/wiki/Template:Linum
+ 'finedetail', //https://en.wikipedia.org/wiki/Template:Finedetail
+ 'nobold', 'noitalic', 'nocaps', 'vanchor', //https://en.wikipedia.org/wiki/Template:Visible_anchor
+ 'rnd', 'date', //Explictly-set dates - https://en.wikipedia.org/wiki/Template:Date
+ 'taste', 'monthname', 'baseball secondary style', 'lang-de', 'nowrap', 'nobr', 'big', 'cquote', 'pull quote', 'small', 'smaller', 'midsize', 'larger', 'big', 'kbd', 'bigger', 'large', 'mono', 'strongbad', 'stronggood', 'huge', 'xt', 'xt2', '!xt', 'xtn', 'xtd', 'dc', 'dcr', 'mxt', '!mxt', 'mxtn', 'mxtd', 'bxt', '!bxt', 'bxtn', 'bxtd', 'delink', //https://en.wikipedia.org/wiki/Template:Delink
+ 'pre', 'var', 'mvar', 'pre2', 'code'];
+ zeros.forEach(k => {
+ templates$9[k] = 0;
+ }); //https://en.wikipedia.org/wiki/Category:Lang-x_templates
- var num = parseInt(arr[i], 10);
+ Object.keys(languages).forEach(k => {
+ templates$9['lang-' + k] = 0;
+ });
+ var shorthand$1 = templates$9;
- if (isNaN(num) === false) {
- obj[units[i]] = num; //we good.
- } else if (units[i] === 'month' && monthName.hasOwnProperty(arr[i])) {
- //try for month-name, like 'january
- var month = monthName[arr[i]];
- obj[units[i]] = month;
- } else {
- //we dead. so skip this unit
- delete obj[units[i]];
- }
- } //try for timezone,too ftw
+ const percentage = function (obj) {
+ if (!obj.numerator && !obj.denominator) {
+ return null;
+ }
+ let perc = Number(obj.numerator) / Number(obj.denominator);
+ perc *= 100;
+ let dec = Number(obj.decimals);
- var last = arr[arr.length - 1] || '';
- last = String(last);
+ if (isNaN(dec)) {
+ dec = 1;
+ }
- if (last.toLowerCase() === 'z') {
- obj.tz = 'UTC';
- } else if (/[+-][0-9]+:[0-9]/.test(last)) {
- obj.tz = arr[6];
- }
+ perc = perc.toFixed(dec);
+ return Number(perc);
+ };
- return obj;
- }; //zero-pad a number
+ const toNumber = function (str = '') {
+ if (typeof str === 'number') {
+ return str;
+ }
+ str = str.replace(/,/g, '');
+ str = str.replace(/−/g, '-');
+ let num = Number(str);
- var pad = function pad(num) {
- if (num < 10) {
- return '0' + num;
+ if (isNaN(num)) {
+ return str;
}
- return String(num);
+ return num;
};
- var toText$1 = function toText(date) {
- //eg '1995'
- var str = String(date.year || '');
-
- if (date.month !== undefined && _months.hasOwnProperty(date.month) === true) {
- if (date.date === undefined) {
- //January 1995
- str = "".concat(_months[date.month], " ").concat(date.year);
- } else {
- //January 5, 1995
- str = "".concat(_months[date.month], " ").concat(date.date, ", ").concat(date.year); //add times, if available
-
- if (date.hour !== undefined && date.minute !== undefined) {
- var time = "".concat(pad(date.hour), ":").concat(pad(date.minute));
-
- if (date.second !== undefined) {
- time = time + ':' + pad(date.second);
- }
-
- str = time + ', ' + str; //add timezone, if there, at the end in brackets
- }
+ const getLang = function (name) {
+ //grab the language from the template name - 'ipa-de'
+ let lang = name.match(/ipac?-(.+)/);
- if (date.tz) {
- str += " (".concat(date.tz, ")");
- }
+ if (lang !== null) {
+ if (languages.hasOwnProperty(lang[1]) === true) {
+ return languages[lang[1]].english_title;
}
+
+ return lang[1];
}
- return str;
+ return null;
};
- var _format = {
- toText: toText$1,
- ymd: ymd
- }; // console.log(toText(ymd([2018, 3, 28])));
+ const titlecase$1 = str => {
+ return str.charAt(0).toUpperCase() + str.substring(1);
+ };
+
+ const sisterProjects = {
+ wikt: 'wiktionary',
+ commons: 'commons',
+ c: 'commons',
+ commonscat: 'commonscat',
+ n: 'wikinews',
+ q: 'wikiquote',
+ s: 'wikisource',
+ a: 'wikiauthor',
+ b: 'wikibooks',
+ voy: 'wikivoyage',
+ v: 'wikiversity',
+ d: 'wikidata',
+ species: 'wikispecies',
+ m: 'meta',
+ mw: 'mediawiki'
+ };
+ var _lib$3 = {
+ titlecase: titlecase$1,
+ sisterProjects: sisterProjects,
+ getLang: getLang,
+ percentage: percentage,
+ toNumber: toNumber
+ };
- var misc = {
- reign: function reign(tmpl) {
- var order = ['start', 'end'];
- var obj = parse$3(tmpl, order);
- return "(r. ".concat(obj.start, " \u2013 ").concat(obj.end, ")");
+ var functions$2 = {
+ //https://en.wikipedia.org/wiki/Template:Ra
+ ra: tmpl => {
+ let obj = toJSON(tmpl, ['hours', 'minutes', 'seconds']);
+ return [obj.hours || 0, obj.minutes || 0, obj.seconds || 0].join(':');
},
- circa: function circa(tmpl) {
- var obj = parse$3(tmpl, ['year']);
- return "c.\u2009".concat(obj.year);
+ //https://en.wikipedia.org/wiki/Template:Deg2HMS
+ deg2hms: tmpl => {
+ //this template should do the conversion
+ let obj = toJSON(tmpl, ['degrees']);
+ return (obj.degrees || '') + '°';
},
- //we can't do timezones, so fake this one a little bit
- //https://en.wikipedia.org/wiki/Template:Time
- time: function time() {
- var d = new Date();
- var obj = _format.ymd([d.getFullYear(), d.getMonth(), d.getDate()]);
- return _format.toText(obj);
+ hms2deg: tmpl => {
+ //this template should do the conversion too
+ let obj = toJSON(tmpl, ['hours', 'minutes', 'seconds']);
+ return [obj.hours || 0, obj.minutes || 0, obj.seconds || 0].join(':');
},
- monthname: 0,
- //https://en.wikipedia.org/wiki/Template:OldStyleDate
- oldstyledate: function oldstyledate(tmpl) {
- var order = ['date', 'year'];
- var obj = parse$3(tmpl, order);
- var str = obj.date;
+ decdeg: tmpl => {
+ //this template should do the conversion too
+ let obj = toJSON(tmpl, ['deg', 'min', 'sec', 'hem', 'rnd']);
+ return (obj.deg || obj.degrees) + '°';
+ },
+ //https://en.wikipedia.org/wiki/Template:Sortname
+ sortname: tmpl => {
+ let order = ['first', 'last', 'target', 'sort'];
+ let obj = toJSON(tmpl, order);
+ let name = `${obj.first || ''} ${obj.last || ''}`;
+ name = name.trim();
- if (obj.year) {
- str += ' ' + obj.year;
+ if (obj.nolink) {
+ return obj.target || name;
}
- return str;
- }
- };
- var misc_1 = misc;
+ if (obj.dab) {
+ name += ` (${obj.dab})`;
- //this is allowed to be rough
- var day = 1000 * 60 * 60 * 24;
- var month = day * 30;
- var year = day * 365;
+ if (obj.target) {
+ obj.target += ` (${obj.dab})`;
+ }
+ }
- var getEpoch = function getEpoch(obj) {
- return new Date("".concat(obj.year, "-").concat(obj.month || 0, "-").concat(obj.date || 1)).getTime();
- }; //very rough!
+ if (obj.target) {
+ return `[[${obj.target}|${name}]]`;
+ }
+ return `[[${name}]]`;
+ },
+ // https://en.wikipedia.org/wiki/Template:First_word
+ 'first word': tmpl => {
+ let obj = toJSON(tmpl, ['text']);
+ let str = obj.text;
- var delta = function delta(from, to) {
- from = getEpoch(from);
- to = getEpoch(to);
- var diff = to - from;
- var obj = {}; //get years
+ if (obj.sep) {
+ return str.split(obj.sep)[0];
+ }
- var years = Math.floor(diff / year, 10);
+ return str.split(' ')[0];
+ },
+ trunc: tmpl => {
+ let order = ['str', 'len'];
+ let obj = toJSON(tmpl, order);
+ return obj.str.substr(0, obj.len);
+ },
+ 'str mid': tmpl => {
+ let order = ['str', 'start', 'end'];
+ let obj = toJSON(tmpl, order);
+ let start = parseInt(obj.start, 10) - 1;
+ let end = parseInt(obj.end, 10);
+ return obj.str.substr(start, end);
+ },
+ reign: tmpl => {
+ let order = ['start', 'end'];
+ let obj = toJSON(tmpl, order);
+ return `(r. ${obj.start} – ${obj.end})`;
+ },
+ circa: tmpl => {
+ let obj = toJSON(tmpl, ['year']);
+ return `c. ${obj.year}`;
+ },
+ // https://en.wikipedia.org/wiki/Template:Decade_link
+ 'decade link': tmpl => {
+ let obj = toJSON(tmpl, ['year']);
+ return `${obj.year}|${obj.year}s`;
+ },
+ // https://en.wikipedia.org/wiki/Template:Decade
+ decade: tmpl => {
+ let obj = toJSON(tmpl, ['year']);
+ let year = Number(obj.year);
+ year = parseInt(year / 10, 10) * 10; // round to decade
- if (years > 0) {
- obj.years = years;
- diff -= obj.years * year;
- } //get months
+ return `${year}s`;
+ },
+ // https://en.wikipedia.org/wiki/Template:Century
+ century: tmpl => {
+ let obj = toJSON(tmpl, ['year']);
+ let year = Number(obj.year);
+ year = parseInt(year / 100, 10) + 1;
+ return `${year}`;
+ },
+ //https://en.wikipedia.org/wiki/Template:Radic
+ radic: tmpl => {
+ let order = ['after', 'before'];
+ let obj = toJSON(tmpl, order);
+ return `${obj.before || ''}√${obj.after || ''}`;
+ },
+ 'medical cases chart/row': tmpl => {
+ // Deprecated template; we keep it.
+ return tmpl;
+ },
+ //https://en.wikipedia.org/wiki/Template:OldStyleDate
+ oldstyledate: tmpl => {
+ let order = ['date', 'year'];
+ let obj = toJSON(tmpl, order);
+ return obj.year ? obj.date + ' ' + obj.year : obj.date;
+ },
+ //formatting things - https://en.wikipedia.org/wiki/Template:Nobold
+ braces: tmpl => {
+ let obj = toJSON(tmpl, ['text']);
+ let attrs = '';
+ if (obj.list) {
+ attrs = '|' + obj.list.join('|');
+ }
- var months = Math.floor(diff / month, 10);
+ return '{{' + (obj.text || '') + attrs + '}}';
+ },
+ hlist: tmpl => {
+ let obj = toJSON(tmpl);
+ obj.list = obj.list || [];
+ return obj.list.join(' · ');
+ },
+ pagelist: tmpl => {
+ let arr = toJSON(tmpl).list || [];
+ return arr.join(', ');
+ },
+ //actually rendering these links removes the text.
+ //https://en.wikipedia.org/wiki/Template:Catlist
+ catlist: tmpl => {
+ let arr = toJSON(tmpl).list || [];
+ return arr.join(', ');
+ },
+ //https://en.wikipedia.org/wiki/Template:Br_separated_entries
+ 'br separated entries': tmpl => {
+ let arr = toJSON(tmpl).list || [];
+ return arr.join('\n\n');
+ },
+ 'comma separated entries': tmpl => {
+ let arr = toJSON(tmpl).list || [];
+ return arr.join(', ');
+ },
+ //https://en.wikipedia.org/wiki/Template:Bare_anchored_list
+ 'anchored list': tmpl => {
+ let arr = toJSON(tmpl).list || [];
+ arr = arr.map((str, i) => `${i + 1}. ${str}`);
+ return arr.join('\n\n');
+ },
+ 'bulleted list': tmpl => {
+ let arr = toJSON(tmpl).list || [];
+ arr = arr.filter(f => f);
+ arr = arr.map(str => '• ' + str);
+ return arr.join('\n\n');
+ },
+ //a strange, newline-based list - https://en.wikipedia.org/wiki/Template:Plainlist
+ plainlist: tmpl => {
+ tmpl = _strip(tmpl);
+ let arr = tmpl.split('|').slice(1); //remove the title
- if (months > 0) {
- obj.months = months;
- diff -= obj.months * month;
- } //get days
+ arr = arr.join('|').split(/\n ?\* ?/); //split on newline
+ arr = arr.filter(s => s);
+ return arr.join('\n\n');
+ },
+ //https://en.wikipedia.org/wiki/Template:Term
+ term: tmpl => {
+ let obj = toJSON(tmpl, ['term']);
+ return `${obj.term}:`;
+ },
+ linum: tmpl => {
+ let obj = toJSON(tmpl, ['num', 'text']);
+ return `${obj.num}. ${obj.text}`;
+ },
+ 'block indent': tmpl => {
+ let obj = toJSON(tmpl);
- var days = Math.floor(diff / day, 10);
+ if (obj['1']) {
+ return '\n' + obj['1'] + '\n';
+ }
- if (days > 0) {
- obj.days = days; // diff -= (obj.days * day);
- }
+ return '';
+ },
+ //https://en.wikipedia.org/wiki/Template:Lbs
+ lbs: tmpl => {
+ let obj = toJSON(tmpl, ['text']);
+ return `[[${obj.text} Lifeboat Station|${obj.text}]]`;
+ },
+ //Foo-class
+ lbc: tmpl => {
+ let obj = toJSON(tmpl, ['text']);
+ return `[[${obj.text}-class lifeboat|${obj.text}-class]]`;
+ },
+ lbb: tmpl => {
+ let obj = toJSON(tmpl, ['text']);
+ return `[[${obj.text}-class lifeboat|${obj.text}]]`;
+ },
+ //https://www.mediawiki.org/wiki/Help:Magic_words#Formatting
+ '#dateformat': tmpl => {
+ tmpl = tmpl.replace(/:/, '|');
+ let obj = toJSON(tmpl, ['date', 'format']);
+ return obj.date;
+ },
+ //https://www.mediawiki.org/wiki/Help:Magic_words#Formatting
+ lc: tmpl => {
+ tmpl = tmpl.replace(/:/, '|');
+ let obj = toJSON(tmpl, ['text']);
+ return (obj.text || '').toLowerCase();
+ },
+ //https://www.mediawiki.org/wiki/Help:Magic_words#Formatting
+ uc: tmpl => {
+ tmpl = tmpl.replace(/:/, '|');
+ let obj = toJSON(tmpl, ['text']);
+ return (obj.text || '').toUpperCase();
+ },
+ lcfirst: tmpl => {
+ tmpl = tmpl.replace(/:/, '|');
+ let text = toJSON(tmpl, ['text']).text;
- return obj;
- };
+ if (!text) {
+ return '';
+ }
- var _delta = delta;
+ return text[0].toLowerCase() + text.substr(1);
+ },
+ ucfirst: tmpl => {
+ tmpl = tmpl.replace(/:/, '|');
+ let text = toJSON(tmpl, ['text']).text;
- var ymd$1 = _format.ymd;
- var toText$2 = _format.toText; //wrap it up as a template
+ if (!text) {
+ return '';
+ }
- var template = function template(date) {
- return {
- template: 'date',
- data: date
- };
- };
+ return text[0].toUpperCase() + text.substr(1);
+ },
+ padleft: tmpl => {
+ tmpl = tmpl.replace(/:/, '|');
+ let obj = toJSON(tmpl, ['text', 'num']);
+ let text = obj.text || '';
+ return text.padStart(obj.num, obj.str || '0');
+ },
+ padright: tmpl => {
+ tmpl = tmpl.replace(/:/, '|');
+ let obj = toJSON(tmpl, ['text', 'num']);
+ let text = obj.text || '';
+ return text.padEnd(obj.num, obj.str || '0');
+ },
+ //https://en.wikipedia.org/wiki/Template:Abbrlink
+ abbrlink: tmpl => {
+ let obj = toJSON(tmpl, ['abbr', 'page']);
- var getBoth = function getBoth(tmpl) {
- tmpl = _strip(tmpl);
- var arr = tmpl.split('|');
- var from = ymd$1(arr.slice(1, 4));
- var to = arr.slice(4, 7); //assume now, if 'to' is empty
+ if (obj.page) {
+ return `[[${obj.page}|${obj.abbr}]]`;
+ }
- if (to.length === 0) {
- var d = new Date();
- to = [d.getFullYear(), d.getMonth(), d.getDate()];
- }
+ return `[[${obj.abbr}]]`;
+ },
+ // https://en.wikipedia.org/wiki/Template:Own
+ own: tmpl => {
+ let obj = toJSON(tmpl, ['author']);
+ let str = 'Own work';
- to = ymd$1(to);
- return {
- from: from,
- to: to
- };
- };
+ if (obj.author) {
+ str += ' by ' + obj.author;
+ }
- var parsers = {
- //generic {{date|year|month|date}} template
- date: function date(tmpl, list) {
- var order = ['year', 'month', 'date', 'hour', 'minute', 'second', 'timezone'];
- var obj = parse$3(tmpl, order);
- var data = ymd$1([obj.year, obj.month, obj.date || obj.day]);
- obj.text = toText$2(data); //make the replacement string
+ return str;
+ },
+ //https://www.mediawiki.org/wiki/Help:Magic_words#Formatting
+ formatnum: tmpl => {
+ tmpl = tmpl.replace(/:/, '|');
+ let obj = toJSON(tmpl, ['number']);
+ let str = obj.number || '';
+ str = str.replace(/,/g, '');
+ let num = Number(str);
+ return num.toLocaleString() || '';
+ },
+ //https://en.wikipedia.org/wiki/Template:Frac
+ frac: tmpl => {
+ let order = ['a', 'b', 'c'];
+ let obj = toJSON(tmpl, order);
- if (obj.timezone) {
- if (obj.timezone === 'Z') {
- obj.timezone = 'UTC';
- }
+ if (obj.c) {
+ return `${obj.a} ${obj.b}/${obj.c}`;
+ }
- obj.text += " (".concat(obj.timezone, ")");
+ if (obj.b) {
+ return `${obj.a}/${obj.b}`;
}
- if (obj.hour && obj.minute) {
- if (obj.second) {
- obj.text = "".concat(obj.hour, ":").concat(obj.minute, ":").concat(obj.second, ", ") + obj.text;
- } else {
- obj.text = "".concat(obj.hour, ":").concat(obj.minute, ", ") + obj.text;
+ return `1/${obj.b}`;
+ },
+ //https://en.wikipedia.org/wiki/Template:Convert#Ranges_of_values
+ convert: tmpl => {
+ let order = ['num', 'two', 'three', 'four'];
+ let obj = toJSON(tmpl, order); //todo: support plural units
+
+ if (obj.two === '-' || obj.two === 'to' || obj.two === 'and') {
+ if (obj.four) {
+ return `${obj.num} ${obj.two} ${obj.three} ${obj.four}`;
}
- }
- if (obj.text) {
- list.push(template(obj));
+ return `${obj.num} ${obj.two} ${obj.three}`;
}
- return obj.text;
+ return `${obj.num} ${obj.two}`;
},
- //support parsing of 'February 10, 1992'
- natural_date: function natural_date(tmpl, list) {
- var order = ['text'];
- var obj = parse$3(tmpl, order);
- var str = obj.text || ''; // - just a year
+ // Large number of aliases - https://en.wikipedia.org/wiki/Template:Tl
+ tl: tmpl => {
+ let order = ['first', 'second'];
+ let obj = toJSON(tmpl, order);
+ return obj.second || obj.first;
+ },
+ //this one's a little different
+ won: tmpl => {
+ let data = toJSON(tmpl, ['text']);
+ return data.place || data.text || _lib$3.titlecase(data.template);
+ },
+ //a convulated way to make a xml tag - https://en.wikipedia.org/wiki/Template:Tag
+ tag: tmpl => {
+ let obj = toJSON(tmpl, ['tag', 'open']);
+ const ignore = {
+ span: true,
+ div: true,
+ p: true
+ }; //pair, empty, close, single
- var date = {};
+ if (!obj.open || obj.open === 'pair') {
+ //just skip generating spans and things..
+ if (ignore[obj.tag]) {
+ return obj.content || '';
+ }
- if (/^[0-9]{4}$/.test(str)) {
- date.year = parseInt(str, 10);
- } else {
- //parse the date, using the js date object (for now?)
- var txt = str.replace(/[a-z]+\/[a-z]+/i, '');
- txt = txt.replace(/[0-9]+:[0-9]+(am|pm)?/i, '');
- var d = new Date(txt);
+ return `<${obj.tag} ${obj.attribs || ''}>${obj.content || ''}${obj.tag}>`;
+ }
- if (isNaN(d.getTime()) === false) {
- date.year = d.getFullYear();
- date.month = d.getMonth() + 1;
- date.date = d.getDate();
+ return '';
+ },
+ //dumb inflector - https://en.wikipedia.org/wiki/Template:Plural
+ plural: tmpl => {
+ tmpl = tmpl.replace(/plural:/, 'plural|');
+ let order = ['num', 'word'];
+ let obj = toJSON(tmpl, order);
+ let num = Number(obj.num);
+ let word = obj.word;
+
+ if (num !== 1) {
+ if (/.y$/.test(word)) {
+ word = word.replace(/y$/, 'ies');
+ } else {
+ word += 's';
}
}
- list.push(template(date));
- return str.trim();
- },
- //just grab the first value, and assume it's a year
- one_year: function one_year(tmpl, list) {
- var order = ['year'];
- var obj = parse$3(tmpl, order);
- var year = Number(obj.year);
- list.push(template({
- year: year
- }));
- return String(year);
+ return num + ' ' + word;
},
- //assume 'y|m|d' | 'y|m|d' // {{BirthDeathAge|B|1976|6|6|1990|8|8}}
- two_dates: function two_dates(tmpl, list) {
- var order = ['b', 'birth_year', 'birth_month', 'birth_date', 'death_year', 'death_month', 'death_date'];
- var obj = parse$3(tmpl, order); //'b' means show birth-date, otherwise show death-date
+ //https://en.wikipedia.org/wiki/Template:DEC
+ dec: tmpl => {
+ let obj = toJSON(tmpl, ['degrees', 'minutes', 'seconds']);
+ let str = (obj.degrees || 0) + '°';
- if (obj.b && obj.b.toLowerCase() === 'b') {
- var _date = ymd$1([obj.birth_year, obj.birth_month, obj.birth_date]);
+ if (obj.minutes) {
+ str += obj.minutes + `′`;
+ }
- list.push(template(_date));
- return toText$2(_date);
+ if (obj.seconds) {
+ str += obj.seconds + '″';
}
- var date = ymd$1([obj.death_year, obj.death_month, obj.death_date]);
- list.push(template(date));
- return toText$2(date);
- },
- age: function age(tmpl) {
- var d = getBoth(tmpl);
- var diff = _delta(d.from, d.to);
- return diff.years || 0;
+ return str;
},
- 'diff-y': function diffY(tmpl) {
- var d = getBoth(tmpl);
- var diff = _delta(d.from, d.to);
+ //https://en.wikipedia.org/wiki/Template:Val
+ val: tmpl => {
+ let obj = toJSON(tmpl, ['number', 'uncertainty']);
+ let num = obj.number;
- if (diff.years === 1) {
- return diff.years + ' year';
+ if (num && Number(num)) {
+ num = Number(num).toLocaleString();
}
- return (diff.years || 0) + ' years';
- },
- 'diff-ym': function diffYm(tmpl) {
- var d = getBoth(tmpl);
- var diff = _delta(d.from, d.to);
- var arr = [];
+ let str = num || ''; //prefix/suffix
- if (diff.years === 1) {
- arr.push(diff.years + ' year');
- } else if (diff.years && diff.years !== 0) {
- arr.push(diff.years + ' years');
+ if (obj.p) {
+ str = obj.p + str;
}
- if (diff.months === 1) {
- arr.push('1 month');
- } else if (diff.months && diff.months !== 0) {
- arr.push(diff.months + ' months');
- }
+ if (obj.s) {
+ str = obj.s + str;
+ } //add units, too
- return arr.join(', ');
- },
- 'diff-ymd': function diffYmd(tmpl) {
- var d = getBoth(tmpl);
- var diff = _delta(d.from, d.to);
- var arr = [];
- if (diff.years === 1) {
- arr.push(diff.years + ' year');
- } else if (diff.years && diff.years !== 0) {
- arr.push(diff.years + ' years');
+ if (obj.u || obj.ul || obj.upl) {
+ str = str + ' ' + (obj.u || obj.ul || obj.upl);
}
- if (diff.months === 1) {
- arr.push('1 month');
- } else if (diff.months && diff.months !== 0) {
- arr.push(diff.months + ' months');
- }
+ return str;
+ },
+ //{{percentage | numerator | denominator | decimals to round to (zero or greater) }}
+ percentage: tmpl => {
+ let obj = toJSON(tmpl, ['numerator', 'denominator', 'decimals']);
+ let num = _lib$3.percentage(obj);
- if (diff.days === 1) {
- arr.push('1 day');
- } else if (diff.days && diff.days !== 0) {
- arr.push(diff.days + ' days');
+ if (num === null) {
+ return '';
}
- return arr.join(', ');
+ return num + '%';
},
- 'diff-yd': function diffYd(tmpl) {
- var d = getBoth(tmpl);
- var diff = _delta(d.from, d.to);
- var arr = [];
+ // {{Percent-done|done=N|total=N|digits=N}}
+ 'percent-done': tmpl => {
+ let obj = toJSON(tmpl, ['done', 'total', 'digits']);
+ let num = _lib$3.percentage({
+ numerator: obj.done,
+ denominator: obj.total,
+ decimals: obj.digits
+ });
- if (diff.years === 1) {
- arr.push(diff.years + ' year');
- } else if (diff.years && diff.years !== 0) {
- arr.push(diff.years + ' years');
- } //ergh...
+ if (num === null) {
+ return '';
+ }
+ return `${obj.done} (${num}%) done`;
+ }
+ };
- diff.days += (diff.months || 0) * 30;
+ var flags = [['🇦🇩', 'and', 'andorra'], ['🇦🇪', 'are', 'united arab emirates'], ['🇦🇫', 'afg', 'afghanistan'], ['🇦🇬', 'atg', 'antigua and barbuda'], ['🇦🇮', 'aia', 'anguilla'], ['🇦🇱', 'alb', 'albania'], ['🇦🇲', 'arm', 'armenia'], ['🇦🇴', 'ago', 'angola'], ['🇦🇶', 'ata', 'antarctica'], ['🇦🇷', 'arg', 'argentina'], ['🇦🇸', 'asm', 'american samoa'], ['🇦🇹', 'aut', 'austria'], ['🇦🇺', 'aus', 'australia'], ['🇦🇼', 'abw', 'aruba'], ['🇦🇽', 'ala', 'åland islands'], ['🇦🇿', 'aze', 'azerbaijan'], ['🇧🇦', 'bih', 'bosnia and herzegovina'], ['🇧🇧', 'brb', 'barbados'], ['🇧🇩', 'bgd', 'bangladesh'], ['🇧🇪', 'bel', 'belgium'], ['🇧🇫', 'bfa', 'burkina faso'], ['🇧🇬', 'bgr', 'bulgaria'], ['🇧🇬', 'bul', 'bulgaria'], //dupe
+ ['🇧🇭', 'bhr', 'bahrain'], ['🇧🇮', 'bdi', 'burundi'], ['🇧🇯', 'ben', 'benin'], ['🇧🇱', 'blm', 'saint barthélemy'], ['🇧🇲', 'bmu', 'bermuda'], ['🇧🇳', 'brn', 'brunei darussalam'], ['🇧🇴', 'bol', 'bolivia'], ['🇧🇶', 'bes', 'bonaire, sint eustatius and saba'], ['🇧🇷', 'bra', 'brazil'], ['🇧🇸', 'bhs', 'bahamas'], ['🇧🇹', 'btn', 'bhutan'], ['🇧🇻', 'bvt', 'bouvet island'], ['🇧🇼', 'bwa', 'botswana'], ['🇧🇾', 'blr', 'belarus'], ['🇧🇿', 'blz', 'belize'], ['🇨🇦', 'can', 'canada'], ['🇨🇨', 'cck', 'cocos (keeling) islands'], ['🇨🇩', 'cod', 'congo'], ['🇨🇫', 'caf', 'central african republic'], ['🇨🇬', 'cog', 'congo'], ['🇨🇭', 'che', 'switzerland'], ['🇨🇮', 'civ', 'côte d\'ivoire'], ['🇨🇰', 'cok', 'cook islands'], ['🇨🇱', 'chl', 'chile'], ['🇨🇲', 'cmr', 'cameroon'], ['🇨🇳', 'chn', 'china'], ['🇨🇴', 'col', 'colombia'], ['🇨🇷', 'cri', 'costa rica'], ['🇨🇺', 'cub', 'cuba'], ['🇨🇻', 'cpv', 'cape verde'], ['🇨🇼', 'cuw', 'curaçao'], ['🇨🇽', 'cxr', 'christmas island'], ['🇨🇾', 'cyp', 'cyprus'], ['🇨🇿', 'cze', 'czech republic'], ['🇩🇪', 'deu', 'germany'], ['🇩🇪', 'ger', 'germany'], //alias
+ ['🇩🇯', 'dji', 'djibouti'], ['🇩🇰', 'dnk', 'denmark'], ['🇩🇲', 'dma', 'dominica'], ['🇩🇴', 'dom', 'dominican republic'], ['🇩🇿', 'dza', 'algeria'], ['🇪🇨', 'ecu', 'ecuador'], ['🇪🇪', 'est', 'estonia'], ['🇪🇬', 'egy', 'egypt'], ['🇪🇭', 'esh', 'western sahara'], ['🇪🇷', 'eri', 'eritrea'], ['🇪🇸', 'esp', 'spain'], ['🇪🇹', 'eth', 'ethiopia'], ['🇫🇮', 'fin', 'finland'], ['🇫🇯', 'fji', 'fiji'], ['🇫🇰', 'flk', 'falkland islands (malvinas)'], ['🇫🇲', 'fsm', 'micronesia'], ['🇫🇴', 'fro', 'faroe islands'], ['🇫🇷', 'fra', 'france'], ['🇬🇦', 'gab', 'gabon'], ['🇬🇧', 'gbr', 'united kingdom'], ['🇬🇩', 'grd', 'grenada'], //['🇬🇪', 'geo', 'georgia'],
+ ['🇬🇫', 'guf', 'french guiana'], ['🇬🇬', 'ggy', 'guernsey'], ['🇬🇭', 'gha', 'ghana'], ['🇬🇮', 'gib', 'gibraltar'], ['🇬🇱', 'grl', 'greenland'], ['🇬🇲', 'gmb', 'gambia'], ['🇬🇳', 'gin', 'guinea'], ['🇬🇵', 'glp', 'guadeloupe'], ['🇬🇶', 'gnq', 'equatorial guinea'], ['🇬🇷', 'grc', 'greece'], ['🇬🇸', 'sgs', 'south georgia'], ['🇬🇹', 'gtm', 'guatemala'], ['🇬🇺', 'gum', 'guam'], ['🇬🇼', 'gnb', 'guinea-bissau'], ['🇬🇾', 'guy', 'guyana'], ['🇭🇰', 'hkg', 'hong kong'], ['🇭🇲', 'hmd', 'heard island and mcdonald islands'], ['🇭🇳', 'hnd', 'honduras'], ['🇭🇷', 'hrv', 'croatia'], ['🇭🇹', 'hti', 'haiti'], ['🇭🇺', 'hun', 'hungary'], ['🇮🇩', 'idn', 'indonesia'], ['🇮🇪', 'irl', 'ireland'], ['🇮🇱', 'isr', 'israel'], ['🇮🇲', 'imn', 'isle of man'], ['🇮🇳', 'ind', 'india'], ['🇮🇴', 'iot', 'british indian ocean territory'], ['🇮🇶', 'irq', 'iraq'], ['🇮🇷', 'irn', 'iran'], ['🇮🇸', 'isl', 'iceland'], ['🇮🇹', 'ita', 'italy'], ['🇯🇪', 'jey', 'jersey'], ['🇯🇲', 'jam', 'jamaica'], ['🇯🇴', 'jor', 'jordan'], ['🇯🇵', 'jpn', 'japan'], ['🇰🇪', 'ken', 'kenya'], ['🇰🇬', 'kgz', 'kyrgyzstan'], ['🇰🇭', 'khm', 'cambodia'], ['🇰🇮', 'kir', 'kiribati'], ['🇰🇲', 'com', 'comoros'], ['🇰🇳', 'kna', 'saint kitts and nevis'], ['🇰🇵', 'prk', 'north korea'], ['🇰🇷', 'kor', 'south korea'], ['🇰🇼', 'kwt', 'kuwait'], ['🇰🇾', 'cym', 'cayman islands'], ['🇰🇿', 'kaz', 'kazakhstan'], ['🇱🇦', 'lao', 'lao people\'s democratic republic'], ['🇱🇧', 'lbn', 'lebanon'], ['🇱🇨', 'lca', 'saint lucia'], ['🇱🇮', 'lie', 'liechtenstein'], ['🇱🇰', 'lka', 'sri lanka'], ['🇱🇷', 'lbr', 'liberia'], ['🇱🇸', 'lso', 'lesotho'], ['🇱🇹', 'ltu', 'lithuania'], ['🇱🇺', 'lux', 'luxembourg'], ['🇱🇻', 'lva', 'latvia'], ['🇱🇾', 'lby', 'libya'], ['🇲🇦', 'mar', 'morocco'], ['🇲🇨', 'mco', 'monaco'], ['🇲🇩', 'mda', 'moldova'], ['🇲🇪', 'mne', 'montenegro'], ['🇲🇫', 'maf', 'saint martin (french part)'], ['🇲🇬', 'mdg', 'madagascar'], ['🇲🇭', 'mhl', 'marshall islands'], ['🇲🇰', 'mkd', 'macedonia'], ['🇲🇱', 'mli', 'mali'], ['🇲🇲', 'mmr', 'myanmar'], ['🇲🇳', 'mng', 'mongolia'], ['🇲🇴', 'mac', 'macao'], ['🇲🇵', 'mnp', 'northern mariana islands'], ['🇲🇶', 'mtq', 'martinique'], ['🇲🇷', 'mrt', 'mauritania'], ['🇲🇸', 'msr', 'montserrat'], ['🇲🇹', 'mlt', 'malta'], ['🇲🇺', 'mus', 'mauritius'], ['🇲🇻', 'mdv', 'maldives'], ['🇲🇼', 'mwi', 'malawi'], ['🇲🇽', 'mex', 'mexico'], ['🇲🇾', 'mys', 'malaysia'], ['🇲🇿', 'moz', 'mozambique'], ['🇳🇦', 'nam', 'namibia'], ['🇳🇨', 'ncl', 'new caledonia'], ['🇳🇪', 'ner', 'niger'], ['🇳🇫', 'nfk', 'norfolk island'], ['🇳🇬', 'nga', 'nigeria'], ['🇳🇮', 'nic', 'nicaragua'], ['🇳🇱', 'nld', 'netherlands'], ['🇳🇴', 'nor', 'norway'], ['🇳🇵', 'npl', 'nepal'], ['🇳🇷', 'nru', 'nauru'], ['🇳🇺', 'niu', 'niue'], ['🇳🇿', 'nzl', 'new zealand'], ['🇴🇲', 'omn', 'oman'], ['🇵🇦', 'pan', 'panama'], ['🇵🇪', 'per', 'peru'], ['🇵🇫', 'pyf', 'french polynesia'], ['🇵🇬', 'png', 'papua new guinea'], ['🇵🇭', 'phl', 'philippines'], ['🇵🇰', 'pak', 'pakistan'], ['🇵🇱', 'pol', 'poland'], ['🇵🇲', 'spm', 'saint pierre and miquelon'], ['🇵🇳', 'pcn', 'pitcairn'], ['🇵🇷', 'pri', 'puerto rico'], ['🇵🇸', 'pse', 'palestinian territory'], ['🇵🇹', 'prt', 'portugal'], ['🇵🇼', 'plw', 'palau'], ['🇵🇾', 'pry', 'paraguay'], ['🇶🇦', 'qat', 'qatar'], ['🇷🇪', 'reu', 'réunion'], ['🇷🇴', 'rou', 'romania'], ['🇷🇸', 'srb', 'serbia'], ['🇷🇺', 'rus', 'russia'], ['🇷🇼', 'rwa', 'rwanda'], ['🇸🇦', 'sau', 'saudi arabia'], ['🇸🇧', 'slb', 'solomon islands'], ['🇸🇨', 'syc', 'seychelles'], ['🇸🇩', 'sdn', 'sudan'], ['🇸🇪', 'swe', 'sweden'], ['🇸🇬', 'sgp', 'singapore'], ['🇸🇭', 'shn', 'saint helena, ascension and tristan da cunha'], ['🇸🇮', 'svn', 'slovenia'], ['🇸🇯', 'sjm', 'svalbard and jan mayen'], ['🇸🇰', 'svk', 'slovakia'], ['🇸🇱', 'sle', 'sierra leone'], ['🇸🇲', 'smr', 'san marino'], ['🇸🇳', 'sen', 'senegal'], ['🇸🇴', 'som', 'somalia'], ['🇸🇷', 'sur', 'suriname'], ['🇸🇸', 'ssd', 'south sudan'], ['🇸🇹', 'stp', 'sao tome and principe'], ['🇸🇻', 'slv', 'el salvador'], ['🇸🇽', 'sxm', 'sint maarten (dutch part)'], ['🇸🇾', 'syr', 'syrian arab republic'], ['🇸🇿', 'swz', 'swaziland'], ['🇹🇨', 'tca', 'turks and caicos islands'], ['🇹🇩', 'tcd', 'chad'], ['🇹🇫', 'atf', 'french southern territories'], ['🇹🇬', 'tgo', 'togo'], ['🇹🇭', 'tha', 'thailand'], ['🇹🇯', 'tjk', 'tajikistan'], ['🇹🇰', 'tkl', 'tokelau'], ['🇹🇱', 'tls', 'timor-leste'], ['🇹🇲', 'tkm', 'turkmenistan'], ['🇹🇳', 'tun', 'tunisia'], ['🇹🇴', 'ton', 'tonga'], ['🇹🇷', 'tur', 'turkey'], ['🇹🇹', 'tto', 'trinidad and tobago'], ['🇹🇻', 'tuv', 'tuvalu'], ['🇹🇼', 'twn', 'taiwan'], ['🇹🇿', 'tza', 'tanzania'], ['🇺🇦', 'ukr', 'ukraine'], ['🇺🇬', 'uga', 'uganda'], ['🇺🇲', 'umi', 'united states minor outlying islands'], ['🇺🇸', 'us', 'united states'], //alias
+ ['🇺🇸', 'usa', 'united states'], ['🇺🇾', 'ury', 'uruguay'], ['🇺🇿', 'uzb', 'uzbekistan'], ['🇻🇦', 'vat', 'vatican city'], ['🇻🇨', 'vct', 'saint vincent and the grenadines'], ['🇻🇪', 'ven', 'venezuela'], ['🇻🇬', 'vgb', 'virgin islands, british'], ['🇻🇮', 'vir', 'virgin islands, u.s.'], ['🇻🇳', 'vnm', 'viet nam'], ['🇻🇺', 'vut', 'vanuatu'], ['🇼🇫', 'wlf', 'wallis and futuna'], ['🇼🇸', 'wsm', 'samoa'], ['🇾🇪', 'yem', 'yemen'], ['🇾🇹', 'myt', 'mayotte'], ['🇿🇦', 'zaf', 'south africa'], ['🇿🇲', 'zmb', 'zambia'], ['🇿🇼 ', 'zwe', 'zimbabwe'], //others (later unicode versions)
+ ['🇺🇳', 'un', 'united nations'], ['🏴', 'eng', 'england'], ['🏴', 'sct', 'scotland'], ['🏴', 'wal', 'wales'], ['🇪🇺', 'eu', 'european union']];
- if (diff.days === 1) {
- arr.push('1 day');
- } else if (diff.days && diff.days !== 0) {
- arr.push(diff.days + ' days');
+ let templates$8 = {
+ //https://en.wikipedia.org/wiki/Template:Flag
+ // {{flag|USA}} → USA
+ flag: tmpl => {
+ let order = ['flag', 'variant'];
+ let obj = toJSON(tmpl, order);
+ let name = obj.flag || '';
+ obj.flag = (obj.flag || '').toLowerCase();
+ let found = flags.find(a => obj.flag === a[1] || obj.flag === a[2]) || [];
+ let flag = found[0] || '';
+ return `${flag} [[${found[2]}|${name}]]`;
+ },
+ // {{flagcountry|USA}} → United States
+ flagcountry: tmpl => {
+ let order = ['flag', 'variant'];
+ let obj = toJSON(tmpl, order);
+ obj.flag = (obj.flag || '').toLowerCase();
+ let found = flags.find(a => obj.flag === a[1] || obj.flag === a[2]) || [];
+ let flag = found[0] || '';
+ return `${flag} [[${found[2]}]]`;
+ },
+ // (unlinked flag-country)
+ flagcu: tmpl => {
+ let order = ['flag', 'variant'];
+ let obj = toJSON(tmpl, order);
+ obj.flag = (obj.flag || '').toLowerCase();
+ let found = flags.find(a => obj.flag === a[1] || obj.flag === a[2]) || [];
+ let flag = found[0] || '';
+ return `${flag} ${found[2]}`;
+ },
+ //https://en.wikipedia.org/wiki/Template:Flagicon
+ // {{flagicon|USA}} → United States
+ flagicon: tmpl => {
+ let order = ['flag', 'variant'];
+ let obj = toJSON(tmpl, order);
+ obj.flag = (obj.flag || '').toLowerCase();
+ let found = flags.find(a => obj.flag === a[1] || obj.flag === a[2]);
+
+ if (!found) {
+ return '';
}
- return arr.join(', ');
+ return `[[${found[2]}|${found[0]}]]`;
+ },
+ //unlinked flagicon
+ flagdeco: tmpl => {
+ let order = ['flag', 'variant'];
+ let obj = toJSON(tmpl, order);
+ obj.flag = (obj.flag || '').toLowerCase();
+ let found = flags.find(a => obj.flag === a[1] || obj.flag === a[2]) || [];
+ return found[0] || '';
},
- 'diff-d': function diffD(tmpl) {
- var d = getBoth(tmpl);
- var diff = _delta(d.from, d.to);
- var arr = []; //ergh...
+ //same, but a soccer team
+ fb: tmpl => {
+ let order = ['flag', 'variant'];
+ let obj = toJSON(tmpl, order);
+ obj.flag = (obj.flag || '').toLowerCase();
+ let found = flags.find(a => obj.flag === a[1] || obj.flag === a[2]);
- diff.days += (diff.years || 0) * 365;
- diff.days += (diff.months || 0) * 30;
+ if (!found) {
+ return '';
+ }
- if (diff.days === 1) {
- arr.push('1 day');
- } else if (diff.days && diff.days !== 0) {
- arr.push(diff.days + ' days');
+ return `${found[0]} [[${found[2]} national football team|${found[2]}]]`;
+ },
+ fbicon: tmpl => {
+ let order = ['flag', 'variant'];
+ let obj = toJSON(tmpl, order);
+ obj.flag = (obj.flag || '').toLowerCase();
+ let found = flags.find(a => obj.flag === a[1] || obj.flag === a[2]);
+
+ if (!found) {
+ return '';
}
- return arr.join(', ');
- }
- };
- var parsers_1 = parsers;
+ return ` [[${found[2]} national football team|${found[0]}]]`;
+ },
+ flagathlete: tmpl => {
+ let order = ['name', 'flag', 'variant'];
+ let obj = toJSON(tmpl, order);
+ obj.flag = (obj.flag || '').toLowerCase();
+ let found = flags.find(a => obj.flag === a[1] || obj.flag === a[2]);
- //not all too fancy - used in {{timesince}}
- var timeSince = function timeSince(str) {
- var d = new Date(str);
+ if (!found) {
+ return `[[${obj.name || ''}]]`;
+ }
- if (isNaN(d.getTime())) {
- return '';
+ return `${found[0]} [[${obj.name || ''}]] (${found[1].toUpperCase()})`;
}
+ }; //support {{can}}
- var now = new Date();
- var delta = now.getTime() - d.getTime();
- var predicate = 'ago';
-
- if (delta < 0) {
- predicate = 'from now';
- delta = Math.abs(delta);
- } //figure out units
+ flags.forEach(a => {
+ templates$8[a[1]] = () => {
+ return a[0];
+ };
+ });
+ var flags_1 = templates$8;
+ const titlecase = _lib$3.titlecase; //https://en.wikipedia.org/wiki/Template:Yes
- var hours = delta / 1000 / 60 / 60;
- var days = hours / 24;
+ let templates$7 = {};
+ let cells = ['rh', 'rh2', 'yes', 'no', 'maybe', 'eliminated', 'lost', 'safe', 'active', 'site active', 'coming soon', 'good', 'won', 'nom', 'sho', 'longlisted', 'tba', 'success', 'operational', 'failure', 'partial', 'regional', 'maybecheck', 'partial success', 'partial failure', 'okay', 'yes-no', 'some', 'nonpartisan', 'pending', 'unofficial', 'unofficial2', 'usually', 'rarely', 'sometimes', 'any', 'varies', 'black', 'non-album single', 'unreleased', 'unknown', 'perhaps', 'depends', 'included', 'dropped', 'terminated', 'beta', 'table-experimental', 'free', 'proprietary', 'nonfree', 'needs', 'nightly', 'release-candidate', 'planned', 'scheduled', 'incorrect', 'no result', 'cmain', 'calso starring', 'crecurring', 'cguest', 'not yet', 'optional'];
+ cells.forEach(str => {
+ templates$7[str] = tmpl => {
+ let data = toJSON(tmpl, ['text']);
+ return data.text || titlecase(data.template);
+ };
+ }); //these ones have a text result
- if (days < 365) {
- return parseInt(days, 10) + ' days ' + predicate;
- }
+ let moreCells = [['active fire', 'Active'], ['site active', 'Active'], ['site inactive', 'Inactive'], ['yes2', ''], ['no2', ''], ['ya', '✅'], ['na', '❌'], ['nom', 'Nominated'], ['sho', 'Shortlisted'], ['tba', 'TBA'], ['maybecheck', '✔️'], ['okay', 'Neutral'], ['n/a', 'N/A'], ['sdash', '—'], ['dunno', '?'], ['draw', ''], ['cnone', ''], ['nocontest', '']];
+ moreCells.forEach(a => {
+ templates$7[a[0]] = tmpl => {
+ let data = toJSON(tmpl, ['text']);
+ return data.text || a[1];
+ };
+ });
+ var tableCell = templates$7;
- var years = days / 365;
- return parseInt(years, 10) + ' years ' + predicate;
- };
+ var textOnly = Object.assign({}, hardcoded, shorthand$1, functions$2, flags_1, tableCell);
- var _timeSince = timeSince;
+ let templates$6 = {}; // these all have ['id', 'name']
- var date = parsers_1.date;
- var natural_date = parsers_1.natural_date;
- var months = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'];
- var days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']; //date- templates we support
+ let idName = ['goodreads author', 'twitter', 'facebook', 'instagram', 'tumblr', 'pinterest', 'espn nfl', 'espn nhl', 'espn fc', 'hockeydb', 'fifa player', 'worldcat', 'worldcat id', 'nfl player', 'ted speaker', 'playmate'];
+ idName.forEach(name => {
+ templates$6[name] = ['id', 'name'];
+ });
+ var idName_1 = templates$6;
- var dateTmpl = Object.assign({}, misc_1, {
- currentday: function currentday() {
- var d = new Date();
- return String(d.getDate());
- },
- currentdayname: function currentdayname() {
- var d = new Date();
- return days[d.getDay()];
- },
- currentmonth: function currentmonth() {
- var d = new Date();
- return months[d.getMonth()];
- },
- currentyear: function currentyear() {
- var d = new Date();
- return String(d.getFullYear());
- },
- monthyear: function monthyear() {
- var d = new Date();
- return months[d.getMonth()] + ' ' + d.getFullYear();
- },
- 'monthyear-1': function monthyear1() {
- var d = new Date();
- d.setMonth(d.getMonth() - 1);
- return months[d.getMonth()] + ' ' + d.getFullYear();
- },
- 'monthyear+1': function monthyear1() {
- var d = new Date();
- d.setMonth(d.getMonth() + 1);
- return months[d.getMonth()] + ' ' + d.getFullYear();
- },
- //Explictly-set dates - https://en.wikipedia.org/wiki/Template:Date
- date: 0,
- 'time ago': function timeAgo(tmpl) {
- var order = ['date', 'fmt'];
- var time = parse$3(tmpl, order).date;
- return _timeSince(time);
- },
- //https://en.wikipedia.org/wiki/Template:Birth_date_and_age
- 'birth date and age': function birthDateAndAge(tmpl, list) {
- var order = ['year', 'month', 'day'];
- var obj = parse$3(tmpl, order); //support 'one property' version
+ let templates$5 = {}; // these all have ['id', 'title', 'description', 'section']
- if (obj.year && /[a-z]/i.test(obj.year)) {
- return natural_date(tmpl, list);
- }
+ let idTitle = ['imdb title', //https://en.wikipedia.org/wiki/Template:IMDb_title
+ 'imdb name', 'imdb episode', 'imdb event', 'afi film', 'allmovie title', 'allgame', 'tcmdb title', 'discogs artist', 'discogs label', 'discogs release', 'discogs master', 'librivox author', 'musicbrainz artist', 'musicbrainz label', 'musicbrainz recording', 'musicbrainz release', 'musicbrainz work', 'youtube', 'goodreads book', 'dmoz' //https://en.wikipedia.org/wiki/Template:DMOZ
+ ];
+ idTitle.forEach(name => {
+ templates$5[name] = ['id', 'title', 'description', 'section'];
+ });
+ var idTitle_1 = templates$5;
+ var functions$1 = {
+ // https://en.wikipedia.org/wiki/Template:IPA
+ ipa: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['transcription', 'lang', 'audio']);
+ obj.lang = _lib$3.getLang(obj.template);
+ obj.template = 'ipa';
list.push(obj);
- obj = _format.ymd([obj.year, obj.month, obj.day]);
- return _format.toText(obj);
+ return '';
},
- 'birth year and age': function birthYearAndAge(tmpl, list) {
- var order = ['birth_year', 'birth_month'];
- var obj = parse$3(tmpl, order); //support 'one property' version
-
- if (obj.death_year && /[a-z]/i.test(obj.death_year)) {
- return natural_date(tmpl, list);
- }
-
+ //https://en.wikipedia.org/wiki/Template:IPAc-en
+ ipac: (tmpl, list) => {
+ let obj = toJSON(tmpl);
+ obj.transcription = (obj.list || []).join(',');
+ delete obj.list;
+ obj.lang = _lib$3.getLang(obj.template);
+ obj.template = 'ipac';
list.push(obj);
- var age = new Date().getFullYear() - parseInt(obj.birth_year, 10);
- obj = _format.ymd([obj.birth_year, obj.birth_month]);
- var str = _format.toText(obj);
+ return '';
+ },
+ quote: (tmpl, list) => {
+ let order = ['text', 'author'];
+ let obj = toJSON(tmpl, order);
+ list.push(obj); //create plaintext version
- if (age) {
- str += " (age ".concat(age, ")");
- }
+ if (obj.text) {
+ let str = `"${obj.text}"`;
- return str;
- },
- 'death year and age': function deathYearAndAge(tmpl, list) {
- var order = ['death_year', 'birth_year', 'death_month'];
- var obj = parse$3(tmpl, order); //support 'one property' version
+ if (obj.author) {
+ str += '\n\n';
+ str += ` - ${obj.author}`;
+ }
- if (obj.death_year && /[a-z]/i.test(obj.death_year)) {
- return natural_date(tmpl, list);
+ return str + '\n';
}
+ return '';
+ },
+ //this one sucks - https://en.wikipedia.org/wiki/Template:GNIS
+ 'cite gnis': (tmpl, list) => {
+ let order = ['id', 'name', 'type'];
+ let obj = toJSON(tmpl, order);
+ obj.type = 'gnis';
+ obj.template = 'citation';
list.push(obj);
- obj = _format.ymd([obj.death_year, obj.death_month]);
- return _format.toText(obj);
+ return '';
},
- //https://en.wikipedia.org/wiki/Template:Birth_date_and_age2
- 'birth date and age2': function birthDateAndAge2(tmpl, list) {
- var order = ['at_year', 'at_month', 'at_day', 'birth_year', 'birth_month', 'birth_day'];
- var obj = parse$3(tmpl, order);
+ 'spoken wikipedia': (tmpl, list) => {
+ let order = ['file', 'date'];
+ let obj = toJSON(tmpl, order);
+ obj.template = 'audio';
list.push(obj);
- obj = _format.ymd([obj.birth_year, obj.birth_month, obj.birth_day]);
- return _format.toText(obj);
+ return '';
},
- //https://en.wikipedia.org/wiki/Template:Birth_based_on_age_as_of_date
- 'birth based on age as of date': function birthBasedOnAgeAsOfDate(tmpl, list) {
- var order = ['age', 'year', 'month', 'day'];
- var obj = parse$3(tmpl, order);
+ //yellow card
+ yel: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['min']);
list.push(obj);
- var age = parseInt(obj.age, 10);
- var year = parseInt(obj.year, 10);
- var born = year - age;
- if (born && age) {
- return "".concat(born, " (age ").concat(obj.age, ")");
+ if (obj.min) {
+ return `yellow: ${obj.min || ''}'`; //no yellow-card emoji
}
- return "(age ".concat(obj.age, ")");
+ return '';
},
- //https://en.wikipedia.org/wiki/Template:Death_date_and_given_age
- 'death date and given age': function deathDateAndGivenAge(tmpl, list) {
- var order = ['year', 'month', 'day', 'age'];
- var obj = parse$3(tmpl, order);
+ subon: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['min']);
list.push(obj);
- obj = _format.ymd([obj.year, obj.month, obj.day]);
- var str = _format.toText(obj);
- if (obj.age) {
- str += " (age ".concat(obj.age, ")");
+ if (obj.min) {
+ return `sub on: ${obj.min || ''}'`; //no yellow-card emoji
}
- return str;
+ return '';
},
- //sortable dates -
- dts: function dts(tmpl) {
- //remove formatting stuff, ewww
- tmpl = tmpl.replace(/\|format=[ymd]+/i, '');
- tmpl = tmpl.replace(/\|abbr=(on|off)/i, '');
- var order = ['year', 'month', 'date', 'bc'];
- var obj = parse$3(tmpl, order);
-
- if (obj.date && obj.month && obj.year) {
- //render 'june 5 2018'
- if (/[a-z]/.test(obj.month) === true) {
- return [obj.month, obj.date, obj.year].join(' ');
- }
-
- return [obj.year, obj.month, obj.date].join('-');
- }
-
- if (obj.month && obj.year) {
- return [obj.year, obj.month].join('-');
- }
-
- if (obj.year) {
- if (obj.year < 0) {
- obj.year = Math.abs(obj.year) + ' BC';
- }
+ suboff: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['min']);
+ list.push(obj);
- return obj.year;
+ if (obj.min) {
+ return `sub off: ${obj.min || ''}'`; //no yellow-card emoji
}
return '';
},
- //date/age/time templates
- start: date,
- end: date,
- birth: date,
- death: date,
- 'start date': date,
- 'end date': date,
- 'birth date': date,
- 'death date': date,
- 'start date and age': date,
- 'end date and age': date,
- //this is insane (hyphen ones are different)
- 'start-date': natural_date,
- 'end-date': natural_date,
- 'birth-date': natural_date,
- 'death-date': natural_date,
- 'birth-date and age': natural_date,
- 'birth-date and given age': natural_date,
- 'death-date and age': natural_date,
- 'death-date and given age': natural_date,
- birthdeathage: parsers_1.two_dates,
- dob: date,
- // 'birth date and age2': date,
- age: parsers_1.age,
- 'age nts': parsers_1.age,
- 'age in years': parsers_1['diff-y'],
- 'age in years and months': parsers_1['diff-ym'],
- 'age in years, months and days': parsers_1['diff-ymd'],
- 'age in years and days': parsers_1['diff-yd'],
- 'age in days': parsers_1['diff-d'] // 'age in years, months, weeks and days': true,
- // 'age as of date': true,
-
- }); //aliases
-
- dateTmpl.localday = dateTmpl.currentday;
- dateTmpl.localdayname = dateTmpl.currentdayname;
- dateTmpl.localmonth = dateTmpl.currentmonth;
- dateTmpl.localyear = dateTmpl.currentyear;
- dateTmpl.currentmonthname = dateTmpl.currentmonth;
- dateTmpl.currentmonthabbrev = dateTmpl.currentmonth;
- dateTmpl['death date and age'] = dateTmpl['birth date and age'];
- dateTmpl.bda = dateTmpl['birth date and age'];
- dateTmpl['birth date based on age at death'] = dateTmpl['birth based on age as of date'];
- var dates = dateTmpl;
-
- var templates = {
- //a convulated way to make a xml tag - https://en.wikipedia.org/wiki/Template:Tag
- tag: function tag(tmpl) {
- var obj = parse$3(tmpl, ['tag', 'open']);
- var ignore = {
- span: true,
- div: true,
- p: true
- }; //pair, empty, close, single
-
- if (!obj.open || obj.open === 'pair') {
- //just skip generating spans and things..
- if (ignore[obj.tag]) {
- return obj.content || '';
- }
+ //https://en.wikipedia.org/wiki/Template:Sfn
+ sfn: (tmpl, list, parser, alias) => {
+ let order = ['author', 'year', 'location'];
+ let obj = toJSON(tmpl, order);
- return "<".concat(obj.tag, " ").concat(obj.attribs || '', ">").concat(obj.content || '', "").concat(obj.tag, ">");
+ if (alias) {
+ obj.name = obj.template;
+ obj.teplate = alias;
}
+ list.push(obj);
return '';
},
- //dumb inflector - https://en.wikipedia.org/wiki/Template:Plural
- plural: function plural(tmpl) {
- tmpl = tmpl.replace(/plural:/, 'plural|');
- var order = ['num', 'word'];
- var obj = parse$3(tmpl, order);
- var num = Number(obj.num);
- var word = obj.word;
-
- if (num !== 1) {
- if (/.y$/.test(word)) {
- word = word.replace(/y$/, 'ies');
- } else {
- word += 's';
- }
- }
-
- return num + ' ' + word;
- },
- // https://en.wikipedia.org/wiki/Template:First_word
- 'first word': function firstWord(tmpl) {
- var obj = parse$3(tmpl, ['text']);
- var str = obj.text;
+ //https://en.wikipedia.org/wiki/Template:Redirect
+ redirect: (tmpl, list) => {
+ let data = toJSON(tmpl, ['redirect']);
+ let lines = data.list || [];
+ let links = [];
- if (obj.sep) {
- return str.split(obj.sep)[0];
+ for (let i = 0; i < lines.length; i += 2) {
+ links.push({
+ page: lines[i + 1],
+ desc: lines[i]
+ });
}
- return str.split(' ')[0];
- },
- trunc: function trunc(tmpl) {
- var order = ['str', 'len'];
- var obj = parse$3(tmpl, order);
- return obj.str.substr(0, obj.len);
- },
- 'str mid': function strMid(tmpl) {
- var order = ['str', 'start', 'end'];
- var obj = parse$3(tmpl, order);
- var start = parseInt(obj.start, 10) - 1;
- var end = parseInt(obj.end, 10);
- return obj.str.substr(start, end);
+ let obj = {
+ template: 'redirect',
+ redirect: data.redirect,
+ links: links
+ };
+ list.push(obj);
+ return '';
},
- //grab the first, second or third pipe
- p1: 0,
- p2: 1,
- p3: 2,
- //formatting things - https://en.wikipedia.org/wiki/Template:Nobold
- braces: function braces(tmpl) {
- var obj = parse$3(tmpl, ['text']);
- var attrs = '';
-
- if (obj.list) {
- attrs = '|' + obj.list.join('|');
- }
+ //https://en.wikipedia.org/wiki/Template:Sister_project_links
+ 'sister project links': (tmpl, list) => {
+ let data = toJSON(tmpl); //rename 'wd' to 'wikidata'
- return '{{' + (obj.text || '') + attrs + '}}';
- },
- nobold: 0,
- noitalic: 0,
- nocaps: 0,
- syntaxhighlight: function syntaxhighlight(tmpl, list) {
- var obj = parse$3(tmpl);
+ let links = {};
+ Object.keys(_lib$3.sisterProjects).forEach(k => {
+ if (data.hasOwnProperty(k) === true) {
+ links[_lib$3.sisterProjects[k]] = data[k]; //.text();
+ }
+ });
+ let obj = {
+ template: 'sister project links',
+ links: links
+ };
list.push(obj);
- return obj.code || '';
+ return '';
},
- samp: function samp(tmpl, list) {
- var obj = parse$3(tmpl, ['1']);
+ //https://en.wikipedia.org/wiki/Template:Subject_bar
+ 'subject bar': (tmpl, list) => {
+ let data = toJSON(tmpl);
+ Object.keys(data).forEach(k => {
+ //rename 'voy' to 'wikivoyage'
+ if (_lib$3.sisterProjects.hasOwnProperty(k)) {
+ data[_lib$3.sisterProjects[k]] = data[k];
+ delete data[k];
+ }
+ });
+ let obj = {
+ template: 'subject bar',
+ links: data
+ };
list.push(obj);
- return obj['1'] || '';
- },
- //https://en.wikipedia.org/wiki/Template:Visible_anchor
- vanchor: 0,
- //https://en.wikipedia.org/wiki/Template:Resize
- resize: 1,
- //https://en.wikipedia.org/wiki/Template:Ra
- ra: function ra(tmpl) {
- var obj = parse$3(tmpl, ['hours', 'minutes', 'seconds']);
- return [obj.hours || 0, obj.minutes || 0, obj.seconds || 0].join(':');
- },
- //https://en.wikipedia.org/wiki/Template:Deg2HMS
- deg2hms: function deg2hms(tmpl) {
- //this template should do the conversion
- var obj = parse$3(tmpl, ['degrees']);
- return (obj.degrees || '') + '°';
+ return '';
},
- hms2deg: function hms2deg(tmpl) {
- //this template should do the conversion too
- var obj = parse$3(tmpl, ['hours', 'minutes', 'seconds']);
- return [obj.hours || 0, obj.minutes || 0, obj.seconds || 0].join(':');
+ //amazingly, this one does not obey any known patterns
+ //https://en.wikipedia.org/wiki/Template:Gallery
+ gallery: (tmpl, list) => {
+ let obj = toJSON(tmpl);
+ let images = (obj.list || []).filter(line => /^ *File ?:/.test(line));
+ images = images.map(file => {
+ let img = {
+ file: file
+ }; // todo: add lang and domain information
+
+ return new Image_1(img).json();
+ });
+ obj = {
+ template: 'gallery',
+ images: images
+ };
+ list.push(obj);
+ return '';
},
- decdeg: function decdeg(tmpl) {
- //this template should do the conversion too
- var obj = parse$3(tmpl, ['deg', 'min', 'sec', 'hem', 'rnd']);
- return (obj.deg || obj.degrees) + '°';
+ //https://en.wikipedia.org/wiki/Template:Sky
+ sky: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['asc_hours', 'asc_minutes', 'asc_seconds', 'dec_sign', 'dec_degrees', 'dec_minutes', 'dec_seconds', 'distance']);
+ let template = {
+ template: 'sky',
+ ascension: {
+ hours: obj.asc_hours,
+ minutes: obj.asc_minutes,
+ seconds: obj.asc_seconds
+ },
+ declination: {
+ sign: obj.dec_sign,
+ degrees: obj.dec_degrees,
+ minutes: obj.dec_minutes,
+ seconds: obj.dec_seconds
+ },
+ distance: obj.distance
+ };
+ list.push(template);
+ return '';
},
- rnd: 0,
- //https://en.wikipedia.org/wiki/Template:DEC
- dec: function dec(tmpl) {
- var obj = parse$3(tmpl, ['degrees', 'minutes', 'seconds']);
- var str = (obj.degrees || 0) + '°';
-
- if (obj.minutes) {
- str += obj.minutes + "\u2032";
- }
-
- if (obj.seconds) {
- str += obj.seconds + '″';
- }
+ // Parse https://en.wikipedia.org/wiki/Template:Medical_cases_chart -- see
+ // https://en.wikipedia.org/wiki/Module:Medical_cases_chart for the original
+ // parsing code.
+ 'medical cases chart': (tmpl, list) => {
+ let order = ['date', 'deathsExpr', 'recoveriesExpr', 'casesExpr', '4thExpr', '5thExpr', 'col1', 'col1Change', 'col2', 'col2Change'];
+ let obj = toJSON(tmpl);
+ obj.data = obj.data || '';
+ let rows = obj.data.split('\n'); // Mimic row parsing in _buildBars in the Lua source, from the following
+ // line on:
+ //
+ // for parameter in mw.text.gsplit(line, ';') do
- return str;
- },
- //https://en.wikipedia.org/wiki/Template:Val
- val: function val(tmpl) {
- var obj = parse$3(tmpl, ['number', 'uncertainty']);
- var num = obj.number;
+ let dataArray = rows.map(row => {
+ let parameters = row.split(';');
+ let rowObject = {
+ options: new Map()
+ };
+ let positionalIndex = 0;
- if (num && Number(num)) {
- num = Number(num).toLocaleString();
- }
+ for (let i = 0; i < parameters.length; i++) {
+ let parameter = parameters[i].trim();
- var str = num || ''; //prefix/suffix
+ if (parameter.match(/^[a-zA-Z_]/)) {
+ // Named argument
+ let [key, value] = parameter.split('='); // At this point, the Lua code evaluates alttot1 and alttot2 values as
+ // #expr expressions, but we just pass them through. See also:
+ // https://www.mediawiki.org/wiki/Help:Extension:ParserFunctions##expr
- if (obj.p) {
- str = obj.p + str;
- }
+ if (value === undefined) {
+ value = null;
+ }
- if (obj.s) {
- str = obj.s + str;
- } //add units, too
+ rowObject.options.set(key, value);
+ } else {
+ // Positional argument
+ // Here again, the Lua code evaluates arguments at index 1 through 5
+ // as #expr expressions, but we just pass them through.
+ if (positionalIndex < order.length) {
+ rowObject[order[positionalIndex]] = parameter;
+ }
+ positionalIndex++;
+ }
+ }
- if (obj.u || obj.ul || obj.upl) {
- str = str + ' ' + (obj.u || obj.ul || obj.upl);
- }
+ for (; positionalIndex < order.length; positionalIndex++) {
+ rowObject[order[positionalIndex]] = null;
+ }
- return str;
- },
- //https://en.wikipedia.org/wiki/Template:Sub
- sub: function sub(tmpl, list) {
- var obj = parse$3(tmpl, ['text']);
+ return rowObject;
+ });
+ obj.data = dataArray;
list.push(obj);
- return obj.text || '';
+ return '';
},
- //https://en.wikipedia.org/wiki/Template:Sup
- sup: function sup(tmpl, list) {
- var obj = parse$3(tmpl, ['text']);
- list.push(obj);
- return obj.text || '';
- }
- }; //aliases
+ graph: (tmpl, list) => {
+ let data = toJSON(tmpl);
- templates['rndfrac'] = templates.rnd;
- templates['rndnear'] = templates.rnd;
- templates['unité'] = templates.val; //templates that we simply grab their insides as plaintext
+ if (data.x) {
+ data.x = data.x.split(',').map(str => str.trim());
+ }
- var inline = ['nowrap', 'nobr', 'big', 'cquote', 'pull quote', 'small', 'smaller', 'midsize', 'larger', 'big', 'kbd', 'bigger', 'large', 'mono', 'strongbad', 'stronggood', 'huge', 'xt', 'xt2', '!xt', 'xtn', 'xtd', 'dc', 'dcr', 'mxt', '!mxt', 'mxtn', 'mxtd', 'bxt', '!bxt', 'bxtn', 'bxtd', 'delink', //https://en.wikipedia.org/wiki/Template:Delink
- //half-supported
- 'pre', 'var', 'mvar', 'pre2', 'code'];
- inline.forEach(function (k) {
- templates[k] = function (tmpl) {
- return parse$3(tmpl, ['text']).text || '';
- };
- });
- var format = templates;
+ if (data.y) {
+ data.y = data.y.split(',').map(str => str.trim());
+ }
- var tmpls = {
- //a strange, newline-based list - https://en.wikipedia.org/wiki/Template:Plainlist
- plainlist: function plainlist(tmpl) {
- tmpl = _strip(tmpl); //remove the title
+ let y = 1;
- var arr = tmpl.split('|');
- arr = arr.slice(1);
- tmpl = arr.join('|'); //split on newline
+ while (data['y' + y]) {
+ data['y' + y] = data['y' + y].split(',').map(str => str.trim());
+ y += 1;
+ }
- arr = tmpl.split(/\n ?\* ?/);
- arr = arr.filter(function (s) {
- return s;
- });
- return arr.join('\n\n');
+ list.push(data);
+ return '';
},
- //show/hide: https://en.wikipedia.org/wiki/Template:Collapsible_list
- 'collapsible list': function collapsibleList(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- var str = '';
+ //https://en.wikipedia.org/wiki/Template:Historical_populations
+ 'historical populations': (tmpl, list) => {
+ let data = toJSON(tmpl);
+ data.list = data.list || [];
+ let years = [];
- if (obj.title) {
- str += "'''".concat(obj.title, "'''") + '\n\n';
+ for (let i = 0; i < data.list.length; i += 2) {
+ let num = data.list[i + 1];
+ years.push({
+ year: data.list[i],
+ val: Number(num) || num
+ });
}
- if (!obj.list) {
- obj.list = [];
+ data.data = years;
+ delete data.list;
+ list.push(data);
+ return '';
+ },
+ // this one is a handful!
+ //https://en.wikipedia.org/wiki/Template:Weather_box
+ 'weather box': (tmpl, list) => {
+ const hasMonth = /^jan /i;
+ const isYear = /^year /i;
+ let obj = toJSON(tmpl);
+ const monthList = ['jan', 'feb', 'mar', 'apr', 'may', 'jun', 'jul', 'aug', 'sep', 'oct', 'nov', 'dec'];
+ let byMonth = {};
+ let properties = Object.keys(obj).filter(k => hasMonth.test(k));
+ properties = properties.map(k => k.replace(hasMonth, ''));
+ properties.forEach(prop => {
+ byMonth[prop] = [];
+ monthList.forEach(m => {
+ let key = `${m} ${prop}`;
- for (var i = 1; i < 10; i += 1) {
- if (obj[i]) {
- obj.list.push(obj[i]);
- delete obj[i];
+ if (obj.hasOwnProperty(key)) {
+ let num = _lib$3.toNumber(obj[key]);
+ delete obj[key];
+ byMonth[prop].push(num);
}
- }
- }
+ });
+ }); //add these to original
- obj.list = obj.list.filter(function (s) {
- return s;
- });
- str += obj.list.join('\n\n');
- return str;
- },
- // https://en.wikipedia.org/wiki/Template:Ordered_list
- 'ordered list': function orderedList(tmpl, list) {
- var obj = parse$3(tmpl);
+ obj.byMonth = byMonth; //collect year-based data
+
+ let byYear = {};
+ Object.keys(obj).forEach(k => {
+ if (isYear.test(k)) {
+ let prop = k.replace(isYear, '');
+ byYear[prop] = obj[k];
+ delete obj[k];
+ }
+ });
+ obj.byYear = byYear;
list.push(obj);
- obj.list = obj.list || [];
- var lines = obj.list.map(function (str, i) {
- return "".concat(i + 1, ". ").concat(str);
+ return '';
+ },
+ //The 36 parameters are: 12 monthly highs (C), 12 lows (total 24) plus an optional 12 monthly rain/precipitation
+ //https://en.wikipedia.org/wiki/Template:Weather_box/concise_C
+ 'weather box/concise c': (tmpl, list) => {
+ let obj = toJSON(tmpl);
+ obj.list = obj.list.map(s => _lib$3.toNumber(s));
+ obj.byMonth = {
+ 'high c': obj.list.slice(0, 12),
+ 'low c': obj.list.slice(12, 24),
+ 'rain mm': obj.list.slice(24, 36)
+ };
+ delete obj.list;
+ obj.template = 'weather box';
+ list.push(obj);
+ return '';
+ },
+ 'weather box/concise f': (tmpl, list) => {
+ let obj = toJSON(tmpl);
+ obj.list = obj.list.map(s => _lib$3.toNumber(s));
+ obj.byMonth = {
+ 'high f': obj.list.slice(0, 12),
+ 'low f': obj.list.slice(12, 24),
+ 'rain inch': obj.list.slice(24, 36)
+ };
+ delete obj.list;
+ obj.template = 'weather box';
+ list.push(obj);
+ return '';
+ },
+ //https://en.wikipedia.org/wiki/Template:Climate_chart
+ 'climate chart': (tmpl, list) => {
+ let lines = toJSON(tmpl).list || [];
+ let title = lines[0];
+ let source = lines[38];
+ lines = lines.slice(1); //amazingly, they use '−' symbol here instead of negatives...
+
+ lines = lines.map(str => {
+ if (str && str[0] === '−') {
+ str = str.replace(/−/, '-');
+ }
+
+ return str;
});
- return lines.join('\n\n');
+ let months = []; //groups of three, for 12 months
+
+ for (let i = 0; i < 36; i += 3) {
+ months.push({
+ low: _lib$3.toNumber(lines[i]),
+ high: _lib$3.toNumber(lines[i + 1]),
+ precip: _lib$3.toNumber(lines[i + 2])
+ });
+ }
+
+ let obj = {
+ template: 'climate chart',
+ data: {
+ title: title,
+ source: source,
+ months: months
+ }
+ };
+ list.push(obj);
+ return '';
+ }
+ };
+
+ let templates$4 = {
+ //https://en.wikipedia.org/wiki/Category:External_link_templates
+ 'find a grave': ['id', 'name', 'work', 'last', 'first', 'date', 'accessdate'],
+ congbio: ['id', 'name', 'date'],
+ 'hollywood walk of fame': ['name'],
+ 'wide image': ['file', 'width', 'caption'],
+ audio: ['file', 'text', 'type'],
+ rp: ['page'],
+ 'short description': ['description'],
+ 'coord missing': ['region'],
+ unreferenced: ['date'],
+ 'taxon info': ['taxon', 'item'],
+ //https://en.wikipedia.org/wiki/Template:Taxon_info
+ 'portuguese name': ['first', 'second', 'suffix'],
+ // https://en.wikipedia.org/wiki/Template:Portuguese_name
+ geo: ['lat', 'lon', 'zoom'] //https://en.wikivoyage.org/wiki/Template:Geo
+
+ };
+ templates$4 = Object.assign(templates$4, idName_1, idTitle_1, functions$1);
+ var dataOnly = templates$4;
+
+ var shorthand = {
+ mlbplayer: {
+ props: ['number', 'name', 'il'],
+ out: 'name'
},
- hlist: function hlist(tmpl) {
- var obj = parse$3(tmpl);
- obj.list = obj.list || [];
- return obj.list.join(' · ');
+ syntaxhighlight: {
+ props: [],
+ out: 'code'
},
- pagelist: function pagelist(tmpl) {
- var arr = parse$3(tmpl).list || [];
- return arr.join(', ');
+ samp: {
+ props: ['1'],
+ out: '1'
},
- //actually rendering these links removes the text.
- //https://en.wikipedia.org/wiki/Template:Catlist
- catlist: function catlist(tmpl) {
- var arr = parse$3(tmpl).list || [];
- return arr.join(', ');
+ //https://en.wikipedia.org/wiki/Template:Sub
+ sub: {
+ props: ['text'],
+ out: 'text'
},
- //https://en.wikipedia.org/wiki/Template:Br_separated_entries
- 'br separated entries': function brSeparatedEntries(tmpl) {
- var arr = parse$3(tmpl).list || [];
- return arr.join('\n\n');
+ //https://en.wikipedia.org/wiki/Template:Sup
+ sup: {
+ props: ['text'],
+ out: 'text'
},
- 'comma separated entries': function commaSeparatedEntries(tmpl) {
- var arr = parse$3(tmpl).list || [];
- return arr.join(', ');
+ //https://en.wikipedia.org/wiki/Template:Chem2
+ chem2: {
+ props: ['equation'],
+ out: 'equation'
},
- //https://en.wikipedia.org/wiki/Template:Bare_anchored_list
- 'anchored list': function anchoredList(tmpl) {
- var arr = parse$3(tmpl).list || [];
- arr = arr.map(function (str, i) {
- return "".concat(i + 1, ". ").concat(str);
- });
- return arr.join('\n\n');
+ //https://en.wikipedia.org/wiki/Template:Interlanguage_link
+ ill: {
+ props: ['text', 'lan1', 'text1', 'lan2', 'text2'],
+ out: 'text'
},
- 'bulleted list': function bulletedList(tmpl) {
- var arr = parse$3(tmpl).list || [];
- arr = arr.filter(function (f) {
- return f;
- });
- arr = arr.map(function (str) {
- return '• ' + str;
- });
- return arr.join('\n\n');
+ //https://en.wikipedia.org/wiki/Template:Abbr
+ abbr: {
+ props: ['abbr', 'meaning', 'ipa'],
+ out: 'abbr'
+ } // name: {
+ // props: [],
+ // out: '',
+ // },
+
+ };
+
+ let templates$3 = {
+ // https://en.wikipedia.org/wiki/Template:Math
+ math: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['formula']);
+ list.push(obj);
+ return '\n\n' + (obj.formula || '') + '\n\n';
},
- //https://en.wikipedia.org/wiki/Template:Columns-list
- 'columns-list': function columnsList(tmpl, list) {
- var arr = parse$3(tmpl).list || [];
- var str = arr[0] || '';
- var lines = str.split(/\n/);
- lines = lines.filter(function (f) {
- return f;
- });
- lines = lines.map(function (s) {
- return s.replace(/\*/, '');
- });
+ isbn: (tmpl, list) => {
+ let order = ['id', 'id2', 'id3'];
+ let obj = toJSON(tmpl, order);
+ list.push(obj);
+ return 'ISBN: ' + (obj.id || '');
+ },
+ //https://en.wikipedia.org/wiki/Template:Based_on
+ 'based on': (tmpl, list) => {
+ let obj = toJSON(tmpl, ['title', 'author']);
+ list.push(obj);
+ return `${obj.title} by ${obj.author || ''}`;
+ },
+ //barrels of oil https://en.wikipedia.org/wiki/Template:Bbl_to_t
+ 'bbl to t': (tmpl, list) => {
+ let obj = toJSON(tmpl, ['barrels']);
+ list.push(obj);
+
+ if (obj.barrels === '0') {
+ return obj.barrels + ' barrel';
+ }
+
+ return obj.barrels + ' barrels';
+ },
+ //minor planet - https://en.wikipedia.org/wiki/Template:MPC
+ mpc: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['number', 'text']);
+ list.push(obj);
+ return `[https://minorplanetcenter.net/db_search/show_object?object_id=P/2011+NO1 ${obj.text || obj.number}]`;
+ },
+ pengoal: (tmpl, list) => {
list.push({
- template: 'columns-list',
- list: lines
+ template: 'pengoal'
});
- lines = lines.map(function (s) {
- return '• ' + s;
+ return '✅';
+ },
+ penmiss: (tmpl, list) => {
+ list.push({
+ template: 'penmiss'
});
+ return '❌';
+ },
+ // https://en.wikipedia.org/wiki/Template:Ordered_list
+ 'ordered list': (tmpl, list) => {
+ let obj = toJSON(tmpl);
+ list.push(obj);
+ obj.list = obj.list || [];
+ let lines = obj.list.map((str, i) => `${i + 1}. ${str}`);
return lines.join('\n\n');
- } // 'pagelist':(tmpl)=>{},
-
- }; //aliases
+ },
+ // https://en.wikipedia.org/wiki/Template:Title_year
+ 'title year': (tmpl, _list, _alias, _parse, doc) => {
+ let obj = toJSON(tmpl, ['match', 'nomatch', 'page']);
+ let title = obj.page || doc.title();
- tmpls.flatlist = tmpls.plainlist;
- tmpls.ublist = tmpls.plainlist;
- tmpls['unbulleted list'] = tmpls['collapsible list'];
- tmpls['ubl'] = tmpls['collapsible list'];
- tmpls['bare anchored list'] = tmpls['anchored list'];
- tmpls['plain list'] = tmpls['plainlist'];
- tmpls.cmn = tmpls['columns-list'];
- tmpls.collist = tmpls['columns-list'];
- tmpls['col-list'] = tmpls['columns-list'];
- tmpls.columnslist = tmpls['columns-list'];
- var lists = tmpls;
-
- var inline$1 = {
- //https://en.wikipedia.org/wiki/Template:Convert#Ranges_of_values
- convert: function convert(tmpl) {
- var order = ['num', 'two', 'three', 'four'];
- var obj = parse$3(tmpl, order); //todo: support plural units
+ if (title) {
+ let m = title.match(/\b[0-9]{4}\b/); //parse the year out of the title's name
- if (obj.two === '-' || obj.two === 'to' || obj.two === 'and') {
- if (obj.four) {
- return "".concat(obj.num, " ").concat(obj.two, " ").concat(obj.three, " ").concat(obj.four);
+ if (m) {
+ return m[0];
}
-
- return "".concat(obj.num, " ").concat(obj.two, " ").concat(obj.three);
}
- return "".concat(obj.num, " ").concat(obj.two);
- },
- //https://en.wikipedia.org/wiki/Template:Term
- term: function term(tmpl) {
- var obj = parse$3(tmpl, ['term']);
- return "".concat(obj.term, ":");
- },
- defn: 0,
- //https://en.wikipedia.org/wiki/Template:Linum
- lino: 0,
- linum: function linum(tmpl) {
- var obj = parse$3(tmpl, ['num', 'text']);
- return "".concat(obj.num, ". ").concat(obj.text);
- },
- //https://en.wikipedia.org/wiki/Template:Interlanguage_link
- ill: function ill(tmpl) {
- var order = ['text', 'lan1', 'text1', 'lan2', 'text2'];
- var obj = parse$3(tmpl, order);
- return obj.text;
+ return obj.nomatch || ''; //use default response
},
- //https://en.wikipedia.org/wiki/Template:Frac
- frac: function frac(tmpl) {
- var order = ['a', 'b', 'c'];
- var obj = parse$3(tmpl, order);
+ // https://en.wikipedia.org/wiki/Template:Title_century
+ 'title century': (tmpl, _list, _alias, _parse, doc) => {
+ let obj = toJSON(tmpl, ['match', 'nomatch', 'page']);
+ let title = obj.page || doc.title();
- if (obj.c) {
- return "".concat(obj.a, " ").concat(obj.b, "/").concat(obj.c);
- }
+ if (title) {
+ let m = title.match(/\b([0-9]+)(st|nd|rd|th)\b/); //parse the century out of the title's name
- if (obj.b) {
- return "".concat(obj.a, "/").concat(obj.b);
+ if (m) {
+ return m[1] || '';
+ }
}
- return "1/".concat(obj.b);
+ return obj.nomatch || ''; //use default response
},
- //https://en.wikipedia.org/wiki/Template:Height - {{height|ft=6|in=1}}
- height: function height(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- var result = [];
- var units = ['m', 'cm', 'ft', 'in']; //order matters
+ // https://en.wikipedia.org/wiki/Template:Title_decade
+ 'title decade': (tmpl, _list, _alias, _parse, doc) => {
+ let obj = toJSON(tmpl, ['match', 'nomatch', 'page']);
+ let title = obj.page || doc.title();
- units.forEach(function (unit) {
- if (obj.hasOwnProperty(unit) === true) {
- result.push(obj[unit] + unit);
+ if (title) {
+ let m = title.match(/\b([0-9]+)s\b/); //parse the decade out of the title's name
+
+ if (m) {
+ return m[1] || '';
}
- });
- return result.join(' ');
+ }
+
+ return obj.nomatch || ''; //use default response
},
- 'block indent': function blockIndent(tmpl) {
- var obj = parse$3(tmpl);
+ //https://en.wikipedia.org/wiki/Template:Nihongo
+ nihongo: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['english', 'kanji', 'romaji', 'extra']);
+ list.push(obj);
+ let str = obj.english || obj.romaji || '';
- if (obj['1']) {
- return '\n' + obj['1'] + '\n';
+ if (obj.kanji) {
+ str += ` (${obj.kanji})`;
}
- return '';
+ return str;
},
- quote: function quote(tmpl, list) {
- var order = ['text', 'author'];
- var obj = parse$3(tmpl, order);
- list.push(obj); //create plaintext version
-
- if (obj.text) {
- var str = "\"".concat(obj.text, "\"");
+ //https://en.wikipedia.org/wiki/Template:Marriage
+ //this one creates a template, and an inline response
+ marriage: (tmpl, list) => {
+ let data = toJSON(tmpl, ['spouse', 'from', 'to', 'end']);
+ list.push(data);
+ let str = data.spouse || '';
- if (obj.author) {
- str += '\n\n';
- str += " - ".concat(obj.author);
+ if (data.from) {
+ if (data.to) {
+ str += ` (m. ${data.from}-${data.to})`;
+ } else {
+ str += ` (m. ${data.from})`;
}
-
- return str + '\n';
}
- return '';
- },
- //https://en.wikipedia.org/wiki/Template:Lbs
- lbs: function lbs(tmpl) {
- var obj = parse$3(tmpl, ['text']);
- return "[[".concat(obj.text, " Lifeboat Station|").concat(obj.text, "]]");
+ return str;
},
- //Foo-class
- lbc: function lbc(tmpl) {
- var obj = parse$3(tmpl, ['text']);
- return "[[".concat(obj.text, "-class lifeboat|").concat(obj.text, "-class]]");
+ //'red' card - {{sent off|cards|min1|min2}}
+ 'sent off': (tmpl, list) => {
+ let obj = toJSON(tmpl, ['cards']);
+ let result = {
+ template: 'sent off',
+ cards: obj.cards,
+ minutes: obj.list || []
+ };
+ list.push(result);
+ let mins = result.minutes.map(m => m + "'").join(', ');
+ return 'sent off: ' + mins;
},
- lbb: function lbb(tmpl) {
- var obj = parse$3(tmpl, ['text']);
- return "[[".concat(obj.text, "-class lifeboat|").concat(obj.text, "]]");
+ transl: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['lang', 'text', 'text2']); // support 3-param
+
+ if (obj.text2) {
+ obj.iso = obj.text;
+ obj.text = obj.text2;
+ delete obj.text2;
+ }
+
+ list.push(obj);
+ return obj.text || '';
},
- // https://en.wikipedia.org/wiki/Template:Own
- own: function own(tmpl) {
- var obj = parse$3(tmpl, ['author']);
- var str = 'Own work';
+ //show/hide: https://en.wikipedia.org/wiki/Template:Collapsible_list
+ 'collapsible list': (tmpl, list) => {
+ let obj = toJSON(tmpl);
+ list.push(obj);
+ let str = '';
- if (obj.author) {
- str += ' by ' + obj.author;
+ if (obj.title) {
+ str += `'''${obj.title}'''` + '\n\n';
+ }
+
+ if (!obj.list) {
+ obj.list = [];
+
+ for (let i = 1; i < 10; i += 1) {
+ if (obj[i]) {
+ obj.list.push(obj[i]);
+ delete obj[i];
+ }
+ }
}
+ obj.list = obj.list.filter(s => s);
+ str += obj.list.join('\n\n');
return str;
},
+ //https://en.wikipedia.org/wiki/Template:Columns-list
+ 'columns-list': (tmpl, list) => {
+ let arr = toJSON(tmpl).list || [];
+ let str = arr[0] || '';
+ let lines = str.split(/\n/).filter(f => f);
+ lines = lines.map(s => s.replace(/\*/, ''));
+ list.push({
+ template: 'columns-list',
+ list: lines
+ });
+ lines = lines.map(s => '• ' + s);
+ return lines.join('\n\n');
+ },
+ //https://en.wikipedia.org/wiki/Template:Height - {{height|ft=6|in=1}}
+ height: (tmpl, list) => {
+ let obj = toJSON(tmpl);
+ list.push(obj);
+ let result = [];
+ let units = ['m', 'cm', 'ft', 'in']; //order matters
+
+ units.forEach(unit => {
+ if (obj.hasOwnProperty(unit) === true) {
+ result.push(obj[unit] + unit);
+ }
+ });
+ return result.join(' ');
+ },
//https://en.wikipedia.org/wiki/Template:Sic
- sic: function sic(tmpl, list) {
- var obj = parse$3(tmpl, ['one', 'two', 'three']);
- var word = (obj.one || '') + (obj.two || ''); //support '[sic?]'
+ sic: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['one', 'two', 'three']);
+ let word = (obj.one || '') + (obj.two || ''); //support '[sic?]'
if (obj.one === '?') {
word = (obj.two || '') + (obj.three || '');
@@ -6080,1240 +6045,1038 @@
return word;
}
- return "".concat(word, " [sic]");
- },
- //https://www.mediawiki.org/wiki/Help:Magic_words#Formatting
- formatnum: function formatnum(tmpl) {
- tmpl = tmpl.replace(/:/, '|');
- var obj = parse$3(tmpl, ['number']);
- var str = obj.number || '';
- str = str.replace(/,/g, '');
- var num = Number(str);
- return num.toLocaleString() || '';
- },
- //https://www.mediawiki.org/wiki/Help:Magic_words#Formatting
- '#dateformat': function dateformat(tmpl) {
- tmpl = tmpl.replace(/:/, '|');
- var obj = parse$3(tmpl, ['date', 'format']);
- return obj.date;
- },
- //https://www.mediawiki.org/wiki/Help:Magic_words#Formatting
- lc: function lc(tmpl) {
- tmpl = tmpl.replace(/:/, '|');
- var obj = parse$3(tmpl, ['text']);
- return (obj.text || '').toLowerCase();
- },
- lcfirst: function lcfirst(tmpl) {
- tmpl = tmpl.replace(/:/, '|');
- var obj = parse$3(tmpl, ['text']);
- var text = obj.text;
+ return `${word} [sic]`;
+ },
+ //
+ inrconvert: (tmpl, list) => {
+ let o = toJSON(tmpl, ['rupee_value', 'currency_formatting']);
+ list.push(o);
+ const mults = {
+ k: 1000,
+ m: 1000000,
+ b: 1000000000,
+ t: 1000000000000,
+ l: 100000,
+ c: 10000000,
+ lc: 1000000000000
+ };
- if (!text) {
- return '';
+ if (o.currency_formatting) {
+ let multiplier = mults[o.currency_formatting] || 1;
+ o.rupee_value = o.rupee_value * multiplier;
}
- return text[0].toLowerCase() + text.substr(1);
+ return `inr ${o.rupee_value || ''}`;
},
- //https://www.mediawiki.org/wiki/Help:Magic_words#Formatting
- uc: function uc(tmpl) {
- tmpl = tmpl.replace(/:/, '|');
- var obj = parse$3(tmpl, ['text']);
- return (obj.text || '').toUpperCase();
+ //fraction - https://en.wikipedia.org/wiki/Template:Sfrac
+ frac: (tmpl, list) => {
+ let order = ['a', 'b', 'c'];
+ let obj = toJSON(tmpl, order);
+ let data = {
+ template: 'sfrac'
+ };
+
+ if (obj.c) {
+ data.integer = obj.a;
+ data.numerator = obj.b;
+ data.denominator = obj.c;
+ } else if (obj.b) {
+ data.numerator = obj.a;
+ data.denominator = obj.b;
+ } else {
+ data.numerator = 1;
+ data.denominator = obj.a;
+ }
+
+ list.push(data);
+
+ if (data.integer) {
+ return `${data.integer} ${data.numerator}⁄${data.denominator}`;
+ }
+
+ return `${data.numerator}⁄${data.denominator}`;
},
- ucfirst: function ucfirst(tmpl) {
- tmpl = tmpl.replace(/:/, '|');
- var obj = parse$3(tmpl, ['text']);
- var text = obj.text;
+ 'winning percentage': (tmpl, list) => {
+ let obj = toJSON(tmpl, ['wins', 'losses', 'ties']);
+ list.push(obj);
+ let wins = Number(obj.wins);
+ let losses = Number(obj.losses);
+ let ties = Number(obj.ties) || 0;
+ let games = wins + losses + ties;
- if (!text) {
+ if (obj.ignore_ties === 'y') {
+ ties = 0;
+ }
+
+ if (ties) {
+ wins += ties / 2;
+ }
+
+ let num = _lib$3.percentage({
+ numerator: wins,
+ denominator: games,
+ decimals: 1
+ });
+
+ if (num === null) {
return '';
}
- return text[0].toUpperCase() + text.substr(1);
- },
- padleft: function padleft(tmpl) {
- tmpl = tmpl.replace(/:/, '|');
- var obj = parse$3(tmpl, ['text', 'num']);
- var text = obj.text || '';
- return text.padStart(obj.num, obj.str || '0');
- },
- padright: function padright(tmpl) {
- tmpl = tmpl.replace(/:/, '|');
- var obj = parse$3(tmpl, ['text', 'num']);
- var text = obj.text || '';
- return text.padEnd(obj.num, obj.str || '0');
+ return `.${num * 10}`;
},
- //abbreviation/meaning
- //https://en.wikipedia.org/wiki/Template:Abbr
- abbr: function abbr(tmpl) {
- var obj = parse$3(tmpl, ['abbr', 'meaning', 'ipa']);
- return obj.abbr;
+ winlosspct: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['wins', 'losses']);
+ list.push(obj);
+ let wins = Number(obj.wins);
+ let losses = Number(obj.losses);
+ let num = _lib$3.percentage({
+ numerator: wins,
+ denominator: wins + losses,
+ decimals: 1
+ });
+
+ if (num === null) {
+ return '';
+ }
+
+ num = `.${num * 10}`;
+ return `${wins || 0} || ${losses || 0} || ${num || '-'}`;
},
- //https://en.wikipedia.org/wiki/Template:Abbrlink
- abbrlink: function abbrlink(tmpl) {
- var obj = parse$3(tmpl, ['abbr', 'page']);
+ //https://en.wikipedia.org/wiki/Template:Video_game_release
+ 'video game release': (tmpl, list) => {
+ let order = ['region', 'date', 'region2', 'date2', 'region3', 'date3', 'region4', 'date4'];
+ let obj = toJSON(tmpl, order);
+ let template = {
+ template: 'video game release',
+ releases: []
+ };
- if (obj.page) {
- return "[[".concat(obj.page, "|").concat(obj.abbr, "]]");
+ for (let i = 0; i < order.length; i += 2) {
+ if (obj[order[i]]) {
+ template.releases.push({
+ region: obj[order[i]],
+ date: obj[order[i + 1]]
+ });
+ }
}
- return "[[".concat(obj.abbr, "]]");
+ list.push(template);
+ let str = template.releases.map(o => `${o.region}: ${o.date || ''}`).join('\n\n');
+ return '\n' + str + '\n';
},
- //https://en.wikipedia.org/wiki/Template:Hover_title
- //technically 'h:title'
- h: 1,
- //https://en.wikipedia.org/wiki/Template:Finedetail
- finedetail: 0,
- //https://en.wikipedia.org/wiki/Template:Sort
- sort: 1
- }; //aliases
+ // https://en.m.wikipedia.org/wiki/Template:USS
+ uss: (tmpl, list) => {
+ let obj = toJSON(tmpl, ['name', 'id']);
+ list.push(obj);
- inline$1['str left'] = inline$1.trunc;
- inline$1['str crop'] = inline$1.trunc;
- inline$1['tooltip'] = inline$1.abbr;
- inline$1['abbrv'] = inline$1.abbr;
- inline$1['define'] = inline$1.abbr;
- inline$1['cvt'] = inline$1.convert;
- var misc$1 = inline$1;
-
- var formatting$1 = Object.assign({}, format, lists, misc$1);
-
- //converts DMS (decimal-minute-second) geo format to lat/lng format.
- //major thank you to https://github.com/gmaclennan/parse-dms
- //and https://github.com/WSDOT-GIS/dms-js 👏
- //accepts an array of descending Degree, Minute, Second values, with a hemisphere at the end
- //must have N/S/E/W as last thing
- function parseDms(arr) {
- var hemisphere = arr.pop();
- var degrees = Number(arr[0] || 0);
- var minutes = Number(arr[1] || 0);
- var seconds = Number(arr[2] || 0);
+ if (obj.id) {
+ return `[[USS ${obj.name} (${obj.id})|USS ''${obj.name}'' (${obj.id})]]`;
+ }
- if (typeof hemisphere !== 'string' || isNaN(degrees)) {
- return null;
+ return `[[USS ${obj.name}|USS ''${obj.name}'']]`;
}
+ };
+ var functions = templates$3;
+
+ const codes$1 = {
+ '£': 'GB£',
+ // https://en.wikipedia.org/wiki/Template:GBP
+ '¥': '¥',
+ // https://en.wikipedia.org/wiki/Template:JPY
+ '৳': '৳',
+ // https://en.wikipedia.org/wiki/Template:BDT
+ '₩': '₩',
+ // https://en.wikipedia.org/wiki/Template:SK_won
+ '€': '€',
+ // https://en.wikipedia.org/wiki/Template:€
+ '₱': '₱',
+ // https://en.wikipedia.org/wiki/Template:Philippine_peso
+ '₹': '₹',
+ // https://en.wikipedia.org/wiki/Template:Indian_Rupee
+ '₽': '₽',
+ // https://en.wikipedia.org/wiki/Template:RUB
+ 'cn¥': 'CN¥',
+ // https://en.wikipedia.org/wiki/Template:CNY
+ 'gb£': 'GB£',
+ // https://en.wikipedia.org/wiki/Template:GBP
+ 'india rs': '₹',
+ // https://en.wikipedia.org/wiki/Template:Indian_Rupee
+ 'indian rupee symbol': '₹',
+ // https://en.wikipedia.org/wiki/Template:Indian_Rupee
+ 'indian rupee': '₹',
+ // https://en.wikipedia.org/wiki/Template:Indian_Rupee
+ 'indian rupees': '₹',
+ // https://en.wikipedia.org/wiki/Template:Indian_Rupee
+ 'philippine peso': '₱',
+ // https://en.wikipedia.org/wiki/Template:Philippine_peso
+ 'russian ruble': '₽',
+ // https://en.wikipedia.org/wiki/Template:Russian_ruble
+ 'SK won': '₩',
+ // https://en.wikipedia.org/wiki/Template:SK_won
+ 'turkish lira': 'TRY',
+ //https://en.wikipedia.org/wiki/Template:Turkish_lira
+ a$: 'A$',
+ // https://en.wikipedia.org/wiki/Template:AUD
+ au$: 'A$',
+ //https://en.wikipedia.org/wiki/Template:AUD
+ aud: 'A$',
+ //https://en.wikipedia.org/wiki/Template:AUD
+ bdt: 'BDT',
+ //https://en.wikipedia.org/wiki/Template:BDT
+ brl: 'BRL',
+ //https://en.wikipedia.org/wiki/Template:BRL
+ ca$: 'CA$',
+ // https://en.wikipedia.org/wiki/Template:CAD
+ cad: 'CA$',
+ // https://en.wikipedia.org/wiki/Template:CAD
+ chf: 'CHF',
+ // https://en.wikipedia.org/wiki/Template:CHF
+ cny: 'CN¥',
+ // https://en.wikipedia.org/wiki/Template:CNY
+ czk: 'czk',
+ // https://en.wikipedia.org/wiki/Template:CZK
+ dkk: 'dkk',
+ // https://en.wikipedia.org/wiki/Template:DKK
+ dkk2: 'dkk',
+ // https://en.wikipedia.org/wiki/Template:DKK
+ euro: '€',
+ // https://en.wikipedia.org/wiki/Template:€
+ gbp: 'GB£',
+ // https://en.wikipedia.org/wiki/Template:GBP
+ hk$: 'HK$',
+ // https://en.wikipedia.org/wiki/Template:HKD
+ hkd: 'HK$',
+ // https://en.wikipedia.org/wiki/Template:HKD
+ ils: 'ILS',
+ // https://en.wikipedia.org/wiki/Template:ILS
+ inr: '₹',
+ // https://en.wikipedia.org/wiki/Template:Indian_Rupee
+ jpy: '¥',
+ // https://en.wikipedia.org/wiki/Template:JPY
+ myr: 'MYR',
+ // https://en.wikipedia.org/wiki/Template:MYR
+ nis: 'ILS',
+ // https://en.wikipedia.org/wiki/Template:ILS
+ nok: 'NOK',
+ //https://en.wikipedia.org/wiki/Template:NOK
+ nok2: 'NOK',
+ //https://en.wikipedia.org/wiki/Template:NOK
+ nz$: 'NZ$',
+ //https://en.wikipedia.org/wiki/Template:NZD
+ nzd: 'NZ$',
+ //https://en.wikipedia.org/wiki/Template:NZD
+ peso: 'peso',
+ //https://en.wikipedia.org/wiki/Template:Peso
+ pkr: '₨',
+ // https://en.wikipedia.org/wiki/Template:Pakistani_Rupee
+ r$: 'BRL',
+ //https://en.wikipedia.org/wiki/Template:BRL
+ rmb: 'CN¥',
+ // https://en.wikipedia.org/wiki/Template:CNY
+ rub: '₽',
+ // https://en.wikipedia.org/wiki/Template:RUB
+ ruble: '₽',
+ // https://en.wikipedia.org/wiki/Template:Ruble
+ rupee: '₹',
+ // https://en.wikipedia.org/wiki/Template:Rupee
+ s$: 'sgd',
+ // https://en.wikipedia.org/wiki/Template:SGD
+ sek: 'SEK',
+ // https://en.wikipedia.org/wiki/Template:SEK
+ sek2: 'SEK',
+ // https://en.wikipedia.org/wiki/Template:SEK
+ sfr: 'CHF',
+ // https://en.wikipedia.org/wiki/Template:CHF
+ sgd: 'sgd',
+ // https://en.wikipedia.org/wiki/Template:SGD
+ shekel: 'ILS',
+ // https://en.wikipedia.org/wiki/Template:ILS
+ sheqel: 'ILS',
+ // https://en.wikipedia.org/wiki/Template:ILS
+ ttd: 'TTD',
+ //https://en.wikipedia.org/wiki/Template:TTD
+ us$: 'US$',
+ // https://en.wikipedia.org/wiki/Template:US$
+ usd: 'US$',
+ // https://en.wikipedia.org/wiki/Template:US$
+ yen: '¥',
+ // https://en.wikipedia.org/wiki/Template:JPY
+ zar: 'R' //https://en.wikipedia.org/wiki/Template:ZAR
- var sign = 1;
+ };
- if (/[SW]/i.test(hemisphere)) {
- sign = -1;
- }
+ const parseCurrency = (tmpl, list) => {
+ let o = toJSON(tmpl, ['amount', 'code']);
+ list.push(o);
+ let code = o.template || '';
- var decDeg = sign * (degrees + minutes / 60 + seconds / 3600);
- return decDeg;
- }
+ if (code === 'currency') {
+ code = o.code;
+
+ if (!code) {
+ o.code = code = 'usd'; //Special case when currency template has no code argument
+ }
+ } else if (code === '' || code === 'monnaie' || code === 'unité' || code === 'nombre' || code === 'nb') {
+ code = o.code;
+ }
- var dmsFormat = parseDms; // console.log(parseDms([57, 18, 22, 'N']));
+ code = (code || '').toLowerCase();
- var round = function round(num) {
- if (typeof num !== 'number') {
- return num;
+ if (code === 'us') {
+ o.code = code = 'usd';
+ } else if (code === 'uk') {
+ o.code = code = 'gbp';
}
- var places = 100000;
- return Math.round(num * places) / places;
- }; //these hemispheres mean negative decimals
+ let str = `${codes$1[code] || ''}${o.amount || ''}`; //support unknown currencies after the number - like '5 BTC'
+ if (o.code && !codes$1[o.code.toLowerCase()]) {
+ str += ' ' + o.code;
+ }
- var negative = {
- s: true,
- w: true
+ return str;
};
- var findLatLng = function findLatLng(arr) {
- var types = arr.map(function (s) {
- return _typeof(s);
- }).join('|'); //support {{lat|lng}}
+ let templates$2 = {
+ currency: parseCurrency
+ }; //and the others fit the same pattern
- if (arr.length === 2 && types === 'number|number') {
- return {
- lat: arr[0],
- lon: arr[1]
- };
- } //support {{dd|N/S|dd|E/W}}
+ Object.keys(codes$1).forEach(k => {
+ templates$2[k] = parseCurrency;
+ });
+ var currency = templates$2;
+ const toOrdinal = function (i) {
+ let j = i % 10;
+ let k = i % 100;
- if (arr.length === 4 && types === 'number|string|number|string') {
- if (negative[arr[1].toLowerCase()]) {
- arr[0] *= -1;
- }
+ if (j === 1 && k !== 11) {
+ return i + 'st';
+ }
- if (arr[3].toLowerCase() === 'w') {
- arr[2] *= -1;
- }
+ if (j === 2 && k !== 12) {
+ return i + 'nd';
+ }
- return {
- lat: arr[0],
- lon: arr[2]
- };
- } //support {{dd|mm|N/S|dd|mm|E/W}}
+ if (j === 3 && k !== 13) {
+ return i + 'rd';
+ }
+ return i + 'th';
+ }; //this is allowed to be rough
- if (arr.length === 6) {
- return {
- lat: dmsFormat(arr.slice(0, 3)),
- lon: dmsFormat(arr.slice(3))
- };
- } //support {{dd|mm|ss|N/S|dd|mm|ss|E/W}}
+ const day = 1000 * 60 * 60 * 24;
+ const month = day * 30;
+ const year = day * 365;
- if (arr.length === 8) {
- return {
- lat: dmsFormat(arr.slice(0, 4)),
- lon: dmsFormat(arr.slice(4))
- };
- }
-
- return {};
- };
+ const getEpoch = function (obj) {
+ return new Date(`${obj.year}-${obj.month || 0}-${obj.date || 1}`).getTime();
+ }; //very rough!
- var parseParams = function parseParams(obj) {
- obj.list = obj.list || [];
- obj.list = obj.list.map(function (str) {
- var num = Number(str);
- if (!isNaN(num)) {
- return num;
- } //these are weird
+ const delta$1 = function (from, to) {
+ from = getEpoch(from);
+ to = getEpoch(to);
+ let diff = to - from;
+ let obj = {}; //get years
+ let years = Math.floor(diff / year, 10);
- var split = str.split(/:/);
+ if (years > 0) {
+ obj.years = years;
+ diff -= obj.years * year;
+ } //get months
- if (split.length > 1) {
- obj.props = obj.props || {};
- obj.props[split[0]] = split.slice(1).join(':');
- return null;
- }
- return str;
- });
- obj.list = obj.list.filter(function (s) {
- return s !== null;
- });
- return obj;
- };
+ let monthCount = Math.floor(diff / month, 10);
- var parseCoor = function parseCoor(tmpl) {
- var obj = parse$3(tmpl);
- obj = parseParams(obj);
- var tmp = findLatLng(obj.list);
- obj.lat = round(tmp.lat);
- obj.lon = round(tmp.lon);
- obj.template = 'coord';
- delete obj.list;
- return obj;
- };
+ if (monthCount > 0) {
+ obj.months = monthCount;
+ diff -= obj.months * month;
+ } //get days
- var coor = parseCoor; // {{Coor title dms|dd|mm|ss|N/S|dd|mm|ss|E/W|template parameters}}
- var templates$1 = {
- coord: function coord(tmpl, list) {
- var obj = coor(tmpl);
- list.push(obj); //display inline, by default
+ let days = Math.floor(diff / day, 10);
- if (!obj.display || obj.display.indexOf('inline') !== -1) {
- return "".concat(obj.lat || '', "\xB0N, ").concat(obj.lon || '', "\xB0W");
- }
+ if (days > 0) {
+ obj.days = days; // diff -= (obj.days * day);
+ }
- return '';
- },
- //https://en.wikivoyage.org/wiki/Template:Geo
- geo: ['lat', 'lon', 'zoom']
- }; // {{coord|latitude|longitude|coordinate parameters|template parameters}}
- // {{coord|dd|N/S|dd|E/W|coordinate parameters|template parameters}}
- // {{coord|dd|mm|N/S|dd|mm|E/W|coordinate parameters|template parameters}}
- // {{coord|dd|mm|ss|N/S|dd|mm|ss|E/W|coordinate parameters|template parameters}}
+ return obj;
+ }; //not all too fancy - used in {{timesince}}
- templates$1['coor'] = templates$1.coord; // these are from the nl wiki
- templates$1['coor title dms'] = templates$1.coord;
- templates$1['coor title dec'] = templates$1.coord;
- templates$1['coor dms'] = templates$1.coord;
- templates$1['coor dm'] = templates$1.coord;
- templates$1['coor dec'] = templates$1.coord;
- var geo = templates$1;
+ const timeSince = function (str) {
+ let d = new Date(str);
- var templates$2 = {
- /* mostly wiktionary*/
- etyl: 1,
- mention: 1,
- link: 1,
- 'la-verb-form': 0,
- 'la-ipa': 0,
- //https://en.wikipedia.org/wiki/Template:Sortname
- sortname: function sortname(tmpl) {
- var order = ['first', 'last', 'target', 'sort'];
- var obj = parse$3(tmpl, order);
- var name = "".concat(obj.first || '', " ").concat(obj.last || '');
- name = name.trim();
+ if (isNaN(d.getTime())) {
+ return '';
+ }
- if (obj.nolink) {
- return obj.target || name;
- }
+ let now = new Date();
+ let delt = now.getTime() - d.getTime();
+ let predicate = 'ago';
- if (obj.dab) {
- name += " (".concat(obj.dab, ")");
+ if (delt < 0) {
+ predicate = 'from now';
+ delt = Math.abs(delt);
+ } //figure out units
- if (obj.target) {
- obj.target += " (".concat(obj.dab, ")");
- }
- }
- if (obj.target) {
- return "[[".concat(obj.target, "|").concat(name, "]]");
- }
+ let hours = delt / 1000 / 60 / 60;
+ let days = hours / 24;
- return "[[".concat(name, "]]");
+ if (days < 365) {
+ return parseInt(days, 10) + ' days ' + predicate;
}
- }; //these are insane
- // https://en.wikipedia.org/wiki/Template:Tl
- var links = ['lts', 't', 'tfd links', 'tiw', 'tltt', 'tetl', 'tsetl', 'ti', 'tic', 'tiw', 'tlt', 'ttl', 'twlh', 'tl2', 'tlu', 'demo', 'hatnote', 'xpd', 'para', 'elc', 'xtag', 'mli', 'mlix', '#invoke', 'url' //https://en.wikipedia.org/wiki/Template:URL
- ]; //keyValues
+ let years = days / 365;
+ return parseInt(years, 10) + ' years ' + predicate;
+ };
+
+ const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'];
+ var _lib$2 = {
+ days: days,
+ timeSince: timeSince,
+ delta: delta$1,
+ toOrdinal: toOrdinal
+ };
- links.forEach(function (k) {
- templates$2[k] = function (tmpl) {
- var order = ['first', 'second'];
- var obj = parse$3(tmpl, order);
- return obj.second || obj.first;
- };
- }); //aliases
+ //assorted parsing methods for date/time templates
+ const months$1 = [undefined, //1-based months.. :/
+ 'January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'];
+ const monthName = months$1.reduce((h, str, i) => {
+ if (i === 0) {
+ return h;
+ }
- templates$2.m = templates$2.mention;
- templates$2['m-self'] = templates$2.mention;
- templates$2.l = templates$2.link;
- templates$2.ll = templates$2.link;
- templates$2['l-self'] = templates$2.link;
- var links_1 = templates$2;
+ h[str.toLowerCase()] = i;
+ return h;
+ }, {}); //parse year|month|date numbers
- var sisterProjects = {
- wikt: 'wiktionary',
- commons: 'commons',
- c: 'commons',
- commonscat: 'commonscat',
- n: 'wikinews',
- q: 'wikiquote',
- s: 'wikisource',
- a: 'wikiauthor',
- b: 'wikibooks',
- voy: 'wikivoyage',
- v: 'wikiversity',
- d: 'wikidata',
- species: 'wikispecies',
- m: 'meta',
- mw: 'mediawiki'
- };
- var parsers$1 = {
- // https://en.wikipedia.org/wiki/Template:About
- about: function about(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- return '';
- },
- // https://en.wikipedia.org/wiki/Template:Main
- main: function main(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- return '';
- },
- // https://en.wikipedia.org/wiki/Template:Main_list
- 'main list': function mainList(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- return '';
- },
- // https://en.wikipedia.org/wiki/Template:See
- see: function see(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- return '';
- },
- // https://en.wikipedia.org/wiki/Template:For
- "for": function _for(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- return '';
- },
- // https://en.wikipedia.org/wiki/Template:Further
- further: function further(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- return '';
- },
- // same as "further" (but this name is still in use)
- 'further information': function furtherInformation(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- return '';
- },
- // https://en.wikipedia.org/wiki/Template:Listen
- listen: function listen(tmpl, list) {
- var obj = parse$3(tmpl);
- list.push(obj);
- return '';
- },
- 'wide image': ['file', 'width', 'caption'],
- //https://en.wikipedia.org/wiki/Template:Redirect
- redirect: function redirect(tmpl, list) {
- var data = parse$3(tmpl, ['redirect']);
- var lines = data.list || [];
- var links = [];
+ const ymd = function (arr) {
+ let obj = {};
+ let units = ['year', 'month', 'date', 'hour', 'minute', 'second']; //parse each unit in sequence..
- for (var i = 0; i < lines.length; i += 2) {
- links.push({
- page: lines[i + 1],
- desc: lines[i]
- });
+ for (let i = 0; i < units.length; i += 1) {
+ //skip it
+ if (!arr[i] && arr[1] !== 0) {
+ continue;
}
- var obj = {
- template: 'redirect',
- redirect: data.redirect,
- links: links
- };
- list.push(obj);
- return '';
- },
- //this one sucks - https://en.wikipedia.org/wiki/Template:GNIS
- 'cite gnis': function citeGnis(tmpl, list) {
- var order = ['id', 'name', 'type'];
- var obj = parse$3(tmpl, order);
- obj.type = 'gnis';
- obj.template = 'citation';
- list.push(obj);
- return '';
- },
- //https://en.wikipedia.org/wiki/Template:Sfn
- sfn: function sfn(tmpl, list, alias) {
- var order = ['author', 'year', 'location'];
- var obj = parse$3(tmpl, order);
+ let num = parseInt(arr[i], 10);
- if (alias) {
- obj.name = obj.template;
- obj.teplate = alias;
+ if (isNaN(num) === false) {
+ obj[units[i]] = num; //we good.
+ } else if (units[i] === 'month' && monthName.hasOwnProperty(arr[i])) {
+ //try for month-name, like 'january
+ let month = monthName[arr[i]];
+ obj[units[i]] = month;
+ } else {
+ //we dead. so skip this unit
+ delete obj[units[i]];
}
+ } //try for timezone,too ftw
- list.push(obj);
- return '';
- },
- audio: ['file', 'text', 'type'],
- rp: ['page'],
- 'spoken wikipedia': function spokenWikipedia(tmpl, list) {
- var order = ['file', 'date'];
- var obj = parse$3(tmpl, order);
- obj.template = 'audio';
- list.push(obj);
- return '';
- },
- //https://en.wikipedia.org/wiki/Template:Sister_project_links
- 'sister project links': function sisterProjectLinks(tmpl, list) {
- var data = parse$3(tmpl); //rename 'wd' to 'wikidata'
-
- var links = {};
- Object.keys(sisterProjects).forEach(function (k) {
- if (data.hasOwnProperty(k) === true) {
- links[sisterProjects[k]] = data[k]; //.text();
- }
- });
- var obj = {
- template: 'sister project links',
- links: links
- };
- list.push(obj);
- return '';
- },
- //https://en.wikipedia.org/wiki/Template:Subject_bar
- 'subject bar': function subjectBar(tmpl, list) {
- var data = parse$3(tmpl);
- Object.keys(data).forEach(function (k) {
- //rename 'voy' to 'wikivoyage'
- if (sisterProjects.hasOwnProperty(k)) {
- data[sisterProjects[k]] = data[k];
- delete data[k];
- }
- });
- var obj = {
- template: 'subject bar',
- links: data
- };
- list.push(obj);
- return '';
- },
- 'short description': ['description'],
- 'coord missing': ['region'],
- //amazingly, this one does not obey any known patterns
- //https://en.wikipedia.org/wiki/Template:Gallery
- gallery: function gallery(tmpl, list) {
- var obj = parse$3(tmpl);
- var images = (obj.list || []).filter(function (line) {
- return /^ *File ?:/.test(line);
- });
- images = images.map(function (file) {
- var img = {
- file: file
- }; // TODO: add lang and domain information
- return new Image_1(img).json();
- });
- obj = {
- template: 'gallery',
- images: images
- };
- list.push(obj);
- return '';
- },
- //https://en.wikipedia.org/wiki/Template:See_also
- 'see also': function seeAlso(tmpl, list) {
- var data = parse$3(tmpl);
- list.push(data);
- return '';
- },
- unreferenced: ['date']
- }; //aliases
+ let last = arr[arr.length - 1] || '';
+ last = String(last);
- parsers$1['cite'] = parsers$1.citation;
- parsers$1['sfnref'] = parsers$1.sfn;
- parsers$1['harvid'] = parsers$1.sfn;
- parsers$1['harvnb'] = parsers$1.sfn;
- parsers$1['unreferenced section'] = parsers$1.unreferenced;
- parsers$1['redir'] = parsers$1.redirect;
- parsers$1['sisterlinks'] = parsers$1['sister project links'];
- parsers$1['main article'] = parsers$1['main'];
- var page = parsers$1;
-
- var titlecase = function titlecase(str) {
- return str.charAt(0).toUpperCase() + str.substring(1);
- }; //https://en.wikipedia.org/wiki/Template:Yes
+ if (last.toLowerCase() === 'z') {
+ obj.tz = 'UTC';
+ } else if (/[+-][0-9]+:[0-9]/.test(last)) {
+ obj.tz = arr[6];
+ }
+ return obj;
+ }; //zero-pad a number
- var templates$3 = {};
- var cells = ['rh', 'rh2', 'yes', 'no', 'maybe', 'eliminated', 'lost', 'safe', 'active', 'site active', 'coming soon', 'good', 'won', 'nom', 'sho', 'longlisted', 'tba', 'success', 'operational', 'failure', 'partial', 'regional', 'maybecheck', 'partial success', 'partial failure', 'okay', 'yes-no', 'some', 'nonpartisan', 'pending', 'unofficial', 'unofficial2', 'usually', 'rarely', 'sometimes', 'any', 'varies', 'black', 'non-album single', 'unreleased', 'unknown', 'perhaps', 'depends', 'included', 'dropped', 'terminated', 'beta', 'table-experimental', 'free', 'proprietary', 'nonfree', 'needs', 'nightly', 'release-candidate', 'planned', 'scheduled', 'incorrect', 'no result', 'cmain', 'calso starring', 'crecurring', 'cguest', 'not yet', 'optional'];
- cells.forEach(function (str) {
- templates$3[str] = function (tmpl) {
- var data = parse$3(tmpl, ['text']);
- return data.text || titlecase(data.template);
- };
- }); //these ones have a text result
- var moreCells = [['active fire', 'Active'], ['site active', 'Active'], ['site inactive', 'Inactive'], ['yes2', ''], ['no2', ''], ['ya', '✅'], ['na', '❌'], ['nom', 'Nominated'], ['sho', 'Shortlisted'], ['tba', 'TBA'], ['maybecheck', '✔️'], ['okay', 'Neutral'], ['n/a', 'N/A'], ['sdash', '—'], ['dunno', '?'], ['draw', ''], ['cnone', ''], ['nocontest', '']];
- moreCells.forEach(function (a) {
- templates$3[a[0]] = function (tmpl) {
- var data = parse$3(tmpl, ['text']);
- return data.text || a[1];
- };
- }); //this one's a little different
+ const pad = function (num) {
+ if (num < 10) {
+ return '0' + num;
+ }
- templates$3.won = function (tmpl) {
- var data = parse$3(tmpl, ['text']);
- return data.place || data.text || titlecase(data.template);
+ return String(num);
};
- var tableCell = templates$3;
+ const toText$1 = function (date) {
+ //eg '1995'
+ let str = String(date.year || '');
+
+ if (date.month !== undefined && months$1.hasOwnProperty(date.month) === true) {
+ if (date.date === undefined) {
+ //January 1995
+ str = `${months$1[date.month]} ${date.year}`;
+ } else {
+ //January 5, 1995
+ str = `${months$1[date.month]} ${date.date}, ${date.year}`; //add times, if available
+
+ if (date.hour !== undefined && date.minute !== undefined) {
+ let time = `${pad(date.hour)}:${pad(date.minute)}`;
- var wikipedia = Object.assign({}, links_1, page, tableCell);
+ if (date.second !== undefined) {
+ time = time + ':' + pad(date.second);
+ }
- var zeroPad = function zeroPad(num) {
- num = String(num);
+ str = time + ', ' + str; //add timezone, if there, at the end in brackets
+ }
- if (num.length === 1) {
- num = '0' + num;
+ if (date.tz) {
+ str += ` (${date.tz})`;
+ }
+ }
}
- return num;
+ return str;
};
- var parseTeam = function parseTeam(obj, round, team) {
- if (obj["rd".concat(round, "-team").concat(zeroPad(team))]) {
- team = zeroPad(team);
- }
-
- var score = obj["rd".concat(round, "-score").concat(team)];
- var num = Number(score);
+ var _format = {
+ toText: toText$1,
+ ymd: ymd
+ }; // console.log(toText(ymd([2018, 3, 28])));
- if (isNaN(num) === false) {
- score = num;
- }
+ const delta = _lib$2.delta; //wrap it up as a template
+ const template$1 = function (date) {
return {
- team: obj["rd".concat(round, "-team").concat(team)],
- score: score,
- seed: obj["rd".concat(round, "-seed").concat(team)]
+ template: 'date',
+ data: date
};
- }; //these are weird.
-
+ };
- var playoffBracket = function playoffBracket(tmpl) {
- var rounds = [];
- var obj = parse$3(tmpl); //try some rounds
+ const getBoth = function (tmpl) {
+ tmpl = _strip(tmpl);
+ let arr = tmpl.split('|');
+ let from = _format.ymd(arr.slice(1, 4));
+ let to = arr.slice(4, 7); //assume now, if 'to' is empty
- for (var i = 1; i < 7; i += 1) {
- var round = [];
-
- for (var t = 1; t < 16; t += 2) {
- var key = "rd".concat(i, "-team");
-
- if (obj[key + t] || obj[key + zeroPad(t)]) {
- var one = parseTeam(obj, i, t);
- var two = parseTeam(obj, i, t + 1);
- round.push([one, two]);
- } else {
- break;
- }
- }
-
- if (round.length > 0) {
- rounds.push(round);
- }
+ if (to.length === 0) {
+ let d = new Date();
+ to = [d.getFullYear(), d.getMonth(), d.getDate()];
}
+ to = _format.ymd(to);
return {
- template: 'playoffbracket',
- rounds: rounds
+ from: from,
+ to: to
};
};
- var all = {
- //playoff brackets
- '4teambracket': function teambracket(tmpl, list) {
- var obj = playoffBracket(tmpl);
- list.push(obj);
- return '';
- }
- }; //a bunch of aliases for these ones:
- // https://en.wikipedia.org/wiki/Category:Tournament_bracket_templates
-
- var brackets = ['2teambracket', '4team2elimbracket', '8teambracket', '16teambracket', '32teambracket', '4roundbracket-byes', 'cwsbracket', 'nhlbracket', 'nhlbracket-reseed', '4teambracket-nhl', '4teambracket-ncaa', '4teambracket-mma', '4teambracket-mlb', '16teambracket-two-reseeds', '8teambracket-nhl', '8teambracket-mlb', '8teambracket-ncaa', '8teambracket-afc', '8teambracket-afl', '8teambracket-tennis3', '8teambracket-tennis5', '16teambracket-nhl', '16teambracket-nhl divisional', '16teambracket-nhl-reseed', '16teambracket-nba', '16teambracket-swtc', '16teambracket-afc', '16teambracket-tennis3', '16teambracket-tennis5'];
- brackets.forEach(function (key) {
- all[key] = all['4teambracket'];
- });
- var brackets_1 = all;
-
- var codes = {
- '£': 'GB£',
- // https://en.wikipedia.org/wiki/Template:GBP
- '¥': '¥',
- // https://en.wikipedia.org/wiki/Template:JPY
- '৳': '৳',
- // https://en.wikipedia.org/wiki/Template:BDT
- '₩': '₩',
- // https://en.wikipedia.org/wiki/Template:SK_won
- '€': '€',
- // https://en.wikipedia.org/wiki/Template:€
- '₱': '₱',
- // https://en.wikipedia.org/wiki/Template:Philippine_peso
- '₹': '₹',
- // https://en.wikipedia.org/wiki/Template:Indian_Rupee
- '₽': '₽',
- // https://en.wikipedia.org/wiki/Template:RUB
- 'cn¥': 'CN¥',
- // https://en.wikipedia.org/wiki/Template:CNY
- 'gb£': 'GB£',
- // https://en.wikipedia.org/wiki/Template:GBP
- 'india rs': '₹',
- // https://en.wikipedia.org/wiki/Template:Indian_Rupee
- 'indian rupee symbol': '₹',
- // https://en.wikipedia.org/wiki/Template:Indian_Rupee
- 'indian rupee': '₹',
- // https://en.wikipedia.org/wiki/Template:Indian_Rupee
- 'indian rupees': '₹',
- // https://en.wikipedia.org/wiki/Template:Indian_Rupee
- 'philippine peso': '₱',
- // https://en.wikipedia.org/wiki/Template:Philippine_peso
- 'russian ruble': '₽',
- // https://en.wikipedia.org/wiki/Template:Russian_ruble
- 'SK won': '₩',
- // https://en.wikipedia.org/wiki/Template:SK_won
- 'turkish lira': 'TRY',
- //https://en.wikipedia.org/wiki/Template:Turkish_lira
- a$: 'A$',
- // https://en.wikipedia.org/wiki/Template:AUD
- au$: 'A$',
- //https://en.wikipedia.org/wiki/Template:AUD
- aud: 'A$',
- //https://en.wikipedia.org/wiki/Template:AUD
- bdt: 'BDT',
- //https://en.wikipedia.org/wiki/Template:BDT
- brl: 'BRL',
- //https://en.wikipedia.org/wiki/Template:BRL
- ca$: 'CA$',
- // https://en.wikipedia.org/wiki/Template:CAD
- cad: 'CA$',
- // https://en.wikipedia.org/wiki/Template:CAD
- chf: 'CHF',
- // https://en.wikipedia.org/wiki/Template:CHF
- cny: 'CN¥',
- // https://en.wikipedia.org/wiki/Template:CNY
- czk: 'czk',
- // https://en.wikipedia.org/wiki/Template:CZK
- dkk: 'dkk',
- // https://en.wikipedia.org/wiki/Template:DKK
- dkk2: 'dkk',
- // https://en.wikipedia.org/wiki/Template:DKK
- euro: '€',
- // https://en.wikipedia.org/wiki/Template:€
- gbp: 'GB£',
- // https://en.wikipedia.org/wiki/Template:GBP
- hk$: 'HK$',
- // https://en.wikipedia.org/wiki/Template:HKD
- hkd: 'HK$',
- // https://en.wikipedia.org/wiki/Template:HKD
- ils: 'ILS',
- // https://en.wikipedia.org/wiki/Template:ILS
- inr: '₹',
- // https://en.wikipedia.org/wiki/Template:Indian_Rupee
- jpy: '¥',
- // https://en.wikipedia.org/wiki/Template:JPY
- myr: 'MYR',
- // https://en.wikipedia.org/wiki/Template:MYR
- nis: 'ILS',
- // https://en.wikipedia.org/wiki/Template:ILS
- nok: 'NOK',
- //https://en.wikipedia.org/wiki/Template:NOK
- nok2: 'NOK',
- //https://en.wikipedia.org/wiki/Template:NOK
- nz$: 'NZ$',
- //https://en.wikipedia.org/wiki/Template:NZD
- nzd: 'NZ$',
- //https://en.wikipedia.org/wiki/Template:NZD
- peso: 'peso',
- //https://en.wikipedia.org/wiki/Template:Peso
- pkr: '₨',
- // https://en.wikipedia.org/wiki/Template:Pakistani_Rupee
- r$: 'BRL',
- //https://en.wikipedia.org/wiki/Template:BRL
- rmb: 'CN¥',
- // https://en.wikipedia.org/wiki/Template:CNY
- rub: '₽',
- // https://en.wikipedia.org/wiki/Template:RUB
- ruble: '₽',
- // https://en.wikipedia.org/wiki/Template:Ruble
- rupee: '₹',
- // https://en.wikipedia.org/wiki/Template:Rupee
- s$: 'sgd',
- // https://en.wikipedia.org/wiki/Template:SGD
- sek: 'SEK',
- // https://en.wikipedia.org/wiki/Template:SEK
- sek2: 'SEK',
- // https://en.wikipedia.org/wiki/Template:SEK
- sfr: 'CHF',
- // https://en.wikipedia.org/wiki/Template:CHF
- sgd: 'sgd',
- // https://en.wikipedia.org/wiki/Template:SGD
- shekel: 'ILS',
- // https://en.wikipedia.org/wiki/Template:ILS
- sheqel: 'ILS',
- // https://en.wikipedia.org/wiki/Template:ILS
- ttd: 'TTD',
- //https://en.wikipedia.org/wiki/Template:TTD
- us$: 'US$',
- // https://en.wikipedia.org/wiki/Template:US$
- usd: 'US$',
- // https://en.wikipedia.org/wiki/Template:US$
- yen: '¥',
- // https://en.wikipedia.org/wiki/Template:JPY
- zar: 'R' //https://en.wikipedia.org/wiki/Template:ZAR
+ const parsers = {
+ //generic {{date|year|month|date}} template
+ date: (tmpl, list) => {
+ let order = ['year', 'month', 'date', 'hour', 'minute', 'second', 'timezone'];
+ let obj = toJSON(tmpl, order);
+ let data = _format.ymd([obj.year, obj.month, obj.date || obj.day]);
+ obj.text = _format.toText(data); //make the replacement string
- };
+ if (obj.timezone) {
+ if (obj.timezone === 'Z') {
+ obj.timezone = 'UTC';
+ }
- var parseCurrency = function parseCurrency(tmpl, list) {
- var o = parse$3(tmpl, ['amount', 'code']);
- list.push(o);
- var code = o.template || '';
+ obj.text += ` (${obj.timezone})`;
+ }
- if (code === 'currency') {
- code = o.code;
+ if (obj.hour && obj.minute) {
+ if (obj.second) {
+ obj.text = `${obj.hour}:${obj.minute}:${obj.second}, ` + obj.text;
+ } else {
+ obj.text = `${obj.hour}:${obj.minute}, ` + obj.text;
+ }
+ }
- if (!code) {
- o.code = code = 'usd'; //Special case when currency template has no code argument
+ if (obj.text) {
+ list.push(template$1(obj));
}
- } else if (code === '' || code === 'monnaie' || code === 'unité' || code === 'nombre' || code === 'nb') {
- code = o.code;
- }
- code = (code || '').toLowerCase();
+ return obj.text;
+ },
+ //support parsing of 'February 10, 1992'
+ natural_date: (tmpl, list) => {
+ let order = ['text'];
+ let obj = toJSON(tmpl, order);
+ let str = obj.text || ''; // - just a year
- switch (code) {
- case 'us':
- o.code = code = 'usd';
- break;
+ let date = {};
- case 'uk':
- o.code = code = 'gbp';
- break;
- }
+ if (/^[0-9]{4}$/.test(str)) {
+ date.year = parseInt(str, 10);
+ } else {
+ //parse the date, using the js date object (for now?)
+ let txt = str.replace(/[a-z]+\/[a-z]+/i, '');
+ txt = txt.replace(/[0-9]+:[0-9]+(am|pm)?/i, '');
+ let d = new Date(txt);
- var out = codes[code] || '';
- var str = "".concat(out).concat(o.amount || ''); //support unknown currencies after the number - like '5 BTC'
+ if (isNaN(d.getTime()) === false) {
+ date.year = d.getFullYear();
+ date.month = d.getMonth() + 1;
+ date.date = d.getDate();
+ }
+ }
- if (o.code && !codes[o.code.toLowerCase()]) {
- str += ' ' + o.code;
- }
+ list.push(template$1(date));
+ return str.trim();
+ },
+ //just grab the first value, and assume it's a year
+ one_year: (tmpl, list) => {
+ let order = ['year'];
+ let obj = toJSON(tmpl, order);
+ let year = Number(obj.year);
+ list.push(template$1({
+ year: year
+ }));
+ return String(year);
+ },
+ //assume 'y|m|d' | 'y|m|d' // {{BirthDeathAge|B|1976|6|6|1990|8|8}}
+ two_dates: (tmpl, list) => {
+ let order = ['b', 'birth_year', 'birth_month', 'birth_date', 'death_year', 'death_month', 'death_date'];
+ let obj = toJSON(tmpl, order); //'b' means show birth-date, otherwise show death-date
- return str;
- };
+ if (obj.b && obj.b.toLowerCase() === 'b') {
+ let date = _format.ymd([obj.birth_year, obj.birth_month, obj.birth_date]);
+ list.push(template$1(date));
+ return _format.toText(date);
+ }
- var inrConvert = function inrConvert(tmpl, list) {
- var o = parse$3(tmpl, ['rupee_value', 'currency_formatting']);
- list.push(o);
- var formatting = o.currency_formatting;
+ let date = _format.ymd([obj.death_year, obj.death_month, obj.death_date]);
+ list.push(template$1(date));
+ return _format.toText(date);
+ },
+ age: tmpl => {
+ let d = getBoth(tmpl);
+ let diff = delta(d.from, d.to);
+ return diff.years || 0;
+ },
+ 'diff-y': tmpl => {
+ let d = getBoth(tmpl);
+ let diff = delta(d.from, d.to);
- if (formatting) {
- var multiplier = 1;
+ if (diff.years === 1) {
+ return diff.years + ' year';
+ }
- switch (formatting) {
- case 'k':
- multiplier = 1000;
- break;
+ return (diff.years || 0) + ' years';
+ },
+ 'diff-ym': tmpl => {
+ let d = getBoth(tmpl);
+ let diff = delta(d.from, d.to);
+ let arr = [];
- case 'm':
- multiplier = 1000000;
- break;
+ if (diff.years === 1) {
+ arr.push(diff.years + ' year');
+ } else if (diff.years && diff.years !== 0) {
+ arr.push(diff.years + ' years');
+ }
- case 'b':
- multiplier = 1000000000;
- break;
+ if (diff.months === 1) {
+ arr.push('1 month');
+ } else if (diff.months && diff.months !== 0) {
+ arr.push(diff.months + ' months');
+ }
- case 't':
- multiplier = 1000000000000;
- break;
+ return arr.join(', ');
+ },
+ 'diff-ymd': tmpl => {
+ let d = getBoth(tmpl);
+ let diff = delta(d.from, d.to);
+ let arr = [];
- case 'l':
- multiplier = 100000;
- break;
+ if (diff.years === 1) {
+ arr.push(diff.years + ' year');
+ } else if (diff.years && diff.years !== 0) {
+ arr.push(diff.years + ' years');
+ }
- case 'c':
- multiplier = 10000000;
- break;
+ if (diff.months === 1) {
+ arr.push('1 month');
+ } else if (diff.months && diff.months !== 0) {
+ arr.push(diff.months + ' months');
+ }
- case 'lc':
- multiplier = 1000000000000;
- break;
+ if (diff.days === 1) {
+ arr.push('1 day');
+ } else if (diff.days && diff.days !== 0) {
+ arr.push(diff.days + ' days');
}
- o.rupee_value = o.rupee_value * multiplier;
- }
+ return arr.join(', ');
+ },
+ 'diff-yd': tmpl => {
+ let d = getBoth(tmpl);
+ let diff = delta(d.from, d.to);
+ let arr = [];
- var str = "inr ".concat(o.rupee_value || '');
- return str;
- };
+ if (diff.years === 1) {
+ arr.push(diff.years + ' year');
+ } else if (diff.years && diff.years !== 0) {
+ arr.push(diff.years + ' years');
+ } //ergh...
- var currencies = {
- //this one is generic https://en.wikipedia.org/wiki/Template:Currency
- currency: parseCurrency,
- monnaie: parseCurrency,
- unité: parseCurrency,
- nombre: parseCurrency,
- nb: parseCurrency,
- iso4217: parseCurrency,
- inrconvert: inrConvert
- }; //the others fit the same pattern..
- Object.keys(codes).forEach(function (k) {
- currencies[k] = parseCurrency;
- });
- var currency = currencies;
+ diff.days += (diff.months || 0) * 30;
- var templates$4 = {
- //https://en.wikipedia.org/wiki/Template:Election_box
- 'election box begin': function electionBoxBegin(tmpl, list) {
- var data = parse$3(tmpl);
- list.push(data);
- return '';
- },
- 'election box candidate': function electionBoxCandidate(tmpl, list) {
- var data = parse$3(tmpl);
- list.push(data);
- return '';
- },
- 'election box hold with party link': function electionBoxHoldWithPartyLink(tmpl, list) {
- var data = parse$3(tmpl);
- list.push(data);
- return '';
+ if (diff.days === 1) {
+ arr.push('1 day');
+ } else if (diff.days && diff.days !== 0) {
+ arr.push(diff.days + ' days');
+ }
+
+ return arr.join(', ');
},
- 'election box gain with party link': function electionBoxGainWithPartyLink(tmpl, list) {
- var data = parse$3(tmpl);
- list.push(data);
- return '';
+ 'diff-d': tmpl => {
+ let d = getBoth(tmpl);
+ let diff = delta(d.from, d.to);
+ let arr = []; //ergh...
+
+ diff.days += (diff.years || 0) * 365;
+ diff.days += (diff.months || 0) * 30;
+
+ if (diff.days === 1) {
+ arr.push('1 day');
+ } else if (diff.days && diff.days !== 0) {
+ arr.push(diff.days + ' days');
+ }
+
+ return arr.join(', ');
}
- }; //aliases
+ };
+ var _parsers = parsers;
- templates$4['election box begin no change'] = templates$4['election box begin'];
- templates$4['election box begin no party'] = templates$4['election box begin'];
- templates$4['election box begin no party no change'] = templates$4['election box begin'];
- templates$4['election box inline begin'] = templates$4['election box begin'];
- templates$4['election box inline begin no change'] = templates$4['election box begin'];
- templates$4['election box candidate for alliance'] = templates$4['election box candidate'];
- templates$4['election box candidate minor party'] = templates$4['election box candidate'];
- templates$4['election box candidate no party link no change'] = templates$4['election box candidate'];
- templates$4['election box candidate with party link'] = templates$4['election box candidate'];
- templates$4['election box candidate with party link coalition 1918'] = templates$4['election box candidate'];
- templates$4['election box candidate with party link no change'] = templates$4['election box candidate'];
- templates$4['election box inline candidate'] = templates$4['election box candidate'];
- templates$4['election box inline candidate no change'] = templates$4['election box candidate'];
- templates$4['election box inline candidate with party link'] = templates$4['election box candidate'];
- templates$4['election box inline candidate with party link no change'] = templates$4['election box candidate'];
- templates$4['election box inline incumbent'] = templates$4['election box candidate'];
- var elections = templates$4;
-
- var flags = [['🇦🇩', 'and', 'andorra'], ['🇦🇪', 'are', 'united arab emirates'], ['🇦🇫', 'afg', 'afghanistan'], ['🇦🇬', 'atg', 'antigua and barbuda'], ['🇦🇮', 'aia', 'anguilla'], ['🇦🇱', 'alb', 'albania'], ['🇦🇲', 'arm', 'armenia'], ['🇦🇴', 'ago', 'angola'], ['🇦🇶', 'ata', 'antarctica'], ['🇦🇷', 'arg', 'argentina'], ['🇦🇸', 'asm', 'american samoa'], ['🇦🇹', 'aut', 'austria'], ['🇦🇺', 'aus', 'australia'], ['🇦🇼', 'abw', 'aruba'], ['🇦🇽', 'ala', 'åland islands'], ['🇦🇿', 'aze', 'azerbaijan'], ['🇧🇦', 'bih', 'bosnia and herzegovina'], ['🇧🇧', 'brb', 'barbados'], ['🇧🇩', 'bgd', 'bangladesh'], ['🇧🇪', 'bel', 'belgium'], ['🇧🇫', 'bfa', 'burkina faso'], ['🇧🇬', 'bgr', 'bulgaria'], ['🇧🇬', 'bul', //dupe
- 'bulgaria'], ['🇧🇭', 'bhr', 'bahrain'], ['🇧🇮', 'bdi', 'burundi'], ['🇧🇯', 'ben', 'benin'], ['🇧🇱', 'blm', 'saint barthélemy'], ['🇧🇲', 'bmu', 'bermuda'], ['🇧🇳', 'brn', 'brunei darussalam'], ['🇧🇴', 'bol', 'bolivia'], ['🇧🇶', 'bes', 'bonaire, sint eustatius and saba'], ['🇧🇷', 'bra', 'brazil'], ['🇧🇸', 'bhs', 'bahamas'], ['🇧🇹', 'btn', 'bhutan'], ['🇧🇻', 'bvt', 'bouvet island'], ['🇧🇼', 'bwa', 'botswana'], ['🇧🇾', 'blr', 'belarus'], ['🇧🇿', 'blz', 'belize'], ['🇨🇦', 'can', 'canada'], ['🇨🇨', 'cck', 'cocos (keeling) islands'], ['🇨🇩', 'cod', 'congo'], ['🇨🇫', 'caf', 'central african republic'], ['🇨🇬', 'cog', 'congo'], ['🇨🇭', 'che', 'switzerland'], ['🇨🇮', 'civ', "côte d'ivoire"], ['🇨🇰', 'cok', 'cook islands'], ['🇨🇱', 'chl', 'chile'], ['🇨🇲', 'cmr', 'cameroon'], ['🇨🇳', 'chn', 'china'], ['🇨🇴', 'col', 'colombia'], ['🇨🇷', 'cri', 'costa rica'], ['🇨🇺', 'cub', 'cuba'], ['🇨🇻', 'cpv', 'cape verde'], ['🇨🇼', 'cuw', 'curaçao'], ['🇨🇽', 'cxr', 'christmas island'], ['🇨🇾', 'cyp', 'cyprus'], ['🇨🇿', 'cze', 'czech republic'], ['🇩🇪', 'deu', 'germany'], ['🇩🇪', 'ger', //alias
- 'germany'], ['🇩🇯', 'dji', 'djibouti'], ['🇩🇰', 'dnk', 'denmark'], ['🇩🇲', 'dma', 'dominica'], ['🇩🇴', 'dom', 'dominican republic'], ['🇩🇿', 'dza', 'algeria'], ['🇪🇨', 'ecu', 'ecuador'], ['🇪🇪', 'est', 'estonia'], ['🇪🇬', 'egy', 'egypt'], ['🇪🇭', 'esh', 'western sahara'], ['🇪🇷', 'eri', 'eritrea'], ['🇪🇸', 'esp', 'spain'], ['🇪🇹', 'eth', 'ethiopia'], ['🇫🇮', 'fin', 'finland'], ['🇫🇯', 'fji', 'fiji'], ['🇫🇰', 'flk', 'falkland islands (malvinas)'], ['🇫🇲', 'fsm', 'micronesia'], ['🇫🇴', 'fro', 'faroe islands'], ['🇫🇷', 'fra', 'france'], ['🇬🇦', 'gab', 'gabon'], ['🇬🇧', 'gbr', 'united kingdom'], ['🇬🇩', 'grd', 'grenada'], // ['🇬🇪', 'geo', 'georgia'],
- ['🇬🇫', 'guf', 'french guiana'], ['🇬🇬', 'ggy', 'guernsey'], ['🇬🇭', 'gha', 'ghana'], ['🇬🇮', 'gib', 'gibraltar'], ['🇬🇱', 'grl', 'greenland'], ['🇬🇲', 'gmb', 'gambia'], ['🇬🇳', 'gin', 'guinea'], ['🇬🇵', 'glp', 'guadeloupe'], ['🇬🇶', 'gnq', 'equatorial guinea'], ['🇬🇷', 'grc', 'greece'], ['🇬🇸', 'sgs', 'south georgia'], ['🇬🇹', 'gtm', 'guatemala'], ['🇬🇺', 'gum', 'guam'], ['🇬🇼', 'gnb', 'guinea-bissau'], ['🇬🇾', 'guy', 'guyana'], ['🇭🇰', 'hkg', 'hong kong'], ['🇭🇲', 'hmd', 'heard island and mcdonald islands'], ['🇭🇳', 'hnd', 'honduras'], ['🇭🇷', 'hrv', 'croatia'], ['🇭🇹', 'hti', 'haiti'], ['🇭🇺', 'hun', 'hungary'], ['🇮🇩', 'idn', 'indonesia'], ['🇮🇪', 'irl', 'ireland'], ['🇮🇱', 'isr', 'israel'], ['🇮🇲', 'imn', 'isle of man'], ['🇮🇳', 'ind', 'india'], ['🇮🇴', 'iot', 'british indian ocean territory'], ['🇮🇶', 'irq', 'iraq'], ['🇮🇷', 'irn', 'iran'], ['🇮🇸', 'isl', 'iceland'], ['🇮🇹', 'ita', 'italy'], ['🇯🇪', 'jey', 'jersey'], ['🇯🇲', 'jam', 'jamaica'], ['🇯🇴', 'jor', 'jordan'], ['🇯🇵', 'jpn', 'japan'], ['🇰🇪', 'ken', 'kenya'], ['🇰🇬', 'kgz', 'kyrgyzstan'], ['🇰🇭', 'khm', 'cambodia'], ['🇰🇮', 'kir', 'kiribati'], ['🇰🇲', 'com', 'comoros'], ['🇰🇳', 'kna', 'saint kitts and nevis'], ['🇰🇵', 'prk', 'north korea'], ['🇰🇷', 'kor', 'south korea'], ['🇰🇼', 'kwt', 'kuwait'], ['🇰🇾', 'cym', 'cayman islands'], ['🇰🇿', 'kaz', 'kazakhstan'], ['🇱🇦', 'lao', "lao people's democratic republic"], ['🇱🇧', 'lbn', 'lebanon'], ['🇱🇨', 'lca', 'saint lucia'], ['🇱🇮', 'lie', 'liechtenstein'], ['🇱🇰', 'lka', 'sri lanka'], ['🇱🇷', 'lbr', 'liberia'], ['🇱🇸', 'lso', 'lesotho'], ['🇱🇹', 'ltu', 'lithuania'], ['🇱🇺', 'lux', 'luxembourg'], ['🇱🇻', 'lva', 'latvia'], ['🇱🇾', 'lby', 'libya'], ['🇲🇦', 'mar', 'morocco'], ['🇲🇨', 'mco', 'monaco'], ['🇲🇩', 'mda', 'moldova'], ['🇲🇪', 'mne', 'montenegro'], ['🇲🇫', 'maf', 'saint martin (french part)'], ['🇲🇬', 'mdg', 'madagascar'], ['🇲🇭', 'mhl', 'marshall islands'], ['🇲🇰', 'mkd', 'macedonia'], ['🇲🇱', 'mli', 'mali'], ['🇲🇲', 'mmr', 'myanmar'], ['🇲🇳', 'mng', 'mongolia'], ['🇲🇴', 'mac', 'macao'], ['🇲🇵', 'mnp', 'northern mariana islands'], ['🇲🇶', 'mtq', 'martinique'], ['🇲🇷', 'mrt', 'mauritania'], ['🇲🇸', 'msr', 'montserrat'], ['🇲🇹', 'mlt', 'malta'], ['🇲🇺', 'mus', 'mauritius'], ['🇲🇻', 'mdv', 'maldives'], ['🇲🇼', 'mwi', 'malawi'], ['🇲🇽', 'mex', 'mexico'], ['🇲🇾', 'mys', 'malaysia'], ['🇲🇿', 'moz', 'mozambique'], ['🇳🇦', 'nam', 'namibia'], ['🇳🇨', 'ncl', 'new caledonia'], ['🇳🇪', 'ner', 'niger'], ['🇳🇫', 'nfk', 'norfolk island'], ['🇳🇬', 'nga', 'nigeria'], ['🇳🇮', 'nic', 'nicaragua'], ['🇳🇱', 'nld', 'netherlands'], ['🇳🇴', 'nor', 'norway'], ['🇳🇵', 'npl', 'nepal'], ['🇳🇷', 'nru', 'nauru'], ['🇳🇺', 'niu', 'niue'], ['🇳🇿', 'nzl', 'new zealand'], ['🇴🇲', 'omn', 'oman'], ['🇵🇦', 'pan', 'panama'], ['🇵🇪', 'per', 'peru'], ['🇵🇫', 'pyf', 'french polynesia'], ['🇵🇬', 'png', 'papua new guinea'], ['🇵🇭', 'phl', 'philippines'], ['🇵🇰', 'pak', 'pakistan'], ['🇵🇱', 'pol', 'poland'], ['🇵🇲', 'spm', 'saint pierre and miquelon'], ['🇵🇳', 'pcn', 'pitcairn'], ['🇵🇷', 'pri', 'puerto rico'], ['🇵🇸', 'pse', 'palestinian territory'], ['🇵🇹', 'prt', 'portugal'], ['🇵🇼', 'plw', 'palau'], ['🇵🇾', 'pry', 'paraguay'], ['🇶🇦', 'qat', 'qatar'], ['🇷🇪', 'reu', 'réunion'], ['🇷🇴', 'rou', 'romania'], ['🇷🇸', 'srb', 'serbia'], ['🇷🇺', 'rus', 'russia'], ['🇷🇼', 'rwa', 'rwanda'], ['🇸🇦', 'sau', 'saudi arabia'], ['🇸🇧', 'slb', 'solomon islands'], ['🇸🇨', 'syc', 'seychelles'], ['🇸🇩', 'sdn', 'sudan'], ['🇸🇪', 'swe', 'sweden'], ['🇸🇬', 'sgp', 'singapore'], ['🇸🇭', 'shn', 'saint helena, ascension and tristan da cunha'], ['🇸🇮', 'svn', 'slovenia'], ['🇸🇯', 'sjm', 'svalbard and jan mayen'], ['🇸🇰', 'svk', 'slovakia'], ['🇸🇱', 'sle', 'sierra leone'], ['🇸🇲', 'smr', 'san marino'], ['🇸🇳', 'sen', 'senegal'], ['🇸🇴', 'som', 'somalia'], ['🇸🇷', 'sur', 'suriname'], ['🇸🇸', 'ssd', 'south sudan'], ['🇸🇹', 'stp', 'sao tome and principe'], ['🇸🇻', 'slv', 'el salvador'], ['🇸🇽', 'sxm', 'sint maarten (dutch part)'], ['🇸🇾', 'syr', 'syrian arab republic'], ['🇸🇿', 'swz', 'swaziland'], ['🇹🇨', 'tca', 'turks and caicos islands'], ['🇹🇩', 'tcd', 'chad'], ['🇹🇫', 'atf', 'french southern territories'], ['🇹🇬', 'tgo', 'togo'], ['🇹🇭', 'tha', 'thailand'], ['🇹🇯', 'tjk', 'tajikistan'], ['🇹🇰', 'tkl', 'tokelau'], ['🇹🇱', 'tls', 'timor-leste'], ['🇹🇲', 'tkm', 'turkmenistan'], ['🇹🇳', 'tun', 'tunisia'], ['🇹🇴', 'ton', 'tonga'], ['🇹🇷', 'tur', 'turkey'], ['🇹🇹', 'tto', 'trinidad and tobago'], ['🇹🇻', 'tuv', 'tuvalu'], ['🇹🇼', 'twn', 'taiwan'], ['🇹🇿', 'tza', 'tanzania'], ['🇺🇦', 'ukr', 'ukraine'], ['🇺🇬', 'uga', 'uganda'], ['🇺🇲', 'umi', 'united states minor outlying islands'], ['🇺🇸', 'usa', 'united states'], ['🇺🇸', 'us', //alias
- 'united states'], ['🇺🇾', 'ury', 'uruguay'], ['🇺🇿', 'uzb', 'uzbekistan'], ['🇻🇦', 'vat', 'vatican city'], ['🇻🇨', 'vct', 'saint vincent and the grenadines'], ['🇻🇪', 'ven', 'venezuela'], ['🇻🇬', 'vgb', 'virgin islands, british'], ['🇻🇮', 'vir', 'virgin islands, u.s.'], ['🇻🇳', 'vnm', 'viet nam'], ['🇻🇺', 'vut', 'vanuatu'], ['', 'win', 'west indies'], ['🇼🇫', 'wlf', 'wallis and futuna'], ['🇼🇸', 'wsm', 'samoa'], ['🇾🇪', 'yem', 'yemen'], ['🇾🇹', 'myt', 'mayotte'], ['🇿🇦', 'zaf', 'south africa'], ['🇿🇲', 'zmb', 'zambia'], ['🇿🇼 ', 'zwe', 'zimbabwe'], //others (later unicode versions)
- ['🇺🇳', 'un', 'united nations'], ['🏴', 'eng', 'england'], ['🏴', 'sct', 'scotland'], ['🏴', 'wal', 'wales'], ['🇪🇺', 'eu', 'european union']];
+ const months = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December']; //date- templates we support
- var templates$5 = {
- //https://en.wikipedia.org/wiki/Template:Flag
- // {{flag|USA}} → USA
- flag: function flag(tmpl) {
- var order = ['flag', 'variant'];
- var obj = parse$3(tmpl, order);
- var name = obj.flag || '';
- obj.flag = (obj.flag || '').toLowerCase();
- var found = flags.find(function (a) {
- return obj.flag === a[1] || obj.flag === a[2];
- }) || [];
- var flag = found[0] || '';
- return "".concat(flag, " [[").concat(found[2], "|").concat(name, "]]");
+ var dates = {
+ currentday: () => {
+ let d = new Date();
+ return String(d.getDate());
},
- // {{flagcountry|USA}} → United States
- flagcountry: function flagcountry(tmpl) {
- var order = ['flag', 'variant'];
- var obj = parse$3(tmpl, order);
- obj.flag = (obj.flag || '').toLowerCase();
- var found = flags.find(function (a) {
- return obj.flag === a[1] || obj.flag === a[2];
- }) || [];
- var flag = found[0] || '';
- return "".concat(flag, " [[").concat(found[2], "]]");
+ currentdayname: () => {
+ let d = new Date();
+ return _lib$2.days[d.getDay()];
},
- // (unlinked flag-country)
- flagcu: function flagcu(tmpl) {
- var order = ['flag', 'variant'];
- var obj = parse$3(tmpl, order);
- obj.flag = (obj.flag || '').toLowerCase();
- var found = flags.find(function (a) {
- return obj.flag === a[1] || obj.flag === a[2];
- }) || [];
- var flag = found[0] || '';
- return "".concat(flag, " ").concat(found[2]);
+ currentmonth: () => {
+ let d = new Date();
+ return months[d.getMonth()];
},
- //https://en.wikipedia.org/wiki/Template:Flagicon
- // {{flagicon|USA}} → United States
- flagicon: function flagicon(tmpl) {
- var order = ['flag', 'variant'];
- var obj = parse$3(tmpl, order);
- obj.flag = (obj.flag || '').toLowerCase();
- var found = flags.find(function (a) {
- return obj.flag === a[1] || obj.flag === a[2];
- });
+ currentyear: () => {
+ let d = new Date();
+ return String(d.getFullYear());
+ },
+ monthyear: () => {
+ let d = new Date();
+ return months[d.getMonth()] + ' ' + d.getFullYear();
+ },
+ 'monthyear-1': () => {
+ let d = new Date();
+ d.setMonth(d.getMonth() - 1);
+ return months[d.getMonth()] + ' ' + d.getFullYear();
+ },
+ 'monthyear+1': () => {
+ let d = new Date();
+ d.setMonth(d.getMonth() + 1);
+ return months[d.getMonth()] + ' ' + d.getFullYear();
+ },
+ 'time ago': tmpl => {
+ let order = ['date', 'fmt'];
+ let time = toJSON(tmpl, order).date;
+ return _lib$2.timeSince(time);
+ },
+ //https://en.wikipedia.org/wiki/Template:Birth_date_and_age
+ 'birth date and age': (tmpl, list) => {
+ let order = ['year', 'month', 'day'];
+ let obj = toJSON(tmpl, order); //support 'one property' version
- if (!found) {
- return '';
+ if (obj.year && /[a-z]/i.test(obj.year)) {
+ return _parsers.natural_date(tmpl, list);
}
- return "[[".concat(found[2], "|").concat(found[0], "]]");
- },
- //unlinked flagicon
- flagdeco: function flagdeco(tmpl) {
- var order = ['flag', 'variant'];
- var obj = parse$3(tmpl, order);
- obj.flag = (obj.flag || '').toLowerCase();
- var found = flags.find(function (a) {
- return obj.flag === a[1] || obj.flag === a[2];
- }) || [];
- return found[0] || '';
+ list.push(obj);
+ obj = _format.ymd([obj.year, obj.month, obj.day]);
+ return _format.toText(obj);
},
- //same, but a soccer team
- fb: function fb(tmpl) {
- var order = ['flag', 'variant'];
- var obj = parse$3(tmpl, order);
- obj.flag = (obj.flag || '').toLowerCase();
- var found = flags.find(function (a) {
- return obj.flag === a[1] || obj.flag === a[2];
- });
+ 'birth year and age': (tmpl, list) => {
+ let order = ['birth_year', 'birth_month'];
+ let obj = toJSON(tmpl, order); //support 'one property' version
- if (!found) {
- return '';
+ if (obj.death_year && /[a-z]/i.test(obj.death_year)) {
+ return _parsers.natural_date(tmpl, list);
}
- return "".concat(found[0], " [[").concat(found[2], " national football team|").concat(found[2], "]]");
+ list.push(obj);
+ let age = new Date().getFullYear() - parseInt(obj.birth_year, 10);
+ obj = _format.ymd([obj.birth_year, obj.birth_month]);
+ let str = _format.toText(obj);
+
+ if (age) {
+ str += ` (age ${age})`;
+ }
+
+ return str;
},
- fbicon: function fbicon(tmpl) {
- var order = ['flag', 'variant'];
- var obj = parse$3(tmpl, order);
- obj.flag = (obj.flag || '').toLowerCase();
- var found = flags.find(function (a) {
- return obj.flag === a[1] || obj.flag === a[2];
- });
+ 'death year and age': (tmpl, list) => {
+ let order = ['death_year', 'birth_year', 'death_month'];
+ let obj = toJSON(tmpl, order); //support 'one property' version
- if (!found) {
- return '';
+ if (obj.death_year && /[a-z]/i.test(obj.death_year)) {
+ return _parsers.natural_date(tmpl, list);
}
- return " [[".concat(found[2], " national football team|").concat(found[0], "]]");
+ list.push(obj);
+ obj = _format.ymd([obj.death_year, obj.death_month]);
+ return _format.toText(obj);
},
- flagathlete: function flagathlete(tmpl) {
- var order = ['name', 'flag', 'variant'];
- var obj = parse$3(tmpl, order);
- obj.flag = (obj.flag || '').toLowerCase();
- var found = flags.find(function (a) {
- return obj.flag === a[1] || obj.flag === a[2];
- });
+ //https://en.wikipedia.org/wiki/Template:Birth_date_and_age2
+ 'birth date and age2': (tmpl, list) => {
+ let order = ['at_year', 'at_month', 'at_day', 'birth_year', 'birth_month', 'birth_day'];
+ let obj = toJSON(tmpl, order);
+ list.push(obj);
+ obj = _format.ymd([obj.birth_year, obj.birth_month, obj.birth_day]);
+ return _format.toText(obj);
+ },
+ //https://en.wikipedia.org/wiki/Template:Birth_based_on_age_as_of_date
+ 'birth based on age as of date': (tmpl, list) => {
+ let order = ['age', 'year', 'month', 'day'];
+ let obj = toJSON(tmpl, order);
+ list.push(obj);
+ let age = parseInt(obj.age, 10);
+ let year = parseInt(obj.year, 10);
+ let born = year - age;
- if (!found) {
- return "[[".concat(obj.name || '', "]]");
+ if (born && age) {
+ return `${born} (age ${obj.age})`;
}
- return "".concat(found[0], " [[").concat(obj.name || '', "]] (").concat(found[1].toUpperCase(), ")");
- }
- }; //support {{can}}
+ return `(age ${obj.age})`;
+ },
+ //https://en.wikipedia.org/wiki/Template:Death_date_and_given_age
+ 'death date and given age': (tmpl, list) => {
+ let order = ['year', 'month', 'day', 'age'];
+ let obj = toJSON(tmpl, order);
+ list.push(obj);
+ obj = _format.ymd([obj.year, obj.month, obj.day]);
+ let str = _format.toText(obj);
- flags.forEach(function (a) {
- templates$5[a[1]] = function () {
- return a[0];
- };
- }); //cricket
+ if (obj.age) {
+ str += ` (age ${obj.age})`;
+ }
- templates$5['cr'] = templates$5.flagcountry;
- templates$5['cr-rt'] = templates$5.flagcountry;
- templates$5['cricon'] = templates$5.flagicon;
- var flags_1 = templates$5;
+ return str;
+ },
+ //sortable dates -
+ dts: tmpl => {
+ //remove formatting stuff, ewww
+ tmpl = tmpl.replace(/\|format=[ymd]+/i, '');
+ tmpl = tmpl.replace(/\|abbr=(on|off)/i, '');
+ let order = ['year', 'month', 'date', 'bc'];
+ let obj = toJSON(tmpl, order);
- var getLang = function getLang(name) {
- //grab the language from the template name - 'ipa-de'
- var lang = name.match(/ipac?-(.+)/);
+ if (obj.date && obj.month && obj.year) {
+ //render 'june 5 2018'
+ if (/[a-z]/.test(obj.month) === true) {
+ return [obj.month, obj.date, obj.year].join(' ');
+ }
- if (lang !== null) {
- if (languages.hasOwnProperty(lang[1]) === true) {
- return languages[lang[1]].english_title;
+ return [obj.year, obj.month, obj.date].join('-');
}
- return lang[1];
- }
+ if (obj.month && obj.year) {
+ return [obj.year, obj.month].join('-');
+ }
- return null;
- }; // pronounciation info
+ if (obj.year) {
+ if (obj.year < 0) {
+ obj.year = Math.abs(obj.year) + ' BC';
+ }
+ return obj.year;
+ }
- var templates$6 = {
- // https://en.wikipedia.org/wiki/Template:IPA
- ipa: function ipa(tmpl, list) {
- var obj = parse$3(tmpl, ['transcription', 'lang', 'audio']);
- obj.lang = getLang(obj.template);
- obj.template = 'ipa';
- list.push(obj);
return '';
},
- //https://en.wikipedia.org/wiki/Template:IPAc-en
- ipac: function ipac(tmpl, list) {
- var obj = parse$3(tmpl);
- obj.transcription = (obj.list || []).join(',');
- delete obj.list;
- obj.lang = getLang(obj.template);
- obj.template = 'ipac';
- list.push(obj);
- return '';
+ //we can't do timezones, so fake this one a little bit
+ //https://en.wikipedia.org/wiki/Template:Time
+ time: () => {
+ let d = new Date();
+ let obj = _format.ymd([d.getFullYear(), d.getMonth(), d.getDate()]);
+ return _format.toText(obj);
},
- transl: function transl(tmpl, list) {
- var obj = parse$3(tmpl, ['lang', 'text', 'text2']); // support 3-param
+ // https://en.wikipedia.org/wiki/Template:MILLENNIUM
+ millennium: tmpl => {
+ let obj = toJSON(tmpl, ['year']);
+ let year = Number(obj.year);
+ year = parseInt(year / 1000, 10) + 1;
- if (obj.text2) {
- obj.iso = obj.text;
- obj.text = obj.text2;
- delete obj.text2;
+ if (obj.abbr && obj.abbr === 'y') {
+ if (year < 0) {
+ return `${_lib$2.toOrdinal(Math.abs(year))} BC`;
+ }
+
+ return `${_lib$2.toOrdinal(year)}`;
}
- list.push(obj);
- return obj.text || '';
- }
- }; // - other languages -
- // Polish, {{IPAc-pl}} {{IPAc-pl|'|sz|cz|e|ć|i|n}} → [ˈʂt͡ʂɛt͡ɕin]
- // Portuguese, {{IPAc-pt}} {{IPAc-pt|p|o|<|r|t|u|'|g|a|l|lang=pt}} and {{IPAc-pt|b|r|a|'|s|i|l|lang=br}} → [puɾtuˈɣaɫ] and [bɾaˈsiw]
+ return `${_lib$2.toOrdinal(year)} millennium`;
+ },
+ //date/age/time templates
+ start: _parsers.date,
+ 'start-date': _parsers.natural_date,
+ birthdeathage: _parsers.two_dates,
+ age: _parsers.age,
+ 'age nts': _parsers.age,
+ 'age in years': _parsers['diff-y'],
+ 'age in years and months': _parsers['diff-ym'],
+ 'age in years, months and days': _parsers['diff-ymd'],
+ 'age in years and days': _parsers['diff-yd'],
+ 'age in days': _parsers['diff-d'] // 'birth date and age2': date,
+ // 'age in years, months, weeks and days': true,
+ // 'age as of date': true,
- Object.keys(languages).forEach(function (lang) {
- templates$6['ipa-' + lang] = templates$6.ipa;
- templates$6['ipac-' + lang] = templates$6.ipac;
- });
- var ipa = templates$6;
+ };
- var templates$7 = {
- lang: 1,
- //this one has a million variants
- 'lang-de': 0,
- 'rtl-lang': 1,
- //german keyboard letterscn
- taste: 0,
- //https://en.wikipedia.org/wiki/Template:Nihongo
- nihongo: function nihongo(tmpl, list) {
- var obj = parse$3(tmpl, ['english', 'kanji', 'romaji', 'extra']);
- list.push(obj);
- var str = obj.english || obj.romaji || '';
+ /**
+ * converts DMS (decimal-minute-second) geo format to lat/lng format.
+ * major thank you to https://github.com/gmaclennan/parse-dms and https://github.com/WSDOT-GIS/dms-js 👏
+ **/
- if (obj.kanji) {
- str += " (".concat(obj.kanji, ")");
- }
+ function parseDMS(arr) {
+ let hemisphere = arr.pop();
+ let degrees = Number(arr[0] || 0);
+ let minutes = Number(arr[1] || 0);
+ let seconds = Number(arr[2] || 0);
- return str;
+ if (typeof hemisphere !== 'string' || isNaN(degrees)) {
+ return null;
}
- }; //https://en.wikipedia.org/wiki/Category:Lang-x_templates
-
- Object.keys(languages).forEach(function (k) {
- templates$7['lang-' + k] = templates$7['lang-de'];
- });
- templates$7['nihongo2'] = templates$7.nihongo;
- templates$7['nihongo3'] = templates$7.nihongo;
- templates$7['nihongo-s'] = templates$7.nihongo;
- templates$7['nihongo foot'] = templates$7.nihongo;
- var languages_1 = templates$7;
- //simply num/denom * 100
+ let sign = 1;
- var _percentage = function percentage(obj) {
- if (!obj.numerator && !obj.denominator) {
- return null;
+ if (/[SW]/i.test(hemisphere)) {
+ sign = -1;
}
- var perc = Number(obj.numerator) / Number(obj.denominator);
- perc *= 100;
- var dec = Number(obj.decimals);
+ return sign * (degrees + minutes / 60 + seconds / 3600);
+ }
- if (isNaN(dec)) {
- dec = 1;
+ const round = function (num) {
+ if (typeof num !== 'number') {
+ return num;
}
- perc = perc.toFixed(dec);
- return Number(perc);
- };
+ let places = 100000;
+ return Math.round(num * places) / places;
+ }; //these hemispheres mean negative decimals
- var templates$8 = {
- // https://en.wikipedia.org/wiki/Template:Math
- math: function math(tmpl, list) {
- var obj = parse$3(tmpl, ['formula']);
- list.push(obj);
- return '\n\n' + (obj.formula || '') + '\n\n';
- },
- //fraction - https://en.wikipedia.org/wiki/Template:Sfrac
- frac: function frac(tmpl, list) {
- var order = ['a', 'b', 'c'];
- var obj = parse$3(tmpl, order);
- var data = {
- template: 'sfrac'
- };
- if (obj.c) {
- data.integer = obj.a;
- data.numerator = obj.b;
- data.denominator = obj.c;
- } else if (obj.b) {
- data.numerator = obj.a;
- data.denominator = obj.b;
- } else {
- data.numerator = 1;
- data.denominator = obj.a;
- }
+ const negative = {
+ s: true,
+ w: true
+ };
- list.push(data);
+ const findLatLng = function (arr) {
+ const types = arr.map(s => typeof s).join('|'); //support {{lat|lng}}
- if (data.integer) {
- return "".concat(data.integer, " ").concat(data.numerator, "\u2044").concat(data.denominator);
- }
+ if (arr.length === 2 && types === 'number|number') {
+ return {
+ lat: arr[0],
+ lon: arr[1]
+ };
+ } //support {{dd|N/S|dd|E/W}}
- return "".concat(data.numerator, "\u2044").concat(data.denominator);
- },
- //https://en.wikipedia.org/wiki/Template:Radic
- radic: function radic(tmpl) {
- var order = ['after', 'before'];
- var obj = parse$3(tmpl, order);
- return "".concat(obj.before || '', "\u221A").concat(obj.after || '');
- },
- //{{percentage | numerator | denominator | decimals to round to (zero or greater) }}
- percentage: function percentage(tmpl) {
- var obj = parse$3(tmpl, ['numerator', 'denominator', 'decimals']);
- var num = _percentage(obj);
+ if (arr.length === 4 && types === 'number|string|number|string') {
+ if (negative[arr[1].toLowerCase()]) {
+ arr[0] *= -1;
+ }
- if (num === null) {
- return '';
+ if (arr[3].toLowerCase() === 'w') {
+ arr[2] *= -1;
}
- return num + '%';
- },
- // {{Percent-done|done=N|total=N|digits=N}}
- 'percent-done': function percentDone(tmpl) {
- var obj = parse$3(tmpl, ['done', 'total', 'digits']);
+ return {
+ lat: arr[0],
+ lon: arr[2]
+ };
+ } //support {{dd|mm|N/S|dd|mm|E/W}}
- var num = _percentage({
- numerator: obj.done,
- denominator: obj.total,
- decimals: obj.digits
- });
- if (num === null) {
- return '';
- }
+ if (arr.length === 6) {
+ return {
+ lat: parseDMS(arr.slice(0, 3)),
+ lon: parseDMS(arr.slice(3))
+ };
+ } //support {{dd|mm|ss|N/S|dd|mm|ss|E/W}}
- return "".concat(obj.done, " (").concat(num, "%) done");
- },
- 'winning percentage': function winningPercentage(tmpl, list) {
- var obj = parse$3(tmpl, ['wins', 'losses', 'ties']);
- list.push(obj);
- var wins = Number(obj.wins);
- var losses = Number(obj.losses);
- var ties = Number(obj.ties) || 0;
- var games = wins + losses + ties;
- if (obj.ignore_ties === 'y') {
- ties = 0;
- }
+ if (arr.length === 8) {
+ return {
+ lat: parseDMS(arr.slice(0, 4)),
+ lon: parseDMS(arr.slice(4))
+ };
+ }
- if (ties) {
- wins += ties / 2;
- }
+ return {};
+ };
- var num = _percentage({
- numerator: wins,
- denominator: games,
- decimals: 1
- });
+ const parseParams = function (obj) {
+ obj.list = obj.list || [];
+ obj.list = obj.list.map(str => {
+ let num = Number(str);
- if (num === null) {
- return '';
+ if (!isNaN(num)) {
+ return num;
+ } //these are weird
+
+
+ let split = str.split(/:/);
+
+ if (split.length > 1) {
+ obj.props = obj.props || {};
+ obj.props[split[0]] = split.slice(1).join(':');
+ return null;
}
- return ".".concat(num * 10);
- },
- winlosspct: function winlosspct(tmpl, list) {
- var obj = parse$3(tmpl, ['wins', 'losses']);
- list.push(obj);
- var wins = Number(obj.wins);
- var losses = Number(obj.losses);
+ return str;
+ });
+ obj.list = obj.list.filter(s => s !== null);
+ return obj;
+ };
- var num = _percentage({
- numerator: wins,
- denominator: wins + losses,
- decimals: 1
- });
+ const parseCoor = function (tmpl) {
+ let obj = toJSON(tmpl);
+ obj = parseParams(obj);
+ let tmp = findLatLng(obj.list);
+ obj.lat = round(tmp.lat);
+ obj.lon = round(tmp.lon);
+ obj.template = 'coord';
+ delete obj.list;
+ return obj;
+ }; //console.log(parseDms([57, 18, 22, 'N']));
+ //console.log(parseDms([4, 27, 32, 'W']));
- if (num === null) {
- return '';
+
+ var _lib$1 = parseCoor; // {{Coor title dms|dd|mm|ss|N/S|dd|mm|ss|E/W|template parameters}}
+
+ const templates$1 = {
+ coord: (tmpl, list) => {
+ let obj = _lib$1(tmpl);
+ list.push(obj); //display inline, by default
+
+ if (!obj.display || obj.display.indexOf('inline') !== -1) {
+ return `${obj.lat || ''}°N, ${obj.lon || ''}°W`;
}
- num = ".".concat(num * 10);
- return "".concat(wins || 0, " || ").concat(losses || 0, " || ").concat(num || '-');
+ return '';
}
- }; //aliases
+ }; //{{coord|latitude|longitude|coordinate parameters|template parameters}}
+ //{{coord|dd|N/S|dd|E/W|coordinate parameters|template parameters}}
+ //{{coord|dd|mm|N/S|dd|mm|E/W|coordinate parameters|template parameters}}
+ //{{coord|dd|mm|ss|N/S|dd|mm|ss|E/W|coordinate parameters|template parameters}}
- templates$8['sfrac'] = templates$8.frac;
- templates$8['sqrt'] = templates$8.radic;
- templates$8['pct'] = templates$8.percentage;
- templates$8['percent'] = templates$8.percentage;
- templates$8['winpct'] = templates$8['winning percentage'];
- templates$8['winperc'] = templates$8['winning percentage'];
- var math = templates$8;
+ var geo = templates$1;
- var generic = function generic(tmpl, list, alias) {
- var obj = parse$3(tmpl);
+ const generic = function (tmpl, list, _parser, alias) {
+ let obj = toJSON(tmpl);
if (alias) {
obj.name = obj.template;
@@ -7322,10 +7085,11 @@
list.push(obj);
return '';
- };
+ }; // it may seem redundant,
+ // but we need these templates for our i18n mappings
- var misc$2 = {
- //i18n templates
+
+ const misc = {
persondata: generic,
taxobox: generic,
citation: generic,
@@ -7335,220 +7099,323 @@
'cite journal': generic,
'cite web': generic,
'commons cat': generic,
- // https://en.wikipedia.org/wiki/Template:Portuguese_name
- 'portuguese name': ['first', 'second', 'suffix'],
- uss: ['ship', 'id'],
- isbn: function isbn(tmpl, list) {
- var order = ['id', 'id2', 'id3'];
- var obj = parse$3(tmpl, order);
- list.push(obj);
- return 'ISBN: ' + (obj.id || '');
- },
- //https://en.wikipedia.org/wiki/Template:Marriage
- //this one creates a template, and an inline response
- marriage: function marriage(tmpl, list) {
- var data = parse$3(tmpl, ['spouse', 'from', 'to', 'end']);
- list.push(data);
- var str = "".concat(data.spouse || '');
+ 'election box candidate': generic,
+ 'election box begin': generic,
+ main: generic
+ };
+ var misc_1 = misc;
- if (data.from) {
- if (data.to) {
- str += " (m. ".concat(data.from, "-").concat(data.to, ")");
- } else {
- str += " (m. ".concat(data.from, ")");
- }
- }
+ const codes = {
+ adx: 'adx',
+ //https://en.wikipedia.org/wiki/Template:Abu_Dhabi_Securities_Exchange
+ aim: 'aim',
+ //https://en.wikipedia.org/wiki/Template:Alternative_Investment_Market
+ amex: 'amex',
+ //https://en.wikipedia.org/wiki/Template:NYSE_American
+ asx: 'asx',
+ //https://en.wikipedia.org/wiki/Template:Australian_Securities_Exchange
+ athex: 'athex',
+ //https://en.wikipedia.org/wiki/Template:Athens_Exchange
+ b3: 'b3',
+ //https://en.wikipedia.org/wiki/Template:BM%26F_Bovespa (redirects to B3 (stock exchange))
+ 'B3 (stock exchange)': 'B3 (stock exchange)',
+ //https://en.wikipedia.org/wiki/Template:B3_(stock_exchange)
+ barbadosse: 'barbadosse',
+ //https://en.wikipedia.org/wiki/Template:Barbados_Stock_Exchange
+ bbv: 'bbv',
+ //https://en.wikipedia.org/wiki/Template:La_Paz_Stock_Exchange
+ bcba: 'bcba',
+ //https://en.wikipedia.org/wiki/Template:Buenos_Aires_Stock_Exchange
+ bcs: 'bcs',
+ //https://en.wikipedia.org/wiki/Template:Santiago_Stock_Exchange
+ bhse: 'bhse',
+ //https://en.wikipedia.org/wiki/Template:Bahrain_Bourse
+ bist: 'bist',
+ //https://en.wikipedia.org/wiki/Template:Borsa_Istanbul
+ bit: 'bit',
+ //https://en.wikipedia.org/wiki/Template:Borsa_Italiana
+ 'bm&f bovespa': 'b3',
+ //https://en.wikipedia.org/wiki/Template:BM%26F_Bovespa
+ 'bm&f': 'b3',
+ //https://en.wikipedia.org/wiki/Template:BM%26F_Bovespa
+ bmad: 'bmad',
+ //https://en.wikipedia.org/wiki/Template:Bolsa_de_Madrid
+ bmv: 'bmv',
+ //https://en.wikipedia.org/wiki/Template:Mexican_Stock_Exchange
+ 'bombay stock exchange': 'bombay stock exchange',
+ //https://en.wikipedia.org/wiki/Template:Bombay_Stock_Exchange
+ 'botswana stock exchange': 'botswana stock exchange',
+ //https://en.wikipedia.org/wiki/Template:BM%26F_Bovespa
+ bpse: 'bpse',
+ //https://en.wikipedia.org/wiki/Template:Budapest_Stock_Exchange
+ bse: 'bse',
+ //https://en.wikipedia.org/wiki/Template:Bombay_Stock_Exchange
+ bsx: 'bsx',
+ //https://en.wikipedia.org/wiki/Template:Bermuda_Stock_Exchange
+ bvb: 'bvb',
+ //https://en.wikipedia.org/wiki/Template:Bucharest_Stock_Exchange
+ bvc: 'bvc',
+ //https://en.wikipedia.org/wiki/Template:Colombian_Securities_Exchange
+ bvl: 'bvl',
+ //https://en.wikipedia.org/wiki/Template:Lima_Stock_Exchange
+ bvpasa: 'bvpasa',
+ //https://en.wikipedia.org/wiki/Template:BVPASA
+ bwse: 'bwse',
+ //https://en.wikipedia.org/wiki/Template:Botswana_Stock_Exchange
+ 'canadian securities exchange': 'canadian securities exchange',
+ //https://en.wikipedia.org/wiki/Template:Canadian_Securities_Exchange
+ cse: 'cse',
+ //https://en.wikipedia.org/wiki/Template:Chittagong_Stock_Exchange
+ darse: 'darse',
+ //https://en.wikipedia.org/wiki/Template:Dar_es_Salaam_Stock_Exchange
+ dfm: 'dfm',
+ //https://en.wikipedia.org/wiki/Template:Dubai_Financial_Market
+ dse: 'dse',
+ //https://en.wikipedia.org/wiki/Template:Dhaka_Stock_Exchange
+ euronext: 'euronext',
+ //https://en.wikipedia.org/wiki/Template:Euronext
+ euronextparis: 'euronextparis',
+ //https://en.wikipedia.org/wiki/Template:EuronextParis
+ fse: 'fse',
+ //https://en.wikipedia.org/wiki/Template:Fukuoka_Stock_Exchange
+ fwb: 'fwb',
+ //https://en.wikipedia.org/wiki/Template:Frankfurt_Stock_Exchange
+ gse: 'gse',
+ //https://en.wikipedia.org/wiki/Template:Ghana_Stock_Exchange
+ gtsm: 'gtsm',
+ //https://en.wikipedia.org/wiki/Template:Gre_Tai_Securities_Market
+ idx: 'idx',
+ //https://en.wikipedia.org/wiki/Template:Indonesia_Stock_Exchange
+ ise: 'ise',
+ //https://en.wikipedia.org/wiki/Template:Irish_Stock_Exchange
+ iseq: 'iseq',
+ //https://en.wikipedia.org/wiki/Template:Irish_Stock_Exchange
+ isin: 'isin',
+ //https://en.wikipedia.org/wiki/Template:ISIN
+ jasdaq: 'jasdaq',
+ //https://en.wikipedia.org/wiki/Template:JASDAQ
+ jse: 'jse',
+ //https://en.wikipedia.org/wiki/Template:Johannesburg_Stock_Exchange
+ kase: 'kase',
+ //https://en.wikipedia.org/wiki/Template:Kazakhstan_Stock_Exchange
+ kn: 'kn',
+ //https://en.wikipedia.org/wiki/Template:Nairobi_Securities_Exchange
+ krx: 'krx',
+ //https://en.wikipedia.org/wiki/Template:Korea_Exchange
+ lse: 'lse',
+ //https://en.wikipedia.org/wiki/Template:London_Stock_Exchange
+ luxse: 'luxse',
+ //https://en.wikipedia.org/wiki/Template:Luxembourg_Stock_Exchange
+ 'malta stock exchange': 'malta stock exchange',
+ //https://en.wikipedia.org/wiki/Template:Malta_Stock_Exchange
+ mai: 'mai',
+ //https://en.wikipedia.org/wiki/Template:Market_for_Alternative_Investment
+ mcx: 'mcx',
+ //https://en.wikipedia.org/wiki/Template:Moscow_Exchange
+ mutf: 'mutf',
+ //https://en.wikipedia.org/wiki/Template:Mutual_fund
+ myx: 'myx',
+ //https://en.wikipedia.org/wiki/Template:Bursa_Malaysia
+ nag: 'nag',
+ //https://en.wikipedia.org/wiki/Template:Nagoya_Stock_Exchange
+ 'nasdaq dubai': 'nasdaq dubai',
+ //https://en.wikipedia.org/wiki/Template:NASDAQ_Dubai
+ nasdaq: 'nasdaq',
+ //https://en.wikipedia.org/wiki/Template:NASDAQ
+ neeq: 'neeq',
+ //https://en.wikipedia.org/wiki/Template:NEEQ
+ nepse: 'nepse',
+ //https://en.wikipedia.org/wiki/Template:Nepal_Stock_Exchange
+ nex: 'nex',
+ //https://en.wikipedia.org/wiki/Template:TSXV_NEX
+ nse: 'nse',
+ //https://en.wikipedia.org/wiki/Template:National_Stock_Exchange_of_India
+ newconnect: 'newconnect',
+ //https://en.wikipedia.org/wiki/Template:NewConnect
+ 'nyse arca': 'nyse arca',
+ //https://en.wikipedia.org/wiki/Template:NYSE_Arca
+ nyse: 'nyse',
+ //https://en.wikipedia.org/wiki/Template:New_York_Stock_Exchange
+ nzx: 'nzx',
+ //https://en.wikipedia.org/wiki/Template:New_Zealand_Exchange
+ 'omx baltic': 'omx baltic',
+ //https://en.wikipedia.org/wiki/Template:OMX_Baltic
+ omx: 'omx',
+ //https://en.wikipedia.org/wiki/Template:OMX
+ ose: 'ose',
+ //https://en.wikipedia.org/wiki/Template:Oslo_Stock_Exchange
+ 'otc expert': 'otc expert',
+ //https://en.wikipedia.org/wiki/Template:OTC_Expert
+ 'otc grey': 'otc grey',
+ //https://en.wikipedia.org/wiki/template:grey_market
+ 'otc pink': 'otc pink',
+ //https://en.wikipedia.org/wiki/Template:OTC_Pink
+ otcqb: 'otcqb',
+ //https://en.wikipedia.org/wiki/Template:OTCQB
+ otcqx: 'otcqx',
+ //https://en.wikipedia.org/wiki/Template:OTCQX
+ 'pfts ukraine stock exchange': 'pfts ukraine stock exchange',
+ //https://en.wikipedia.org/wiki/Template:PFTS_Ukraine_Stock_Exchange
+ 'philippine stock exchange': 'philippine stock exchange',
+ //https://en.wikipedia.org/wiki/Template:Philippine_Stock_Exchange
+ prse: 'prse',
+ //https://en.wikipedia.org/wiki/Template:Prague_Stock_Exchange
+ psx: 'psx',
+ //https://en.wikipedia.org/wiki/Template:Pakistan_Stock_Exchange
+ karse: 'karse',
+ //https://en.wikipedia.org/w/index.php?title=Template:Karse&redirect=no (redirects to psx)
+ qe: 'qe',
+ //https://en.wikipedia.org/wiki/Template:Qatar_Stock_Exchange
+ 'saudi stock exchange': 'saudi stock exchange',
+ //https://en.wikipedia.org/wiki/Template:Saudi_Stock_Exchange
+ sehk: 'sehk',
+ //https://en.wikipedia.org/wiki/Template:Hong_Kong_Stock_Exchange
+ 'Stock Exchange of Thailand': 'Stock Exchange of Thailand',
+ //https://en.wikipedia.org/wiki/Template:Stock_Exchange_of_Thailand (alternative for SET)
+ set: 'set',
+ //https://en.wikipedia.org/wiki/Template:Stock_Exchange_of_Thailand
+ sgx: 'sgx',
+ //https://en.wikipedia.org/wiki/Template:Singapore_Exchange
+ sse: 'sse',
+ //https://en.wikipedia.org/wiki/Template:Shanghai_Stock_Exchange
+ swx: 'swx',
+ //https://en.wikipedia.org/wiki/Template:SIX_Swiss_Exchange
+ szse: 'szse',
+ //https://en.wikipedia.org/wiki/Template:Shenzhen_Stock_Exchange
+ tase: 'tase',
+ //https://en.wikipedia.org/wiki/Template:Tel_Aviv_Stock_Exchange
+ 'tsx-v': 'tsx-v',
+ //https://en.wikipedia.org/wiki/Template:TSX_Venture_Exchange
+ tsx: 'tsx',
+ //https://en.wikipedia.org/wiki/Template:Toronto_Stock_Exchange
+ tsxv: 'tsxv',
+ //https://en.wikipedia.org/wiki/Template:TSX_Venture_Exchange
+ ttse: 'ttse',
+ //https://en.wikipedia.org/wiki/Template:Trinidad_and_Tobago_Stock_Exchange
+ twse: 'twse',
+ //https://en.wikipedia.org/wiki/Template:Taiwan_Stock_Exchange
+ tyo: 'tyo',
+ //https://en.wikipedia.org/wiki/Template:Tokyo_Stock_Exchange
+ wbag: 'wbag',
+ //https://en.wikipedia.org/wiki/Template:Wiener_B%C3%B6rse
+ wse: 'wse',
+ //https://en.wikipedia.org/wiki/Template:Warsaw_Stock_Exchange
+ 'zagreb stock exchange': 'zagreb stock exchange',
+ //https://en.wikipedia.org/wiki/Template:Zagreb_Stock_Exchange
+ 'zimbabwe stock exchange': 'zimbabwe stock exchange',
+ //https://en.wikipedia.org/wiki/Template:Zimbabwe_Stock_Exchange
+ zse: 'zse' //https://en.wikipedia.org/wiki/Template:Zagreb_Stock_Exchange
- return str;
- },
- //https://en.wikipedia.org/wiki/Template:Based_on
- 'based on': function basedOn(tmpl, list) {
- var obj = parse$3(tmpl, ['title', 'author']);
- list.push(obj);
- return "".concat(obj.title, " by ").concat(obj.author || '');
- },
- //https://en.wikipedia.org/wiki/Template:Video_game_release
- 'video game release': function videoGameRelease(tmpl, list) {
- var order = ['region', 'date', 'region2', 'date2', 'region3', 'date3', 'region4', 'date4'];
- var obj = parse$3(tmpl, order);
- var template = {
- template: 'video game release',
- releases: []
- };
+ };
- for (var i = 0; i < order.length; i += 2) {
- if (obj[order[i]]) {
- template.releases.push({
- region: obj[order[i]],
- date: obj[order[i + 1]]
- });
- }
- }
-
- list.push(template);
- var str = template.releases.map(function (o) {
- return "".concat(o.region, ": ").concat(o.date || '');
- }).join('\n\n');
- return '\n' + str + '\n';
- },
- //barrels of oil https://en.wikipedia.org/wiki/Template:Bbl_to_t
- 'bbl to t': function bblToT(tmpl, list) {
- var obj = parse$3(tmpl, ['barrels']);
- list.push(obj);
+ const parseStockExchange = (tmpl, list) => {
+ let o = toJSON(tmpl, ['ticketnumber', 'code']);
+ list.push(o);
+ let code = o.template || '';
- if (obj.barrels === '0') {
- return obj.barrels + ' barrel';
- }
+ if (code === '') {
+ code = o.code;
+ }
- return obj.barrels + ' barrels';
- },
- //https://en.wikipedia.org/wiki/Template:Historical_populations
- 'historical populations': function historicalPopulations(tmpl, list) {
- var data = parse$3(tmpl);
- data.list = data.list || [];
- var years = [];
+ code = (code || '').toLowerCase();
+ let str = codes[code] || '';
- for (var i = 0; i < data.list.length; i += 2) {
- var num = data.list[i + 1];
- years.push({
- year: data.list[i],
- val: Number(num) || num
- });
- }
+ if (o.ticketnumber) {
+ str = `${str}: ${o.ticketnumber}`;
+ }
- data.data = years;
- delete data.list;
- list.push(data);
- return '';
+ if (o.code && !codes[o.code.toLowerCase()]) {
+ str += ' ' + o.code;
}
+
+ return str;
};
- var misc_1$1 = misc$2;
- // okay, these just hurts my feelings
- // https://www.mediawiki.org/wiki/Help:Magic_words#Other
- var punctuation = [// https://en.wikipedia.org/wiki/Template:%C2%B7
- ['·', '·'], ['·', '·'], ['dot', '·'], ['middot', '·'], ['•', ' • '], //yup, oxford comma template
- [',', ','], ['1/2', '1⁄2'], ['1/3', '1⁄3'], ['2/3', '2⁄3'], ['1/4', '1⁄4'], ['3/4', '3⁄4'], ['–', '–'], ['ndash', '–'], ['en dash', '–'], ['spaced ndash', ' – '], ['—', '—'], ['mdash', '—'], ['em dash', '—'], ['number sign', '#'], ['ibeam', 'I'], ['&', '&'], [';', ';'], ['ampersand', '&'], ['snds', ' – '], ['snd', ' – '], // these '{{^}}' things are nuts, and used as some ilicit spacing thing.
- ['^', ' '], ['!', '|'], ['\\', ' /'], ['`', '`'], ['=', '='], ['bracket', '['], ['[', '['], ['*', '*'], ['asterisk', '*'], ['long dash', '———'], ['clear', '\n\n'], ['h.', 'ḥ']];
- var templates$9 = {};
- punctuation.forEach(function (a) {
- templates$9[a[0]] = a[1];
+ const exchanges = {}; //the others fit the same pattern..
+
+ Object.keys(codes).forEach(k => {
+ exchanges[k] = parseStockExchange;
});
- var punctuation_1 = templates$9;
+ var stockExchanges = exchanges;
- var templates$a = {
- //https://en.wikipedia.org/wiki/Template:Taxon_info
- 'taxon info': ['taxon', 'item'],
- //minor planet - https://en.wikipedia.org/wiki/Template:MPC
- mpc: function mpc(tmpl, list) {
- var obj = parse$3(tmpl, ['number', 'text']);
- list.push(obj);
- return "[https://minorplanetcenter.net/db_search/show_object?object_id=P/2011+NO1 ".concat(obj.text || obj.number, "]");
- },
- //https://en.wikipedia.org/wiki/Template:Chem2
- chem2: function chem2(tmpl, list) {
- var obj = parse$3(tmpl, ['equation']);
- list.push(obj);
- return obj.equation;
- },
- //https://en.wikipedia.org/wiki/Template:Sky
- sky: function sky(tmpl, list) {
- var obj = parse$3(tmpl, ['asc_hours', 'asc_minutes', 'asc_seconds', 'dec_sign', 'dec_degrees', 'dec_minutes', 'dec_seconds', 'distance']);
- var template = {
- template: 'sky',
- ascension: {
- hours: obj.asc_hours,
- minutes: obj.asc_minutes,
- seconds: obj.asc_seconds
- },
- declination: {
- sign: obj.dec_sign,
- degrees: obj.dec_degrees,
- minutes: obj.dec_minutes,
- seconds: obj.dec_seconds
- },
- distance: obj.distance
- };
- list.push(template);
- return '';
- },
- // Parse https://en.wikipedia.org/wiki/Template:Medical_cases_chart -- see
- // https://en.wikipedia.org/wiki/Module:Medical_cases_chart for the original
- // parsing code.
- 'medical cases chart': function medicalCasesChart(tmpl, list) {
- var order = ['date', 'deathsExpr', 'recoveriesExpr', 'casesExpr', '4thExpr', '5thExpr', 'col1', 'col1Change', 'col2', 'col2Change'];
- var obj = parse$3(tmpl);
- obj.data = obj.data || '';
- var rows = obj.data.split('\n'); // Mimic row parsing in _buildBars in the Lua source, from the following
- // line on:
- //
- // for parameter in mw.text.gsplit(line, ';') do
+ const zeroPad = function (num) {
+ num = String(num);
- var dataArray = rows.map(function (row) {
- var parameters = row.split(';');
- var rowObject = {
- options: new Map()
- };
- var positionalIndex = 0;
+ if (num.length === 1) {
+ num = '0' + num;
+ }
- for (var i = 0; i < parameters.length; i++) {
- var parameter = parameters[i].trim();
+ return num;
+ };
- if (parameter.match(/^[a-zA-Z_]/)) {
- // Named argument
- var _parameter$split = parameter.split('='),
- _parameter$split2 = _slicedToArray(_parameter$split, 2),
- key = _parameter$split2[0],
- value = _parameter$split2[1]; // At this point, the Lua code evaluates alttot1 and alttot2 values as
- // #expr expressions, but we just pass them through. See also:
- // https://www.mediawiki.org/wiki/Help:Extension:ParserFunctions##expr
+ const parseTeam = function (obj, round, team) {
+ if (obj[`rd${round}-team${zeroPad(team)}`]) {
+ team = zeroPad(team);
+ }
+ let score = obj[`rd${round}-score${team}`];
+ let num = Number(score);
- if (value === undefined) {
- value = null;
- }
+ if (isNaN(num) === false) {
+ score = num;
+ }
- rowObject.options.set(key, value);
- } else {
- // Positional argument
- // Here again, the Lua code evaluates arguments at index 1 through 5
- // as #expr expressions, but we just pass them through.
- if (positionalIndex < order.length) {
- rowObject[order[positionalIndex]] = parameter;
- }
+ return {
+ team: obj[`rd${round}-team${team}`],
+ score: score,
+ seed: obj[`rd${round}-seed${team}`]
+ };
+ }; // https://en.wikipedia.org/wiki/Category:Tournament_bracket_templates
+ //these are weird.
- positionalIndex++;
- }
- }
- for (; positionalIndex < order.length; positionalIndex++) {
- rowObject[order[positionalIndex]] = null;
+ const playoffBracket = function (tmpl) {
+ let rounds = [];
+ let obj = toJSON(tmpl); //try some rounds
+
+ for (let i = 1; i < 7; i += 1) {
+ let round = [];
+
+ for (let t = 1; t < 16; t += 2) {
+ let key = `rd${i}-team`;
+
+ if (obj[key + t] || obj[key + zeroPad(t)]) {
+ let one = parseTeam(obj, i, t);
+ let two = parseTeam(obj, i, t + 1);
+ round.push([one, two]);
+ } else {
+ break;
}
+ }
- return rowObject;
- });
- obj.data = dataArray;
- list.push(obj);
- return '';
- },
- 'medical cases chart/row': function medicalCasesChartRow(tmpl) {
- // Deprecated template; we keep it.
- return tmpl;
+ if (round.length > 0) {
+ rounds.push(round);
+ }
}
+
+ return {
+ template: 'playoffbracket',
+ rounds: rounds
+ };
};
- var science = templates$a;
- var sports = {
- player: function player(tmpl, list) {
- var res = parse$3(tmpl, ['number', 'country', 'name', 'dl']);
+ var _lib = playoffBracket;
+
+ let sports = {
+ //playoff brackets
+ '4teambracket': function (tmpl, list) {
+ let obj = _lib(tmpl);
+ list.push(obj);
+ return '';
+ },
+ player: (tmpl, list) => {
+ let res = toJSON(tmpl, ['number', 'country', 'name', 'dl']);
list.push(res);
- var str = "[[".concat(res.name, "]]");
+ let str = `[[${res.name}]]`;
if (res.country) {
- var country = (res.country || '').toLowerCase();
- var flag = flags.find(function (a) {
- return country === a[1] || country === a[2];
- }) || [];
+ let country = (res.country || '').toLowerCase();
+ let flag = flags.find(a => country === a[1] || country === a[2]) || [];
if (flag && flag[0]) {
str = flag[0] + ' ' + str;
@@ -7562,15 +7429,15 @@
return str;
},
//https://en.wikipedia.org/wiki/Template:Goal
- goal: function goal(tmpl, list) {
- var res = parse$3(tmpl);
- var obj = {
+ goal: (tmpl, list) => {
+ let res = toJSON(tmpl);
+ let obj = {
template: 'goal',
data: []
};
- var arr = res.list || [];
+ let arr = res.list || [];
- for (var i = 0; i < arr.length; i += 2) {
+ for (let i = 0; i < arr.length; i += 2) {
obj.data.push({
min: arr[i],
note: arr[i + 1] || ''
@@ -7579,1143 +7446,1970 @@
list.push(obj); //generate a little text summary
- var summary = '⚽ ';
- summary += obj.data.map(function (o) {
- var note = o.note;
+ let summary = '⚽ ';
+ summary += obj.data.map(o => {
+ let note = o.note;
if (note) {
- note = " (".concat(note, ")");
+ note = ` (${note})`;
}
return o.min + "'" + note;
}).join(', ');
return summary;
},
- //yellow card
- yel: function yel(tmpl, list) {
- var obj = parse$3(tmpl, ['min']);
- list.push(obj);
+ //a transcluded sports module - https://en.m.wikipedia.org/w/index.php?title=Special:WhatLinksHere/Module:Sports_table
+ // https://en.wikipedia.org/wiki/Template:2020–21_NHL_North_Division_standings
+ 'sports table': (tmpl, list) => {
+ let obj = toJSON(tmpl);
+ let byTeam = {};
+ let teams = Object.keys(obj).filter(k => /^team[0-9]/.test(k)).map(k => obj[k].toLowerCase());
+ teams.forEach(team => {
+ byTeam[team] = {
+ name: obj[`name_${team}`],
+ win: Number(obj[`win_${team}`]) || 0,
+ loss: Number(obj[`loss_${team}`]) || 0,
+ tie: Number(obj[`tie_${team}`]) || 0,
+ otloss: Number(obj[`otloss_${team}`]) || 0,
+ goals_for: Number(obj[`gf_${team}`]) || 0,
+ goals_against: Number(obj[`ga_${team}`]) || 0
+ };
+ });
+ let res = {
+ date: obj.update,
+ header: obj.table_header,
+ teams: byTeam
+ };
+ list.push(res);
+ }
+ };
+ var sports_1 = sports;
- if (obj.min) {
- return "yellow: ".concat(obj.min || '', "'"); //no yellow-card emoji
+ var textAndData = Object.assign({}, shorthand, functions, currency, dates, geo, misc_1, stockExchanges, _lib, sports_1, _lib, sports_1);
+
+ let templates = Object.assign({}, textOnly, dataOnly, textAndData);
+ Object.keys(aliases_1).forEach(k => {
+ // if (templates[aliases[k]] === undefined) {
+ // console.error(`Missing template: '${aliases[k]}'`)
+ // }
+ templates[k] = templates[aliases_1[k]];
+ });
+ var custom = templates; // console.log(Object.keys(templates).length)
+
+ const {
+ isArray: isArray$1,
+ isObject
+ } = helpers;
+ const nums = ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9']; //this gets all the {{template}} objects and decides how to parse them
+
+ const parseTemplate = function (tmpl, doc) {
+ let name = tmpl.name; // dont bother with some junk templates
+
+ if (_ignore.hasOwnProperty(name) === true) {
+ return [''];
+ } //{{infobox settlement...}}
+
+
+ if (_infobox.isInfobox(name) === true) {
+ let obj = toJSON(tmpl.body, [], 'raw'); // list.push(infobox.format(obj))
+
+ return ['', _infobox.format(obj)];
+ } //cite book, cite arxiv...
+
+
+ if (/^cite [a-z]/.test(name) === true) {
+ let obj = toJSON(tmpl.body);
+ obj.type = obj.template;
+ obj.template = 'citation'; // list.push(obj)
+
+ return ['', obj];
+ } // ok, here we go!
+ //parse some known templates
+
+
+ if (custom.hasOwnProperty(name) === true) {
+ //handle number-syntax
+ if (typeof custom[name] === 'number') {
+ let obj = toJSON(tmpl.body, nums);
+ let key = String(custom[name]);
+ return [obj[key] || ''];
+ } //handle string-syntax
+
+
+ if (typeof custom[name] === 'string') {
+ return [custom[name]];
+ } //handle array sytax
+
+
+ if (isArray$1(custom[name]) === true) {
+ let obj = toJSON(tmpl.body, custom[name]); // list.push(obj)
+
+ return ['', obj];
+ } //handle object sytax
+
+
+ if (isObject(custom[name]) === true) {
+ let obj = toJSON(tmpl.body, custom[name].props); // list.push(obj)
+
+ return [obj[custom[name].out], obj];
+ } //handle function syntax
+
+
+ if (typeof custom[name] === 'function') {
+ // let json = toJSON(tmpl.body)
+ //(tmpl, list, alias, doc)
+ let arr = [];
+ let txt = custom[name](tmpl.body, arr, toJSON, null, doc);
+ return [txt, arr[0]];
}
+ } //an unknown template with data, so just keep it.
- return '';
+
+ let json = toJSON(tmpl.body);
+
+ if (Object.keys(json).length === 0) {
+ // list.push(json)
+ json = null;
+ } //..then remove it
+
+
+ return ['', json];
+ };
+
+ var parse$3 = parseTemplate;
+
+ const toJson = function (infobox, options) {
+ let json = Object.keys(infobox.data).reduce((h, k) => {
+ if (infobox.data[k]) {
+ h[k] = infobox.data[k].json();
+ }
+
+ return h;
+ }, {}); //support mongo-encoding keys
+
+ if (options.encode === true) {
+ json = encode.encodeObj(json);
+ }
+
+ return json;
+ };
+
+ var toJson_1 = toJson;
+
+ const {
+ isArray
+ } = helpers;
+
+ const normalize = (str = '') => {
+ str = str.toLowerCase();
+ str = str.replace(/[-_]/g, ' ');
+ return str.trim();
+ }; //a formal key-value data table about a topic
+
+
+ const Infobox = function (obj, wiki) {
+ this._type = obj.type;
+ this.domain = obj.domain;
+ Object.defineProperty(this, 'data', {
+ enumerable: false,
+ value: obj.data
+ });
+ Object.defineProperty(this, 'wiki', {
+ enumerable: false,
+ value: wiki
+ });
+ };
+
+ const methods$1 = {
+ type: function () {
+ return this._type;
},
- subon: function subon(tmpl, list) {
- var obj = parse$3(tmpl, ['min']);
- list.push(obj);
+ links: function (n) {
+ let arr = [];
+ Object.keys(this.data).forEach(k => {
+ this.data[k].links().forEach(l => arr.push(l));
+ });
- if (obj.min) {
- return "sub on: ".concat(obj.min || '', "'"); //no yellow-card emoji
+ if (typeof n === 'string') {
+ //grab a link like .links('Fortnight')
+ n = n.charAt(0).toUpperCase() + n.substring(1); //titlecase it
+
+ let link = arr.find(o => o.page() === n);
+ return link === undefined ? [] : [link];
}
- return '';
+ return arr;
},
- suboff: function suboff(tmpl, list) {
- var obj = parse$3(tmpl, ['min']);
- list.push(obj);
+ image: function () {
+ let s = this.data.image || this.data.image2 || this.data.logo || this.data.image_skyline || this.data.image_flag;
- if (obj.min) {
- return "sub off: ".concat(obj.min || '', "'"); //no yellow-card emoji
+ if (!s) {
+ return null;
}
+ let obj = s.json();
+ obj.file = obj.text;
+ obj.text = '';
+ obj.domain = this.domain; // add domain information for image
+
+ return new Image_1(obj);
+ },
+ get: function (keys) {
+ let allKeys = Object.keys(this.data);
+
+ if (typeof keys === 'string') {
+ let key = normalize(keys);
+
+ for (let i = 0; i < allKeys.length; i += 1) {
+ let tmp = normalize(allKeys[i]);
+
+ if (key === tmp) {
+ return this.data[allKeys[i]];
+ }
+ }
+
+ return new Sentence_1();
+ }
+
+ if (isArray(keys)) {
+ // support array-input
+ keys = keys.map(normalize);
+ return keys.map(k => {
+ for (let i = 0; i < allKeys.length; i += 1) {
+ let tmp = normalize(allKeys[i]);
+
+ if (k === tmp) {
+ return this.data[allKeys[i]];
+ }
+ }
+
+ return new Sentence_1();
+ });
+ }
+
+ return new Sentence_1();
+ },
+ text: function () {
return '';
},
- pengoal: function pengoal(tmpl, list) {
- list.push({
- template: 'pengoal'
- });
- return '✅';
+ json: function (options) {
+ options = options || {};
+ return toJson_1(this, options);
},
- penmiss: function penmiss(tmpl, list) {
- list.push({
- template: 'penmiss'
- });
- return '❌';
+ wikitext: function () {
+ return this.wiki || '';
},
- //'red' card - {{sent off|cards|min1|min2}}
- 'sent off': function sentOff(tmpl, list) {
- var obj = parse$3(tmpl, ['cards']);
- var result = {
- template: 'sent off',
- cards: obj.cards,
- minutes: obj.list || []
- };
- list.push(result);
- var mins = result.minutes.map(function (m) {
- return m + "'";
- }).join(', ');
- return 'sent off: ' + mins;
+ keyValue: function () {
+ return Object.keys(this.data).reduce((h, k) => {
+ if (this.data[k]) {
+ h[k] = this.data[k].text();
+ }
+
+ return h;
+ }, {});
}
- };
- var soccer = sports;
+ }; //aliases
- var misc$3 = {
- 'baseball secondary style': 0,
- mlbplayer: function mlbplayer(tmpl, list) {
- var obj = parse$3(tmpl, ['number', 'name', 'dl']);
- list.push(obj);
- return obj.name;
+ Object.keys(methods$1).forEach(k => {
+ Infobox.prototype[k] = methods$1[k];
+ });
+ Infobox.prototype.data = Infobox.prototype.keyValue;
+ Infobox.prototype.template = Infobox.prototype.type;
+ Infobox.prototype.images = Infobox.prototype.image;
+ var Infobox_1 = Infobox;
+
+ const toText = _04Sentence.fromText;
+ const methods = {
+ text: function () {
+ let str = this._text || '';
+ return toText(str).text();
+ },
+ json: function () {
+ return this.data || {};
+ },
+ wikitext: function () {
+ return this.wiki || '';
}
};
- var sports$1 = Object.assign({}, misc$3, brackets_1, soccer);
- var codes$1 = {
- adx: 'adx',
- //https://en.wikipedia.org/wiki/Template:Abu_Dhabi_Securities_Exchange
- aim: 'aim',
- //https://en.wikipedia.org/wiki/Template:Alternative_Investment_Market
- bvpasa: 'bvpasa',
- //https://en.wikipedia.org/wiki/Template:BVPASA
- asx: 'asx',
- //https://en.wikipedia.org/wiki/Template:Australian_Securities_Exchange
- athex: 'athex',
- //https://en.wikipedia.org/wiki/Template:Athens_Exchange
- bhse: 'bhse',
- //https://en.wikipedia.org/wiki/Template:Bahrain_Bourse
- bvb: 'bvb',
- //https://en.wikipedia.org/wiki/Template:Bucharest_Stock_Exchange
- bbv: 'bbv',
- //https://en.wikipedia.org/wiki/Template:BBV
- bsx: 'bsx',
- //https://en.wikipedia.org/wiki/Template:Bermuda_Stock_Exchange
- b3: 'b3',
- //https://en.wikipedia.org/wiki/Template:BM%26F_Bovespa
- 'bm&f': 'b3',
- //https://en.wikipedia.org/wiki/Template:BM%26F_Bovespa
- 'bm&f bovespa': 'b3',
- //https://en.wikipedia.org/wiki/Template:BM%26F_Bovespa
- bwse: 'bwse',
- //https://en.wikipedia.org/wiki/Template:Botswana_Stock_Exchange
- 'botswana stock exchange': 'botswana stock exchange',
- //https://en.wikipedia.org/wiki/Template:BM%26F_Bovespa
- bse: 'bse',
- //https://en.wikipedia.org/wiki/Template:Bombay_Stock_Exchange
- 'bombay stock exchange': 'bombay stock exchange',
- //https://en.wikipedia.org/wiki/Template:Bombay_Stock_Exchange
- bpse: 'bpse',
- //https://en.wikipedia.org/wiki/Template:Budapest_Stock_Exchange
- bcba: 'bcba',
- //https://en.wikipedia.org/wiki/Template:Buenos_Aires_Stock_Exchange
- 'canadian securities exchange': 'canadian securities exchange',
- //https://en.wikipedia.org/wiki/Template:Canadian_Securities_Exchange
- bvc: 'bvc',
- //https://en.wikipedia.org/wiki/Template:Colombian_Securities_Exchange
- cse: 'cse',
- //https://en.wikipedia.org/wiki/Template:Chittagong_Stock_Exchange
- darse: 'darse',
- //https://en.wikipedia.org/wiki/Template:Dar_es_Salaam_Stock_Exchange
- dse: 'dse',
- //https://en.wikipedia.org/wiki/Template:Dhaka_Stock_Exchange
- dfm: 'dfm',
- //https://en.wikipedia.org/wiki/Template:Dubai_Financial_Market
- euronext: 'euronext',
- //https://en.wikipedia.org/wiki/Template:Euronext
- fwb: 'fwb',
- //https://en.wikipedia.org/wiki/Template:Frankfurt_Stock_Exchange
- fse: 'fse',
- //https://en.wikipedia.org/wiki/Template:Fukuoka_Stock_Exchange
- gse: 'gse',
- //https://en.wikipedia.org/wiki/Template:Ghana_Stock_Exchange
- gtsm: 'gtsm',
- //https://en.wikipedia.org/wiki/Template:Gre_Tai_Securities_Market
- sehk: 'sehk',
- //https://en.wikipedia.org/wiki/Template:Hong_Kong_Stock_Exchange
- idx: 'idx',
- //https://en.wikipedia.org/wiki/Template:Indonesia_Stock_Exchange
- nse: 'nse',
- //https://en.wikipedia.org/wiki/Template:National_Stock_Exchange_of_India
- ise: 'ise',
- //https://en.wikipedia.org/wiki/Template:Irish_Stock_Exchange
- isin: 'isin',
- //https://en.wikipedia.org/wiki/Template:ISIN
- bist: 'bist',
- //https://en.wikipedia.org/wiki/Template:Borsa_Istanbul
- bit: 'bit',
- //https://en.wikipedia.org/wiki/Template:Borsa_Italiana
- jasdaq: 'jasdaq',
- //https://en.wikipedia.org/wiki/Template:JASDAQ
- jse: 'jse',
- //https://en.wikipedia.org/wiki/Template:Johannesburg_Stock_Exchange
- kase: 'kase',
- //https://en.wikipedia.org/wiki/Template:Kazakhstan_Stock_Exchange
- krx: 'krx',
- //https://en.wikipedia.org/wiki/Template:Korea_Exchange
- bvl: 'bvl',
- //https://en.wikipedia.org/wiki/Template:Lima_Stock_Exchange
- lse: 'lse',
- //https://en.wikipedia.org/wiki/Template:London_Stock_Exchange
- luxse: 'luxse',
- //https://en.wikipedia.org/wiki/Template:Luxembourg_Stock_Exchange
- bmad: 'bmad',
- //https://en.wikipedia.org/wiki/Template:Bolsa_de_Madrid
- myx: 'myx',
- //https://en.wikipedia.org/wiki/Template:Bursa_Malaysia
- bmv: 'bmv',
- //https://en.wikipedia.org/wiki/Template:Mexican_Stock_Exchange
- mcx: 'mcx',
- //https://en.wikipedia.org/wiki/Template:Moscow_Exchange
- mutf: 'mutf',
- //https://en.wikipedia.org/wiki/Template:Mutual_fund
- nag: 'nag',
- //https://en.wikipedia.org/wiki/Template:Nagoya_Stock_Exchange
- kn: 'kn',
- //https://en.wikipedia.org/wiki/Template:Nairobi_Securities_Exchange
- 'nasdaq dubai': 'nasdaq dubai',
- //https://en.wikipedia.org/wiki/Template:NASDAQ_Dubai
- nasdaq: 'nasdaq',
- //https://en.wikipedia.org/wiki/Template:NASDAQ
- neeq: 'neeq',
- //https://en.wikipedia.org/wiki/Template:NEEQ
- nepse: 'nepse',
- //https://en.wikipedia.org/wiki/Template:Nepal_Stock_Exchange
- nyse: 'nyse',
- //https://en.wikipedia.org/wiki/Template:New_York_Stock_Exchange
- nzx: 'nzx',
- //https://en.wikipedia.org/wiki/Template:New_Zealand_Exchange
- amex: 'amex',
- //https://en.wikipedia.org/wiki/Template:NYSE_American
- 'nyse arca': 'nyse arca',
- //https://en.wikipedia.org/wiki/Template:NYSE_Arca
- omx: 'omx',
- //https://en.wikipedia.org/wiki/Template:OMX
- 'omx baltic': 'omx baltic',
- //https://en.wikipedia.org/wiki/Template:OMX_Baltic
- ose: 'ose',
- //https://en.wikipedia.org/wiki/Template:Oslo_Stock_Exchange
- 'otc pink': 'otc pink',
- //https://en.wikipedia.org/wiki/Template:OTC_Pink
- otcqb: 'otcqb',
- //https://en.wikipedia.org/wiki/Template:OTCQB
- otcqx: 'otcqx',
- //https://en.wikipedia.org/wiki/Template:OTCQX
- 'philippine stock exchange': 'philippine stock exchange',
- //https://en.wikipedia.org/wiki/Template:Philippine_Stock_Exchange
- prse: 'prse',
- //https://en.wikipedia.org/wiki/Template:Prague_Stock_Exchange
- qe: 'qe',
- //https://en.wikipedia.org/wiki/Template:Qatar_Stock_Exchange
- bcs: 'bcs',
- //https://en.wikipedia.org/wiki/Template:Santiago_Stock_Exchange
- 'saudi stock exchange': 'saudi stock exchange',
- //https://en.wikipedia.org/wiki/Template:Saudi_Stock_Exchange
- sgx: 'sgx',
- //https://en.wikipedia.org/wiki/Template:Singapore_Exchange
- sse: 'sse',
- //https://en.wikipedia.org/wiki/Template:Shanghai_Stock_Exchange
- szse: 'szse',
- //https://en.wikipedia.org/wiki/Template:Shenzhen_Stock_Exchange
- swx: 'swx',
- //https://en.wikipedia.org/wiki/Template:SIX_Swiss_Exchange
- set: 'set',
- //https://en.wikipedia.org/wiki/Template:Stock_Exchange_of_Thailand
- tase: 'tase',
- //https://en.wikipedia.org/wiki/Template:Tel_Aviv_Stock_Exchange
- tyo: 'tyo',
- //https://en.wikipedia.org/wiki/Template:Tokyo_Stock_Exchange
- tsx: 'tsx',
- //https://en.wikipedia.org/wiki/Template:Toronto_Stock_Exchange
- twse: 'twse',
- //https://en.wikipedia.org/wiki/Template:Taiwan_Stock_Exchange
- 'tsx-v': 'tsx-v',
- //https://en.wikipedia.org/wiki/Template:TSX_Venture_Exchange
- tsxv: 'tsxv',
- //https://en.wikipedia.org/wiki/Template:TSX_Venture_Exchange
- nex: 'nex',
- //https://en.wikipedia.org/wiki/Template:TSXV_NEX
- ttse: 'ttse',
- //https://en.wikipedia.org/wiki/Template:Trinidad_and_Tobago_Stock_Exchange
- 'pfts ukraine stock exchange': 'pfts ukraine stock exchange',
- //https://en.wikipedia.org/wiki/Template:PFTS_Ukraine_Stock_Exchange
- wse: 'wse',
- //https://en.wikipedia.org/wiki/Template:Warsaw_Stock_Exchange
- wbag: 'wbag',
- //https://en.wikipedia.org/wiki/Template:Wiener_B%C3%B6rse
- zse: 'zse',
- //https://en.wikipedia.org/wiki/Template:Zagreb_Stock_Exchange
- 'zagreb stock exchange': 'zagreb stock exchange',
- //https://en.wikipedia.org/wiki/Template:Zagreb_Stock_Exchange
- 'zimbabwe stock exchange': 'zimbabwe stock exchange' //https://en.wikipedia.org/wiki/Template:Zimbabwe_Stock_Exchange
+ const Template = function (data, text = '', wiki = '') {
+ Object.defineProperty(this, 'data', {
+ enumerable: false,
+ value: data
+ });
+ Object.defineProperty(this, '_text', {
+ enumerable: false,
+ value: text
+ });
+ Object.defineProperty(this, 'wiki', {
+ enumerable: false,
+ value: wiki
+ });
+ };
+
+ Object.keys(methods).forEach(k => {
+ Template.prototype[k] = methods[k];
+ });
+ var Template_1 = Template;
+
+ const isCitation = /^(cite |citation)/i;
+ const referenceTypes = {
+ citation: true,
+ refn: true,
+ harvnb: true,
+ source: true //wikinews
+
+ }; // split Infoboxes from templates and references
+
+ const sortOut = function (list, domain) {
+ let res = {
+ infoboxes: [],
+ templates: [],
+ references: []
+ }; //remove references and infoboxes from our list
+
+ list.forEach(obj => {
+ let json = obj.json;
+ let kind = json.template || json.type || json.name; // is it a Reference?
+
+ if (referenceTypes[kind] === true || isCitation.test(kind) === true) {
+ res.references.push(new Reference_1(json, obj.wiki));
+ return;
+ } // is it an Infobox?
+
+
+ if (json.template === 'infobox' && json.subbox !== 'yes') {
+ json.domain = domain; //infoboxes need this for images, i guess
+
+ json.data = json.data || {}; //validate it a little
+
+ res.infoboxes.push(new Infobox_1(json, obj.wiki));
+ return;
+ } // otherwise, it's just a template
+
+
+ res.templates.push(new Template_1(json, obj.text, obj.wiki));
+ });
+ return res;
+ };
+
+ var sortOut_1 = sortOut;
+
+ const allTemplates = function (wiki, doc) {
+ let list = []; //nested data-structure of templates
+
+ let nested = _01Nested(wiki); //recursive template-parser
+
+ const parseNested = function (obj, parent) {
+ obj.parent = parent; //do tail-first recursion
+
+ if (obj.children && obj.children.length > 0) {
+ obj.children.forEach(ch => parseNested(ch, obj));
+ } //parse template into json, return replacement wikitext
+
+
+ let [text, json] = parse$3(obj, doc);
+ obj.wiki = text;
+
+ if (json) {
+ list.push({
+ name: obj.name,
+ wiki: obj.body,
+ text: text,
+ json: json
+ });
+ } //remove the text from every parent
+
+
+ const removeIt = function (node, body, out) {
+ if (node.parent) {
+ node.parent.body = node.parent.body.replace(body, out);
+ removeIt(node.parent, body, out);
+ }
+ };
+
+ removeIt(obj, obj.body, obj.wiki);
+ wiki = wiki.replace(obj.body, obj.wiki);
+ }; //kick it off
+
+
+ nested.forEach(node => parseNested(node, null)); //remove the templates from our wiki text
+
+ nested.forEach(node => {
+ wiki = wiki.replace(node.body, node.wiki);
+ });
+ return {
+ list: list,
+ wiki: wiki
+ };
+ }; //find + parse all templates in the section
+
+
+ const process = function (section, doc) {
+ // find+parse them all
+ let {
+ list,
+ wiki
+ } = allTemplates(section._wiki, doc); // split-out references and infoboxes
+
+ let domain = doc ? doc._domain : null;
+ let {
+ infoboxes,
+ references,
+ templates
+ } = sortOut_1(list, domain); //sort-out the templates we decide to keep
+
+ section._infoboxes = section._infoboxes || [];
+ section._references = section._references || [];
+ section._templates = section._templates || [];
+ section._infoboxes = section._infoboxes.concat(infoboxes);
+ section._references = section._references.concat(references);
+ section._templates = section._templates.concat(templates);
+ section._wiki = wiki;
+ };
+
+ var template = process;
+
+ const parseSentence$1 = _04Sentence.fromText; //okay, is a xml-tag, with newline-separated data, somehow pivoted by '|'...
+ //all deities help us. truly -> https://en.wikipedia.org/wiki/Help:Gallery_tag
+ //- not to be confused with https://en.wikipedia.org/wiki/Template:Gallery...
+
+ /**
+ *
+ * @private
+ * @param {object} catcher
+ * @param {Document} doc
+ * @param {Section} section
+ */
+
+ const parseGallery = function (catcher, doc, section) {
+ catcher.text = catcher.text.replace(/]*?)>([\s\S]+?)<\/gallery>/g, (_, attrs, inside) => {
+ let images = inside.split(/\n/g);
+ images = images.filter(str => str && str.trim() !== ''); //parse the line, which has an image and sometimes a caption
+
+ images = images.map(str => {
+ let arr = str.split(/\|/);
+ let obj = {
+ file: arr[0].trim(),
+ lang: doc.lang(),
+ domain: doc.domain()
+ };
+ let img = new Image_1(obj).json();
+ let caption = arr.slice(1).join('|');
+
+ if (caption !== '') {
+ img.caption = parseSentence$1(caption);
+ }
+
+ return img;
+ }); //add it to our templates list
+
+ if (images.length > 0) {
+ catcher.templates.push({
+ template: 'gallery',
+ images: images,
+ pos: section.title
+ });
+ } //return empty string to remove the template from the wiki text
+
+
+ return '';
+ });
+ };
+
+ var gallery = parseGallery;
+
+ /**
+ * parses out the `Election_box` template from the wiki text
+ *
+ * this is a non-traditional template, for some reason
+ * https://en.wikipedia.org/wiki/Template:Election_box
+ *
+ * @private
+ * @param {object} catcher an object to provide and catch data
+ */
+
+ const parseElection = function (catcher) {
+ catcher.text = catcher.text.replace(/\{\{election box begin([\s\S]+?)\{\{election box end\}\}/gi, tmpl => {
+ let data = {
+ _wiki: tmpl,
+ _templates: []
+ }; //put it through our full template parser..
+
+ template(data); //okay, pull it apart into something sensible..
+
+ let templates = data._templates.map(t => t.json());
+
+ let start = templates.find(t => t.template === 'election box') || {};
+ let candidates = templates.filter(t => t.template === 'election box candidate');
+ let summary = templates.find(t => t.template === 'election box gain' || t.template === 'election box hold') || {};
+ if (candidates.length > 0 || summary) {
+ catcher.templates.push({
+ template: 'election box',
+ title: start.title,
+ candidates: candidates,
+ summary: summary.data
+ });
+ } //return empty string to remove the template from the wiki text
+
+
+ return '';
+ });
};
- var parseStockExchange = function parseStockExchange(tmpl, list) {
- var o = parse$3(tmpl, ['ticketnumber', 'code']);
- list.push(o);
- var code = o.template || '';
+ var election = parseElection;
- if (code === '') {
- code = o.code;
- }
+ const keys = {
+ coach: ['team', 'year', 'g', 'w', 'l', 'w-l%', 'finish', 'pg', 'pw', 'pl', 'pw-l%'],
+ player: ['year', 'team', 'gp', 'gs', 'mpg', 'fg%', '3p%', 'ft%', 'rpg', 'apg', 'spg', 'bpg', 'ppg'],
+ roster: ['player', 'gp', 'gs', 'mpg', 'fg%', '3fg%', 'ft%', 'rpg', 'apg', 'spg', 'bpg', 'ppg']
+ };
+ /**
+ * https://en.wikipedia.org/wiki/Template:NBA_player_statistics_start
+ *
+ * @private
+ * @param {object} catcher
+ */
- code = (code || '').toLowerCase();
- var out = codes$1[code] || '';
- var str = out;
+ const parseNBA = function (catcher) {
+ catcher.text = catcher.text.replace(/\{\{nba (coach|player|roster) statistics start([\s\S]+?)\{\{s-end\}\}/gi, (tmpl, name) => {
+ tmpl = tmpl.replace(/^\{\{.*?\}\}/, '');
+ tmpl = tmpl.replace(/\{\{s-end\}\}/, '');
+ name = name.toLowerCase().trim();
+ let headers = '! ' + keys[name].join(' !! ');
+ let table = '{|\n' + headers + '\n' + tmpl + '\n|}';
+ let rows = parse$5(table);
+ rows = rows.map(row => {
+ Object.keys(row).forEach(k => {
+ row[k] = row[k].text();
+ });
+ return row;
+ });
+ catcher.templates.push({
+ template: 'NBA ' + name + ' statistics',
+ data: rows
+ }); //return empty string to remove the template from the wiki text
- if (o.ticketnumber) {
- str = "".concat(str, ": ").concat(o.ticketnumber);
+ return '';
+ });
+ };
+
+ var nba = parseNBA;
+
+ //this is pretty nuts
+
+ const whichHeadings = function (tmpl) {
+ let headings = ['#', 'date', 'opponent', 'score', 'win', 'loss', 'save', 'attendance', 'record'];
+
+ if (/\|stadium=y/i.test(tmpl) === true) {
+ headings.splice(7, 0, 'stadium'); //save, stadium, attendance
}
- if (o.code && !codes$1[o.code.toLowerCase()]) {
- str += ' ' + o.code;
+ if (/\|time=y/i.test(tmpl) === true) {
+ headings.splice(7, 0, 'time'); //save, time, stadium, attendance
}
- return str;
+ if (/\|box=y/i.test(tmpl) === true) {
+ headings.push('box'); //record, box
+ }
+
+ return headings;
};
+ /**
+ *
+ * @private
+ * @param {object} catcher
+ */
- var currencies$1 = {}; //the others fit the same pattern..
- Object.keys(codes$1).forEach(function (k) {
- currencies$1[k] = parseStockExchange;
- });
- var stockExchanges = currencies$1;
+ const parseMlb = function (catcher) {
+ catcher.text = catcher.text.replace(/\{\{mlb game log (section|month)[\s\S]+?\{\{mlb game log (section|month) end\}\}/gi, tmpl => {
+ let headings = whichHeadings(tmpl);
+ tmpl = tmpl.replace(/^\{\{.*?\}\}/, '');
+ tmpl = tmpl.replace(/\{\{mlb game log (section|month) end\}\}/i, '');
+ let headers = '! ' + headings.join(' !! ');
+ let table = '{|\n' + headers + '\n' + tmpl + '\n|}';
+ let rows = parse$5(table);
+ rows = rows.map(row => {
+ Object.keys(row).forEach(k => {
+ row[k] = row[k].text();
+ });
+ return row;
+ });
+ catcher.templates.push({
+ template: 'mlb game log section',
+ data: rows
+ }); //return empty string to remove the template from the wiki text
- var hasMonth = /^jan /i;
- var isYear = /^year /i;
- var monthList = ['jan', 'feb', 'mar', 'apr', 'may', 'jun', 'jul', 'aug', 'sep', 'oct', 'nov', 'dec'];
+ return '';
+ });
+ };
- var toNumber = function toNumber(str) {
- str = str.replace(/,/g, '');
- str = str.replace(/−/g, '-');
- var num = Number(str);
+ var mlb = parseMlb;
- if (isNaN(num)) {
- return str;
- }
+ let headings = ['res', 'record', 'opponent', 'method', 'event', 'date', 'round', 'time', 'location', 'notes'];
+ /**
+ *
+ * https://en.wikipedia.org/wiki/Template:MMA_record_start
+ *
+ * @private
+ * @param {object} catcher
+ */
+
+ const parseMMA = function (catcher) {
+ catcher.text = catcher.text.replace(/\{\{mma record start[\s\S]+?\{\{end\}\}/gi, tmpl => {
+ tmpl = tmpl.replace(/^\{\{.*?\}\}/, '');
+ tmpl = tmpl.replace(/\{\{end\}\}/i, '');
+ let headers = '! ' + headings.join(' !! ');
+ let table = '{|\n' + headers + '\n' + tmpl + '\n|}';
+ let rows = parse$5(table);
+ rows = rows.map(row => {
+ Object.keys(row).forEach(k => {
+ row[k] = row[k].text();
+ });
+ return row;
+ });
+ catcher.templates.push({
+ template: 'mma record start',
+ data: rows
+ }); //return empty string to remove the template from the wiki text
- return num;
+ return '';
+ });
};
- var templates$b = {
- // this one is a handful!
- //https://en.wikipedia.org/wiki/Template:Weather_box
- 'weather box': function weatherBox(tmpl, list) {
- var obj = parse$3(tmpl); //collect all month-based data
+ var mma = parseMMA;
+
+ const parseSentence = _04Sentence.fromText;
+ /**
+ * try to parse out the math and chem templates
+ *
+ * xml support
+ * https://en.wikipedia.org/wiki/Help:Displaying_a_formula
+ *
+ * @private
+ * @param {object} catcher
+ */
+
+ const parseMath = function (catcher) {
+ catcher.text = catcher.text.replace(/]