diff --git a/Includes/Classroom-Setup.py b/Includes/Classroom-Setup.py
index e5ac63a..0b86399 100644
--- a/Includes/Classroom-Setup.py
+++ b/Includes/Classroom-Setup.py
@@ -5,11 +5,13 @@
DA = DBAcademyHelper(course_config, lesson_config) # Create the DA object
DA.reset_lesson() # Reset the lesson to a clean state
-DA.init() # Performs basic initialization including creating schemas and catalogs
+DA.init() # Performs basic intialization including creating schemas and catalogs
DA.paths.working_dir = DA.paths.to_vm_path(DA.paths.working_dir)
DA.paths.datasets = DA.paths.to_vm_path(DA.paths.datasets)
+DA.init_mlflow_as_job()
+
# COMMAND ----------
# MAGIC %run ./Test-Framework
@@ -24,8 +26,11 @@
import torch
+TORCH_DEVICE = "cpu"
+NO_CUDA = True
if torch.cuda.is_available():
- torch.device("cuda")
-else:
- torch.device("cpu")
+ NO_CUDA = False
+ TORCH_DEVICE = "cuda"
+
+torch.device(TORCH_DEVICE)
diff --git a/LLM 00 - Introduction/LLM 00a - Install Datasets.py b/LLM 00 - Introduction/LLM 00a - Install Datasets.py
new file mode 100644
index 0000000..cb3ab65
--- /dev/null
+++ b/LLM 00 - Introduction/LLM 00a - Install Datasets.py
@@ -0,0 +1,28 @@
+# Databricks notebook source
+# MAGIC %md-sandbox
+# MAGIC
+# MAGIC
+# MAGIC
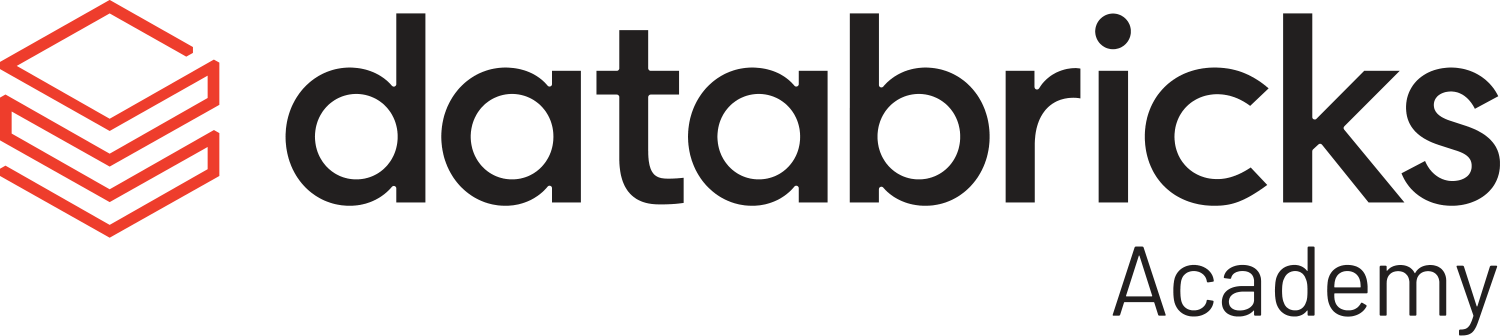
+# MAGIC
+
+# COMMAND ----------
+
+# MAGIC %md # Install Datasets
+# MAGIC
+# MAGIC We need to "install" the datasets this course uses by copying them from their current location in the cloud to a location relative to your workspace.
+# MAGIC
+# MAGIC All that is required is to run the following cell. By default, the **`Classroom-Setup`** script will not reinstall the datasets upon subsequent invocation but this behavior can be adjusted by modifying the parameters below.
+# MAGIC
+# MAGIC Feel free to leave this notebook running and proceed with the course while the install completes in the background.
+
+# COMMAND ----------
+
+# MAGIC %run ../Includes/Classroom-Setup
+
+# COMMAND ----------
+
+# MAGIC %md-sandbox
+# MAGIC © 2023 Databricks, Inc. All rights reserved.
+# MAGIC Apache, Apache Spark, Spark and the Spark logo are trademarks of the Apache Software Foundation.
+# MAGIC
+# MAGIC Privacy Policy | Terms of Use | Support
diff --git a/LLM 02 - PEFT/LLM 02 - Prompt Tuning with PEFT.py b/LLM 02 - PEFT/LLM 02 - Prompt Tuning with PEFT.py
index f1f765a..6ee51b2 100644
--- a/LLM 02 - PEFT/LLM 02 - Prompt Tuning with PEFT.py
+++ b/LLM 02 - PEFT/LLM 02 - Prompt Tuning with PEFT.py
@@ -128,7 +128,7 @@
training_args = TrainingArguments(
output_dir=output_directory, # Where the model predictions and checkpoints will be written
- no_cuda=True, # This is necessary for CPU clusters.
+ no_cuda=NO_CUDA, # This is necessary for CPU clusters.
auto_find_batch_size=True, # Find a suitable batch size that will fit into memory automatically
learning_rate= 3e-2, # Higher learning rate than full fine-tuning
num_train_epochs=5 # Number of passes to go through the entire fine-tuning dataset
@@ -187,7 +187,7 @@
from peft import PeftModel
-loaded_model = PeftModel.from_pretrained(foundation_model,
+loaded_model = PeftModel.from_pretrained(foundation_model.to("cpu"),
peft_model_path,
is_trainable=False)
@@ -257,13 +257,13 @@
# Load model
loaded_text_model = PeftModel.from_pretrained(
- foundation_model,
+ foundation_model.to("cpu"),
text_peft_model_path,
is_trainable=False
)
# Generate output
-text_outputs = text_peft_model.generate(
+text_outputs = loaded_text_model.generate(
input_ids=input1["input_ids"],
attention_mask=input1["attention_mask"],
max_new_tokens=7,
diff --git a/LLM 03 - Deployment of LLMs/LLM 03L - Deployment of LLMs Lab.py b/LLM 03 - Deployment of LLMs/LLM 03L - Deployment of LLMs Lab.py
index 9a5877b..2fad8d5 100644
--- a/LLM 03 - Deployment of LLMs/LLM 03L - Deployment of LLMs Lab.py
+++ b/LLM 03 - Deployment of LLMs/LLM 03L - Deployment of LLMs Lab.py
@@ -33,10 +33,6 @@
# COMMAND ----------
-import torch
-
-# COMMAND ----------
-
# MAGIC %md
# MAGIC # Section 1: An Overview of Mixture-of-Experts (MoE)
# MAGIC Mixture-of-Experts (MoE) is a machine learning architecture that incorporates the idea of "divide and conquer" to solve complex problems. In this approach, the model is composed of multiple individual models, referred to as "experts", each of which specializes in some aspect of the data. The model also includes a "gating" function that determines which expert or combination of experts to consult for a given input.
@@ -64,6 +60,7 @@
# Import the necessary libraries
# transformers is a state-of-the-art library for Natural Language Processing tasks, providing a wide range of pre-trained models
from transformers import GPT2LMHeadModel, GPT2Tokenizer, BertForSequenceClassification, BertTokenizer, T5ForConditionalGeneration, T5Tokenizer
+import torch
# torch.nn.functional provides functions that don't have any parameters, such as activation functions, loss functions etc.
import torch.nn.functional as F
diff --git a/LLM 04 - Multimodal LLMs/LLM 04 - Image Captioning.py b/LLM 04 - Multimodal LLMs/LLM 04 - Image Captioning.py
index 46782cf..2ace584 100644
--- a/LLM 04 - Multimodal LLMs/LLM 04 - Image Captioning.py
+++ b/LLM 04 - Multimodal LLMs/LLM 04 - Image Captioning.py
@@ -181,7 +181,7 @@ def __getitem__(self, idx):
do_train=True,
num_train_epochs=TRAIN_EPOCHS, # number of passes to see the entire dataset
overwrite_output_dir=True,
- no_cuda=True, # Not using GPU
+ no_cuda=NO_CUDA, # Not using GPU when on CPU-only cluster
dataloader_pin_memory=False # this specifies whether you want to pin memory in data loaders or not
)
@@ -224,7 +224,7 @@ def __getitem__(self, idx):
# COMMAND ----------
-caption = tokenizer.decode(trainer.model.generate(feature_extractor(test_image, return_tensors="pt").pixel_values)[0])
+caption = tokenizer.decode(trainer.model.to("cpu").generate(feature_extractor(test_image, return_tensors="pt").pixel_values)[0])
print("--"*20)
print(caption)
diff --git a/Version Info.py b/Version Info.py
index e55966e..1177315 100644
--- a/Version Info.py
+++ b/Version Info.py
@@ -11,8 +11,8 @@
# MAGIC # Project Information
# MAGIC
# MAGIC * Name: **LLM: Foundation Models from the Ground Up**
-# MAGIC * Version: **1.1.0**
-# MAGIC * Built On: **Oct 16, 2023 at 15:48:41 UTC**
+# MAGIC * Version: **2.0.0**
+# MAGIC * Built On: **Nov 14, 2023 at 17:25:05 UTC**
# COMMAND ----------